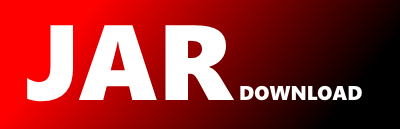
org.openapitools.client.api.QueryRunsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.api;
import org.openapitools.client.ApiCallback;
import org.openapitools.client.ApiClient;
import org.openapitools.client.ApiException;
import org.openapitools.client.model.Headers;
import org.openapitools.client.model.ListQueryParams;
import org.openapitools.client.model.GetByIdQueryParams;
import org.openapitools.client.ApiResponse;
import org.openapitools.client.Configuration;
import org.openapitools.client.Pair;
import org.openapitools.client.ProgressRequestBody;
import org.openapitools.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import java.math.BigDecimal;
import org.openapitools.client.model.ErrorResponse;
import org.openapitools.client.model.QueryRun;
import org.openapitools.client.model.QueryRunCreateRequest;
import org.openapitools.client.model.ErrorResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class QueryRunsApi {
private ApiClient localVarApiClient;
private int localHostIndex;
private String localCustomBaseUrl;
public QueryRunsApi() {
this(Configuration.getDefaultApiClient());
}
public QueryRunsApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public int getHostIndex() {
return localHostIndex;
}
public void setHostIndex(int hostIndex) {
this.localHostIndex = hostIndex;
}
public String getCustomBaseUrl() {
return localCustomBaseUrl;
}
public void setCustomBaseUrl(String customBaseUrl) {
this.localCustomBaseUrl = customBaseUrl;
}
private okhttp3.Call cancelQueryRunCall(String queryRunId, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/query_runs/{query_run_id}/cancel"
.replace("{" + "query_run_id" + "}", localVarApiClient.escapeString(queryRunId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (queryRunFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "query_run.fields[]", queryRunFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call cancelQueryRunValidateBeforeCall(String queryRunId, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'queryRunId' is set
if (queryRunId == null) {
throw new ApiException("Missing the required parameter 'queryRunId' when calling cancelQueryRun(Async)");
}
return cancelQueryRunCall(queryRunId, fields, queryRunFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse cancelQueryRunWithHttpInfo(String queryRunId, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = cancelQueryRunValidateBeforeCall(queryRunId, fields, queryRunFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call cancelQueryRunAsync(String queryRunId, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = cancelQueryRunValidateBeforeCall(queryRunId, fields, queryRunFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class CancelQueryRunParams {
private final String queryRunId;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List queryRunFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public CancelQueryRunParams(String queryRunId) {
this.queryRunId = queryRunId;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return CancelQueryRunParams
*/
public CancelQueryRunParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return CancelQueryRunParams
*/
public CancelQueryRunParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `id`, `sql`, `remaining_attempts`, `updated_time`, `file`, `number_of_rows`, `processing_duration`, `state`, `column_separator` </details> (optional)
* @return CancelQueryRunParams
*/
public CancelQueryRunParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set queryRunFields
* @param queryRunFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `id`, `sql`, `remaining_attempts`, `updated_time`, `file`, `number_of_rows`, `processing_duration`, `state`, `column_separator` </details> (optional)
* @return CancelQueryRunParams
*/
public CancelQueryRunParams queryRunFields(List queryRunFields) {
this.queryRunFields = queryRunFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return CancelQueryRunParams
*/
public CancelQueryRunParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return CancelQueryRunParams
*/
public CancelQueryRunParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return CancelQueryRunParams
*/
public CancelQueryRunParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Cancel a query run
* Cancels a query run. This operation is only applicable if the state of the query run is `accepted` or `in_progress`.
* @param queryRunId query_run_id (required)
* @return QueryRun
* @http.response.details
Status Code Description Response Headers
200 Default Response -
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public CancelQueryRunParams cancelQueryRunParams(String queryRunId) {
return new CancelQueryRunParams(queryRunId);
}
public QueryRun cancelQueryRun(String queryRunId) throws ApiException {
CancelQueryRunParams params = new CancelQueryRunParams(queryRunId);
return executeCancelQueryRunAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse cancelQueryRunWithHttpInfo(String queryRunId) throws ApiException {
CancelQueryRunParams params = new CancelQueryRunParams(queryRunId);
return executeCancelQueryRunAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public QueryRun cancelQueryRun( String queryRunId , List expand) throws ApiException {
CancelQueryRunParams params = new CancelQueryRunParams(queryRunId);
params.expand(expand);
return executeCancelQueryRunAPICall(params).getData();
}
/*
* add header methods
*/
public QueryRun cancelQueryRun(String queryRunId ,List expand,Headers headers) throws ApiException {
CancelQueryRunParams params = new CancelQueryRunParams(queryRunId)
.expand(expand)
.headers(headers);
return executeCancelQueryRunAPICall(params).getData();
}
/**
* Cancel a query run
* Cancels a query run. This operation is only applicable if the state of the query run is `accepted` or `in_progress`.
* @param params CancelQueryRunParams
* @return QueryRun
* @http.response.details
Status Code Description Response Headers
200 Default Response -
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public QueryRun cancelQueryRun(CancelQueryRunParams params) throws ApiException {
return executeCancelQueryRunAPICall(params).getData();
}
public ApiResponse cancelQueryRunWithHttpInfo(CancelQueryRunParams params) throws ApiException {
return executeCancelQueryRunAPICall(params);
}
ApiResponse executeCancelQueryRunAPICall(CancelQueryRunParams params) throws ApiException {
return cancelQueryRunWithHttpInfo(params.queryRunId, params.fields, params.queryRunFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
private okhttp3.Call createQueryRunCall(QueryRunCreateRequest queryRunCreateRequest, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = queryRunCreateRequest;
// create path and map variables
String localVarPath = "/query_runs";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (queryRunFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "query_run.fields[]", queryRunFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call createQueryRunValidateBeforeCall(QueryRunCreateRequest queryRunCreateRequest, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'queryRunCreateRequest' is set
if (queryRunCreateRequest == null) {
throw new ApiException("Missing the required parameter 'queryRunCreateRequest' when calling createQueryRun(Async)");
}
return createQueryRunCall(queryRunCreateRequest, fields, queryRunFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse createQueryRunWithHttpInfo(QueryRunCreateRequest queryRunCreateRequest, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = createQueryRunValidateBeforeCall(queryRunCreateRequest, fields, queryRunFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call createQueryRunAsync(QueryRunCreateRequest queryRunCreateRequest, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = createQueryRunValidateBeforeCall(queryRunCreateRequest, fields, queryRunFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class CreateQueryRunParams {
private final QueryRunCreateRequest queryRunCreateRequest;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List queryRunFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public CreateQueryRunParams(QueryRunCreateRequest queryRunCreateRequest) {
this.queryRunCreateRequest = queryRunCreateRequest;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return CreateQueryRunParams
*/
public CreateQueryRunParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return CreateQueryRunParams
*/
public CreateQueryRunParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `id`, `sql`, `remaining_attempts`, `updated_time`, `file`, `number_of_rows`, `processing_duration`, `state`, `column_separator` </details> (optional)
* @return CreateQueryRunParams
*/
public CreateQueryRunParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set queryRunFields
* @param queryRunFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `id`, `sql`, `remaining_attempts`, `updated_time`, `file`, `number_of_rows`, `processing_duration`, `state`, `column_separator` </details> (optional)
* @return CreateQueryRunParams
*/
public CreateQueryRunParams queryRunFields(List queryRunFields) {
this.queryRunFields = queryRunFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return CreateQueryRunParams
*/
public CreateQueryRunParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return CreateQueryRunParams
*/
public CreateQueryRunParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return CreateQueryRunParams
*/
public CreateQueryRunParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Create a query run
* Creates a new query run job.
* @param queryRunCreateRequest (required)
* @return QueryRun
* @http.response.details
Status Code Description Response Headers
201 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public CreateQueryRunParams createQueryRunParams(QueryRunCreateRequest queryRunCreateRequest) {
return new CreateQueryRunParams(queryRunCreateRequest);
}
public QueryRun createQueryRun(QueryRunCreateRequest queryRunCreateRequest) throws ApiException {
CreateQueryRunParams params = new CreateQueryRunParams(queryRunCreateRequest);
return executeCreateQueryRunAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse createQueryRunWithHttpInfo(QueryRunCreateRequest queryRunCreateRequest) throws ApiException {
CreateQueryRunParams params = new CreateQueryRunParams(queryRunCreateRequest);
return executeCreateQueryRunAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public QueryRun createQueryRun( QueryRunCreateRequest queryRunCreateRequest , List expand) throws ApiException {
CreateQueryRunParams params = new CreateQueryRunParams(queryRunCreateRequest);
params.expand(expand);
return executeCreateQueryRunAPICall(params).getData();
}
/*
* add header methods
*/
public QueryRun createQueryRun(QueryRunCreateRequest queryRunCreateRequest ,List expand,Headers headers) throws ApiException {
CreateQueryRunParams params = new CreateQueryRunParams(queryRunCreateRequest)
.expand(expand)
.headers(headers);
return executeCreateQueryRunAPICall(params).getData();
}
/**
* Create a query run
* Creates a new query run job.
* @param params CreateQueryRunParams
* @return QueryRun
* @http.response.details
Status Code Description Response Headers
201 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public QueryRun createQueryRun(CreateQueryRunParams params) throws ApiException {
return executeCreateQueryRunAPICall(params).getData();
}
public ApiResponse createQueryRunWithHttpInfo(CreateQueryRunParams params) throws ApiException {
return executeCreateQueryRunAPICall(params);
}
ApiResponse executeCreateQueryRunAPICall(CreateQueryRunParams params) throws ApiException {
return createQueryRunWithHttpInfo(params.queryRunCreateRequest, params.fields, params.queryRunFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
private okhttp3.Call getQueryRunCall(String queryRunId, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/query_runs/{query_run_id}"
.replace("{" + "query_run_id" + "}", localVarApiClient.escapeString(queryRunId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (queryRunFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "query_run.fields[]", queryRunFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getQueryRunValidateBeforeCall(String queryRunId, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'queryRunId' is set
if (queryRunId == null) {
throw new ApiException("Missing the required parameter 'queryRunId' when calling getQueryRun(Async)");
}
return getQueryRunCall(queryRunId, fields, queryRunFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse getQueryRunWithHttpInfo(String queryRunId, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = getQueryRunValidateBeforeCall(queryRunId, fields, queryRunFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call getQueryRunAsync(String queryRunId, List fields, List queryRunFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = getQueryRunValidateBeforeCall(queryRunId, fields, queryRunFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class GetQueryRunParams {
private final String queryRunId;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List queryRunFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public GetQueryRunParams(String queryRunId) {
this.queryRunId = queryRunId;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return GetQueryRunParams
*/
public GetQueryRunParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return GetQueryRunParams
*/
public GetQueryRunParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `id`, `sql`, `remaining_attempts`, `updated_time`, `file`, `number_of_rows`, `processing_duration`, `state`, `column_separator` </details> (optional)
* @return GetQueryRunParams
*/
public GetQueryRunParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set queryRunFields
* @param queryRunFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `id`, `sql`, `remaining_attempts`, `updated_time`, `file`, `number_of_rows`, `processing_duration`, `state`, `column_separator` </details> (optional)
* @return GetQueryRunParams
*/
public GetQueryRunParams queryRunFields(List queryRunFields) {
this.queryRunFields = queryRunFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return GetQueryRunParams
*/
public GetQueryRunParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return GetQueryRunParams
*/
public GetQueryRunParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return GetQueryRunParams
*/
public GetQueryRunParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Retrieve a query run
* Retrieves the query run with the given ID.
* @param queryRunId query_run_id (required)
* @return QueryRun
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public GetQueryRunParams getQueryRunParams(String queryRunId) {
return new GetQueryRunParams(queryRunId);
}
public QueryRun getQueryRun(String queryRunId) throws ApiException {
GetQueryRunParams params = new GetQueryRunParams(queryRunId);
return executeGetQueryRunAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse getQueryRunWithHttpInfo(String queryRunId) throws ApiException {
GetQueryRunParams params = new GetQueryRunParams(queryRunId);
return executeGetQueryRunAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public QueryRun getQueryRun( String queryRunId , List expand) throws ApiException {
GetQueryRunParams params = new GetQueryRunParams(queryRunId);
params.expand(expand);
return executeGetQueryRunAPICall(params).getData();
}
/*
* add header methods
*/
public QueryRun getQueryRun(String queryRunId ,List expand,Headers headers) throws ApiException {
GetQueryRunParams params = new GetQueryRunParams(queryRunId)
.expand(expand)
.headers(headers);
return executeGetQueryRunAPICall(params).getData();
}
/**
* Retrieve a query run
* Retrieves the query run with the given ID.
* @param params GetQueryRunParams
* @return QueryRun
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public QueryRun getQueryRun(GetQueryRunParams params) throws ApiException {
return executeGetQueryRunAPICall(params).getData();
}
public ApiResponse getQueryRunWithHttpInfo(GetQueryRunParams params) throws ApiException {
return executeGetQueryRunAPICall(params);
}
ApiResponse executeGetQueryRunAPICall(GetQueryRunParams params) throws ApiException {
return getQueryRunWithHttpInfo(params.queryRunId, params.fields, params.queryRunFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy