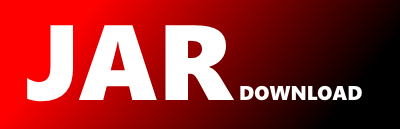
org.openapitools.client.api.SubscriptionPlansApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.api;
import org.openapitools.client.ApiCallback;
import org.openapitools.client.ApiClient;
import org.openapitools.client.ApiException;
import org.openapitools.client.model.Headers;
import org.openapitools.client.model.ListQueryParams;
import org.openapitools.client.model.GetByIdQueryParams;
import org.openapitools.client.ApiResponse;
import org.openapitools.client.Configuration;
import org.openapitools.client.Pair;
import org.openapitools.client.ProgressRequestBody;
import org.openapitools.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import java.math.BigDecimal;
import org.openapitools.client.model.ErrorResponse;
import org.openapitools.client.model.SubscriptionPlan;
import org.openapitools.client.model.SubscriptionPlanListResponse;
import org.openapitools.client.model.ErrorResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SubscriptionPlansApi {
private ApiClient localVarApiClient;
private int localHostIndex;
private String localCustomBaseUrl;
public SubscriptionPlansApi() {
this(Configuration.getDefaultApiClient());
}
public SubscriptionPlansApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public int getHostIndex() {
return localHostIndex;
}
public void setHostIndex(int hostIndex) {
this.localHostIndex = hostIndex;
}
public String getCustomBaseUrl() {
return localCustomBaseUrl;
}
public void setCustomBaseUrl(String customBaseUrl) {
this.localCustomBaseUrl = customBaseUrl;
}
private okhttp3.Call getSubscriptionPlanCall(String subscriptionPlanId, List fields, List subscriptionPlanFields, List subscriptionFields, List subscriptionItemsFields, List planFields, List productFields, List accountFields, List priceFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/subscription_plans/{subscription_plan_id}"
.replace("{" + "subscription_plan_id" + "}", localVarApiClient.escapeString(subscriptionPlanId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (subscriptionPlanFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "subscription_plan.fields[]", subscriptionPlanFields));
}
if (subscriptionFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "subscription.fields[]", subscriptionFields));
}
if (subscriptionItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "subscription_items.fields[]", subscriptionItemsFields));
}
if (planFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "plan.fields[]", planFields));
}
if (productFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "product.fields[]", productFields));
}
if (accountFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "account.fields[]", accountFields));
}
if (priceFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "price.fields[]", priceFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getSubscriptionPlanValidateBeforeCall(String subscriptionPlanId, List fields, List subscriptionPlanFields, List subscriptionFields, List subscriptionItemsFields, List planFields, List productFields, List accountFields, List priceFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'subscriptionPlanId' is set
if (subscriptionPlanId == null) {
throw new ApiException("Missing the required parameter 'subscriptionPlanId' when calling getSubscriptionPlan(Async)");
}
return getSubscriptionPlanCall(subscriptionPlanId, fields, subscriptionPlanFields, subscriptionFields, subscriptionItemsFields, planFields, productFields, accountFields, priceFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse getSubscriptionPlanWithHttpInfo(String subscriptionPlanId, List fields, List subscriptionPlanFields, List subscriptionFields, List subscriptionItemsFields, List planFields, List productFields, List accountFields, List priceFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = getSubscriptionPlanValidateBeforeCall(subscriptionPlanId, fields, subscriptionPlanFields, subscriptionFields, subscriptionItemsFields, planFields, productFields, accountFields, priceFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call getSubscriptionPlanAsync(String subscriptionPlanId, List fields, List subscriptionPlanFields, List subscriptionFields, List subscriptionItemsFields, List planFields, List productFields, List accountFields, List priceFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = getSubscriptionPlanValidateBeforeCall(subscriptionPlanId, fields, subscriptionPlanFields, subscriptionFields, subscriptionItemsFields, planFields, productFields, accountFields, priceFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class GetSubscriptionPlanParams {
private final String subscriptionPlanId;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List subscriptionPlanFields;
private List subscriptionFields;
private List subscriptionItemsFields;
private List planFields;
private List productFields;
private List accountFields;
private List priceFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public GetSubscriptionPlanParams(String subscriptionPlanId) {
this.subscriptionPlanId = subscriptionPlanId;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `name`, `plan_id`, `subscription_id`, `product_id`, `subscription_plan_number` </details> (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set subscriptionPlanFields
* @param subscriptionPlanFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `name`, `plan_id`, `subscription_id`, `product_id`, `subscription_plan_number` </details> (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams subscriptionPlanFields(List subscriptionPlanFields) {
this.subscriptionPlanFields = subscriptionPlanFields;
return this;
}
/**
* Set subscriptionFields
* @param subscriptionFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `subscription_number`, `state`, `account_id`, `invoice_owner_account_id`, `auto_renew`, `version`, `initial_term`, `current_term`, `renewal_term`, `start_date`, `end_date`, `description`, `contract_effective`, `service_activation`, `customer_acceptance`, `invoice_separately`, `latest_version`, `payment_terms`, `billing_document_settings`, `bill_to_id`, `sold_to_id`, `contracted_mrr`, `currency`, `cancel_reason`, `last_booking_date`, `order_number` </details> (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams subscriptionFields(List subscriptionFields) {
this.subscriptionFields = subscriptionFields;
return this;
}
/**
* Set subscriptionItemsFields
* @param subscriptionItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `start_date`, `end_date`, `charge_model`, `charge_type`, `tiers`, `subscription_item_number`, `name`, `description`, `charged_through_date`, `recurring`, `price_id`, `start_event`, `tax_code`, `tax_inclusive`, `unit_of_measure`, `quantity`, `price_base_interval`, `overage`, `subscription_plan_id`, `tiers_mode`, `processed_through_date`, `active`, `state`, `unit_amount`, `amount`, `discount_amount`, `discount_percent`, `price_change_percentage`, `price_change_option` </details> (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams subscriptionItemsFields(List subscriptionItemsFields) {
this.subscriptionItemsFields = subscriptionItemsFields;
return this;
}
/**
* Set planFields
* @param planFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `start_date`, `end_date`, `name`, `active`, `description`, `plan_number`, `product_id`, `active_currencies` </details> (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams planFields(List planFields) {
this.planFields = planFields;
return this;
}
/**
* Set productFields
* @param productFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `start_date`, `end_date`, `active`, `name`, `type`, `sku`, `description` </details> (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams productFields(List productFields) {
this.productFields = productFields;
return this;
}
/**
* Set accountFields
* @param accountFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `auto_pay`, `account_number`, `bill_to_id`, `sold_to_id`, `billing_document_settings`, `communication_profile_id`, `crm_id`, `sales_rep`, `parent_account_id`, `payment_gateway`, `payment_terms`, `remaining_credit_memo_balance`, `remaining_debit_memo_balance`, `remaining_invoice_balance`, `remaining_payment_balance`, `sequence_set_id`, `tax_certificate`, `batch`, `tax_identifier`, `bill_cycle_day`, `description`, `name`, `currency`, `default_payment_method_id`, `enabled`, `organization_id` </details> (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams accountFields(List accountFields) {
this.accountFields = accountFields;
return this;
}
/**
* Set priceFields
* @param priceFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `tiers`, `charge_model`, `charge_type`, `name`, `description`, `revenue_recognition_rule`, `stacked_discount`, `recognized_revenue_accounting_code`, `deferred_revenue_accounting_code`, `accounting_code`, `recurring`, `start_event`, `tax_code`, `tax_inclusive`, `taxable`, `unit_of_measure`, `quantity`, `min_quantity`, `max_quantity`, `price_base_interval`, `discount_level`, `overage`, `plan_id`, `tiers_mode`, `apply_discount_to`, `prepayment`, `drawdown`, `discount_amounts`, `unit_amounts`, `discount_percent`, `amounts`, `price_change_percentage`, `price_change_option`, `price_increase_option` </details> (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams priceFields(List priceFields) {
this.priceFields = priceFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return GetSubscriptionPlanParams
*/
public GetSubscriptionPlanParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Retrieve a subscription plan
* Retrieves the subscription plan with the given ID.
* @param subscriptionPlanId Suscription Plan Id (required)
* @return SubscriptionPlan
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public GetSubscriptionPlanParams getSubscriptionPlanParams(String subscriptionPlanId) {
return new GetSubscriptionPlanParams(subscriptionPlanId);
}
public SubscriptionPlan getSubscriptionPlan(String subscriptionPlanId) throws ApiException {
GetSubscriptionPlanParams params = new GetSubscriptionPlanParams(subscriptionPlanId);
return executeGetSubscriptionPlanAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse getSubscriptionPlanWithHttpInfo(String subscriptionPlanId) throws ApiException {
GetSubscriptionPlanParams params = new GetSubscriptionPlanParams(subscriptionPlanId);
return executeGetSubscriptionPlanAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public SubscriptionPlan getSubscriptionPlan( String subscriptionPlanId , List expand) throws ApiException {
GetSubscriptionPlanParams params = new GetSubscriptionPlanParams(subscriptionPlanId);
params.expand(expand);
return executeGetSubscriptionPlanAPICall(params).getData();
}
/*
* add header methods
*/
public SubscriptionPlan getSubscriptionPlan(String subscriptionPlanId ,List expand,Headers headers) throws ApiException {
GetSubscriptionPlanParams params = new GetSubscriptionPlanParams(subscriptionPlanId)
.expand(expand)
.headers(headers);
return executeGetSubscriptionPlanAPICall(params).getData();
}
/**
* Retrieve a subscription plan
* Retrieves the subscription plan with the given ID.
* @param params GetSubscriptionPlanParams
* @return SubscriptionPlan
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public SubscriptionPlan get(GetSubscriptionPlanParams params) throws ApiException {
return executeGetSubscriptionPlanAPICall(params).getData();
}
public ApiResponse getWithHttpInfo(GetSubscriptionPlanParams params) throws ApiException {
return executeGetSubscriptionPlanAPICall(params);
}
ApiResponse executeGetSubscriptionPlanAPICall(GetSubscriptionPlanParams params) throws ApiException {
return getSubscriptionPlanWithHttpInfo(params.subscriptionPlanId, params.fields, params.subscriptionPlanFields, params.subscriptionFields, params.subscriptionItemsFields, params.planFields, params.productFields, params.accountFields, params.priceFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
private okhttp3.Call getSubscriptionPlansCall(String cursor, List expand, List filter, List sort, Integer pageSize, List fields, List subscriptionPlanFields, List subscriptionFields, List subscriptionItemsFields, List planFields, List productFields, List accountFields, List priceFields, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/subscription_plans";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (cursor != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("cursor", cursor));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (sort != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "sort[]", sort));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (subscriptionPlanFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "subscription_plan.fields[]", subscriptionPlanFields));
}
if (subscriptionFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "subscription.fields[]", subscriptionFields));
}
if (subscriptionItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "subscription_items.fields[]", subscriptionItemsFields));
}
if (planFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "plan.fields[]", planFields));
}
if (productFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "product.fields[]", productFields));
}
if (accountFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "account.fields[]", accountFields));
}
if (priceFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "price.fields[]", priceFields));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getSubscriptionPlansValidateBeforeCall(String cursor, List expand, List filter, List sort, Integer pageSize, List fields, List subscriptionPlanFields, List subscriptionFields, List subscriptionItemsFields, List planFields, List productFields, List accountFields, List priceFields, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
return getSubscriptionPlansCall(cursor, expand, filter, sort, pageSize, fields, subscriptionPlanFields, subscriptionFields, subscriptionItemsFields, planFields, productFields, accountFields, priceFields, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse getSubscriptionPlansWithHttpInfo(String cursor, List expand, List filter, List sort, Integer pageSize, List fields, List subscriptionPlanFields, List subscriptionFields, List subscriptionItemsFields, List planFields, List productFields, List accountFields, List priceFields, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = getSubscriptionPlansValidateBeforeCall(cursor, expand, filter, sort, pageSize, fields, subscriptionPlanFields, subscriptionFields, subscriptionItemsFields, planFields, productFields, accountFields, priceFields, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call getSubscriptionPlansAsync(String cursor, List expand, List filter, List sort, Integer pageSize, List fields, List subscriptionPlanFields, List subscriptionFields, List subscriptionItemsFields, List planFields, List productFields, List accountFields, List priceFields, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = getSubscriptionPlansValidateBeforeCall(cursor, expand, filter, sort, pageSize, fields, subscriptionPlanFields, subscriptionFields, subscriptionItemsFields, planFields, productFields, accountFields, priceFields, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class GetSubscriptionPlansParams {
private Headers headers;
/*
* Check for list params
*/
private ListQueryParams listQueryParams;
private String cursor;
private List expand;
private List filter;
private List sort;
private Integer pageSize;
private List fields;
private List subscriptionPlanFields;
private List subscriptionFields;
private List subscriptionItemsFields;
private List planFields;
private List productFields;
private List accountFields;
private List priceFields;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public GetSubscriptionPlansParams() {
}
/**
* Set listQueryParams
* @param listQueryParams ListQueryParams
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams listQueryParams(ListQueryParams listQueryParams) {
this.listQueryParams = listQueryParams;
if (cursor != null) {
this.cursor = listQueryParams.getCursor();
}
if (expand != null) {
this.expand = listQueryParams.getExpand();
}
if (filter != null) {
this.filter = listQueryParams.getFilter();
}
if (sort != null) {
this.sort = listQueryParams.getSort();
}
if (pageSize != null) {
this.pageSize = listQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set cursor
* @param cursor A cursor for use in pagination. A cursor defines the starting place in a list. For instance, if you make a list request and receive 100 objects, ending with `next_page=W3sib3JkZXJ=`, your subsequent call can include `cursor=W3sib3JkZXJ=` in order to fetch the next page of the list. (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams cursor(String cursor) {
this.cursor = cursor;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set sort
* @param sort A case-sensitive query parameter that specifies the sort order of the list, which can be either ascending (e.g. `account_number.asc`) or descending (e.g. `account_number.desc`). You cannot sort on properties that are arrays. If the array-type properties are specified for the `sort[]` parameter, they are ignored. (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams sort(List sort) {
this.sort = sort;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `name`, `plan_id`, `subscription_id`, `product_id`, `subscription_plan_number` </details> (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set subscriptionPlanFields
* @param subscriptionPlanFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `name`, `plan_id`, `subscription_id`, `product_id`, `subscription_plan_number` </details> (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams subscriptionPlanFields(List subscriptionPlanFields) {
this.subscriptionPlanFields = subscriptionPlanFields;
return this;
}
/**
* Set subscriptionFields
* @param subscriptionFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `subscription_number`, `state`, `account_id`, `invoice_owner_account_id`, `auto_renew`, `version`, `initial_term`, `current_term`, `renewal_term`, `start_date`, `end_date`, `description`, `contract_effective`, `service_activation`, `customer_acceptance`, `invoice_separately`, `latest_version`, `payment_terms`, `billing_document_settings`, `bill_to_id`, `sold_to_id`, `contracted_mrr`, `currency`, `cancel_reason`, `last_booking_date`, `order_number` </details> (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams subscriptionFields(List subscriptionFields) {
this.subscriptionFields = subscriptionFields;
return this;
}
/**
* Set subscriptionItemsFields
* @param subscriptionItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `start_date`, `end_date`, `charge_model`, `charge_type`, `tiers`, `subscription_item_number`, `name`, `description`, `charged_through_date`, `recurring`, `price_id`, `start_event`, `tax_code`, `tax_inclusive`, `unit_of_measure`, `quantity`, `price_base_interval`, `overage`, `subscription_plan_id`, `tiers_mode`, `processed_through_date`, `active`, `state`, `unit_amount`, `amount`, `discount_amount`, `discount_percent`, `price_change_percentage`, `price_change_option` </details> (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams subscriptionItemsFields(List subscriptionItemsFields) {
this.subscriptionItemsFields = subscriptionItemsFields;
return this;
}
/**
* Set planFields
* @param planFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `start_date`, `end_date`, `name`, `active`, `description`, `plan_number`, `product_id`, `active_currencies` </details> (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams planFields(List planFields) {
this.planFields = planFields;
return this;
}
/**
* Set productFields
* @param productFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `start_date`, `end_date`, `active`, `name`, `type`, `sku`, `description` </details> (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams productFields(List productFields) {
this.productFields = productFields;
return this;
}
/**
* Set accountFields
* @param accountFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `auto_pay`, `account_number`, `bill_to_id`, `sold_to_id`, `billing_document_settings`, `communication_profile_id`, `crm_id`, `sales_rep`, `parent_account_id`, `payment_gateway`, `payment_terms`, `remaining_credit_memo_balance`, `remaining_debit_memo_balance`, `remaining_invoice_balance`, `remaining_payment_balance`, `sequence_set_id`, `tax_certificate`, `batch`, `tax_identifier`, `bill_cycle_day`, `description`, `name`, `currency`, `default_payment_method_id`, `enabled`, `organization_id` </details> (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams accountFields(List accountFields) {
this.accountFields = accountFields;
return this;
}
/**
* Set priceFields
* @param priceFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `tiers`, `charge_model`, `charge_type`, `name`, `description`, `revenue_recognition_rule`, `stacked_discount`, `recognized_revenue_accounting_code`, `deferred_revenue_accounting_code`, `accounting_code`, `recurring`, `start_event`, `tax_code`, `tax_inclusive`, `taxable`, `unit_of_measure`, `quantity`, `min_quantity`, `max_quantity`, `price_base_interval`, `discount_level`, `overage`, `plan_id`, `tiers_mode`, `apply_discount_to`, `prepayment`, `drawdown`, `discount_amounts`, `unit_amounts`, `discount_percent`, `amounts`, `price_change_percentage`, `price_change_option`, `price_increase_option` </details> (optional)
* @return GetSubscriptionPlansParams
*/
public GetSubscriptionPlansParams priceFields(List priceFields) {
this.priceFields = priceFields;
return this;
}
}
/**
* List subscription plans
* Returns a dictionary with a data property that contains an array of subscription plans, starting after cursor. Each entry in the array is a separate object. If no more are available, the resulting array will be empty. This request should never return an error.
* @return SubscriptionPlanListResponse
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public GetSubscriptionPlansParams getSubscriptionPlansParams() {
return new GetSubscriptionPlansParams();
}
public SubscriptionPlanListResponse getSubscriptionPlans() throws ApiException {
GetSubscriptionPlansParams params = new GetSubscriptionPlansParams();
return executeGetSubscriptionPlansAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse getSubscriptionPlansWithHttpInfo() throws ApiException {
GetSubscriptionPlansParams params = new GetSubscriptionPlansParams();
return executeGetSubscriptionPlansAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public SubscriptionPlanListResponse getSubscriptionPlans(String cursor, List expand, List filter) throws ApiException {
GetSubscriptionPlansParams params = new GetSubscriptionPlansParams();
params.cursor(cursor);
params.expand(expand);
params.filter(filter);
return executeGetSubscriptionPlansAPICall(params).getData();
}
/*
* add header methods
*/
public SubscriptionPlanListResponse getSubscriptionPlans(String cursor,List expand,List filter,Headers headers) throws ApiException {
GetSubscriptionPlansParams params = new GetSubscriptionPlansParams()
.cursor(cursor)
.expand(expand)
.filter(filter)
.headers(headers);
return executeGetSubscriptionPlansAPICall(params).getData();
}
/**
* List subscription plans
* Returns a dictionary with a data property that contains an array of subscription plans, starting after cursor. Each entry in the array is a separate object. If no more are available, the resulting array will be empty. This request should never return an error.
* @param params GetSubscriptionPlansParams
* @return SubscriptionPlanListResponse
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public SubscriptionPlanListResponse list(GetSubscriptionPlansParams params) throws ApiException {
return executeGetSubscriptionPlansAPICall(params).getData();
}
public ApiResponse listWithHttpInfo(GetSubscriptionPlansParams params) throws ApiException {
return executeGetSubscriptionPlansAPICall(params);
}
ApiResponse executeGetSubscriptionPlansAPICall(GetSubscriptionPlansParams params) throws ApiException {
return getSubscriptionPlansWithHttpInfo(params.cursor, params.expand, params.filter, params.sort, params.pageSize, params.fields, params.subscriptionPlanFields, params.subscriptionFields, params.subscriptionItemsFields, params.planFields, params.productFields, params.accountFields, params.priceFields, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy