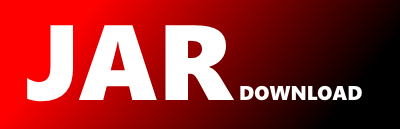
org.openapitools.client.model.AccountCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.AccountContactCreateRequest;
import org.openapitools.client.model.AccountPaymentMethodRequest;
import org.openapitools.client.model.BillingDocumentSettings;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.TaxCertificate;
import org.openapitools.client.model.TaxIdentifier;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* AccountCreateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class AccountCreateRequest {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS = "billing_document_settings";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS)
private BillingDocumentSettings billingDocumentSettings;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "bill_cycle_day";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Integer billCycleDay;
public static final String SERIALIZED_NAME_BILL_TO = "bill_to";
@SerializedName(SERIALIZED_NAME_BILL_TO)
private AccountContactCreateRequest billTo;
public static final String SERIALIZED_NAME_BILL_TO_ID = "bill_to_id";
@SerializedName(SERIALIZED_NAME_BILL_TO_ID)
private String billToId;
public static final String SERIALIZED_NAME_SOLD_TO = "sold_to";
@SerializedName(SERIALIZED_NAME_SOLD_TO)
private AccountContactCreateRequest soldTo;
public static final String SERIALIZED_NAME_SOLD_TO_ID = "sold_to_id";
@SerializedName(SERIALIZED_NAME_SOLD_TO_ID)
private String soldToId;
public static final String SERIALIZED_NAME_COMMUNICATION_PROFILE_ID = "communication_profile_id";
@SerializedName(SERIALIZED_NAME_COMMUNICATION_PROFILE_ID)
private String communicationProfileId;
public static final String SERIALIZED_NAME_CRM_ID = "crm_id";
@SerializedName(SERIALIZED_NAME_CRM_ID)
private String crmId;
public static final String SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD_ID = "default_payment_method_id";
@SerializedName(SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD_ID)
private String defaultPaymentMethodId;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_PARENT_ACCOUNT_ID = "parent_account_id";
@SerializedName(SERIALIZED_NAME_PARENT_ACCOUNT_ID)
private String parentAccountId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY = "payment_gateway";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY)
@JsonAdapter(NullableFieldAdapter.class)
private String paymentGateway;
public static final String SERIALIZED_NAME_PAYMENT_TERMS = "payment_terms";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERMS)
private String paymentTerms;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequence_set_id";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_AUTO_PAY = "auto_pay";
@SerializedName(SERIALIZED_NAME_AUTO_PAY)
private Boolean autoPay;
public static final String SERIALIZED_NAME_TAX_CERTIFICATE = "tax_certificate";
@SerializedName(SERIALIZED_NAME_TAX_CERTIFICATE)
private TaxCertificate taxCertificate;
public static final String SERIALIZED_NAME_TAX_IDENTIFIER = "tax_identifier";
@SerializedName(SERIALIZED_NAME_TAX_IDENTIFIER)
private TaxIdentifier taxIdentifier;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_SALES_REP = "sales_rep";
@SerializedName(SERIALIZED_NAME_SALES_REP)
private String salesRep;
public static final String SERIALIZED_NAME_PAYMENT_METHOD = "payment_method";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD)
private AccountPaymentMethodRequest paymentMethod;
public static final String SERIALIZED_NAME_ORGANIZATION_LABEL = "organization_label";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_LABEL)
private String organizationLabel;
public AccountCreateRequest() {
}
public AccountCreateRequest(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public AccountCreateRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public AccountCreateRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public AccountCreateRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Human-readable identifier of the account. It can be user-supplied.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "A-100001", value = "Human-readable identifier of the account. It can be user-supplied.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public AccountCreateRequest billingDocumentSettings(BillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
return this;
}
/**
* Get billingDocumentSettings
* @return billingDocumentSettings
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public BillingDocumentSettings getBillingDocumentSettings() {
return billingDocumentSettings;
}
public void setBillingDocumentSettings(BillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
}
public AccountCreateRequest batch(String batch) {
this.batch = batch;
return this;
}
/**
* The identifier of a bill run batch.
* @return batch
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of a bill run batch.")
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public AccountCreateRequest billCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* The day of the month on which your customer will be invoiced. For month-end specify 31.
* minimum: 0
* maximum: 31
* @return billCycleDay
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The day of the month on which your customer will be invoiced. For month-end specify 31.")
public Integer getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
}
public AccountCreateRequest billTo(AccountContactCreateRequest billTo) {
this.billTo = billTo;
return this;
}
/**
* Customer billing address.
* @return billTo
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "Customer billing address.")
public AccountContactCreateRequest getBillTo() {
return billTo;
}
public void setBillTo(AccountContactCreateRequest billTo) {
this.billTo = billTo;
}
public AccountCreateRequest billToId(String billToId) {
this.billToId = billToId;
return this;
}
/**
* Bill To Contact Id
* @return billToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0946a6dffc0016a7faab604299b", value = "Bill To Contact Id")
public String getBillToId() {
return billToId;
}
public void setBillToId(String billToId) {
this.billToId = billToId;
}
public AccountCreateRequest soldTo(AccountContactCreateRequest soldTo) {
this.soldTo = soldTo;
return this;
}
/**
* Customer address used for calculating tax.
* @return soldTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Customer address used for calculating tax.")
public AccountContactCreateRequest getSoldTo() {
return soldTo;
}
public void setSoldTo(AccountContactCreateRequest soldTo) {
this.soldTo = soldTo;
}
public AccountCreateRequest soldToId(String soldToId) {
this.soldToId = soldToId;
return this;
}
/**
* Sold To Contact Id
* @return soldToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0946a6dffc0016a7faab604299b", value = "Sold To Contact Id")
public String getSoldToId() {
return soldToId;
}
public void setSoldToId(String soldToId) {
this.soldToId = soldToId;
}
public AccountCreateRequest communicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
return this;
}
/**
* Identifier of the communication profile associated with this customer.
* @return communicationProfileId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0946a6dffc0016a7faab604299b", value = "Identifier of the communication profile associated with this customer.")
public String getCommunicationProfileId() {
return communicationProfileId;
}
public void setCommunicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
}
public AccountCreateRequest crmId(String crmId) {
this.crmId = crmId;
return this;
}
/**
* CRM account identifier.
* @return crmId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1a2b3c4d5e", value = "CRM account identifier.")
public String getCrmId() {
return crmId;
}
public void setCrmId(String crmId) {
this.crmId = crmId;
}
public AccountCreateRequest defaultPaymentMethodId(String defaultPaymentMethodId) {
this.defaultPaymentMethodId = defaultPaymentMethodId;
return this;
}
/**
* Identifier of the default payment method on the customer account.
* @return defaultPaymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8a95b1946b6aeac8718c32aab8c395f", value = "Identifier of the default payment method on the customer account.")
public String getDefaultPaymentMethodId() {
return defaultPaymentMethodId;
}
public void setDefaultPaymentMethodId(String defaultPaymentMethodId) {
this.defaultPaymentMethodId = defaultPaymentMethodId;
}
public AccountCreateRequest name(String name) {
this.name = name;
return this;
}
/**
* The name of the customer account.
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "test account", required = true, value = "The name of the customer account.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public AccountCreateRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "description of test account", value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public AccountCreateRequest parentAccountId(String parentAccountId) {
this.parentAccountId = parentAccountId;
return this;
}
/**
* Identifier of this customer's parent account, if any.
* @return parentAccountId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad093f27d6eee80017d6effd7a66759", value = "Identifier of this customer's parent account, if any.")
public String getParentAccountId() {
return parentAccountId;
}
public void setParentAccountId(String parentAccountId) {
this.parentAccountId = parentAccountId;
}
public AccountCreateRequest paymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
return this;
}
/**
* Payment gateway name.
* @return paymentGateway
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "adyen gateway", value = "Payment gateway name.")
public String getPaymentGateway() {
return paymentGateway;
}
public void setPaymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
}
public AccountCreateRequest paymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
return this;
}
/**
* Payment terms configured in **Billing Settings > Payment Terms** of your Zuora tenant.
* @return paymentTerms
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Net 30", value = "Payment terms configured in **Billing Settings > Payment Terms** of your Zuora tenant.")
public String getPaymentTerms() {
return paymentTerms;
}
public void setPaymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
}
public AccountCreateRequest sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The identifier or the billing document sequence set that is assigned to the customer account.
* @return sequenceSetId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92a4204a6dffc0016a7faab723041c", value = "The identifier or the billing document sequence set that is assigned to the customer account.")
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public AccountCreateRequest autoPay(Boolean autoPay) {
this.autoPay = autoPay;
return this;
}
/**
* Controls whether future payments are automatically billed when they are due.
* @return autoPay
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "true", value = "Controls whether future payments are automatically billed when they are due.")
public Boolean getAutoPay() {
return autoPay;
}
public void setAutoPay(Boolean autoPay) {
this.autoPay = autoPay;
}
public AccountCreateRequest taxCertificate(TaxCertificate taxCertificate) {
this.taxCertificate = taxCertificate;
return this;
}
/**
* Get taxCertificate
* @return taxCertificate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public TaxCertificate getTaxCertificate() {
return taxCertificate;
}
public void setTaxCertificate(TaxCertificate taxCertificate) {
this.taxCertificate = taxCertificate;
}
public AccountCreateRequest taxIdentifier(TaxIdentifier taxIdentifier) {
this.taxIdentifier = taxIdentifier;
return this;
}
/**
* Get taxIdentifier
* @return taxIdentifier
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public TaxIdentifier getTaxIdentifier() {
return taxIdentifier;
}
public void setTaxIdentifier(TaxIdentifier taxIdentifier) {
this.taxIdentifier = taxIdentifier;
}
public AccountCreateRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* Three-letter ISO currency code. Once the currency is set for an account, it cannot be updated.
* @return currency
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "USD", required = true, value = "Three-letter ISO currency code. Once the currency is set for an account, it cannot be updated.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public AccountCreateRequest salesRep(String salesRep) {
this.salesRep = salesRep;
return this;
}
/**
* The name of the sales representative associated with this account
* @return salesRep
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Max", value = "The name of the sales representative associated with this account")
public String getSalesRep() {
return salesRep;
}
public void setSalesRep(String salesRep) {
this.salesRep = salesRep;
}
public AccountCreateRequest paymentMethod(AccountPaymentMethodRequest paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* A new payment method for the account.
* @return paymentMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A new payment method for the account.")
public AccountPaymentMethodRequest getPaymentMethod() {
return paymentMethod;
}
public void setPaymentMethod(AccountPaymentMethodRequest paymentMethod) {
this.paymentMethod = paymentMethod;
}
public AccountCreateRequest organizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
return this;
}
/**
* The organization label associated with the account.
* @return organizationLabel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The organization label associated with the account.")
public String getOrganizationLabel() {
return organizationLabel;
}
public void setOrganizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AccountCreateRequest accountCreateRequest = (AccountCreateRequest) o;
return Objects.equals(this.id, accountCreateRequest.id) &&
Objects.equals(this.updatedById, accountCreateRequest.updatedById) &&
Objects.equals(this.updatedTime, accountCreateRequest.updatedTime) &&
Objects.equals(this.createdById, accountCreateRequest.createdById) &&
Objects.equals(this.createdTime, accountCreateRequest.createdTime) &&
Objects.equals(this.customFields, accountCreateRequest.customFields) &&
Objects.equals(this.customObjects, accountCreateRequest.customObjects) &&
Objects.equals(this.accountNumber, accountCreateRequest.accountNumber) &&
Objects.equals(this.billingDocumentSettings, accountCreateRequest.billingDocumentSettings) &&
Objects.equals(this.batch, accountCreateRequest.batch) &&
Objects.equals(this.billCycleDay, accountCreateRequest.billCycleDay) &&
Objects.equals(this.billTo, accountCreateRequest.billTo) &&
Objects.equals(this.billToId, accountCreateRequest.billToId) &&
Objects.equals(this.soldTo, accountCreateRequest.soldTo) &&
Objects.equals(this.soldToId, accountCreateRequest.soldToId) &&
Objects.equals(this.communicationProfileId, accountCreateRequest.communicationProfileId) &&
Objects.equals(this.crmId, accountCreateRequest.crmId) &&
Objects.equals(this.defaultPaymentMethodId, accountCreateRequest.defaultPaymentMethodId) &&
Objects.equals(this.name, accountCreateRequest.name) &&
Objects.equals(this.description, accountCreateRequest.description) &&
Objects.equals(this.parentAccountId, accountCreateRequest.parentAccountId) &&
Objects.equals(this.paymentGateway, accountCreateRequest.paymentGateway) &&
Objects.equals(this.paymentTerms, accountCreateRequest.paymentTerms) &&
Objects.equals(this.sequenceSetId, accountCreateRequest.sequenceSetId) &&
Objects.equals(this.autoPay, accountCreateRequest.autoPay) &&
Objects.equals(this.taxCertificate, accountCreateRequest.taxCertificate) &&
Objects.equals(this.taxIdentifier, accountCreateRequest.taxIdentifier) &&
Objects.equals(this.currency, accountCreateRequest.currency) &&
Objects.equals(this.salesRep, accountCreateRequest.salesRep) &&
Objects.equals(this.paymentMethod, accountCreateRequest.paymentMethod) &&
Objects.equals(this.organizationLabel, accountCreateRequest.organizationLabel);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, accountNumber, billingDocumentSettings, batch, billCycleDay, billTo, billToId, soldTo, soldToId, communicationProfileId, crmId, defaultPaymentMethodId, name, description, parentAccountId, paymentGateway, paymentTerms, sequenceSetId, autoPay, taxCertificate, taxIdentifier, currency, salesRep, paymentMethod, organizationLabel);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AccountCreateRequest {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" billingDocumentSettings: ").append(toIndentedString(billingDocumentSettings)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billTo: ").append(toIndentedString(billTo)).append("\n");
sb.append(" billToId: ").append(toIndentedString(billToId)).append("\n");
sb.append(" soldTo: ").append(toIndentedString(soldTo)).append("\n");
sb.append(" soldToId: ").append(toIndentedString(soldToId)).append("\n");
sb.append(" communicationProfileId: ").append(toIndentedString(communicationProfileId)).append("\n");
sb.append(" crmId: ").append(toIndentedString(crmId)).append("\n");
sb.append(" defaultPaymentMethodId: ").append(toIndentedString(defaultPaymentMethodId)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" parentAccountId: ").append(toIndentedString(parentAccountId)).append("\n");
sb.append(" paymentGateway: ").append(toIndentedString(paymentGateway)).append("\n");
sb.append(" paymentTerms: ").append(toIndentedString(paymentTerms)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" autoPay: ").append(toIndentedString(autoPay)).append("\n");
sb.append(" taxCertificate: ").append(toIndentedString(taxCertificate)).append("\n");
sb.append(" taxIdentifier: ").append(toIndentedString(taxIdentifier)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" salesRep: ").append(toIndentedString(salesRep)).append("\n");
sb.append(" paymentMethod: ").append(toIndentedString(paymentMethod)).append("\n");
sb.append(" organizationLabel: ").append(toIndentedString(organizationLabel)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy