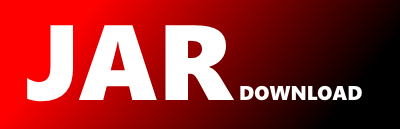
org.openapitools.client.model.AccountPatchRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.AccountContactPatchRequest;
import org.openapitools.client.model.BillingDocumentSettings;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.TaxCertificate;
import org.openapitools.client.model.TaxIdentifier;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* AccountPatchRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class AccountPatchRequest {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS = "billing_document_settings";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS)
private BillingDocumentSettings billingDocumentSettings;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "bill_cycle_day";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Integer billCycleDay;
public static final String SERIALIZED_NAME_BILL_TO = "bill_to";
@SerializedName(SERIALIZED_NAME_BILL_TO)
private AccountContactPatchRequest billTo;
public static final String SERIALIZED_NAME_BILL_TO_ID = "bill_to_id";
@SerializedName(SERIALIZED_NAME_BILL_TO_ID)
private String billToId;
public static final String SERIALIZED_NAME_SOLD_TO = "sold_to";
@SerializedName(SERIALIZED_NAME_SOLD_TO)
private AccountContactPatchRequest soldTo;
public static final String SERIALIZED_NAME_SOLD_TO_ID = "sold_to_id";
@SerializedName(SERIALIZED_NAME_SOLD_TO_ID)
private String soldToId;
public static final String SERIALIZED_NAME_COMMUNICATION_PROFILE_ID = "communication_profile_id";
@SerializedName(SERIALIZED_NAME_COMMUNICATION_PROFILE_ID)
private String communicationProfileId;
public static final String SERIALIZED_NAME_CRM_ID = "crm_id";
@SerializedName(SERIALIZED_NAME_CRM_ID)
private String crmId;
public static final String SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD_ID = "default_payment_method_id";
@SerializedName(SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD_ID)
private String defaultPaymentMethodId;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_PARENT_ACCOUNT_ID = "parent_account_id";
@SerializedName(SERIALIZED_NAME_PARENT_ACCOUNT_ID)
private String parentAccountId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY = "payment_gateway";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY)
@JsonAdapter(NullableFieldAdapter.class)
private String paymentGateway;
public static final String SERIALIZED_NAME_PAYMENT_TERMS = "payment_terms";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERMS)
private String paymentTerms;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequence_set_id";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_AUTO_PAY = "auto_pay";
@SerializedName(SERIALIZED_NAME_AUTO_PAY)
private Boolean autoPay;
public static final String SERIALIZED_NAME_TAX_CERTIFICATE = "tax_certificate";
@SerializedName(SERIALIZED_NAME_TAX_CERTIFICATE)
private TaxCertificate taxCertificate;
public static final String SERIALIZED_NAME_TAX_IDENTIFIER = "tax_identifier";
@SerializedName(SERIALIZED_NAME_TAX_IDENTIFIER)
private TaxIdentifier taxIdentifier;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_SALES_REP = "sales_rep";
@SerializedName(SERIALIZED_NAME_SALES_REP)
private String salesRep;
public AccountPatchRequest() {
}
public AccountPatchRequest(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public AccountPatchRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public AccountPatchRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public AccountPatchRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Human-readable identifier of the account. It can be user-supplied.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "A-100001", value = "Human-readable identifier of the account. It can be user-supplied.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public AccountPatchRequest billingDocumentSettings(BillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
return this;
}
/**
* Get billingDocumentSettings
* @return billingDocumentSettings
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public BillingDocumentSettings getBillingDocumentSettings() {
return billingDocumentSettings;
}
public void setBillingDocumentSettings(BillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
}
public AccountPatchRequest batch(String batch) {
this.batch = batch;
return this;
}
/**
* The identifier of a bill run batch.
* @return batch
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of a bill run batch.")
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public AccountPatchRequest billCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* The day of the month on which your customer will be invoiced. For month-end specify 31.
* minimum: 0
* maximum: 31
* @return billCycleDay
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The day of the month on which your customer will be invoiced. For month-end specify 31.")
public Integer getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
}
public AccountPatchRequest billTo(AccountContactPatchRequest billTo) {
this.billTo = billTo;
return this;
}
/**
* Customer billing address.
* @return billTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Customer billing address.")
public AccountContactPatchRequest getBillTo() {
return billTo;
}
public void setBillTo(AccountContactPatchRequest billTo) {
this.billTo = billTo;
}
public AccountPatchRequest billToId(String billToId) {
this.billToId = billToId;
return this;
}
/**
* Bill To Contact Id
* @return billToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0946a6dffc0016a7faab604299b", value = "Bill To Contact Id")
public String getBillToId() {
return billToId;
}
public void setBillToId(String billToId) {
this.billToId = billToId;
}
public AccountPatchRequest soldTo(AccountContactPatchRequest soldTo) {
this.soldTo = soldTo;
return this;
}
/**
* Customer address used for calculating tax.
* @return soldTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Customer address used for calculating tax.")
public AccountContactPatchRequest getSoldTo() {
return soldTo;
}
public void setSoldTo(AccountContactPatchRequest soldTo) {
this.soldTo = soldTo;
}
public AccountPatchRequest soldToId(String soldToId) {
this.soldToId = soldToId;
return this;
}
/**
* Sold To Contact Id
* @return soldToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0946a6dffc0016a7faab604299b", value = "Sold To Contact Id")
public String getSoldToId() {
return soldToId;
}
public void setSoldToId(String soldToId) {
this.soldToId = soldToId;
}
public AccountPatchRequest communicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
return this;
}
/**
* Identifier of the communication profile associated with this customer.
* @return communicationProfileId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0946a6dffc0016a7faab604299b", value = "Identifier of the communication profile associated with this customer.")
public String getCommunicationProfileId() {
return communicationProfileId;
}
public void setCommunicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
}
public AccountPatchRequest crmId(String crmId) {
this.crmId = crmId;
return this;
}
/**
* CRM account identifier.
* @return crmId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1a2b3c4d5e", value = "CRM account identifier.")
public String getCrmId() {
return crmId;
}
public void setCrmId(String crmId) {
this.crmId = crmId;
}
public AccountPatchRequest defaultPaymentMethodId(String defaultPaymentMethodId) {
this.defaultPaymentMethodId = defaultPaymentMethodId;
return this;
}
/**
* Identifier of the default payment method on the customer account.
* @return defaultPaymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8a95b1946b6aeac8718c32aab8c395f", value = "Identifier of the default payment method on the customer account.")
public String getDefaultPaymentMethodId() {
return defaultPaymentMethodId;
}
public void setDefaultPaymentMethodId(String defaultPaymentMethodId) {
this.defaultPaymentMethodId = defaultPaymentMethodId;
}
public AccountPatchRequest name(String name) {
this.name = name;
return this;
}
/**
* The name of the customer account.
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "test account", value = "The name of the customer account.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public AccountPatchRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "description of test account", value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public AccountPatchRequest parentAccountId(String parentAccountId) {
this.parentAccountId = parentAccountId;
return this;
}
/**
* Identifier of this customer's parent account, if any.
* @return parentAccountId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad093f27d6eee80017d6effd7a66759", value = "Identifier of this customer's parent account, if any.")
public String getParentAccountId() {
return parentAccountId;
}
public void setParentAccountId(String parentAccountId) {
this.parentAccountId = parentAccountId;
}
public AccountPatchRequest paymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
return this;
}
/**
* Payment gateway name.
* @return paymentGateway
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "adyen gateway", value = "Payment gateway name.")
public String getPaymentGateway() {
return paymentGateway;
}
public void setPaymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
}
public AccountPatchRequest paymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
return this;
}
/**
* Payment terms configured in **Billing Settings > Payment Terms** of your Zuora tenant.
* @return paymentTerms
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Net 30", value = "Payment terms configured in **Billing Settings > Payment Terms** of your Zuora tenant.")
public String getPaymentTerms() {
return paymentTerms;
}
public void setPaymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
}
public AccountPatchRequest sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The identifier or the billing document sequence set that is assigned to the customer account.
* @return sequenceSetId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92a4204a6dffc0016a7faab723041c", value = "The identifier or the billing document sequence set that is assigned to the customer account.")
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public AccountPatchRequest autoPay(Boolean autoPay) {
this.autoPay = autoPay;
return this;
}
/**
* Controls whether future payments are automatically billed when they are due.
* @return autoPay
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "true", value = "Controls whether future payments are automatically billed when they are due.")
public Boolean getAutoPay() {
return autoPay;
}
public void setAutoPay(Boolean autoPay) {
this.autoPay = autoPay;
}
public AccountPatchRequest taxCertificate(TaxCertificate taxCertificate) {
this.taxCertificate = taxCertificate;
return this;
}
/**
* Get taxCertificate
* @return taxCertificate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public TaxCertificate getTaxCertificate() {
return taxCertificate;
}
public void setTaxCertificate(TaxCertificate taxCertificate) {
this.taxCertificate = taxCertificate;
}
public AccountPatchRequest taxIdentifier(TaxIdentifier taxIdentifier) {
this.taxIdentifier = taxIdentifier;
return this;
}
/**
* Get taxIdentifier
* @return taxIdentifier
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public TaxIdentifier getTaxIdentifier() {
return taxIdentifier;
}
public void setTaxIdentifier(TaxIdentifier taxIdentifier) {
this.taxIdentifier = taxIdentifier;
}
public AccountPatchRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* Three-letter ISO currency code. Once the currency is set for an account, it cannot be updated.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USD", value = "Three-letter ISO currency code. Once the currency is set for an account, it cannot be updated.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public AccountPatchRequest salesRep(String salesRep) {
this.salesRep = salesRep;
return this;
}
/**
* The name of the sales representative associated with this account
* @return salesRep
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Max", value = "The name of the sales representative associated with this account")
public String getSalesRep() {
return salesRep;
}
public void setSalesRep(String salesRep) {
this.salesRep = salesRep;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AccountPatchRequest accountPatchRequest = (AccountPatchRequest) o;
return Objects.equals(this.id, accountPatchRequest.id) &&
Objects.equals(this.updatedById, accountPatchRequest.updatedById) &&
Objects.equals(this.updatedTime, accountPatchRequest.updatedTime) &&
Objects.equals(this.createdById, accountPatchRequest.createdById) &&
Objects.equals(this.createdTime, accountPatchRequest.createdTime) &&
Objects.equals(this.customFields, accountPatchRequest.customFields) &&
Objects.equals(this.customObjects, accountPatchRequest.customObjects) &&
Objects.equals(this.accountNumber, accountPatchRequest.accountNumber) &&
Objects.equals(this.billingDocumentSettings, accountPatchRequest.billingDocumentSettings) &&
Objects.equals(this.batch, accountPatchRequest.batch) &&
Objects.equals(this.billCycleDay, accountPatchRequest.billCycleDay) &&
Objects.equals(this.billTo, accountPatchRequest.billTo) &&
Objects.equals(this.billToId, accountPatchRequest.billToId) &&
Objects.equals(this.soldTo, accountPatchRequest.soldTo) &&
Objects.equals(this.soldToId, accountPatchRequest.soldToId) &&
Objects.equals(this.communicationProfileId, accountPatchRequest.communicationProfileId) &&
Objects.equals(this.crmId, accountPatchRequest.crmId) &&
Objects.equals(this.defaultPaymentMethodId, accountPatchRequest.defaultPaymentMethodId) &&
Objects.equals(this.name, accountPatchRequest.name) &&
Objects.equals(this.description, accountPatchRequest.description) &&
Objects.equals(this.parentAccountId, accountPatchRequest.parentAccountId) &&
Objects.equals(this.paymentGateway, accountPatchRequest.paymentGateway) &&
Objects.equals(this.paymentTerms, accountPatchRequest.paymentTerms) &&
Objects.equals(this.sequenceSetId, accountPatchRequest.sequenceSetId) &&
Objects.equals(this.autoPay, accountPatchRequest.autoPay) &&
Objects.equals(this.taxCertificate, accountPatchRequest.taxCertificate) &&
Objects.equals(this.taxIdentifier, accountPatchRequest.taxIdentifier) &&
Objects.equals(this.currency, accountPatchRequest.currency) &&
Objects.equals(this.salesRep, accountPatchRequest.salesRep);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, accountNumber, billingDocumentSettings, batch, billCycleDay, billTo, billToId, soldTo, soldToId, communicationProfileId, crmId, defaultPaymentMethodId, name, description, parentAccountId, paymentGateway, paymentTerms, sequenceSetId, autoPay, taxCertificate, taxIdentifier, currency, salesRep);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AccountPatchRequest {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" billingDocumentSettings: ").append(toIndentedString(billingDocumentSettings)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billTo: ").append(toIndentedString(billTo)).append("\n");
sb.append(" billToId: ").append(toIndentedString(billToId)).append("\n");
sb.append(" soldTo: ").append(toIndentedString(soldTo)).append("\n");
sb.append(" soldToId: ").append(toIndentedString(soldToId)).append("\n");
sb.append(" communicationProfileId: ").append(toIndentedString(communicationProfileId)).append("\n");
sb.append(" crmId: ").append(toIndentedString(crmId)).append("\n");
sb.append(" defaultPaymentMethodId: ").append(toIndentedString(defaultPaymentMethodId)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" parentAccountId: ").append(toIndentedString(parentAccountId)).append("\n");
sb.append(" paymentGateway: ").append(toIndentedString(paymentGateway)).append("\n");
sb.append(" paymentTerms: ").append(toIndentedString(paymentTerms)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" autoPay: ").append(toIndentedString(autoPay)).append("\n");
sb.append(" taxCertificate: ").append(toIndentedString(taxCertificate)).append("\n");
sb.append(" taxIdentifier: ").append(toIndentedString(taxIdentifier)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" salesRep: ").append(toIndentedString(salesRep)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy