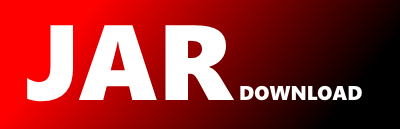
org.openapitools.client.model.AccountPreviewRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* AccountPreviewRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class AccountPreviewRequest {
/**
* Any combination of one-time, recurring, and usage.
*/
@JsonAdapter(ExcludeEnum.Adapter.class)
public enum ExcludeEnum {
ONE_TIME("one_time"),
RECURRING("recurring"),
USAGE("usage"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ExcludeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ExcludeEnum fromValue(String value) {
for (ExcludeEnum b : ExcludeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ExcludeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ExcludeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ExcludeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_EXCLUDE = "exclude";
@SerializedName(SERIALIZED_NAME_EXCLUDE)
private ExcludeEnum exclude;
public static final String SERIALIZED_NAME_INCLUDE_DRAFT_ITEMS = "include_draft_items";
@SerializedName(SERIALIZED_NAME_INCLUDE_DRAFT_ITEMS)
private Boolean includeDraftItems;
public static final String SERIALIZED_NAME_INCLUDE_EVERGREEN_SUBSCRIPTIONS = "include_evergreen_subscriptions";
@SerializedName(SERIALIZED_NAME_INCLUDE_EVERGREEN_SUBSCRIPTIONS)
private Boolean includeEvergreenSubscriptions;
public static final String SERIALIZED_NAME_TARGET_DATE = "target_date";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public AccountPreviewRequest() {
}
public AccountPreviewRequest exclude(ExcludeEnum exclude) {
this.exclude = exclude;
return this;
}
/**
* Any combination of one-time, recurring, and usage.
* @return exclude
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Any combination of one-time, recurring, and usage.")
public ExcludeEnum getExclude() {
return exclude;
}
public void setExclude(ExcludeEnum exclude) {
this.exclude = exclude;
}
public AccountPreviewRequest includeDraftItems(Boolean includeDraftItems) {
this.includeDraftItems = includeDraftItems;
return this;
}
/**
* Indicates whether to include items in the draft status.
* @return includeDraftItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to include items in the draft status.")
public Boolean getIncludeDraftItems() {
return includeDraftItems;
}
public void setIncludeDraftItems(Boolean includeDraftItems) {
this.includeDraftItems = includeDraftItems;
}
public AccountPreviewRequest includeEvergreenSubscriptions(Boolean includeEvergreenSubscriptions) {
this.includeEvergreenSubscriptions = includeEvergreenSubscriptions;
return this;
}
/**
* Indicates whether to include evergreen subscriptions.
* @return includeEvergreenSubscriptions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to include evergreen subscriptions.")
public Boolean getIncludeEvergreenSubscriptions() {
return includeEvergreenSubscriptions;
}
public void setIncludeEvergreenSubscriptions(Boolean includeEvergreenSubscriptions) {
this.includeEvergreenSubscriptions = includeEvergreenSubscriptions;
}
public AccountPreviewRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date is used to determine which charges to bill. All unbilled charges as of or prior to the target date are included. Zuora automatically keeps track of all charges that need to be billed and that have not been billed prior to the target date.
* @return targetDate
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", required = true, value = "The target date is used to determine which charges to bill. All unbilled charges as of or prior to the target date are included. Zuora automatically keeps track of all charges that need to be billed and that have not been billed prior to the target date.")
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AccountPreviewRequest accountPreviewRequest = (AccountPreviewRequest) o;
return Objects.equals(this.exclude, accountPreviewRequest.exclude) &&
Objects.equals(this.includeDraftItems, accountPreviewRequest.includeDraftItems) &&
Objects.equals(this.includeEvergreenSubscriptions, accountPreviewRequest.includeEvergreenSubscriptions) &&
Objects.equals(this.targetDate, accountPreviewRequest.targetDate);
}
@Override
public int hashCode() {
return Objects.hash(exclude, includeDraftItems, includeEvergreenSubscriptions, targetDate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AccountPreviewRequest {\n");
sb.append(" exclude: ").append(toIndentedString(exclude)).append("\n");
sb.append(" includeDraftItems: ").append(toIndentedString(includeDraftItems)).append("\n");
sb.append(" includeEvergreenSubscriptions: ").append(toIndentedString(includeEvergreenSubscriptions)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy