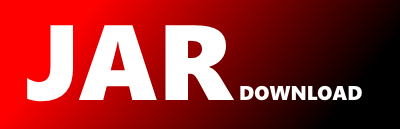
org.openapitools.client.model.ApplePayCreate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.Mandate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* If the `type` of the payment method is `apple_pay`, this hash contains details about the Apple Pay payment method. See [Supported payment methods](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/L_Payment_Methods/A_Supported_Payment_Methods) for payment gateways that support this type of payment method.
*/
@ApiModel(description = "If the `type` of the payment method is `apple_pay`, this hash contains details about the Apple Pay payment method. See [Supported payment methods](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/L_Payment_Methods/A_Supported_Payment_Methods) for payment gateways that support this type of payment method.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class ApplePayCreate {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CUSTOMER_TOKEN = "customer_token";
@SerializedName(SERIALIZED_NAME_CUSTOMER_TOKEN)
private Map customerToken = new HashMap();
public static final String SERIALIZED_NAME_COLLECT_PAYMENT = "collect_payment";
@SerializedName(SERIALIZED_NAME_COLLECT_PAYMENT)
private Boolean collectPayment;
public static final String SERIALIZED_NAME_INVOICE_ID = "invoice_id";
@SerializedName(SERIALIZED_NAME_INVOICE_ID)
private String invoiceId;
public static final String SERIALIZED_NAME_MANDATE = "mandate";
@SerializedName(SERIALIZED_NAME_MANDATE)
private Mandate mandate;
public ApplePayCreate() {
}
public ApplePayCreate id(String id) {
this.id = id;
return this;
}
/**
* The Merchant ID that was configured for use with Apple Pay in the Apple iOS Developer Center.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The Merchant ID that was configured for use with Apple Pay in the Apple iOS Developer Center.")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ApplePayCreate customerToken(Map customerToken) {
this.customerToken = customerToken;
return this;
}
public ApplePayCreate putCustomerTokenItem(String key, Object customerTokenItem) {
this.customerToken.put(key, customerTokenItem);
return this;
}
/**
* The complete JSON Object representing the encrypted payment token payload returned in the response from the Apple Pay session.
* @return customerToken
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The complete JSON Object representing the encrypted payment token payload returned in the response from the Apple Pay session.")
public Map getCustomerToken() {
return customerToken;
}
public void setCustomerToken(Map customerToken) {
this.customerToken = customerToken;
}
public ApplePayCreate collectPayment(Boolean collectPayment) {
this.collectPayment = collectPayment;
return this;
}
/**
* A boolean flag to control whether a payment should be processed after creating payment method. The payment amount will be equivalent to the amount the merchant supplied in the ApplePay session. Default is false. If this field is set to `true`, you must specify the `gateway_id` field with the payment gateway instance name. If this field is set to `false`: - The default payment gateway of your Zuora customer account will be used no matter whether a payment gateway instance is specified in the `gateway_id` field. - You must select the **Verify new credit card** check box on the gateway instance settings page. Otherwise, the cryptogram will not be sent to the gateway. - A separate subscribe or payment API call is required after this payment method creation call.
* @return collectPayment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A boolean flag to control whether a payment should be processed after creating payment method. The payment amount will be equivalent to the amount the merchant supplied in the ApplePay session. Default is false. If this field is set to `true`, you must specify the `gateway_id` field with the payment gateway instance name. If this field is set to `false`: - The default payment gateway of your Zuora customer account will be used no matter whether a payment gateway instance is specified in the `gateway_id` field. - You must select the **Verify new credit card** check box on the gateway instance settings page. Otherwise, the cryptogram will not be sent to the gateway. - A separate subscribe or payment API call is required after this payment method creation call. ")
public Boolean getCollectPayment() {
return collectPayment;
}
public void setCollectPayment(Boolean collectPayment) {
this.collectPayment = collectPayment;
}
public ApplePayCreate invoiceId(String invoiceId) {
this.invoiceId = invoiceId;
return this;
}
/**
* The id of invoice this payment will apply to. Note: When `collect_payment` is true, this field is required. Only one invoice can be paid; for scenarios where you want to pay for multiple invoices, set collect_payment to false and use [Create Payment](#operation/createPayment) API separately.
* @return invoiceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The id of invoice this payment will apply to. Note: When `collect_payment` is true, this field is required. Only one invoice can be paid; for scenarios where you want to pay for multiple invoices, set collect_payment to false and use [Create Payment](#operation/createPayment) API separately.")
public String getInvoiceId() {
return invoiceId;
}
public void setInvoiceId(String invoiceId) {
this.invoiceId = invoiceId;
}
public ApplePayCreate mandate(Mandate mandate) {
this.mandate = mandate;
return this;
}
/**
* Get mandate
* @return mandate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Mandate getMandate() {
return mandate;
}
public void setMandate(Mandate mandate) {
this.mandate = mandate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ApplePayCreate applePayCreate = (ApplePayCreate) o;
return Objects.equals(this.id, applePayCreate.id) &&
Objects.equals(this.customerToken, applePayCreate.customerToken) &&
Objects.equals(this.collectPayment, applePayCreate.collectPayment) &&
Objects.equals(this.invoiceId, applePayCreate.invoiceId) &&
Objects.equals(this.mandate, applePayCreate.mandate);
}
@Override
public int hashCode() {
return Objects.hash(id, customerToken, collectPayment, invoiceId, mandate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ApplePayCreate {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" customerToken: ").append(toIndentedString(customerToken)).append("\n");
sb.append(" collectPayment: ").append(toIndentedString(collectPayment)).append("\n");
sb.append(" invoiceId: ").append(toIndentedString(invoiceId)).append("\n");
sb.append(" mandate: ").append(toIndentedString(mandate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy