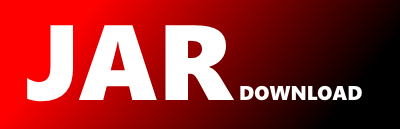
org.openapitools.client.model.ArTransactions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.openapitools.client.model.CreditMemo;
import org.openapitools.client.model.Invoice;
import org.openapitools.client.model.PaymentTransactions;
import org.openapitools.client.model.RefundTransactions;
import org.openapitools.jackson.nullable.JsonNullable;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* ArTransactions
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class ArTransactions {
public static final String SERIALIZED_NAME_CREDIT_MEMO_NUMBERS = "credit_memo_numbers";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_NUMBERS)
private List creditMemoNumbers = null;
public static final String SERIALIZED_NAME_CREDIT_MEMOS = "credit_memos";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMOS)
private CreditMemo creditMemos;
public static final String SERIALIZED_NAME_INVOICE_NUMBERS = "invoice_numbers";
@SerializedName(SERIALIZED_NAME_INVOICE_NUMBERS)
private List invoiceNumbers = null;
public static final String SERIALIZED_NAME_INVOICES = "invoices";
@SerializedName(SERIALIZED_NAME_INVOICES)
private Invoice invoices;
public static final String SERIALIZED_NAME_REFUNDS = "refunds";
@SerializedName(SERIALIZED_NAME_REFUNDS)
private List refunds = null;
public static final String SERIALIZED_NAME_PAYMENTS = "payments";
@SerializedName(SERIALIZED_NAME_PAYMENTS)
private List payments = null;
public ArTransactions() {
}
public ArTransactions creditMemoNumbers(List creditMemoNumbers) {
this.creditMemoNumbers = creditMemoNumbers;
return this;
}
public ArTransactions addCreditMemoNumbersItem(String creditMemoNumbersItem) {
if (this.creditMemoNumbers == null) {
this.creditMemoNumbers = new ArrayList();
}
this.creditMemoNumbers.add(creditMemoNumbersItem);
return this;
}
/**
* Credit memo numbers.
* @return creditMemoNumbers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Credit memo numbers.")
public List getCreditMemoNumbers() {
return creditMemoNumbers;
}
public void setCreditMemoNumbers(List creditMemoNumbers) {
this.creditMemoNumbers = creditMemoNumbers;
}
public ArTransactions creditMemos(CreditMemo creditMemos) {
this.creditMemos = creditMemos;
return this;
}
/**
* The related credit memos.
* @return creditMemos
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The related credit memos.")
public CreditMemo getCreditMemos() {
return creditMemos;
}
public void setCreditMemos(CreditMemo creditMemos) {
this.creditMemos = creditMemos;
}
public ArTransactions invoiceNumbers(List invoiceNumbers) {
this.invoiceNumbers = invoiceNumbers;
return this;
}
public ArTransactions addInvoiceNumbersItem(String invoiceNumbersItem) {
if (this.invoiceNumbers == null) {
this.invoiceNumbers = new ArrayList();
}
this.invoiceNumbers.add(invoiceNumbersItem);
return this;
}
/**
* The related invoice numbers.
* @return invoiceNumbers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The related invoice numbers.")
public List getInvoiceNumbers() {
return invoiceNumbers;
}
public void setInvoiceNumbers(List invoiceNumbers) {
this.invoiceNumbers = invoiceNumbers;
}
public ArTransactions invoices(Invoice invoices) {
this.invoices = invoices;
return this;
}
/**
* The related invoices.
* @return invoices
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The related invoices.")
public Invoice getInvoices() {
return invoices;
}
public void setInvoices(Invoice invoices) {
this.invoices = invoices;
}
public ArTransactions refunds(List refunds) {
this.refunds = refunds;
return this;
}
public ArTransactions addRefundsItem(RefundTransactions refundsItem) {
if (this.refunds == null) {
this.refunds = new ArrayList();
}
this.refunds.add(refundsItem);
return this;
}
/**
* The related refunds.
* @return refunds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The related refunds.")
public List getRefunds() {
return refunds;
}
public void setRefunds(List refunds) {
this.refunds = refunds;
}
public ArTransactions payments(List payments) {
this.payments = payments;
return this;
}
public ArTransactions addPaymentsItem(PaymentTransactions paymentsItem) {
if (this.payments == null) {
this.payments = new ArrayList();
}
this.payments.add(paymentsItem);
return this;
}
/**
* The related payments.
* @return payments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The related payments.")
public List getPayments() {
return payments;
}
public void setPayments(List payments) {
this.payments = payments;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ArTransactions arTransactions = (ArTransactions) o;
return Objects.equals(this.creditMemoNumbers, arTransactions.creditMemoNumbers) &&
Objects.equals(this.creditMemos, arTransactions.creditMemos) &&
Objects.equals(this.invoiceNumbers, arTransactions.invoiceNumbers) &&
Objects.equals(this.invoices, arTransactions.invoices) &&
Objects.equals(this.refunds, arTransactions.refunds) &&
Objects.equals(this.payments, arTransactions.payments);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(creditMemoNumbers, creditMemos, invoiceNumbers, invoices, refunds, payments);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ArTransactions {\n");
sb.append(" creditMemoNumbers: ").append(toIndentedString(creditMemoNumbers)).append("\n");
sb.append(" creditMemos: ").append(toIndentedString(creditMemos)).append("\n");
sb.append(" invoiceNumbers: ").append(toIndentedString(invoiceNumbers)).append("\n");
sb.append(" invoices: ").append(toIndentedString(invoices)).append("\n");
sb.append(" refunds: ").append(toIndentedString(refunds)).append("\n");
sb.append(" payments: ").append(toIndentedString(payments)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy