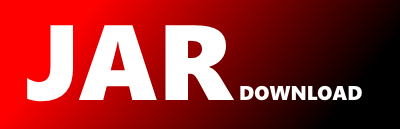
org.openapitools.client.model.BillRunPreviewCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* BillRunPreviewCreateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class BillRunPreviewCreateRequest {
/**
* Indicates whether to generate a preview of future invoice items and credit memo items with the assumption that the subscriptions are renewed. **all**: The assumption is applied to all the subscriptions. Zuora generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the target date. **auto_renew_only**: The assumption is applied to the subscriptions that have auto_renew enabled. Zuora generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the target date.
*/
@JsonAdapter(AssumeRenewalEnum.Adapter.class)
public enum AssumeRenewalEnum {
ALL("all"),
AUTO_RENEW_ONLY("auto_renew_only"),
NONE("none"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
AssumeRenewalEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static AssumeRenewalEnum fromValue(String value) {
for (AssumeRenewalEnum b : AssumeRenewalEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final AssumeRenewalEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public AssumeRenewalEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return AssumeRenewalEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_ASSUME_RENEWAL = "assume_renewal";
@SerializedName(SERIALIZED_NAME_ASSUME_RENEWAL)
private AssumeRenewalEnum assumeRenewal;
public static final String SERIALIZED_NAME_BATCHES = "batches";
@SerializedName(SERIALIZED_NAME_BATCHES)
private List batches = null;
/**
* Gets or Sets chargesExcluded
*/
@JsonAdapter(ChargesExcludedEnum.Adapter.class)
public enum ChargesExcludedEnum {
ONE_TIME("one_time"),
RECURRING("recurring"),
USAGE("usage"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ChargesExcludedEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ChargesExcludedEnum fromValue(String value) {
for (ChargesExcludedEnum b : ChargesExcludedEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ChargesExcludedEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ChargesExcludedEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ChargesExcludedEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_CHARGES_EXCLUDED = "charges_excluded";
@SerializedName(SERIALIZED_NAME_CHARGES_EXCLUDED)
private List chargesExcluded = null;
public static final String SERIALIZED_NAME_INCLUDE_DRAFT_ITEMS = "include_draft_items";
@SerializedName(SERIALIZED_NAME_INCLUDE_DRAFT_ITEMS)
private Boolean includeDraftItems;
public static final String SERIALIZED_NAME_INCLUDE_EVERGREEN_SUBSCRIPTIONS = "include_evergreen_subscriptions";
@SerializedName(SERIALIZED_NAME_INCLUDE_EVERGREEN_SUBSCRIPTIONS)
private Boolean includeEvergreenSubscriptions;
public static final String SERIALIZED_NAME_TARGET_DATE = "target_date";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public BillRunPreviewCreateRequest() {
}
public BillRunPreviewCreateRequest assumeRenewal(AssumeRenewalEnum assumeRenewal) {
this.assumeRenewal = assumeRenewal;
return this;
}
/**
* Indicates whether to generate a preview of future invoice items and credit memo items with the assumption that the subscriptions are renewed. **all**: The assumption is applied to all the subscriptions. Zuora generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the target date. **auto_renew_only**: The assumption is applied to the subscriptions that have auto_renew enabled. Zuora generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the target date.
* @return assumeRenewal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to generate a preview of future invoice items and credit memo items with the assumption that the subscriptions are renewed. **all**: The assumption is applied to all the subscriptions. Zuora generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the target date. **auto_renew_only**: The assumption is applied to the subscriptions that have auto_renew enabled. Zuora generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the target date.")
public AssumeRenewalEnum getAssumeRenewal() {
return assumeRenewal;
}
public void setAssumeRenewal(AssumeRenewalEnum assumeRenewal) {
this.assumeRenewal = assumeRenewal;
}
public BillRunPreviewCreateRequest batches(List batches) {
this.batches = batches;
return this;
}
public BillRunPreviewCreateRequest addBatchesItem(String batchesItem) {
if (this.batches == null) {
this.batches = new ArrayList();
}
this.batches.add(batchesItem);
return this;
}
/**
* Identifiers of the customer account batches to be included in this bill run preview.
* @return batches
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifiers of the customer account batches to be included in this bill run preview.")
public List getBatches() {
return batches;
}
public void setBatches(List batches) {
this.batches = batches;
}
public BillRunPreviewCreateRequest chargesExcluded(List chargesExcluded) {
this.chargesExcluded = chargesExcluded;
return this;
}
public BillRunPreviewCreateRequest addChargesExcludedItem(ChargesExcludedEnum chargesExcludedItem) {
if (this.chargesExcluded == null) {
this.chargesExcluded = new ArrayList();
}
this.chargesExcluded.add(chargesExcludedItem);
return this;
}
/**
* Charge type or types to be excluded from this bill run preview.
* @return chargesExcluded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Charge type or types to be excluded from this bill run preview.")
public List getChargesExcluded() {
return chargesExcluded;
}
public void setChargesExcluded(List chargesExcluded) {
this.chargesExcluded = chargesExcluded;
}
public BillRunPreviewCreateRequest includeDraftItems(Boolean includeDraftItems) {
this.includeDraftItems = includeDraftItems;
return this;
}
/**
* If true, draft items will be included in this bill run preview.
* @return includeDraftItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, draft items will be included in this bill run preview.")
public Boolean getIncludeDraftItems() {
return includeDraftItems;
}
public void setIncludeDraftItems(Boolean includeDraftItems) {
this.includeDraftItems = includeDraftItems;
}
public BillRunPreviewCreateRequest includeEvergreenSubscriptions(Boolean includeEvergreenSubscriptions) {
this.includeEvergreenSubscriptions = includeEvergreenSubscriptions;
return this;
}
/**
* If true, evergreen subscriptions will be included in this bill run preview.
* @return includeEvergreenSubscriptions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, evergreen subscriptions will be included in this bill run preview.")
public Boolean getIncludeEvergreenSubscriptions() {
return includeEvergreenSubscriptions;
}
public void setIncludeEvergreenSubscriptions(Boolean includeEvergreenSubscriptions) {
this.includeEvergreenSubscriptions = includeEvergreenSubscriptions;
}
public BillRunPreviewCreateRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* All unbilled items on or before this date are included in this bill run.
* @return targetDate
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "All unbilled items on or before this date are included in this bill run.")
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BillRunPreviewCreateRequest billRunPreviewCreateRequest = (BillRunPreviewCreateRequest) o;
return Objects.equals(this.assumeRenewal, billRunPreviewCreateRequest.assumeRenewal) &&
Objects.equals(this.batches, billRunPreviewCreateRequest.batches) &&
Objects.equals(this.chargesExcluded, billRunPreviewCreateRequest.chargesExcluded) &&
Objects.equals(this.includeDraftItems, billRunPreviewCreateRequest.includeDraftItems) &&
Objects.equals(this.includeEvergreenSubscriptions, billRunPreviewCreateRequest.includeEvergreenSubscriptions) &&
Objects.equals(this.targetDate, billRunPreviewCreateRequest.targetDate);
}
@Override
public int hashCode() {
return Objects.hash(assumeRenewal, batches, chargesExcluded, includeDraftItems, includeEvergreenSubscriptions, targetDate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BillRunPreviewCreateRequest {\n");
sb.append(" assumeRenewal: ").append(toIndentedString(assumeRenewal)).append("\n");
sb.append(" batches: ").append(toIndentedString(batches)).append("\n");
sb.append(" chargesExcluded: ").append(toIndentedString(chargesExcluded)).append("\n");
sb.append(" includeDraftItems: ").append(toIndentedString(includeDraftItems)).append("\n");
sb.append(" includeEvergreenSubscriptions: ").append(toIndentedString(includeEvergreenSubscriptions)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy