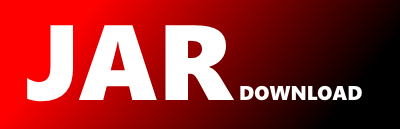
org.openapitools.client.model.BillingDocumentCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.BillingDocumentItemCreateRequest;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* BillingDocumentCreateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class BillingDocumentCreateRequest {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DUE_DATE = "due_date";
@SerializedName(SERIALIZED_NAME_DUE_DATE)
private LocalDate dueDate;
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "document_date";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
public static final String SERIALIZED_NAME_REASON_CODE = "reason_code";
@SerializedName(SERIALIZED_NAME_REASON_CODE)
private String reasonCode;
public static final String SERIALIZED_NAME_INVOICE_ID = "invoice_id";
@SerializedName(SERIALIZED_NAME_INVOICE_ID)
private String invoiceId;
public static final String SERIALIZED_NAME_TRANSFER_TO_ACCOUNTING = "transfer_to_accounting";
@SerializedName(SERIALIZED_NAME_TRANSFER_TO_ACCOUNTING)
private Boolean transferToAccounting;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_EXCLUDE_FROM_AUTO_APPLY_RULES = "exclude_from_auto_apply_rules";
@SerializedName(SERIALIZED_NAME_EXCLUDE_FROM_AUTO_APPLY_RULES)
private Boolean excludeFromAutoApplyRules;
public static final String SERIALIZED_NAME_PAY = "pay";
@SerializedName(SERIALIZED_NAME_PAY)
private Boolean pay;
/**
* The type of billing document. Can be one of the credit memo, debit memo, or invoice.
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
CREDIT_MEMO("credit_memo"),
DEBIT_MEMO("debit_memo"),
INVOICE("invoice"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TypeEnum type;
public static final String SERIALIZED_NAME_ITEMS = "items";
@SerializedName(SERIALIZED_NAME_ITEMS)
private List items = null;
public static final String SERIALIZED_NAME_APPLY = "apply";
@SerializedName(SERIALIZED_NAME_APPLY)
private Boolean apply;
public static final String SERIALIZED_NAME_POST = "post";
@SerializedName(SERIALIZED_NAME_POST)
private Boolean post;
public BillingDocumentCreateRequest() {
}
public BillingDocumentCreateRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the account that owns the billing document.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the billing document.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public BillingDocumentCreateRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Human-readable identifier of the account that owns the billing document.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the account that owns the billing document.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public BillingDocumentCreateRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string associated with the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string associated with the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public BillingDocumentCreateRequest dueDate(LocalDate dueDate) {
this.dueDate = dueDate;
return this;
}
/**
* The date on which payment for the billing document is due.
* @return dueDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "The date on which payment for the billing document is due.")
public LocalDate getDueDate() {
return dueDate;
}
public void setDueDate(LocalDate dueDate) {
this.dueDate = dueDate;
}
public BillingDocumentCreateRequest documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* The date when the billing document takes effect.
* @return documentDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The date when the billing document takes effect.")
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public BillingDocumentCreateRequest reasonCode(String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* Reason for issuing this billing document. This field is applicable only if the `type` field is set to `credit_memo` or `debit_memo`.
* @return reasonCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Reason for issuing this billing document. This field is applicable only if the `type` field is set to `credit_memo` or `debit_memo`.")
public String getReasonCode() {
return reasonCode;
}
public void setReasonCode(String reasonCode) {
this.reasonCode = reasonCode;
}
public BillingDocumentCreateRequest invoiceId(String invoiceId) {
this.invoiceId = invoiceId;
return this;
}
/**
* The identifier of the invoice billing document from which this credit memo or debit memo billing document is created. This field is applicable only if the `type` field is set to `credit_memo` or `debit_memo`.
* @return invoiceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of the invoice billing document from which this credit memo or debit memo billing document is created. This field is applicable only if the `type` field is set to `credit_memo` or `debit_memo`.")
public String getInvoiceId() {
return invoiceId;
}
public void setInvoiceId(String invoiceId) {
this.invoiceId = invoiceId;
}
public BillingDocumentCreateRequest transferToAccounting(Boolean transferToAccounting) {
this.transferToAccounting = transferToAccounting;
return this;
}
/**
* Whether to transfer to an external accounting system.
* @return transferToAccounting
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether to transfer to an external accounting system.")
public Boolean getTransferToAccounting() {
return transferToAccounting;
}
public void setTransferToAccounting(Boolean transferToAccounting) {
this.transferToAccounting = transferToAccounting;
}
public BillingDocumentCreateRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public BillingDocumentCreateRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public BillingDocumentCreateRequest excludeFromAutoApplyRules(Boolean excludeFromAutoApplyRules) {
this.excludeFromAutoApplyRules = excludeFromAutoApplyRules;
return this;
}
/**
* Indicates whether to exclude this credit memo billing document from the rule of automatically applying it to invoices. This field is applicable only if the `type` field is set to `credit_memo`.
* @return excludeFromAutoApplyRules
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to exclude this credit memo billing document from the rule of automatically applying it to invoices. This field is applicable only if the `type` field is set to `credit_memo`.")
public Boolean getExcludeFromAutoApplyRules() {
return excludeFromAutoApplyRules;
}
public void setExcludeFromAutoApplyRules(Boolean excludeFromAutoApplyRules) {
this.excludeFromAutoApplyRules = excludeFromAutoApplyRules;
}
public BillingDocumentCreateRequest pay(Boolean pay) {
this.pay = pay;
return this;
}
/**
* Indicates whether the billing document is automatically picked up for processing in the corresponding payment run.
* @return pay
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether the billing document is automatically picked up for processing in the corresponding payment run.")
public Boolean getPay() {
return pay;
}
public void setPay(Boolean pay) {
this.pay = pay;
}
public BillingDocumentCreateRequest type(TypeEnum type) {
this.type = type;
return this;
}
/**
* The type of billing document. Can be one of the credit memo, debit memo, or invoice.
* @return type
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The type of billing document. Can be one of the credit memo, debit memo, or invoice.")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public BillingDocumentCreateRequest items(List items) {
this.items = items;
return this;
}
public BillingDocumentCreateRequest addItemsItem(BillingDocumentItemCreateRequest itemsItem) {
if (this.items == null) {
this.items = new ArrayList();
}
this.items.add(itemsItem);
return this;
}
/**
* Information of all billing document items.
* @return items
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Information of all billing document items.")
public List getItems() {
return items;
}
public void setItems(List items) {
this.items = items;
}
public BillingDocumentCreateRequest apply(Boolean apply) {
this.apply = apply;
return this;
}
/**
* Whether to automatically apply the billing document upon posting.
* @return apply
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether to automatically apply the billing document upon posting.")
public Boolean getApply() {
return apply;
}
public void setApply(Boolean apply) {
this.apply = apply;
}
public BillingDocumentCreateRequest post(Boolean post) {
this.post = post;
return this;
}
/**
* Whether to automatically post a billing document after it is created.
* @return post
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether to automatically post a billing document after it is created.")
public Boolean getPost() {
return post;
}
public void setPost(Boolean post) {
this.post = post;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BillingDocumentCreateRequest billingDocumentCreateRequest = (BillingDocumentCreateRequest) o;
return Objects.equals(this.accountId, billingDocumentCreateRequest.accountId) &&
Objects.equals(this.accountNumber, billingDocumentCreateRequest.accountNumber) &&
Objects.equals(this.description, billingDocumentCreateRequest.description) &&
Objects.equals(this.dueDate, billingDocumentCreateRequest.dueDate) &&
Objects.equals(this.documentDate, billingDocumentCreateRequest.documentDate) &&
Objects.equals(this.reasonCode, billingDocumentCreateRequest.reasonCode) &&
Objects.equals(this.invoiceId, billingDocumentCreateRequest.invoiceId) &&
Objects.equals(this.transferToAccounting, billingDocumentCreateRequest.transferToAccounting) &&
Objects.equals(this.customFields, billingDocumentCreateRequest.customFields) &&
Objects.equals(this.excludeFromAutoApplyRules, billingDocumentCreateRequest.excludeFromAutoApplyRules) &&
Objects.equals(this.pay, billingDocumentCreateRequest.pay) &&
Objects.equals(this.type, billingDocumentCreateRequest.type) &&
Objects.equals(this.items, billingDocumentCreateRequest.items) &&
Objects.equals(this.apply, billingDocumentCreateRequest.apply) &&
Objects.equals(this.post, billingDocumentCreateRequest.post);
}
@Override
public int hashCode() {
return Objects.hash(accountId, accountNumber, description, dueDate, documentDate, reasonCode, invoiceId, transferToAccounting, customFields, excludeFromAutoApplyRules, pay, type, items, apply, post);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BillingDocumentCreateRequest {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" dueDate: ").append(toIndentedString(dueDate)).append("\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" invoiceId: ").append(toIndentedString(invoiceId)).append("\n");
sb.append(" transferToAccounting: ").append(toIndentedString(transferToAccounting)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" excludeFromAutoApplyRules: ").append(toIndentedString(excludeFromAutoApplyRules)).append("\n");
sb.append(" pay: ").append(toIndentedString(pay)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" items: ").append(toIndentedString(items)).append("\n");
sb.append(" apply: ").append(toIndentedString(apply)).append("\n");
sb.append(" post: ").append(toIndentedString(post)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy