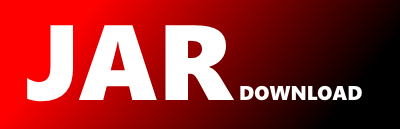
org.openapitools.client.model.BillingDocumentItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.BillingDocument;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.Subscription;
import org.openapitools.client.model.SubscriptionItem;
import org.openapitools.client.model.TaxationItemListResponse;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* BillingDocumentItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class BillingDocumentItem {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_SUBTOTAL = "subtotal";
@SerializedName(SERIALIZED_NAME_SUBTOTAL)
private BigDecimal subtotal;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT = "deferred_revenue_account";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT)
private String deferredRevenueAccount;
public static final String SERIALIZED_NAME_ON_ACCOUNT_ACCOUNT = "on_account_account";
@SerializedName(SERIALIZED_NAME_ON_ACCOUNT_ACCOUNT)
private String onAccountAccount;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT = "recognized_revenue_account";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT)
private String recognizedRevenueAccount;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT = "billing_document";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT)
private BillingDocument billingDocument;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT_ID = "billing_document_id";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT_ID)
private String billingDocumentId;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME = "revenue_recognition_rule_name";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME)
private String revenueRecognitionRuleName;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_SERVICE_END = "service_end";
@SerializedName(SERIALIZED_NAME_SERVICE_END)
private String serviceEnd;
public static final String SERIALIZED_NAME_ACCOUNTS_RECEIVABLE_ACCOUNT = "accounts_receivable_account";
@SerializedName(SERIALIZED_NAME_ACCOUNTS_RECEIVABLE_ACCOUNT)
private String accountsReceivableAccount;
public static final String SERIALIZED_NAME_DISCOUNT_ITEM = "discount_item";
@SerializedName(SERIALIZED_NAME_DISCOUNT_ITEM)
private Boolean discountItem;
public static final String SERIALIZED_NAME_APPLIED_TO_ITEM_ID = "applied_to_item_id";
@SerializedName(SERIALIZED_NAME_APPLIED_TO_ITEM_ID)
private String appliedToItemId;
public static final String SERIALIZED_NAME_SERVICE_START = "service_start";
@SerializedName(SERIALIZED_NAME_SERVICE_START)
private String serviceStart;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accounting_code";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_SKU = "sku";
@SerializedName(SERIALIZED_NAME_SKU)
private String sku;
public static final String SERIALIZED_NAME_PRODUCT_NAME = "product_name";
@SerializedName(SERIALIZED_NAME_PRODUCT_NAME)
private String productName;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ID = "subscription_id";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ID)
private String subscriptionId;
public static final String SERIALIZED_NAME_SUBSCRIPTION = "subscription";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION)
private Subscription subscription;
public static final String SERIALIZED_NAME_TAX_INCLUSIVE = "tax_inclusive";
@SerializedName(SERIALIZED_NAME_TAX_INCLUSIVE)
private Boolean taxInclusive;
public static final String SERIALIZED_NAME_REMAINING_BALANCE = "remaining_balance";
@SerializedName(SERIALIZED_NAME_REMAINING_BALANCE)
private BigDecimal remainingBalance;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unit_of_measure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public static final String SERIALIZED_NAME_UNIT_AMOUNT = "unit_amount";
@SerializedName(SERIALIZED_NAME_UNIT_AMOUNT)
private BigDecimal unitAmount;
public static final String SERIALIZED_NAME_BOOKING_REFERENCE = "booking_reference";
@SerializedName(SERIALIZED_NAME_BOOKING_REFERENCE)
private String bookingReference;
public static final String SERIALIZED_NAME_PRICE_DESCRIPTION = "price_description";
@SerializedName(SERIALIZED_NAME_PRICE_DESCRIPTION)
private String priceDescription;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_PRICE_ID = "price_id";
@SerializedName(SERIALIZED_NAME_PRICE_ID)
private String priceId;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchase_order_number";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_TAX = "tax";
@SerializedName(SERIALIZED_NAME_TAX)
private BigDecimal tax;
public static final String SERIALIZED_NAME_TAX_CODE = "tax_code";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_ID = "subscription_item_id";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_ID)
private String subscriptionItemId;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM = "subscription_item";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM)
private SubscriptionItem subscriptionItem;
public static final String SERIALIZED_NAME_INVOICE_ITEM_ID = "invoice_item_id";
@SerializedName(SERIALIZED_NAME_INVOICE_ITEM_ID)
private String invoiceItemId;
public static final String SERIALIZED_NAME_DOCUMENT_ITEM_DATE = "document_item_date";
@SerializedName(SERIALIZED_NAME_DOCUMENT_ITEM_DATE)
private OffsetDateTime documentItemDate;
public static final String SERIALIZED_NAME_TAXATION_ITEMS = "taxation_items";
@SerializedName(SERIALIZED_NAME_TAXATION_ITEMS)
private TaxationItemListResponse taxationItems;
/**
* The type of billing document, one of credit_memo, debit_memo or invoice.
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
CREDIT_MEMO("credit_memo"),
DEBIT_MEMO("debit_memo"),
INVOICE("invoice"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TypeEnum type;
public BillingDocumentItem() {
}
public BillingDocumentItem(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public BillingDocumentItem customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public BillingDocumentItem putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public BillingDocumentItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The total amount of this billing document item.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount of this billing document item.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public BillingDocumentItem subtotal(BigDecimal subtotal) {
this.subtotal = subtotal;
return this;
}
/**
* The total amount of this billing document item exclusive of tax.
* @return subtotal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount of this billing document item exclusive of tax.")
public BigDecimal getSubtotal() {
return subtotal;
}
public void setSubtotal(BigDecimal subtotal) {
this.subtotal = subtotal;
}
public BillingDocumentItem description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string associated with the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string associated with the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public BillingDocumentItem deferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
return this;
}
/**
* The accounting code for the deferred revenue, such as Monthly Recurring Liability.
* @return deferredRevenueAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The accounting code for the deferred revenue, such as Monthly Recurring Liability.")
public String getDeferredRevenueAccount() {
return deferredRevenueAccount;
}
public void setDeferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
}
public BillingDocumentItem onAccountAccount(String onAccountAccount) {
this.onAccountAccount = onAccountAccount;
return this;
}
/**
* The accounting code that maps to an on account in your accounting system.
* @return onAccountAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The accounting code that maps to an on account in your accounting system.")
public String getOnAccountAccount() {
return onAccountAccount;
}
public void setOnAccountAccount(String onAccountAccount) {
this.onAccountAccount = onAccountAccount;
}
public BillingDocumentItem recognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
return this;
}
/**
* The accounting code for the recognized revenue, such as Monthly Recurring Charges or Overage Charges.
* @return recognizedRevenueAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The accounting code for the recognized revenue, such as Monthly Recurring Charges or Overage Charges.")
public String getRecognizedRevenueAccount() {
return recognizedRevenueAccount;
}
public void setRecognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
}
public BillingDocumentItem billingDocument(BillingDocument billingDocument) {
this.billingDocument = billingDocument;
return this;
}
/**
* The related billing document.
* @return billingDocument
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The related billing document.")
public BillingDocument getBillingDocument() {
return billingDocument;
}
public void setBillingDocument(BillingDocument billingDocument) {
this.billingDocument = billingDocument;
}
public BillingDocumentItem billingDocumentId(String billingDocumentId) {
this.billingDocumentId = billingDocumentId;
return this;
}
/**
* The related billing document id.
* @return billingDocumentId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The related billing document id.")
public String getBillingDocumentId() {
return billingDocumentId;
}
public void setBillingDocumentId(String billingDocumentId) {
this.billingDocumentId = billingDocumentId;
}
public BillingDocumentItem revenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
return this;
}
/**
* The name of the revenue recognition rule governing the revenue schedule.
* @return revenueRecognitionRuleName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the revenue recognition rule governing the revenue schedule.")
public String getRevenueRecognitionRuleName() {
return revenueRecognitionRuleName;
}
public void setRevenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
}
public BillingDocumentItem quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The number of units of this item.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of units of this item.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public BillingDocumentItem serviceEnd(String serviceEnd) {
this.serviceEnd = serviceEnd;
return this;
}
/**
* The end date of the service period associated with this billing document item. If the associated charge is a one-time fee, then this date is the date of that charge.
* @return serviceEnd
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The end date of the service period associated with this billing document item. If the associated charge is a one-time fee, then this date is the date of that charge.")
public String getServiceEnd() {
return serviceEnd;
}
public void setServiceEnd(String serviceEnd) {
this.serviceEnd = serviceEnd;
}
public BillingDocumentItem accountsReceivableAccount(String accountsReceivableAccount) {
this.accountsReceivableAccount = accountsReceivableAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return accountsReceivableAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getAccountsReceivableAccount() {
return accountsReceivableAccount;
}
public void setAccountsReceivableAccount(String accountsReceivableAccount) {
this.accountsReceivableAccount = accountsReceivableAccount;
}
public BillingDocumentItem discountItem(Boolean discountItem) {
this.discountItem = discountItem;
return this;
}
/**
* If true, indicates that the item is a discount item.
* @return discountItem
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, indicates that the item is a discount item.")
public Boolean getDiscountItem() {
return discountItem;
}
public void setDiscountItem(Boolean discountItem) {
this.discountItem = discountItem;
}
public BillingDocumentItem appliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
return this;
}
/**
* Identifier of an invoice item or a debit memo item that this discount item or credit memo item is applied to.
* @return appliedToItemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of an invoice item or a debit memo item that this discount item or credit memo item is applied to.")
public String getAppliedToItemId() {
return appliedToItemId;
}
public void setAppliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
}
public BillingDocumentItem serviceStart(String serviceStart) {
this.serviceStart = serviceStart;
return this;
}
/**
* The start date of the service period associated with this billing document item. If the associated charge is a one-time fee, then this date is the date of that charge.
* @return serviceStart
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The start date of the service period associated with this billing document item. If the associated charge is a one-time fee, then this date is the date of that charge.")
public String getServiceStart() {
return serviceStart;
}
public void setServiceStart(String serviceStart) {
this.serviceStart = serviceStart;
}
public BillingDocumentItem accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* Get accountingCode
* @return accountingCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public BillingDocumentItem sku(String sku) {
this.sku = sku;
return this;
}
/**
* The unique SKU (stock keeping unit) of the product associated with this item.
* @return sku
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unique SKU (stock keeping unit) of the product associated with this item.")
public String getSku() {
return sku;
}
public void setSku(String sku) {
this.sku = sku;
}
public BillingDocumentItem productName(String productName) {
this.productName = productName;
return this;
}
/**
* The name of the product associated with this item.
* @return productName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the product associated with this item.")
public String getProductName() {
return productName;
}
public void setProductName(String productName) {
this.productName = productName;
}
public BillingDocumentItem subscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* The identifier of the subscription associated with the billing document item.
* @return subscriptionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of the subscription associated with the billing document item.")
public String getSubscriptionId() {
return subscriptionId;
}
public void setSubscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
}
public BillingDocumentItem subscription(Subscription subscription) {
this.subscription = subscription;
return this;
}
/**
* The expandable subscription associated with the billing document item.
* @return subscription
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The expandable subscription associated with the billing document item.")
public Subscription getSubscription() {
return subscription;
}
public void setSubscription(Subscription subscription) {
this.subscription = subscription;
}
public BillingDocumentItem taxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
return this;
}
/**
* This specifies if the billing document item amount is inclusive or exclusive of tax.
* @return taxInclusive
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "This specifies if the billing document item amount is inclusive or exclusive of tax.")
public Boolean getTaxInclusive() {
return taxInclusive;
}
public void setTaxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
}
public BillingDocumentItem remainingBalance(BigDecimal remainingBalance) {
this.remainingBalance = remainingBalance;
return this;
}
/**
* The remaining balance of this billing document item.
* @return remainingBalance
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The remaining balance of this billing document item.")
public BigDecimal getRemainingBalance() {
return remainingBalance;
}
public void setRemainingBalance(BigDecimal remainingBalance) {
this.remainingBalance = remainingBalance;
}
public BillingDocumentItem unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* Specifies the units used to measure usage.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the units used to measure usage.")
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
public BillingDocumentItem unitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* Unit amount (in the currency specified) of the billing document item.
* @return unitAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unit amount (in the currency specified) of the billing document item.")
public BigDecimal getUnitAmount() {
return unitAmount;
}
public void setUnitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
}
public BillingDocumentItem bookingReference(String bookingReference) {
this.bookingReference = bookingReference;
return this;
}
/**
* The booking reference for this billing document item.
* @return bookingReference
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The booking reference for this billing document item.")
public String getBookingReference() {
return bookingReference;
}
public void setBookingReference(String bookingReference) {
this.bookingReference = bookingReference;
}
public BillingDocumentItem priceDescription(String priceDescription) {
this.priceDescription = priceDescription;
return this;
}
/**
* The description of the price this billing document item is associated with.
* @return priceDescription
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The description of the price this billing document item is associated with.")
public String getPriceDescription() {
return priceDescription;
}
public void setPriceDescription(String priceDescription) {
this.priceDescription = priceDescription;
}
public BillingDocumentItem name(String name) {
this.name = name;
return this;
}
/**
* Name of the billing document item displayed to customers on the billing document.
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the billing document item displayed to customers on the billing document.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public BillingDocumentItem priceId(String priceId) {
this.priceId = priceId;
return this;
}
/**
* The identifier of the price this billing document item is associated with.
* @return priceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of the price this billing document item is associated with.")
public String getPriceId() {
return priceId;
}
public void setPriceId(String priceId) {
this.priceId = priceId;
}
public BillingDocumentItem purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* The purchase order number associated with this billing document item.
* @return purchaseOrderNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The purchase order number associated with this billing document item.")
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public BillingDocumentItem tax(BigDecimal tax) {
this.tax = tax;
return this;
}
/**
* The amount of tax applied to the billing document item.
* @return tax
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of tax applied to the billing document item.")
public BigDecimal getTax() {
return tax;
}
public void setTax(BigDecimal tax) {
this.tax = tax;
}
public BillingDocumentItem taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* The designated tax code.
* @return taxCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The designated tax code.")
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public BillingDocumentItem subscriptionItemId(String subscriptionItemId) {
this.subscriptionItemId = subscriptionItemId;
return this;
}
/**
* The identifier the subscription item associated with this billing document item.
* @return subscriptionItemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier the subscription item associated with this billing document item.")
public String getSubscriptionItemId() {
return subscriptionItemId;
}
public void setSubscriptionItemId(String subscriptionItemId) {
this.subscriptionItemId = subscriptionItemId;
}
public BillingDocumentItem subscriptionItem(SubscriptionItem subscriptionItem) {
this.subscriptionItem = subscriptionItem;
return this;
}
/**
* The expandable subscription item associated with this billing document item.
* @return subscriptionItem
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The expandable subscription item associated with this billing document item.")
public SubscriptionItem getSubscriptionItem() {
return subscriptionItem;
}
public void setSubscriptionItem(SubscriptionItem subscriptionItem) {
this.subscriptionItem = subscriptionItem;
}
public BillingDocumentItem invoiceItemId(String invoiceItemId) {
this.invoiceItemId = invoiceItemId;
return this;
}
/**
* The identifier of the invoice item associated with this billing document item.
* @return invoiceItemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of the invoice item associated with this billing document item.")
public String getInvoiceItemId() {
return invoiceItemId;
}
public void setInvoiceItemId(String invoiceItemId) {
this.invoiceItemId = invoiceItemId;
}
public BillingDocumentItem documentItemDate(OffsetDateTime documentItemDate) {
this.documentItemDate = documentItemDate;
return this;
}
/**
* The date when the billing document item takes effect.
* @return documentItemDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2022-01-01T07:08:12-07:00", value = "The date when the billing document item takes effect.")
public OffsetDateTime getDocumentItemDate() {
return documentItemDate;
}
public void setDocumentItemDate(OffsetDateTime documentItemDate) {
this.documentItemDate = documentItemDate;
}
public BillingDocumentItem taxationItems(TaxationItemListResponse taxationItems) {
this.taxationItems = taxationItems;
return this;
}
/**
* List of taxation items.
* @return taxationItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of taxation items.")
public TaxationItemListResponse getTaxationItems() {
return taxationItems;
}
public void setTaxationItems(TaxationItemListResponse taxationItems) {
this.taxationItems = taxationItems;
}
public BillingDocumentItem type(TypeEnum type) {
this.type = type;
return this;
}
/**
* The type of billing document, one of credit_memo, debit_memo or invoice.
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The type of billing document, one of credit_memo, debit_memo or invoice.")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BillingDocumentItem billingDocumentItem = (BillingDocumentItem) o;
return Objects.equals(this.id, billingDocumentItem.id) &&
Objects.equals(this.updatedById, billingDocumentItem.updatedById) &&
Objects.equals(this.updatedTime, billingDocumentItem.updatedTime) &&
Objects.equals(this.createdById, billingDocumentItem.createdById) &&
Objects.equals(this.createdTime, billingDocumentItem.createdTime) &&
Objects.equals(this.customFields, billingDocumentItem.customFields) &&
Objects.equals(this.customObjects, billingDocumentItem.customObjects) &&
Objects.equals(this.amount, billingDocumentItem.amount) &&
Objects.equals(this.subtotal, billingDocumentItem.subtotal) &&
Objects.equals(this.description, billingDocumentItem.description) &&
Objects.equals(this.deferredRevenueAccount, billingDocumentItem.deferredRevenueAccount) &&
Objects.equals(this.onAccountAccount, billingDocumentItem.onAccountAccount) &&
Objects.equals(this.recognizedRevenueAccount, billingDocumentItem.recognizedRevenueAccount) &&
Objects.equals(this.billingDocument, billingDocumentItem.billingDocument) &&
Objects.equals(this.billingDocumentId, billingDocumentItem.billingDocumentId) &&
Objects.equals(this.revenueRecognitionRuleName, billingDocumentItem.revenueRecognitionRuleName) &&
Objects.equals(this.quantity, billingDocumentItem.quantity) &&
Objects.equals(this.serviceEnd, billingDocumentItem.serviceEnd) &&
Objects.equals(this.accountsReceivableAccount, billingDocumentItem.accountsReceivableAccount) &&
Objects.equals(this.discountItem, billingDocumentItem.discountItem) &&
Objects.equals(this.appliedToItemId, billingDocumentItem.appliedToItemId) &&
Objects.equals(this.serviceStart, billingDocumentItem.serviceStart) &&
Objects.equals(this.accountingCode, billingDocumentItem.accountingCode) &&
Objects.equals(this.sku, billingDocumentItem.sku) &&
Objects.equals(this.productName, billingDocumentItem.productName) &&
Objects.equals(this.subscriptionId, billingDocumentItem.subscriptionId) &&
Objects.equals(this.subscription, billingDocumentItem.subscription) &&
Objects.equals(this.taxInclusive, billingDocumentItem.taxInclusive) &&
Objects.equals(this.remainingBalance, billingDocumentItem.remainingBalance) &&
Objects.equals(this.unitOfMeasure, billingDocumentItem.unitOfMeasure) &&
Objects.equals(this.unitAmount, billingDocumentItem.unitAmount) &&
Objects.equals(this.bookingReference, billingDocumentItem.bookingReference) &&
Objects.equals(this.priceDescription, billingDocumentItem.priceDescription) &&
Objects.equals(this.name, billingDocumentItem.name) &&
Objects.equals(this.priceId, billingDocumentItem.priceId) &&
Objects.equals(this.purchaseOrderNumber, billingDocumentItem.purchaseOrderNumber) &&
Objects.equals(this.tax, billingDocumentItem.tax) &&
Objects.equals(this.taxCode, billingDocumentItem.taxCode) &&
Objects.equals(this.subscriptionItemId, billingDocumentItem.subscriptionItemId) &&
Objects.equals(this.subscriptionItem, billingDocumentItem.subscriptionItem) &&
Objects.equals(this.invoiceItemId, billingDocumentItem.invoiceItemId) &&
Objects.equals(this.documentItemDate, billingDocumentItem.documentItemDate) &&
Objects.equals(this.taxationItems, billingDocumentItem.taxationItems) &&
Objects.equals(this.type, billingDocumentItem.type);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, amount, subtotal, description, deferredRevenueAccount, onAccountAccount, recognizedRevenueAccount, billingDocument, billingDocumentId, revenueRecognitionRuleName, quantity, serviceEnd, accountsReceivableAccount, discountItem, appliedToItemId, serviceStart, accountingCode, sku, productName, subscriptionId, subscription, taxInclusive, remainingBalance, unitOfMeasure, unitAmount, bookingReference, priceDescription, name, priceId, purchaseOrderNumber, tax, taxCode, subscriptionItemId, subscriptionItem, invoiceItemId, documentItemDate, taxationItems, type);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BillingDocumentItem {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" subtotal: ").append(toIndentedString(subtotal)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" deferredRevenueAccount: ").append(toIndentedString(deferredRevenueAccount)).append("\n");
sb.append(" onAccountAccount: ").append(toIndentedString(onAccountAccount)).append("\n");
sb.append(" recognizedRevenueAccount: ").append(toIndentedString(recognizedRevenueAccount)).append("\n");
sb.append(" billingDocument: ").append(toIndentedString(billingDocument)).append("\n");
sb.append(" billingDocumentId: ").append(toIndentedString(billingDocumentId)).append("\n");
sb.append(" revenueRecognitionRuleName: ").append(toIndentedString(revenueRecognitionRuleName)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" serviceEnd: ").append(toIndentedString(serviceEnd)).append("\n");
sb.append(" accountsReceivableAccount: ").append(toIndentedString(accountsReceivableAccount)).append("\n");
sb.append(" discountItem: ").append(toIndentedString(discountItem)).append("\n");
sb.append(" appliedToItemId: ").append(toIndentedString(appliedToItemId)).append("\n");
sb.append(" serviceStart: ").append(toIndentedString(serviceStart)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" sku: ").append(toIndentedString(sku)).append("\n");
sb.append(" productName: ").append(toIndentedString(productName)).append("\n");
sb.append(" subscriptionId: ").append(toIndentedString(subscriptionId)).append("\n");
sb.append(" subscription: ").append(toIndentedString(subscription)).append("\n");
sb.append(" taxInclusive: ").append(toIndentedString(taxInclusive)).append("\n");
sb.append(" remainingBalance: ").append(toIndentedString(remainingBalance)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append(" unitAmount: ").append(toIndentedString(unitAmount)).append("\n");
sb.append(" bookingReference: ").append(toIndentedString(bookingReference)).append("\n");
sb.append(" priceDescription: ").append(toIndentedString(priceDescription)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" priceId: ").append(toIndentedString(priceId)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" tax: ").append(toIndentedString(tax)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" subscriptionItemId: ").append(toIndentedString(subscriptionItemId)).append("\n");
sb.append(" subscriptionItem: ").append(toIndentedString(subscriptionItem)).append("\n");
sb.append(" invoiceItemId: ").append(toIndentedString(invoiceItemId)).append("\n");
sb.append(" documentItemDate: ").append(toIndentedString(documentItemDate)).append("\n");
sb.append(" taxationItems: ").append(toIndentedString(taxationItems)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy