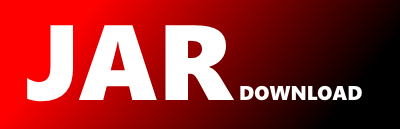
org.openapitools.client.model.CardMandate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.openapitools.client.model.CustomerAcceptanceMandate;
import org.openapitools.jackson.nullable.JsonNullable;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* A mandate is a record of the permission a customer has given you to debit their payment method. This hash contains details about the mandate.
*/
@ApiModel(description = "A mandate is a record of the permission a customer has given you to debit their payment method. This hash contains details about the mandate.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class CardMandate {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_REASON = "reason";
@SerializedName(SERIALIZED_NAME_REASON)
private String reason;
/**
* The status of the mandate, which indicates whether it can be used to initiate a payment.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
ACTIVE("active"),
CANCELED("canceled"),
EXPIRED("expired"),
AGREED("agreed"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_NETWORK_TRANSACTION_ID = "network_transaction_id";
@SerializedName(SERIALIZED_NAME_NETWORK_TRANSACTION_ID)
private String networkTransactionId;
public static final String SERIALIZED_NAME_CUSTOMER_ACCEPTANCE = "customer_acceptance";
@SerializedName(SERIALIZED_NAME_CUSTOMER_ACCEPTANCE)
private CustomerAcceptanceMandate customerAcceptance;
/**
* Indicates the type of the stored credential profile, if any.
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
MULTI_USE("multi_use"),
SINGLE_USE("single_use"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TypeEnum type;
public static final String SERIALIZED_NAME_VERIFIED = "verified";
@SerializedName(SERIALIZED_NAME_VERIFIED)
private Boolean verified;
public static final String SERIALIZED_NAME_VERIFY = "verify";
@SerializedName(SERIALIZED_NAME_VERIFY)
private Boolean verify;
public CardMandate() {
}
public CardMandate(
StateEnum state,
String networkTransactionId,
CustomerAcceptanceMandate customerAcceptance,
TypeEnum type,
Boolean verified
) {
this();
this.state = state;
this.networkTransactionId = networkTransactionId;
this.customerAcceptance = customerAcceptance;
this.type = type;
this.verified = verified;
}
public CardMandate id(String id) {
this.id = id;
return this;
}
/**
* Identifier of the single- or multi-use mandate generated by the payment gateway.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the single- or multi-use mandate generated by the payment gateway.")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public CardMandate reason(String reason) {
this.reason = reason;
return this;
}
/**
* Reason for the mandate.
* @return reason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Reason for the mandate.")
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
/**
* The status of the mandate, which indicates whether it can be used to initiate a payment.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The status of the mandate, which indicates whether it can be used to initiate a payment.")
public StateEnum getState() {
return state;
}
/**
* Identifier of a network transaction.
* @return networkTransactionId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1234567890", value = "Identifier of a network transaction.")
public String getNetworkTransactionId() {
return networkTransactionId;
}
/**
* A reference to the consent agreement you have with your customer.
* @return customerAcceptance
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A reference to the consent agreement you have with your customer.")
public CustomerAcceptanceMandate getCustomerAcceptance() {
return customerAcceptance;
}
/**
* Indicates the type of the stored credential profile, if any.
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates the type of the stored credential profile, if any.")
public TypeEnum getType() {
return type;
}
/**
* Indicates the mandate was verified with the payment gateway by Zuora.
* @return verified
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "true", value = "Indicates the mandate was verified with the payment gateway by Zuora.")
public Boolean getVerified() {
return verified;
}
public CardMandate verify(Boolean verify) {
this.verify = verify;
return this;
}
/**
* Specifies if Zuora should verify this mandate.
* @return verify
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Specifies if Zuora should verify this mandate.")
public Boolean getVerify() {
return verify;
}
public void setVerify(Boolean verify) {
this.verify = verify;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CardMandate cardMandate = (CardMandate) o;
return Objects.equals(this.id, cardMandate.id) &&
Objects.equals(this.reason, cardMandate.reason) &&
Objects.equals(this.state, cardMandate.state) &&
Objects.equals(this.networkTransactionId, cardMandate.networkTransactionId) &&
Objects.equals(this.customerAcceptance, cardMandate.customerAcceptance) &&
Objects.equals(this.type, cardMandate.type) &&
Objects.equals(this.verified, cardMandate.verified) &&
Objects.equals(this.verify, cardMandate.verify);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, reason, state, networkTransactionId, customerAcceptance, type, verified, verify);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CardMandate {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" reason: ").append(toIndentedString(reason)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" networkTransactionId: ").append(toIndentedString(networkTransactionId)).append("\n");
sb.append(" customerAcceptance: ").append(toIndentedString(customerAcceptance)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" verified: ").append(toIndentedString(verified)).append("\n");
sb.append(" verify: ").append(toIndentedString(verify)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy