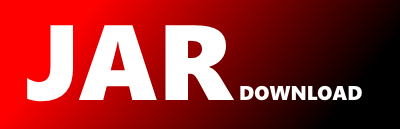
org.openapitools.client.model.ContactCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.Address;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* ContactCreateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class ContactCreateRequest {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_ADDRESS = "address";
@SerializedName(SERIALIZED_NAME_ADDRESS)
private Address address;
public static final String SERIALIZED_NAME_FIRST_NAME = "first_name";
@SerializedName(SERIALIZED_NAME_FIRST_NAME)
private String firstName;
public static final String SERIALIZED_NAME_HOME_PHONE = "home_phone";
@SerializedName(SERIALIZED_NAME_HOME_PHONE)
private String homePhone;
public static final String SERIALIZED_NAME_LAST_NAME = "last_name";
@SerializedName(SERIALIZED_NAME_LAST_NAME)
private String lastName;
public static final String SERIALIZED_NAME_MOBILE_PHONE = "mobile_phone";
@SerializedName(SERIALIZED_NAME_MOBILE_PHONE)
private String mobilePhone;
public static final String SERIALIZED_NAME_NICKNAME = "nickname";
@SerializedName(SERIALIZED_NAME_NICKNAME)
private String nickname;
public static final String SERIALIZED_NAME_OTHER_PHONE = "other_phone";
@SerializedName(SERIALIZED_NAME_OTHER_PHONE)
private String otherPhone;
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
public static final String SERIALIZED_NAME_TAX_REGION = "tax_region";
@SerializedName(SERIALIZED_NAME_TAX_REGION)
private String taxRegion;
public static final String SERIALIZED_NAME_WORK_EMAIL = "work_email";
@SerializedName(SERIALIZED_NAME_WORK_EMAIL)
private String workEmail;
public static final String SERIALIZED_NAME_WORK_PHONE = "work_phone";
@SerializedName(SERIALIZED_NAME_WORK_PHONE)
private String workPhone;
/**
* The type of the additional phone number.
*/
@JsonAdapter(OtherPhoneTypeEnum.Adapter.class)
public enum OtherPhoneTypeEnum {
WORK("work"),
MOBILE("mobile"),
HOME("home"),
OTHER("other"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
OtherPhoneTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static OtherPhoneTypeEnum fromValue(String value) {
for (OtherPhoneTypeEnum b : OtherPhoneTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final OtherPhoneTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public OtherPhoneTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return OtherPhoneTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_OTHER_PHONE_TYPE = "other_phone_type";
@SerializedName(SERIALIZED_NAME_OTHER_PHONE_TYPE)
private OtherPhoneTypeEnum otherPhoneType;
public static final String SERIALIZED_NAME_FAX = "fax";
@SerializedName(SERIALIZED_NAME_FAX)
private String fax;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public ContactCreateRequest() {
}
public ContactCreateRequest(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public ContactCreateRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public ContactCreateRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public ContactCreateRequest address(Address address) {
this.address = address;
return this;
}
/**
* Get address
* @return address
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
public ContactCreateRequest firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* Customer first name.
* @return firstName
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "Amy", required = true, value = "Customer first name.")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public ContactCreateRequest homePhone(String homePhone) {
this.homePhone = homePhone;
return this;
}
/**
* Customer home phone (including extension).
* @return homePhone
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "(888)976-9056", value = "Customer home phone (including extension).")
public String getHomePhone() {
return homePhone;
}
public void setHomePhone(String homePhone) {
this.homePhone = homePhone;
}
public ContactCreateRequest lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Customer last name.
* @return lastName
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "Lawrence", required = true, value = "Customer last name.")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public ContactCreateRequest mobilePhone(String mobilePhone) {
this.mobilePhone = mobilePhone;
return this;
}
/**
* Customer phone (including extension).
* @return mobilePhone
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "(888)101-0011", value = "Customer phone (including extension).")
public String getMobilePhone() {
return mobilePhone;
}
public void setMobilePhone(String mobilePhone) {
this.mobilePhone = mobilePhone;
}
public ContactCreateRequest nickname(String nickname) {
this.nickname = nickname;
return this;
}
/**
* Nickname for this contact.
* @return nickname
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Ami", value = "Nickname for this contact.")
public String getNickname() {
return nickname;
}
public void setNickname(String nickname) {
this.nickname = nickname;
}
public ContactCreateRequest otherPhone(String otherPhone) {
this.otherPhone = otherPhone;
return this;
}
/**
* Other customer phone (including extension).
* @return otherPhone
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "(888)100-0001", value = "Other customer phone (including extension).")
public String getOtherPhone() {
return otherPhone;
}
public void setOtherPhone(String otherPhone) {
this.otherPhone = otherPhone;
}
public ContactCreateRequest email(String email) {
this.email = email;
return this;
}
/**
* Customer email address.
* @return email
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[email protected]", value = "Customer email address.")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public ContactCreateRequest taxRegion(String taxRegion) {
this.taxRegion = taxRegion;
return this;
}
/**
* A region defined in your Zuora Tax rules.
* @return taxRegion
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Georgia", value = "A region defined in your Zuora Tax rules.")
public String getTaxRegion() {
return taxRegion;
}
public void setTaxRegion(String taxRegion) {
this.taxRegion = taxRegion;
}
public ContactCreateRequest workEmail(String workEmail) {
this.workEmail = workEmail;
return this;
}
/**
* Customer work email.
* @return workEmail
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[email protected]", value = "Customer work email.")
public String getWorkEmail() {
return workEmail;
}
public void setWorkEmail(String workEmail) {
this.workEmail = workEmail;
}
public ContactCreateRequest workPhone(String workPhone) {
this.workPhone = workPhone;
return this;
}
/**
* Customer work phone.
* @return workPhone
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "(888)976-9056", value = "Customer work phone.")
public String getWorkPhone() {
return workPhone;
}
public void setWorkPhone(String workPhone) {
this.workPhone = workPhone;
}
public ContactCreateRequest otherPhoneType(OtherPhoneTypeEnum otherPhoneType) {
this.otherPhoneType = otherPhoneType;
return this;
}
/**
* The type of the additional phone number.
* @return otherPhoneType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The type of the additional phone number.")
public OtherPhoneTypeEnum getOtherPhoneType() {
return otherPhoneType;
}
public void setOtherPhoneType(OtherPhoneTypeEnum otherPhoneType) {
this.otherPhoneType = otherPhoneType;
}
public ContactCreateRequest fax(String fax) {
this.fax = fax;
return this;
}
/**
* The contact's fax number.
* @return fax
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The contact's fax number.")
public String getFax() {
return fax;
}
public void setFax(String fax) {
this.fax = fax;
}
public ContactCreateRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of a customer account with which this contact is associated. Either `account_id` or `account_number` is required.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "Identifier of a customer account with which this contact is associated. Either `account_id` or `account_number` is required.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public ContactCreateRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Human-readable identifier of the account with which this contact is associated. Either `account_number` or `account_id` is required.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "RC-00020831", value = "Human-readable identifier of the account with which this contact is associated. Either `account_number` or `account_id` is required.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ContactCreateRequest contactCreateRequest = (ContactCreateRequest) o;
return Objects.equals(this.id, contactCreateRequest.id) &&
Objects.equals(this.updatedById, contactCreateRequest.updatedById) &&
Objects.equals(this.updatedTime, contactCreateRequest.updatedTime) &&
Objects.equals(this.createdById, contactCreateRequest.createdById) &&
Objects.equals(this.createdTime, contactCreateRequest.createdTime) &&
Objects.equals(this.customFields, contactCreateRequest.customFields) &&
Objects.equals(this.customObjects, contactCreateRequest.customObjects) &&
Objects.equals(this.address, contactCreateRequest.address) &&
Objects.equals(this.firstName, contactCreateRequest.firstName) &&
Objects.equals(this.homePhone, contactCreateRequest.homePhone) &&
Objects.equals(this.lastName, contactCreateRequest.lastName) &&
Objects.equals(this.mobilePhone, contactCreateRequest.mobilePhone) &&
Objects.equals(this.nickname, contactCreateRequest.nickname) &&
Objects.equals(this.otherPhone, contactCreateRequest.otherPhone) &&
Objects.equals(this.email, contactCreateRequest.email) &&
Objects.equals(this.taxRegion, contactCreateRequest.taxRegion) &&
Objects.equals(this.workEmail, contactCreateRequest.workEmail) &&
Objects.equals(this.workPhone, contactCreateRequest.workPhone) &&
Objects.equals(this.otherPhoneType, contactCreateRequest.otherPhoneType) &&
Objects.equals(this.fax, contactCreateRequest.fax) &&
Objects.equals(this.accountId, contactCreateRequest.accountId) &&
Objects.equals(this.accountNumber, contactCreateRequest.accountNumber);
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, address, firstName, homePhone, lastName, mobilePhone, nickname, otherPhone, email, taxRegion, workEmail, workPhone, otherPhoneType, fax, accountId, accountNumber);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ContactCreateRequest {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" address: ").append(toIndentedString(address)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" homePhone: ").append(toIndentedString(homePhone)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" mobilePhone: ").append(toIndentedString(mobilePhone)).append("\n");
sb.append(" nickname: ").append(toIndentedString(nickname)).append("\n");
sb.append(" otherPhone: ").append(toIndentedString(otherPhone)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" taxRegion: ").append(toIndentedString(taxRegion)).append("\n");
sb.append(" workEmail: ").append(toIndentedString(workEmail)).append("\n");
sb.append(" workPhone: ").append(toIndentedString(workPhone)).append("\n");
sb.append(" otherPhoneType: ").append(toIndentedString(otherPhoneType)).append("\n");
sb.append(" fax: ").append(toIndentedString(fax)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy