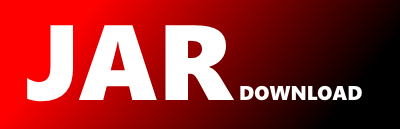
org.openapitools.client.model.CreditMemoItemPreviewResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* CreditMemoItemPreviewResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class CreditMemoItemPreviewResponse {
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_SUBTOTAL = "subtotal";
@SerializedName(SERIALIZED_NAME_SUBTOTAL)
private BigDecimal subtotal;
public static final String SERIALIZED_NAME_APPLIED_TO_ITEM_ID = "applied_to_item_id";
@SerializedName(SERIALIZED_NAME_APPLIED_TO_ITEM_ID)
private String appliedToItemId;
public static final String SERIALIZED_NAME_DOCUMENT_ITEM_DATE = "document_item_date";
@SerializedName(SERIALIZED_NAME_DOCUMENT_ITEM_DATE)
private OffsetDateTime documentItemDate;
public static final String SERIALIZED_NAME_DOCUMENT_ITEM_NUMBER = "document_item_number";
@SerializedName(SERIALIZED_NAME_DOCUMENT_ITEM_NUMBER)
private String documentItemNumber;
public static final String SERIALIZED_NAME_CHARGE_TYPE = "charge_type";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE)
private String chargeType;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
/**
* Gets or Sets processingType
*/
@JsonAdapter(ProcessingTypeEnum.Adapter.class)
public enum ProcessingTypeEnum {
SUBSCRIPTION_ITEM("subscription_item"),
DISCOUNT("discount"),
PREPAYMENT("prepayment"),
TAX("tax"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ProcessingTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ProcessingTypeEnum fromValue(String value) {
for (ProcessingTypeEnum b : ProcessingTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ProcessingTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ProcessingTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ProcessingTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PROCESSING_TYPE = "processing_type";
@SerializedName(SERIALIZED_NAME_PROCESSING_TYPE)
private ProcessingTypeEnum processingType;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_ID = "subscription_item_id";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_ID)
private String subscriptionItemId;
public static final String SERIALIZED_NAME_SERVICE_START_DATE = "service_start_date";
@SerializedName(SERIALIZED_NAME_SERVICE_START_DATE)
private String serviceStartDate;
public static final String SERIALIZED_NAME_SERVICE_END_DATE = "service_end_date";
@SerializedName(SERIALIZED_NAME_SERVICE_END_DATE)
private String serviceEndDate;
public static final String SERIALIZED_NAME_SKU = "sku";
@SerializedName(SERIALIZED_NAME_SKU)
private String sku;
public static final String SERIALIZED_NAME_SKU_NAME = "sku_name";
@SerializedName(SERIALIZED_NAME_SKU_NAME)
private String skuName;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ID = "subscription_id";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ID)
private String subscriptionId;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBER = "subscription_number";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBER)
private String subscriptionNumber;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unit_of_measure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public CreditMemoItemPreviewResponse() {
}
public CreditMemoItemPreviewResponse amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The total amount of this credit memo item.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount of this credit memo item.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public CreditMemoItemPreviewResponse subtotal(BigDecimal subtotal) {
this.subtotal = subtotal;
return this;
}
/**
* The total amount of this credit memo item exclusive of tax.
* @return subtotal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount of this credit memo item exclusive of tax.")
public BigDecimal getSubtotal() {
return subtotal;
}
public void setSubtotal(BigDecimal subtotal) {
this.subtotal = subtotal;
}
public CreditMemoItemPreviewResponse appliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
return this;
}
/**
* Identifier of an invoice item that this credit memo item is applied to.
* @return appliedToItemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of an invoice item that this credit memo item is applied to.")
public String getAppliedToItemId() {
return appliedToItemId;
}
public void setAppliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
}
public CreditMemoItemPreviewResponse documentItemDate(OffsetDateTime documentItemDate) {
this.documentItemDate = documentItemDate;
return this;
}
/**
* The date when the credit memo item takes effect.
* @return documentItemDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2022-01-01T07:08:12-07:00", value = "The date when the credit memo item takes effect.")
public OffsetDateTime getDocumentItemDate() {
return documentItemDate;
}
public void setDocumentItemDate(OffsetDateTime documentItemDate) {
this.documentItemDate = documentItemDate;
}
public CreditMemoItemPreviewResponse documentItemNumber(String documentItemNumber) {
this.documentItemNumber = documentItemNumber;
return this;
}
/**
* Get documentItemNumber
* @return documentItemNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getDocumentItemNumber() {
return documentItemNumber;
}
public void setDocumentItemNumber(String documentItemNumber) {
this.documentItemNumber = documentItemNumber;
}
public CreditMemoItemPreviewResponse chargeType(String chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Type of the charge. It can be one of the following types: one-time, recurring, or usage.
* @return chargeType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Type of the charge. It can be one of the following types: one-time, recurring, or usage.")
public String getChargeType() {
return chargeType;
}
public void setChargeType(String chargeType) {
this.chargeType = chargeType;
}
public CreditMemoItemPreviewResponse description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string associated with the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string associated with the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public CreditMemoItemPreviewResponse id(String id) {
this.id = id;
return this;
}
/**
* Unique identifier of the object
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the object")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public CreditMemoItemPreviewResponse processingType(ProcessingTypeEnum processingType) {
this.processingType = processingType;
return this;
}
/**
* Get processingType
* @return processingType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ProcessingTypeEnum getProcessingType() {
return processingType;
}
public void setProcessingType(ProcessingTypeEnum processingType) {
this.processingType = processingType;
}
public CreditMemoItemPreviewResponse quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The number of units of this item.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of units of this item.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public CreditMemoItemPreviewResponse subscriptionItemId(String subscriptionItemId) {
this.subscriptionItemId = subscriptionItemId;
return this;
}
/**
* The identifier the subscription item associated with this credit memo item.
* @return subscriptionItemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier the subscription item associated with this credit memo item.")
public String getSubscriptionItemId() {
return subscriptionItemId;
}
public void setSubscriptionItemId(String subscriptionItemId) {
this.subscriptionItemId = subscriptionItemId;
}
public CreditMemoItemPreviewResponse serviceStartDate(String serviceStartDate) {
this.serviceStartDate = serviceStartDate;
return this;
}
/**
* The start date of the service period associated with this credit memo item. If the associated charge is a one-time fee, then this date is the date of that charge.
* @return serviceStartDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The start date of the service period associated with this credit memo item. If the associated charge is a one-time fee, then this date is the date of that charge.")
public String getServiceStartDate() {
return serviceStartDate;
}
public void setServiceStartDate(String serviceStartDate) {
this.serviceStartDate = serviceStartDate;
}
public CreditMemoItemPreviewResponse serviceEndDate(String serviceEndDate) {
this.serviceEndDate = serviceEndDate;
return this;
}
/**
* The end date of the service period associated with this credit memo item. If the associated charge is a one-time fee, then this date is the date of that charge.
* @return serviceEndDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The end date of the service period associated with this credit memo item. If the associated charge is a one-time fee, then this date is the date of that charge.")
public String getServiceEndDate() {
return serviceEndDate;
}
public void setServiceEndDate(String serviceEndDate) {
this.serviceEndDate = serviceEndDate;
}
public CreditMemoItemPreviewResponse sku(String sku) {
this.sku = sku;
return this;
}
/**
* The unique SKU (stock keeping unit) of the product associated with this item.
* @return sku
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unique SKU (stock keeping unit) of the product associated with this item.")
public String getSku() {
return sku;
}
public void setSku(String sku) {
this.sku = sku;
}
public CreditMemoItemPreviewResponse skuName(String skuName) {
this.skuName = skuName;
return this;
}
/**
* The name of the SKU associated with this item
* @return skuName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the SKU associated with this item")
public String getSkuName() {
return skuName;
}
public void setSkuName(String skuName) {
this.skuName = skuName;
}
public CreditMemoItemPreviewResponse subscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* The identifier of the subscription associated with the credit memo item.
* @return subscriptionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of the subscription associated with the credit memo item.")
public String getSubscriptionId() {
return subscriptionId;
}
public void setSubscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
}
public CreditMemoItemPreviewResponse subscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
return this;
}
/**
* Human-readable identifier of the subscription. It can be user-supplied.
* @return subscriptionNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the subscription. It can be user-supplied.")
public String getSubscriptionNumber() {
return subscriptionNumber;
}
public void setSubscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
}
public CreditMemoItemPreviewResponse unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* Specifies the units used to measure usage.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the units used to measure usage.")
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreditMemoItemPreviewResponse creditMemoItemPreviewResponse = (CreditMemoItemPreviewResponse) o;
return Objects.equals(this.amount, creditMemoItemPreviewResponse.amount) &&
Objects.equals(this.subtotal, creditMemoItemPreviewResponse.subtotal) &&
Objects.equals(this.appliedToItemId, creditMemoItemPreviewResponse.appliedToItemId) &&
Objects.equals(this.documentItemDate, creditMemoItemPreviewResponse.documentItemDate) &&
Objects.equals(this.documentItemNumber, creditMemoItemPreviewResponse.documentItemNumber) &&
Objects.equals(this.chargeType, creditMemoItemPreviewResponse.chargeType) &&
Objects.equals(this.description, creditMemoItemPreviewResponse.description) &&
Objects.equals(this.id, creditMemoItemPreviewResponse.id) &&
Objects.equals(this.processingType, creditMemoItemPreviewResponse.processingType) &&
Objects.equals(this.quantity, creditMemoItemPreviewResponse.quantity) &&
Objects.equals(this.subscriptionItemId, creditMemoItemPreviewResponse.subscriptionItemId) &&
Objects.equals(this.serviceStartDate, creditMemoItemPreviewResponse.serviceStartDate) &&
Objects.equals(this.serviceEndDate, creditMemoItemPreviewResponse.serviceEndDate) &&
Objects.equals(this.sku, creditMemoItemPreviewResponse.sku) &&
Objects.equals(this.skuName, creditMemoItemPreviewResponse.skuName) &&
Objects.equals(this.subscriptionId, creditMemoItemPreviewResponse.subscriptionId) &&
Objects.equals(this.subscriptionNumber, creditMemoItemPreviewResponse.subscriptionNumber) &&
Objects.equals(this.unitOfMeasure, creditMemoItemPreviewResponse.unitOfMeasure);
}
@Override
public int hashCode() {
return Objects.hash(amount, subtotal, appliedToItemId, documentItemDate, documentItemNumber, chargeType, description, id, processingType, quantity, subscriptionItemId, serviceStartDate, serviceEndDate, sku, skuName, subscriptionId, subscriptionNumber, unitOfMeasure);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreditMemoItemPreviewResponse {\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" subtotal: ").append(toIndentedString(subtotal)).append("\n");
sb.append(" appliedToItemId: ").append(toIndentedString(appliedToItemId)).append("\n");
sb.append(" documentItemDate: ").append(toIndentedString(documentItemDate)).append("\n");
sb.append(" documentItemNumber: ").append(toIndentedString(documentItemNumber)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" processingType: ").append(toIndentedString(processingType)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" subscriptionItemId: ").append(toIndentedString(subscriptionItemId)).append("\n");
sb.append(" serviceStartDate: ").append(toIndentedString(serviceStartDate)).append("\n");
sb.append(" serviceEndDate: ").append(toIndentedString(serviceEndDate)).append("\n");
sb.append(" sku: ").append(toIndentedString(sku)).append("\n");
sb.append(" skuName: ").append(toIndentedString(skuName)).append("\n");
sb.append(" subscriptionId: ").append(toIndentedString(subscriptionId)).append("\n");
sb.append(" subscriptionNumber: ").append(toIndentedString(subscriptionNumber)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy