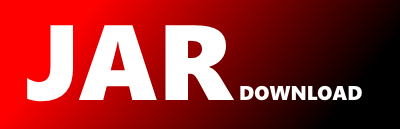
org.openapitools.client.model.DebitMemo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.Account;
import org.openapitools.client.model.BillingDocumentItemListResponse;
import org.openapitools.client.model.Contact;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.FlexibleBillingDocumentSettings;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* DebitMemo
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class DebitMemo {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DUE_DATE = "due_date";
@SerializedName(SERIALIZED_NAME_DUE_DATE)
private LocalDate dueDate;
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "document_date";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
public static final String SERIALIZED_NAME_REASON_CODE = "reason_code";
@SerializedName(SERIALIZED_NAME_REASON_CODE)
private String reasonCode;
public static final String SERIALIZED_NAME_INVOICE_ID = "invoice_id";
@SerializedName(SERIALIZED_NAME_INVOICE_ID)
private String invoiceId;
public static final String SERIALIZED_NAME_TRANSFER_TO_ACCOUNTING = "transfer_to_accounting";
@SerializedName(SERIALIZED_NAME_TRANSFER_TO_ACCOUNTING)
private Boolean transferToAccounting;
public static final String SERIALIZED_NAME_EXCLUDE_FROM_AUTO_APPLY_RULES = "exclude_from_auto_apply_rules";
@SerializedName(SERIALIZED_NAME_EXCLUDE_FROM_AUTO_APPLY_RULES)
private Boolean excludeFromAutoApplyRules;
public static final String SERIALIZED_NAME_PAY = "pay";
@SerializedName(SERIALIZED_NAME_PAY)
private Boolean pay;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DEBIT_MEMO_NUMBER = "debit_memo_number";
@SerializedName(SERIALIZED_NAME_DEBIT_MEMO_NUMBER)
private String debitMemoNumber;
public static final String SERIALIZED_NAME_AMOUNT_REFUNDED = "amount_refunded";
@SerializedName(SERIALIZED_NAME_AMOUNT_REFUNDED)
private BigDecimal amountRefunded;
public static final String SERIALIZED_NAME_STATE_TRANSITIONS = "state_transitions";
@SerializedName(SERIALIZED_NAME_STATE_TRANSITIONS)
private Object stateTransitions;
public static final String SERIALIZED_NAME_POSTED_BY_ID = "posted_by_id";
@SerializedName(SERIALIZED_NAME_POSTED_BY_ID)
private String postedById;
/**
* The status of the debit memo.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
DRAFT("draft"),
POSTED("posted"),
OPEN("open"),
CANCELED("canceled"),
FAILED("failed"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private Account account;
public static final String SERIALIZED_NAME_ITEMS = "items";
@SerializedName(SERIALIZED_NAME_ITEMS)
private BillingDocumentItemListResponse items;
public static final String SERIALIZED_NAME_TOTAL = "total";
@SerializedName(SERIALIZED_NAME_TOTAL)
private BigDecimal total;
public static final String SERIALIZED_NAME_SUBTOTAL = "subtotal";
@SerializedName(SERIALIZED_NAME_SUBTOTAL)
private BigDecimal subtotal;
public static final String SERIALIZED_NAME_TAX = "tax";
@SerializedName(SERIALIZED_NAME_TAX)
private BigDecimal tax;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_REMAINING_BALANCE = "remaining_balance";
@SerializedName(SERIALIZED_NAME_REMAINING_BALANCE)
private BigDecimal remainingBalance;
public static final String SERIALIZED_NAME_AMOUNT_PAID = "amount_paid";
@SerializedName(SERIALIZED_NAME_AMOUNT_PAID)
private BigDecimal amountPaid;
public static final String SERIALIZED_NAME_PAID = "paid";
@SerializedName(SERIALIZED_NAME_PAID)
private Boolean paid;
public static final String SERIALIZED_NAME_PAST_DUE = "past_due";
@SerializedName(SERIALIZED_NAME_PAST_DUE)
private Boolean pastDue;
public static final String SERIALIZED_NAME_BILL_TO_ID = "bill_to_id";
@SerializedName(SERIALIZED_NAME_BILL_TO_ID)
@JsonAdapter(NullableFieldAdapter.class)
private String billToId;
public static final String SERIALIZED_NAME_PAYMENT_TERMS = "payment_terms";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERMS)
@JsonAdapter(NullableFieldAdapter.class)
private String paymentTerms;
public static final String SERIALIZED_NAME_BILL_TO = "bill_to";
@SerializedName(SERIALIZED_NAME_BILL_TO)
private Contact billTo;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS = "billing_document_settings";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS)
private FlexibleBillingDocumentSettings billingDocumentSettings;
public DebitMemo() {
}
public DebitMemo(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects,
String debitMemoNumber,
String postedById,
BillingDocumentItemListResponse items,
BigDecimal total,
BigDecimal subtotal,
BigDecimal tax,
BigDecimal balance,
BigDecimal remainingBalance,
BigDecimal amountPaid,
Boolean paid,
Boolean pastDue,
Contact billTo
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
this.debitMemoNumber = debitMemoNumber;
this.postedById = postedById;
this.items = items;
this.total = total;
this.subtotal = subtotal;
this.tax = tax;
this.balance = balance;
this.remainingBalance = remainingBalance;
this.amountPaid = amountPaid;
this.paid = paid;
this.pastDue = pastDue;
this.billTo = billTo;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public DebitMemo customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public DebitMemo putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public DebitMemo accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the account that owns the debit memo.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the debit memo.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public DebitMemo accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Human-readable identifier of the account that owns the debit memo.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the account that owns the debit memo.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public DebitMemo description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string associated with the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string associated with the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public DebitMemo dueDate(LocalDate dueDate) {
this.dueDate = dueDate;
return this;
}
/**
* The date on which payment for the billing document is due.
* @return dueDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The date on which payment for the billing document is due.")
public LocalDate getDueDate() {
return dueDate;
}
public void setDueDate(LocalDate dueDate) {
this.dueDate = dueDate;
}
public DebitMemo documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* The date when the debit memo takes effect.
* @return documentDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The date when the debit memo takes effect.")
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public DebitMemo reasonCode(String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* Reason for issuing this debit memo. This field is applicable only if the `type` field is set to `credit_memo` or `debit_memo`.
* @return reasonCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Reason for issuing this debit memo. This field is applicable only if the `type` field is set to `credit_memo` or `debit_memo`.")
public String getReasonCode() {
return reasonCode;
}
public void setReasonCode(String reasonCode) {
this.reasonCode = reasonCode;
}
public DebitMemo invoiceId(String invoiceId) {
this.invoiceId = invoiceId;
return this;
}
/**
* The identifier of the invoice from which this credit memo or debit memo is created. This field is applicable only if the `type` field is set to `credit_memo` or `debit_memo`.
* @return invoiceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of the invoice from which this credit memo or debit memo is created. This field is applicable only if the `type` field is set to `credit_memo` or `debit_memo`.")
public String getInvoiceId() {
return invoiceId;
}
public void setInvoiceId(String invoiceId) {
this.invoiceId = invoiceId;
}
public DebitMemo transferToAccounting(Boolean transferToAccounting) {
this.transferToAccounting = transferToAccounting;
return this;
}
/**
* Whether to transfer to an external accounting system.
* @return transferToAccounting
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether to transfer to an external accounting system.")
public Boolean getTransferToAccounting() {
return transferToAccounting;
}
public void setTransferToAccounting(Boolean transferToAccounting) {
this.transferToAccounting = transferToAccounting;
}
public DebitMemo excludeFromAutoApplyRules(Boolean excludeFromAutoApplyRules) {
this.excludeFromAutoApplyRules = excludeFromAutoApplyRules;
return this;
}
/**
* Indicates whether to exclude this credit memo from the rule of automatically applying it to invoices. This field is applicable only if the `type` field is set to `credit_memo`.
* @return excludeFromAutoApplyRules
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to exclude this credit memo from the rule of automatically applying it to invoices. This field is applicable only if the `type` field is set to `credit_memo`.")
public Boolean getExcludeFromAutoApplyRules() {
return excludeFromAutoApplyRules;
}
public void setExcludeFromAutoApplyRules(Boolean excludeFromAutoApplyRules) {
this.excludeFromAutoApplyRules = excludeFromAutoApplyRules;
}
public DebitMemo pay(Boolean pay) {
this.pay = pay;
return this;
}
/**
* Indicates whether this billing document is automatically picked up for processing in the corresponding payment run.
* @return pay
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether this billing document is automatically picked up for processing in the corresponding payment run.")
public Boolean getPay() {
return pay;
}
public void setPay(Boolean pay) {
this.pay = pay;
}
public DebitMemo currency(String currency) {
this.currency = currency;
return this;
}
/**
* 3-letter ISO 4217 currency code
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "3-letter ISO 4217 currency code")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
/**
* A human-readable identifier for the billing document; may be user-supplied.
* @return debitMemoNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A human-readable identifier for the billing document; may be user-supplied.")
public String getDebitMemoNumber() {
return debitMemoNumber;
}
public DebitMemo amountRefunded(BigDecimal amountRefunded) {
this.amountRefunded = amountRefunded;
return this;
}
/**
* The amount of this billing document item refunded.
* @return amountRefunded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of this billing document item refunded.")
public BigDecimal getAmountRefunded() {
return amountRefunded;
}
public void setAmountRefunded(BigDecimal amountRefunded) {
this.amountRefunded = amountRefunded;
}
public DebitMemo stateTransitions(Object stateTransitions) {
this.stateTransitions = stateTransitions;
return this;
}
/**
* Get stateTransitions
* @return stateTransitions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Object getStateTransitions() {
return stateTransitions;
}
public void setStateTransitions(Object stateTransitions) {
this.stateTransitions = stateTransitions;
}
/**
* Identifier of the Zuora user who posted the debit memo.
* @return postedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the Zuora user who posted the debit memo.")
public String getPostedById() {
return postedById;
}
public DebitMemo state(StateEnum state) {
this.state = state;
return this;
}
/**
* The status of the debit memo.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The status of the debit memo.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public DebitMemo account(Account account) {
this.account = account;
return this;
}
/**
* The account that owns the billing document. EXPANDABLE
* @return account
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The account that owns the billing document. EXPANDABLE")
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
/**
* List of debit memo items.
* @return items
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of debit memo items.")
public BillingDocumentItemListResponse getItems() {
return items;
}
/**
* The total amount.
* @return total
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount.")
public BigDecimal getTotal() {
return total;
}
/**
* The total amount exclusive of tax.
* @return subtotal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount exclusive of tax.")
public BigDecimal getSubtotal() {
return subtotal;
}
/**
* The total tax amount.
* @return tax
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total tax amount.")
public BigDecimal getTax() {
return tax;
}
/**
* The total balance remaining. This field is deprecated. Use `remaining_balance` field.
* @return balance
* @deprecated
**/
@Deprecated
@javax.annotation.Nullable
@ApiModelProperty(value = "The total balance remaining. This field is deprecated. Use `remaining_balance` field.")
public BigDecimal getBalance() {
return balance;
}
/**
* The total balance remaining.
* @return remainingBalance
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total balance remaining.")
public BigDecimal getRemainingBalance() {
return remainingBalance;
}
/**
* The total amount paid.
* @return amountPaid
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount paid.")
public BigDecimal getAmountPaid() {
return amountPaid;
}
/**
* Whether payment was successfully collected for this invoice. An invoice can be paid with a payment or a credit memo.
* @return paid
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether payment was successfully collected for this invoice. An invoice can be paid with a payment or a credit memo.")
public Boolean getPaid() {
return paid;
}
/**
* Whether payment is past due.
* @return pastDue
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether payment is past due.")
public Boolean getPastDue() {
return pastDue;
}
public DebitMemo billToId(String billToId) {
this.billToId = billToId;
return this;
}
/**
* ID of the bill-to contact.
* @return billToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "ID of the bill-to contact.")
public String getBillToId() {
return billToId;
}
public void setBillToId(String billToId) {
this.billToId = billToId;
}
public DebitMemo paymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
return this;
}
/**
* The name of payment term associated with the invoice.
* @return paymentTerms
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of payment term associated with the invoice.")
public String getPaymentTerms() {
return paymentTerms;
}
public void setPaymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
}
/**
* The billing address for the customer.
* @return billTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing address for the customer.")
public Contact getBillTo() {
return billTo;
}
public DebitMemo billingDocumentSettings(FlexibleBillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
return this;
}
/**
* The billing document settings for the customer.
* @return billingDocumentSettings
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing document settings for the customer.")
public FlexibleBillingDocumentSettings getBillingDocumentSettings() {
return billingDocumentSettings;
}
public void setBillingDocumentSettings(FlexibleBillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DebitMemo debitMemo = (DebitMemo) o;
return Objects.equals(this.id, debitMemo.id) &&
Objects.equals(this.updatedById, debitMemo.updatedById) &&
Objects.equals(this.updatedTime, debitMemo.updatedTime) &&
Objects.equals(this.createdById, debitMemo.createdById) &&
Objects.equals(this.createdTime, debitMemo.createdTime) &&
Objects.equals(this.customFields, debitMemo.customFields) &&
Objects.equals(this.customObjects, debitMemo.customObjects) &&
Objects.equals(this.accountId, debitMemo.accountId) &&
Objects.equals(this.accountNumber, debitMemo.accountNumber) &&
Objects.equals(this.description, debitMemo.description) &&
Objects.equals(this.dueDate, debitMemo.dueDate) &&
Objects.equals(this.documentDate, debitMemo.documentDate) &&
Objects.equals(this.reasonCode, debitMemo.reasonCode) &&
Objects.equals(this.invoiceId, debitMemo.invoiceId) &&
Objects.equals(this.transferToAccounting, debitMemo.transferToAccounting) &&
Objects.equals(this.excludeFromAutoApplyRules, debitMemo.excludeFromAutoApplyRules) &&
Objects.equals(this.pay, debitMemo.pay) &&
Objects.equals(this.currency, debitMemo.currency) &&
Objects.equals(this.debitMemoNumber, debitMemo.debitMemoNumber) &&
Objects.equals(this.amountRefunded, debitMemo.amountRefunded) &&
Objects.equals(this.stateTransitions, debitMemo.stateTransitions) &&
Objects.equals(this.postedById, debitMemo.postedById) &&
Objects.equals(this.state, debitMemo.state) &&
Objects.equals(this.account, debitMemo.account) &&
Objects.equals(this.items, debitMemo.items) &&
Objects.equals(this.total, debitMemo.total) &&
Objects.equals(this.subtotal, debitMemo.subtotal) &&
Objects.equals(this.tax, debitMemo.tax) &&
Objects.equals(this.balance, debitMemo.balance) &&
Objects.equals(this.remainingBalance, debitMemo.remainingBalance) &&
Objects.equals(this.amountPaid, debitMemo.amountPaid) &&
Objects.equals(this.paid, debitMemo.paid) &&
Objects.equals(this.pastDue, debitMemo.pastDue) &&
Objects.equals(this.billToId, debitMemo.billToId) &&
Objects.equals(this.paymentTerms, debitMemo.paymentTerms) &&
Objects.equals(this.billTo, debitMemo.billTo) &&
Objects.equals(this.billingDocumentSettings, debitMemo.billingDocumentSettings);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, accountId, accountNumber, description, dueDate, documentDate, reasonCode, invoiceId, transferToAccounting, excludeFromAutoApplyRules, pay, currency, debitMemoNumber, amountRefunded, stateTransitions, postedById, state, account, items, total, subtotal, tax, balance, remainingBalance, amountPaid, paid, pastDue, billToId, paymentTerms, billTo, billingDocumentSettings);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DebitMemo {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" dueDate: ").append(toIndentedString(dueDate)).append("\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" invoiceId: ").append(toIndentedString(invoiceId)).append("\n");
sb.append(" transferToAccounting: ").append(toIndentedString(transferToAccounting)).append("\n");
sb.append(" excludeFromAutoApplyRules: ").append(toIndentedString(excludeFromAutoApplyRules)).append("\n");
sb.append(" pay: ").append(toIndentedString(pay)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" debitMemoNumber: ").append(toIndentedString(debitMemoNumber)).append("\n");
sb.append(" amountRefunded: ").append(toIndentedString(amountRefunded)).append("\n");
sb.append(" stateTransitions: ").append(toIndentedString(stateTransitions)).append("\n");
sb.append(" postedById: ").append(toIndentedString(postedById)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" items: ").append(toIndentedString(items)).append("\n");
sb.append(" total: ").append(toIndentedString(total)).append("\n");
sb.append(" subtotal: ").append(toIndentedString(subtotal)).append("\n");
sb.append(" tax: ").append(toIndentedString(tax)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" remainingBalance: ").append(toIndentedString(remainingBalance)).append("\n");
sb.append(" amountPaid: ").append(toIndentedString(amountPaid)).append("\n");
sb.append(" paid: ").append(toIndentedString(paid)).append("\n");
sb.append(" pastDue: ").append(toIndentedString(pastDue)).append("\n");
sb.append(" billToId: ").append(toIndentedString(billToId)).append("\n");
sb.append(" paymentTerms: ").append(toIndentedString(paymentTerms)).append("\n");
sb.append(" billTo: ").append(toIndentedString(billTo)).append("\n");
sb.append(" billingDocumentSettings: ").append(toIndentedString(billingDocumentSettings)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy