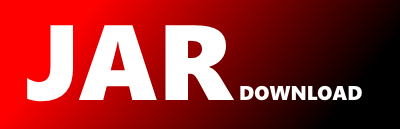
org.openapitools.client.model.Drawdown Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Drawdown
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Drawdown {
public static final String SERIALIZED_NAME_CONVERSION_RATE = "conversion_rate";
@SerializedName(SERIALIZED_NAME_CONVERSION_RATE)
private BigDecimal conversionRate;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unit_of_measure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public Drawdown() {
}
public Drawdown conversionRate(BigDecimal conversionRate) {
this.conversionRate = conversionRate;
return this;
}
/**
* The conversion rate between usage unit of measure (UOM) and drawdown unit of measure for a drawdown charge. **Note**: <ul> <li>Must be a positive number (>0).</li> <li>Must be `1` when usage UOM and drawdown UOM are the same.</li> <li>If both `conversion_rate` and `unit_of_measure` for the drawdown are empty, the system will set default values respectively: <ul> <li> `conversion_rate`: 1 </li> <li> `unit_of_measure`: Same as the usage UOM of this drawdown charge. </li></ul></li></ul> The `conversion_rate` and `unit_of_measure` fields need to have values or be empty at the same time.
* @return conversionRate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The conversion rate between usage unit of measure (UOM) and drawdown unit of measure for a drawdown charge. **Note**: - Must be a positive number (>0).
- Must be `1` when usage UOM and drawdown UOM are the same.
- If both `conversion_rate` and `unit_of_measure` for the drawdown are empty, the system will set default values respectively:
- `conversion_rate`: 1
- `unit_of_measure`: Same as the usage UOM of this drawdown charge.
The `conversion_rate` and `unit_of_measure` fields need to have values or be empty at the same time. ")
public BigDecimal getConversionRate() {
return conversionRate;
}
public void setConversionRate(BigDecimal conversionRate) {
this.conversionRate = conversionRate;
}
public Drawdown unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* Unit of measurement for a drawdown charge.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unit of measurement for a drawdown charge.")
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Drawdown drawdown = (Drawdown) o;
return Objects.equals(this.conversionRate, drawdown.conversionRate) &&
Objects.equals(this.unitOfMeasure, drawdown.unitOfMeasure);
}
@Override
public int hashCode() {
return Objects.hash(conversionRate, unitOfMeasure);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Drawdown {\n");
sb.append(" conversionRate: ").append(toIndentedString(conversionRate)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy