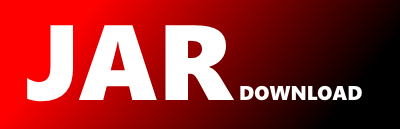
org.openapitools.client.model.Fulfillment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.Revenue;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Fulfillment
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Fulfillment {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_ORDER_LINE_ITEM_ID = "order_line_item_id";
@SerializedName(SERIALIZED_NAME_ORDER_LINE_ITEM_ID)
private String orderLineItemId;
public static final String SERIALIZED_NAME_FULFILLMENT_NUMBER = "fulfillment_number";
@SerializedName(SERIALIZED_NAME_FULFILLMENT_NUMBER)
private String fulfillmentNumber;
public static final String SERIALIZED_NAME_FULFILLMENT_DATE = "fulfillment_date";
@SerializedName(SERIALIZED_NAME_FULFILLMENT_DATE)
private LocalDate fulfillmentDate;
/**
* The type of fulfillment.
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
DELIVERY("delivery"),
RETURN("return"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TypeEnum type;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
/**
* The status of the invoice.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
ACCEPTED("accepted"),
BOOKED("booked"),
SENT_TO_BILLING("sent_to_billing"),
COMPLETE("complete"),
CANCELED("canceled"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_TARGET_DATE = "target_date";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_TRACKING_NUMBER = "tracking_number";
@SerializedName(SERIALIZED_NAME_TRACKING_NUMBER)
private String trackingNumber;
public static final String SERIALIZED_NAME_CARRIER = "carrier";
@SerializedName(SERIALIZED_NAME_CARRIER)
private String carrier;
public static final String SERIALIZED_NAME_FULFILLMENT_SYSTEM = "fulfillment_system";
@SerializedName(SERIALIZED_NAME_FULFILLMENT_SYSTEM)
private String fulfillmentSystem;
public static final String SERIALIZED_NAME_EXTERNAL_ID = "external_id";
@SerializedName(SERIALIZED_NAME_EXTERNAL_ID)
private String externalId;
public static final String SERIALIZED_NAME_REVENUE = "revenue";
@SerializedName(SERIALIZED_NAME_REVENUE)
private Revenue revenue;
public static final String SERIALIZED_NAME_LOCATION = "location";
@SerializedName(SERIALIZED_NAME_LOCATION)
private Map location = null;
public Fulfillment() {
}
public Fulfillment(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public Fulfillment customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public Fulfillment putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public Fulfillment orderLineItemId(String orderLineItemId) {
this.orderLineItemId = orderLineItemId;
return this;
}
/**
* The unique identifier of the associated order line item.
* @return orderLineItemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unique identifier of the associated order line item.")
public String getOrderLineItemId() {
return orderLineItemId;
}
public void setOrderLineItemId(String orderLineItemId) {
this.orderLineItemId = orderLineItemId;
}
public Fulfillment fulfillmentNumber(String fulfillmentNumber) {
this.fulfillmentNumber = fulfillmentNumber;
return this;
}
/**
* Human-readable identifier for the object. It can be user-supplied.
* @return fulfillmentNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier for the object. It can be user-supplied.")
public String getFulfillmentNumber() {
return fulfillmentNumber;
}
public void setFulfillmentNumber(String fulfillmentNumber) {
this.fulfillmentNumber = fulfillmentNumber;
}
public Fulfillment fulfillmentDate(LocalDate fulfillmentDate) {
this.fulfillmentDate = fulfillmentDate;
return this;
}
/**
* The date of the fulfillment.
* @return fulfillmentDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The date of the fulfillment.")
public LocalDate getFulfillmentDate() {
return fulfillmentDate;
}
public void setFulfillmentDate(LocalDate fulfillmentDate) {
this.fulfillmentDate = fulfillmentDate;
}
public Fulfillment type(TypeEnum type) {
this.type = type;
return this;
}
/**
* The type of fulfillment.
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The type of fulfillment.")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public Fulfillment quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The number of units of this item.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of units of this item.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public Fulfillment state(StateEnum state) {
this.state = state;
return this;
}
/**
* The status of the invoice.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The status of the invoice.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public Fulfillment targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* All order line items associated with this fulfillment that were unbilled on or before this date are included in future bill runs.
* @return targetDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "All order line items associated with this fulfillment that were unbilled on or before this date are included in future bill runs.")
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public Fulfillment description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Fulfillment trackingNumber(String trackingNumber) {
this.trackingNumber = trackingNumber;
return this;
}
/**
* The tracking number of the fulfillment.
* @return trackingNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The tracking number of the fulfillment.")
public String getTrackingNumber() {
return trackingNumber;
}
public void setTrackingNumber(String trackingNumber) {
this.trackingNumber = trackingNumber;
}
public Fulfillment carrier(String carrier) {
this.carrier = carrier;
return this;
}
/**
* The name of the shipping carrier for this fulfillment.
* @return carrier
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the shipping carrier for this fulfillment.")
public String getCarrier() {
return carrier;
}
public void setCarrier(String carrier) {
this.carrier = carrier;
}
public Fulfillment fulfillmentSystem(String fulfillmentSystem) {
this.fulfillmentSystem = fulfillmentSystem;
return this;
}
/**
* The fulfillment system for the fulfillment.
* @return fulfillmentSystem
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The fulfillment system for the fulfillment.")
public String getFulfillmentSystem() {
return fulfillmentSystem;
}
public void setFulfillmentSystem(String fulfillmentSystem) {
this.fulfillmentSystem = fulfillmentSystem;
}
public Fulfillment externalId(String externalId) {
this.externalId = externalId;
return this;
}
/**
* An external identifier for the fulfillment.
* @return externalId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An external identifier for the fulfillment.")
public String getExternalId() {
return externalId;
}
public void setExternalId(String externalId) {
this.externalId = externalId;
}
public Fulfillment revenue(Revenue revenue) {
this.revenue = revenue;
return this;
}
/**
* Get revenue
* @return revenue
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Revenue getRevenue() {
return revenue;
}
public void setRevenue(Revenue revenue) {
this.revenue = revenue;
}
public Fulfillment location(Map location) {
this.location = location;
return this;
}
public Fulfillment putLocationItem(String key, Object locationItem) {
if (this.location == null) {
this.location = new HashMap();
}
this.location.put(key, locationItem);
return this;
}
/**
* The fulfillment location of the fulfillment.
* @return location
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The fulfillment location of the fulfillment.")
public Map getLocation() {
return location;
}
public void setLocation(Map location) {
this.location = location;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Fulfillment fulfillment = (Fulfillment) o;
return Objects.equals(this.id, fulfillment.id) &&
Objects.equals(this.updatedById, fulfillment.updatedById) &&
Objects.equals(this.updatedTime, fulfillment.updatedTime) &&
Objects.equals(this.createdById, fulfillment.createdById) &&
Objects.equals(this.createdTime, fulfillment.createdTime) &&
Objects.equals(this.customFields, fulfillment.customFields) &&
Objects.equals(this.customObjects, fulfillment.customObjects) &&
Objects.equals(this.orderLineItemId, fulfillment.orderLineItemId) &&
Objects.equals(this.fulfillmentNumber, fulfillment.fulfillmentNumber) &&
Objects.equals(this.fulfillmentDate, fulfillment.fulfillmentDate) &&
Objects.equals(this.type, fulfillment.type) &&
Objects.equals(this.quantity, fulfillment.quantity) &&
Objects.equals(this.state, fulfillment.state) &&
Objects.equals(this.targetDate, fulfillment.targetDate) &&
Objects.equals(this.description, fulfillment.description) &&
Objects.equals(this.trackingNumber, fulfillment.trackingNumber) &&
Objects.equals(this.carrier, fulfillment.carrier) &&
Objects.equals(this.fulfillmentSystem, fulfillment.fulfillmentSystem) &&
Objects.equals(this.externalId, fulfillment.externalId) &&
Objects.equals(this.revenue, fulfillment.revenue) &&
Objects.equals(this.location, fulfillment.location);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, orderLineItemId, fulfillmentNumber, fulfillmentDate, type, quantity, state, targetDate, description, trackingNumber, carrier, fulfillmentSystem, externalId, revenue, location);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Fulfillment {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" orderLineItemId: ").append(toIndentedString(orderLineItemId)).append("\n");
sb.append(" fulfillmentNumber: ").append(toIndentedString(fulfillmentNumber)).append("\n");
sb.append(" fulfillmentDate: ").append(toIndentedString(fulfillmentDate)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" trackingNumber: ").append(toIndentedString(trackingNumber)).append("\n");
sb.append(" carrier: ").append(toIndentedString(carrier)).append("\n");
sb.append(" fulfillmentSystem: ").append(toIndentedString(fulfillmentSystem)).append("\n");
sb.append(" externalId: ").append(toIndentedString(externalId)).append("\n");
sb.append(" revenue: ").append(toIndentedString(revenue)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy