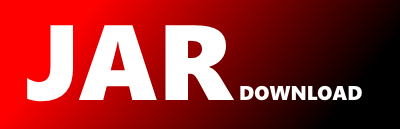
org.openapitools.client.model.FulfillmentItemCreateRequestForFulfillmentPost Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* FulfillmentItemCreateRequestForFulfillmentPost
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class FulfillmentItemCreateRequestForFulfillmentPost {
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_FULFILLMENT_ITEM_NUMBER = "fulfillment_item_number";
@SerializedName(SERIALIZED_NAME_FULFILLMENT_ITEM_NUMBER)
private String fulfillmentItemNumber;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public FulfillmentItemCreateRequestForFulfillmentPost() {
}
public FulfillmentItemCreateRequestForFulfillmentPost description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public FulfillmentItemCreateRequestForFulfillmentPost fulfillmentItemNumber(String fulfillmentItemNumber) {
this.fulfillmentItemNumber = fulfillmentItemNumber;
return this;
}
/**
* Human-readable identifier for the object. It can be user-supplied.
* @return fulfillmentItemNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier for the object. It can be user-supplied.")
public String getFulfillmentItemNumber() {
return fulfillmentItemNumber;
}
public void setFulfillmentItemNumber(String fulfillmentItemNumber) {
this.fulfillmentItemNumber = fulfillmentItemNumber;
}
public FulfillmentItemCreateRequestForFulfillmentPost customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public FulfillmentItemCreateRequestForFulfillmentPost putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
FulfillmentItemCreateRequestForFulfillmentPost fulfillmentItemCreateRequestForFulfillmentPost = (FulfillmentItemCreateRequestForFulfillmentPost) o;
return Objects.equals(this.description, fulfillmentItemCreateRequestForFulfillmentPost.description) &&
Objects.equals(this.fulfillmentItemNumber, fulfillmentItemCreateRequestForFulfillmentPost.fulfillmentItemNumber) &&
Objects.equals(this.customFields, fulfillmentItemCreateRequestForFulfillmentPost.customFields);
}
@Override
public int hashCode() {
return Objects.hash(description, fulfillmentItemNumber, customFields);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class FulfillmentItemCreateRequestForFulfillmentPost {\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" fulfillmentItemNumber: ").append(toIndentedString(fulfillmentItemNumber)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy