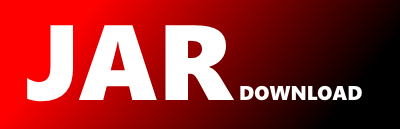
org.openapitools.client.model.GenerateBillingDocumentsAccountRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* GenerateBillingDocumentsAccountRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class GenerateBillingDocumentsAccountRequest {
public static final String SERIALIZED_NAME_POST = "post";
@SerializedName(SERIALIZED_NAME_POST)
private Boolean post;
public static final String SERIALIZED_NAME_RENEW = "renew";
@SerializedName(SERIALIZED_NAME_RENEW)
private Boolean renew;
public static final String SERIALIZED_NAME_CHARGES_EXCLUDED = "charges_excluded";
@SerializedName(SERIALIZED_NAME_CHARGES_EXCLUDED)
private List chargesExcluded = null;
public static final String SERIALIZED_NAME_CREDIT_MEMO_REASON_CODE = "credit_memo_reason_code";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_REASON_CODE)
private String creditMemoReasonCode;
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "document_date";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
public static final String SERIALIZED_NAME_SUBSCRIPTION_IDS = "subscription_ids";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_IDS)
private List subscriptionIds = null;
public static final String SERIALIZED_NAME_TARGET_DATE = "target_date";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public GenerateBillingDocumentsAccountRequest() {
}
public GenerateBillingDocumentsAccountRequest post(Boolean post) {
this.post = post;
return this;
}
/**
* If true, invoices will be automatically posted.
* @return post
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "true", value = "If true, invoices will be automatically posted.")
public Boolean getPost() {
return post;
}
public void setPost(Boolean post) {
this.post = post;
}
public GenerateBillingDocumentsAccountRequest renew(Boolean renew) {
this.renew = renew;
return this;
}
/**
* If true, subscriptions will be automatically renewed.
* @return renew
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "true", value = "If true, subscriptions will be automatically renewed.")
public Boolean getRenew() {
return renew;
}
public void setRenew(Boolean renew) {
this.renew = renew;
}
public GenerateBillingDocumentsAccountRequest chargesExcluded(List chargesExcluded) {
this.chargesExcluded = chargesExcluded;
return this;
}
public GenerateBillingDocumentsAccountRequest addChargesExcludedItem(String chargesExcludedItem) {
if (this.chargesExcluded == null) {
this.chargesExcluded = new ArrayList();
}
this.chargesExcluded.add(chargesExcludedItem);
return this;
}
/**
* Charge type or types to be excluded. Can be `one_time`,`recurring`, or `usage`.
* @return chargesExcluded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Charge type or types to be excluded. Can be `one_time`,`recurring`, or `usage`.")
public List getChargesExcluded() {
return chargesExcluded;
}
public void setChargesExcluded(List chargesExcluded) {
this.chargesExcluded = chargesExcluded;
}
public GenerateBillingDocumentsAccountRequest creditMemoReasonCode(String creditMemoReasonCode) {
this.creditMemoReasonCode = creditMemoReasonCode;
return this;
}
/**
* A code identifying the reason for the credit memo transaction that is generated by the request. The value must be an existing reason code. If you do not pass the field or pass the field with empty value, Zuora uses the default reason code.
* @return creditMemoReasonCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A code identifying the reason for the credit memo transaction that is generated by the request. The value must be an existing reason code. If you do not pass the field or pass the field with empty value, Zuora uses the default reason code.")
public String getCreditMemoReasonCode() {
return creditMemoReasonCode;
}
public void setCreditMemoReasonCode(String creditMemoReasonCode) {
this.creditMemoReasonCode = creditMemoReasonCode;
}
public GenerateBillingDocumentsAccountRequest documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* The date when the credit memo is applied
* @return documentDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The date when the credit memo is applied")
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public GenerateBillingDocumentsAccountRequest subscriptionIds(List subscriptionIds) {
this.subscriptionIds = subscriptionIds;
return this;
}
public GenerateBillingDocumentsAccountRequest addSubscriptionIdsItem(String subscriptionIdsItem) {
if (this.subscriptionIds == null) {
this.subscriptionIds = new ArrayList();
}
this.subscriptionIds.add(subscriptionIdsItem);
return this;
}
/**
* The IDs of the subscriptions that you want to create the billing documents for. Each value must be the unique identifier of the latest version of an active subscription.
* @return subscriptionIds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The IDs of the subscriptions that you want to create the billing documents for. Each value must be the unique identifier of the latest version of an active subscription.")
public List getSubscriptionIds() {
return subscriptionIds;
}
public void setSubscriptionIds(List subscriptionIds) {
this.subscriptionIds = subscriptionIds;
}
public GenerateBillingDocumentsAccountRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* All unbilled items on or before this date are included.
* @return targetDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "All unbilled items on or before this date are included.")
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GenerateBillingDocumentsAccountRequest generateBillingDocumentsAccountRequest = (GenerateBillingDocumentsAccountRequest) o;
return Objects.equals(this.post, generateBillingDocumentsAccountRequest.post) &&
Objects.equals(this.renew, generateBillingDocumentsAccountRequest.renew) &&
Objects.equals(this.chargesExcluded, generateBillingDocumentsAccountRequest.chargesExcluded) &&
Objects.equals(this.creditMemoReasonCode, generateBillingDocumentsAccountRequest.creditMemoReasonCode) &&
Objects.equals(this.documentDate, generateBillingDocumentsAccountRequest.documentDate) &&
Objects.equals(this.subscriptionIds, generateBillingDocumentsAccountRequest.subscriptionIds) &&
Objects.equals(this.targetDate, generateBillingDocumentsAccountRequest.targetDate);
}
@Override
public int hashCode() {
return Objects.hash(post, renew, chargesExcluded, creditMemoReasonCode, documentDate, subscriptionIds, targetDate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GenerateBillingDocumentsAccountRequest {\n");
sb.append(" post: ").append(toIndentedString(post)).append("\n");
sb.append(" renew: ").append(toIndentedString(renew)).append("\n");
sb.append(" chargesExcluded: ").append(toIndentedString(chargesExcluded)).append("\n");
sb.append(" creditMemoReasonCode: ").append(toIndentedString(creditMemoReasonCode)).append("\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" subscriptionIds: ").append(toIndentedString(subscriptionIds)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy