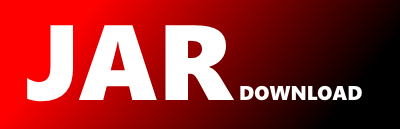
org.openapitools.client.model.GetByIdQueryParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* GetByIdQueryParams
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class GetByIdQueryParams extends HashMap {
public static final String SERIALIZED_NAME_EXPAND = "expand[]";
@SerializedName(SERIALIZED_NAME_EXPAND)
private List expand = null;
public static final String SERIALIZED_NAME_FILTER = "filter[]";
@SerializedName(SERIALIZED_NAME_FILTER)
private List filter = null;
public static final String SERIALIZED_NAME_PAGE_SIZE = "page_size";
@SerializedName(SERIALIZED_NAME_PAGE_SIZE)
private Integer pageSize;
public GetByIdQueryParams() {
}
public GetByIdQueryParams expand(List expand) {
this.expand = expand;
return this;
}
public GetByIdQueryParams addExpandItem(String expandItem) {
if (this.expand == null) {
this.expand = new ArrayList();
}
this.expand.add(expandItem);
return this;
}
/**
* Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions.
* @return expand
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions.")
public List getExpand() {
return expand;
}
public void setExpand(List expand) {
this.expand = expand;
}
public GetByIdQueryParams filter(List filter) {
this.filter = filter;
return this;
}
public GetByIdQueryParams addFilterItem(String filterItem) {
if (this.filter == null) {
this.filter = new ArrayList();
}
this.filter.add(filterItem);
return this;
}
/**
* A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63`
* @return filter
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63`")
public List getFilter() {
return filter;
}
public void setFilter(List filter) {
this.filter = filter;
}
public GetByIdQueryParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error.
* minimum: 1
* maximum: 99
* @return pageSize
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error.")
public Integer getPageSize() {
return pageSize;
}
public void setPageSize(Integer pageSize) {
this.pageSize = pageSize;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetByIdQueryParams getByIdQueryParams = (GetByIdQueryParams) o;
return Objects.equals(this.expand, getByIdQueryParams.expand) &&
Objects.equals(this.filter, getByIdQueryParams.filter) &&
Objects.equals(this.pageSize, getByIdQueryParams.pageSize) &&
super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(expand, filter, pageSize, super.hashCode());
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetByIdQueryParams {\n");
sb.append(" ").append(toIndentedString(super.toString())).append("\n");
sb.append(" expand: ").append(toIndentedString(expand)).append("\n");
sb.append(" filter: ").append(toIndentedString(filter)).append("\n");
sb.append(" pageSize: ").append(toIndentedString(pageSize)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy