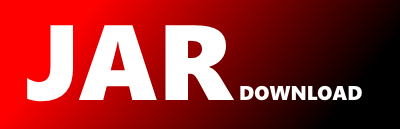
org.openapitools.client.model.Headers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Headers
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Headers {
public static final String SERIALIZED_NAME_ZUORA_TRACK_ID = "zuora-track-id";
@SerializedName(SERIALIZED_NAME_ZUORA_TRACK_ID)
private String zuoraTrackId;
public static final String SERIALIZED_NAME_ASYNC = "async";
@SerializedName(SERIALIZED_NAME_ASYNC)
private Boolean async = false;
public static final String SERIALIZED_NAME_ZUORA_CACHE_ENABLED = "zuora-cache-enabled";
@SerializedName(SERIALIZED_NAME_ZUORA_CACHE_ENABLED)
private Boolean zuoraCacheEnabled = false;
public static final String SERIALIZED_NAME_ZUORA_ENTITY_IDS = "zuora-entity-ids";
@SerializedName(SERIALIZED_NAME_ZUORA_ENTITY_IDS)
private String zuoraEntityIds;
public static final String SERIALIZED_NAME_IDEMPOTENCY_KEY = "idempotency-key";
@SerializedName(SERIALIZED_NAME_IDEMPOTENCY_KEY)
private String idempotencyKey;
public static final String SERIALIZED_NAME_ACCEPT_ENCODING = "accept-encoding";
@SerializedName(SERIALIZED_NAME_ACCEPT_ENCODING)
private String acceptEncoding;
public static final String SERIALIZED_NAME_CONTENT_ENCODING = "content-encoding";
@SerializedName(SERIALIZED_NAME_CONTENT_ENCODING)
private String contentEncoding;
public static final String SERIALIZED_NAME_ZUORA_ORG_IDS = "zuora-org-ids";
@SerializedName(SERIALIZED_NAME_ZUORA_ORG_IDS)
private String zuoraOrgIds;
public Headers() {
}
public Headers zuoraTrackId(String zuoraTrackId) {
this.zuoraTrackId = zuoraTrackId;
return this;
}
/**
* A custom identifier for tracking API requests. If you set a value for this header, Zuora returns the same value in the response header. This header enables you to track your API calls to assist with troubleshooting in the event of an issue. The value of this field must use the US-ASCII character set and must not include any of the following characters: colon (:), semicolon (;), double quote (\"), or quote (').
* @return zuoraTrackId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A custom identifier for tracking API requests. If you set a value for this header, Zuora returns the same value in the response header. This header enables you to track your API calls to assist with troubleshooting in the event of an issue. The value of this field must use the US-ASCII character set and must not include any of the following characters: colon (:), semicolon (;), double quote (\"), or quote (').")
public String getZuoraTrackId() {
return zuoraTrackId;
}
public void setZuoraTrackId(String zuoraTrackId) {
this.zuoraTrackId = zuoraTrackId;
}
public Headers async(Boolean async) {
this.async = async;
return this;
}
/**
* Making asynchronous requests allows you to scale your applications more efficiently by leveraging Zuora's infrastructure to enqueue and execute requests for you without blocking. These requests also use built-in retry semantics, which makes them much less likely to fail for non-deterministic reasons, even in extreme high-throughput scenarios. Meanwhile, when you send a request to one of these endpoints, you can expect to receive a response in less than 150 milliseconds and these calls are unlikely to trigger rate limit errors. If set to true, Zuora returns a 202 Accepted response, and the response body contains only a request ID.
* @return async
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Making asynchronous requests allows you to scale your applications more efficiently by leveraging Zuora's infrastructure to enqueue and execute requests for you without blocking. These requests also use built-in retry semantics, which makes them much less likely to fail for non-deterministic reasons, even in extreme high-throughput scenarios. Meanwhile, when you send a request to one of these endpoints, you can expect to receive a response in less than 150 milliseconds and these calls are unlikely to trigger rate limit errors. If set to true, Zuora returns a 202 Accepted response, and the response body contains only a request ID.")
public Boolean getAsync() {
return async;
}
public void setAsync(Boolean async) {
this.async = async;
}
public Headers zuoraCacheEnabled(Boolean zuoraCacheEnabled) {
this.zuoraCacheEnabled = zuoraCacheEnabled;
return this;
}
/**
* It indicates whether the Zuora cache is enabled to store the request and response for the purpose of performance improvement.
* @return zuoraCacheEnabled
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "It indicates whether the Zuora cache is enabled to store the request and response for the purpose of performance improvement.")
public Boolean getZuoraCacheEnabled() {
return zuoraCacheEnabled;
}
public void setZuoraCacheEnabled(Boolean zuoraCacheEnabled) {
this.zuoraCacheEnabled = zuoraCacheEnabled;
}
public Headers zuoraEntityIds(String zuoraEntityIds) {
this.zuoraEntityIds = zuoraEntityIds;
return this;
}
/**
* An entity ID. If you have multi-entity enabled and the authorization token is valid for more than one entity, you must use this header to specify which entity to perform the operation on. If the authorization token is only valid for a single entity, or you do not have multi-entity enabled, you do not need to set this header.
* @return zuoraEntityIds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An entity ID. If you have multi-entity enabled and the authorization token is valid for more than one entity, you must use this header to specify which entity to perform the operation on. If the authorization token is only valid for a single entity, or you do not have multi-entity enabled, you do not need to set this header.")
public String getZuoraEntityIds() {
return zuoraEntityIds;
}
public void setZuoraEntityIds(String zuoraEntityIds) {
this.zuoraEntityIds = zuoraEntityIds;
}
public Headers idempotencyKey(String idempotencyKey) {
this.idempotencyKey = idempotencyKey;
return this;
}
/**
* Specify a unique idempotency key if you want to perform an idempotent POST or PATCH request. Do not use this header in other request types. This idempotency key should be a unique value, and the Zuora server identifies subsequent retries of the same request using this value. For more information, see [Idempotent Requests](https://developer.zuora.com/api-references/quickstart-api/tag/Idempotent-Requests/).
* @return idempotencyKey
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify a unique idempotency key if you want to perform an idempotent POST or PATCH request. Do not use this header in other request types. This idempotency key should be a unique value, and the Zuora server identifies subsequent retries of the same request using this value. For more information, see [Idempotent Requests](https://developer.zuora.com/api-references/quickstart-api/tag/Idempotent-Requests/).")
public String getIdempotencyKey() {
return idempotencyKey;
}
public void setIdempotencyKey(String idempotencyKey) {
this.idempotencyKey = idempotencyKey;
}
public Headers acceptEncoding(String acceptEncoding) {
this.acceptEncoding = acceptEncoding;
return this;
}
/**
* Include a `accept-encoding: gzip` header to compress responses, which can reduce the bandwidth required for a response. If specified, Zuora automatically compresses responses that contain over 1000 bytes. For more information about this header, see [Request and Response Compression](https://developer.zuora.com/api-references/quickstart-api/tag/Request-and-Response-Compression/).
* @return acceptEncoding
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Include a `accept-encoding: gzip` header to compress responses, which can reduce the bandwidth required for a response. If specified, Zuora automatically compresses responses that contain over 1000 bytes. For more information about this header, see [Request and Response Compression](https://developer.zuora.com/api-references/quickstart-api/tag/Request-and-Response-Compression/).")
public String getAcceptEncoding() {
return acceptEncoding;
}
public void setAcceptEncoding(String acceptEncoding) {
this.acceptEncoding = acceptEncoding;
}
public Headers contentEncoding(String contentEncoding) {
this.contentEncoding = contentEncoding;
return this;
}
/**
* Include a `content-encoding: gzip` header to compress a request. Upload a gzipped file for the payload if you specify this header. For more information, see [Request and Response Compression](https://developer.zuora.com/api-references/quickstart-api/tag/Request-and-Response-Compression/).
* @return contentEncoding
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Include a `content-encoding: gzip` header to compress a request. Upload a gzipped file for the payload if you specify this header. For more information, see [Request and Response Compression](https://developer.zuora.com/api-references/quickstart-api/tag/Request-and-Response-Compression/).")
public String getContentEncoding() {
return contentEncoding;
}
public void setContentEncoding(String contentEncoding) {
this.contentEncoding = contentEncoding;
}
public Headers zuoraOrgIds(String zuoraOrgIds) {
this.zuoraOrgIds = zuoraOrgIds;
return this;
}
/**
* A comma-separated list of organization IDs. If you have multi-org enabled, you can use this header to limit the data scope to the specified organizations.
* @return zuoraOrgIds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A comma-separated list of organization IDs. If you have multi-org enabled, you can use this header to limit the data scope to the specified organizations.")
public String getZuoraOrgIds() {
return zuoraOrgIds;
}
public void setZuoraOrgIds(String zuoraOrgIds) {
this.zuoraOrgIds = zuoraOrgIds;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Headers headers = (Headers) o;
return Objects.equals(this.zuoraTrackId, headers.zuoraTrackId) &&
Objects.equals(this.async, headers.async) &&
Objects.equals(this.zuoraCacheEnabled, headers.zuoraCacheEnabled) &&
Objects.equals(this.zuoraEntityIds, headers.zuoraEntityIds) &&
Objects.equals(this.idempotencyKey, headers.idempotencyKey) &&
Objects.equals(this.acceptEncoding, headers.acceptEncoding) &&
Objects.equals(this.contentEncoding, headers.contentEncoding) &&
Objects.equals(this.zuoraOrgIds, headers.zuoraOrgIds);
}
@Override
public int hashCode() {
return Objects.hash(zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Headers {\n");
sb.append(" zuoraTrackId: ").append(toIndentedString(zuoraTrackId)).append("\n");
sb.append(" async: ").append(toIndentedString(async)).append("\n");
sb.append(" zuoraCacheEnabled: ").append(toIndentedString(zuoraCacheEnabled)).append("\n");
sb.append(" zuoraEntityIds: ").append(toIndentedString(zuoraEntityIds)).append("\n");
sb.append(" idempotencyKey: ").append(toIndentedString(idempotencyKey)).append("\n");
sb.append(" acceptEncoding: ").append(toIndentedString(acceptEncoding)).append("\n");
sb.append(" contentEncoding: ").append(toIndentedString(contentEncoding)).append("\n");
sb.append(" zuoraOrgIds: ").append(toIndentedString(zuoraOrgIds)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy