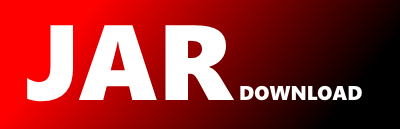
org.openapitools.client.model.InvoiceItemPreviewResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* InvoiceItemPreviewResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class InvoiceItemPreviewResponse {
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_APPLIED_TO_ITEM_ID = "applied_to_item_id";
@SerializedName(SERIALIZED_NAME_APPLIED_TO_ITEM_ID)
private String appliedToItemId;
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "document_date";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_DESCRIPTION = "subscription_item_description";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_DESCRIPTION)
private String subscriptionItemDescription;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_ID = "subscription_item_id";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_ID)
private String subscriptionItemId;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_NAME = "subscription_item_name";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_NAME)
private String subscriptionItemName;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_NUMBER = "subscription_item_number";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_NUMBER)
private String subscriptionItemNumber;
public static final String SERIALIZED_NAME_CHARGE_TYPE = "charge_type";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE)
private String chargeType;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
/**
* Gets or Sets processingType
*/
@JsonAdapter(ProcessingTypeEnum.Adapter.class)
public enum ProcessingTypeEnum {
SUBSCRIPTION_ITEM("subscription_item"),
DISCOUNT("discount"),
PREPAYMENT("prepayment"),
TAX("tax"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ProcessingTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ProcessingTypeEnum fromValue(String value) {
for (ProcessingTypeEnum b : ProcessingTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ProcessingTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ProcessingTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ProcessingTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PROCESSING_TYPE = "processing_type";
@SerializedName(SERIALIZED_NAME_PROCESSING_TYPE)
private ProcessingTypeEnum processingType;
public static final String SERIALIZED_NAME_PRODUCT_NAME = "product_name";
@SerializedName(SERIALIZED_NAME_PRODUCT_NAME)
private String productName;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_SERVICE_START_DATE = "service_start_date";
@SerializedName(SERIALIZED_NAME_SERVICE_START_DATE)
private String serviceStartDate;
public static final String SERIALIZED_NAME_SERVICE_END_DATE = "service_end_date";
@SerializedName(SERIALIZED_NAME_SERVICE_END_DATE)
private String serviceEndDate;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ID = "subscription_id";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ID)
private String subscriptionId;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBER = "subscription_number";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBER)
private String subscriptionNumber;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NAME = "subscription_name";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NAME)
private String subscriptionName;
public static final String SERIALIZED_NAME_TAX = "tax";
@SerializedName(SERIALIZED_NAME_TAX)
private BigDecimal tax;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unit_of_measure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public InvoiceItemPreviewResponse() {
}
public InvoiceItemPreviewResponse amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The total amount of this invoice item.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount of this invoice item.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public InvoiceItemPreviewResponse appliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
return this;
}
/**
* Identifier of an invoice item or a debit memo item that this discount item or credit memo item is applied to.
* @return appliedToItemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of an invoice item or a debit memo item that this discount item or credit memo item is applied to.")
public String getAppliedToItemId() {
return appliedToItemId;
}
public void setAppliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
}
public InvoiceItemPreviewResponse documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* Get documentDate
* @return documentDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "")
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public InvoiceItemPreviewResponse subscriptionItemDescription(String subscriptionItemDescription) {
this.subscriptionItemDescription = subscriptionItemDescription;
return this;
}
/**
* Get subscriptionItemDescription
* @return subscriptionItemDescription
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSubscriptionItemDescription() {
return subscriptionItemDescription;
}
public void setSubscriptionItemDescription(String subscriptionItemDescription) {
this.subscriptionItemDescription = subscriptionItemDescription;
}
public InvoiceItemPreviewResponse subscriptionItemId(String subscriptionItemId) {
this.subscriptionItemId = subscriptionItemId;
return this;
}
/**
* The identifier the subscription item associated with this invoice item.
* @return subscriptionItemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier the subscription item associated with this invoice item.")
public String getSubscriptionItemId() {
return subscriptionItemId;
}
public void setSubscriptionItemId(String subscriptionItemId) {
this.subscriptionItemId = subscriptionItemId;
}
public InvoiceItemPreviewResponse subscriptionItemName(String subscriptionItemName) {
this.subscriptionItemName = subscriptionItemName;
return this;
}
/**
* Get subscriptionItemName
* @return subscriptionItemName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSubscriptionItemName() {
return subscriptionItemName;
}
public void setSubscriptionItemName(String subscriptionItemName) {
this.subscriptionItemName = subscriptionItemName;
}
public InvoiceItemPreviewResponse subscriptionItemNumber(String subscriptionItemNumber) {
this.subscriptionItemNumber = subscriptionItemNumber;
return this;
}
/**
* Human-readable identifier of the subscription item. It can be user-supplied.
* @return subscriptionItemNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the subscription item. It can be user-supplied.")
public String getSubscriptionItemNumber() {
return subscriptionItemNumber;
}
public void setSubscriptionItemNumber(String subscriptionItemNumber) {
this.subscriptionItemNumber = subscriptionItemNumber;
}
public InvoiceItemPreviewResponse chargeType(String chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Type of the charge. It can be one of the following types: one-time, recurring, or usage.
* @return chargeType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Type of the charge. It can be one of the following types: one-time, recurring, or usage.")
public String getChargeType() {
return chargeType;
}
public void setChargeType(String chargeType) {
this.chargeType = chargeType;
}
public InvoiceItemPreviewResponse id(String id) {
this.id = id;
return this;
}
/**
* Unique identifier of the object
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the object")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public InvoiceItemPreviewResponse processingType(ProcessingTypeEnum processingType) {
this.processingType = processingType;
return this;
}
/**
* Get processingType
* @return processingType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ProcessingTypeEnum getProcessingType() {
return processingType;
}
public void setProcessingType(ProcessingTypeEnum processingType) {
this.processingType = processingType;
}
public InvoiceItemPreviewResponse productName(String productName) {
this.productName = productName;
return this;
}
/**
* Get productName
* @return productName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getProductName() {
return productName;
}
public void setProductName(String productName) {
this.productName = productName;
}
public InvoiceItemPreviewResponse quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The number of units of this item.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of units of this item.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public InvoiceItemPreviewResponse serviceStartDate(String serviceStartDate) {
this.serviceStartDate = serviceStartDate;
return this;
}
/**
* The start date of the service period associated with this invoice item. If the associated charge is a one-time fee, then this date is the date of that charge.
* @return serviceStartDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The start date of the service period associated with this invoice item. If the associated charge is a one-time fee, then this date is the date of that charge.")
public String getServiceStartDate() {
return serviceStartDate;
}
public void setServiceStartDate(String serviceStartDate) {
this.serviceStartDate = serviceStartDate;
}
public InvoiceItemPreviewResponse serviceEndDate(String serviceEndDate) {
this.serviceEndDate = serviceEndDate;
return this;
}
/**
* The end date of the service period associated with this invoice item. If the associated charge is a one-time fee, then this date is the date of that charge.
* @return serviceEndDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The end date of the service period associated with this invoice item. If the associated charge is a one-time fee, then this date is the date of that charge.")
public String getServiceEndDate() {
return serviceEndDate;
}
public void setServiceEndDate(String serviceEndDate) {
this.serviceEndDate = serviceEndDate;
}
public InvoiceItemPreviewResponse subscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* The identifier of the subscription associated with the invoice item.
* @return subscriptionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of the subscription associated with the invoice item.")
public String getSubscriptionId() {
return subscriptionId;
}
public void setSubscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
}
public InvoiceItemPreviewResponse subscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
return this;
}
/**
* The subscription number of the subscription associated with this item.
* @return subscriptionNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The subscription number of the subscription associated with this item.")
public String getSubscriptionNumber() {
return subscriptionNumber;
}
public void setSubscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
}
public InvoiceItemPreviewResponse subscriptionName(String subscriptionName) {
this.subscriptionName = subscriptionName;
return this;
}
/**
* The name of the subscription associated with this item.
* @return subscriptionName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the subscription associated with this item.")
public String getSubscriptionName() {
return subscriptionName;
}
public void setSubscriptionName(String subscriptionName) {
this.subscriptionName = subscriptionName;
}
public InvoiceItemPreviewResponse tax(BigDecimal tax) {
this.tax = tax;
return this;
}
/**
* The amount of tax applied to the invoice item.
* @return tax
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of tax applied to the invoice item.")
public BigDecimal getTax() {
return tax;
}
public void setTax(BigDecimal tax) {
this.tax = tax;
}
public InvoiceItemPreviewResponse unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* Specifies the units used to measure usage.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the units used to measure usage.")
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InvoiceItemPreviewResponse invoiceItemPreviewResponse = (InvoiceItemPreviewResponse) o;
return Objects.equals(this.amount, invoiceItemPreviewResponse.amount) &&
Objects.equals(this.appliedToItemId, invoiceItemPreviewResponse.appliedToItemId) &&
Objects.equals(this.documentDate, invoiceItemPreviewResponse.documentDate) &&
Objects.equals(this.subscriptionItemDescription, invoiceItemPreviewResponse.subscriptionItemDescription) &&
Objects.equals(this.subscriptionItemId, invoiceItemPreviewResponse.subscriptionItemId) &&
Objects.equals(this.subscriptionItemName, invoiceItemPreviewResponse.subscriptionItemName) &&
Objects.equals(this.subscriptionItemNumber, invoiceItemPreviewResponse.subscriptionItemNumber) &&
Objects.equals(this.chargeType, invoiceItemPreviewResponse.chargeType) &&
Objects.equals(this.id, invoiceItemPreviewResponse.id) &&
Objects.equals(this.processingType, invoiceItemPreviewResponse.processingType) &&
Objects.equals(this.productName, invoiceItemPreviewResponse.productName) &&
Objects.equals(this.quantity, invoiceItemPreviewResponse.quantity) &&
Objects.equals(this.serviceStartDate, invoiceItemPreviewResponse.serviceStartDate) &&
Objects.equals(this.serviceEndDate, invoiceItemPreviewResponse.serviceEndDate) &&
Objects.equals(this.subscriptionId, invoiceItemPreviewResponse.subscriptionId) &&
Objects.equals(this.subscriptionNumber, invoiceItemPreviewResponse.subscriptionNumber) &&
Objects.equals(this.subscriptionName, invoiceItemPreviewResponse.subscriptionName) &&
Objects.equals(this.tax, invoiceItemPreviewResponse.tax) &&
Objects.equals(this.unitOfMeasure, invoiceItemPreviewResponse.unitOfMeasure);
}
@Override
public int hashCode() {
return Objects.hash(amount, appliedToItemId, documentDate, subscriptionItemDescription, subscriptionItemId, subscriptionItemName, subscriptionItemNumber, chargeType, id, processingType, productName, quantity, serviceStartDate, serviceEndDate, subscriptionId, subscriptionNumber, subscriptionName, tax, unitOfMeasure);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class InvoiceItemPreviewResponse {\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" appliedToItemId: ").append(toIndentedString(appliedToItemId)).append("\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" subscriptionItemDescription: ").append(toIndentedString(subscriptionItemDescription)).append("\n");
sb.append(" subscriptionItemId: ").append(toIndentedString(subscriptionItemId)).append("\n");
sb.append(" subscriptionItemName: ").append(toIndentedString(subscriptionItemName)).append("\n");
sb.append(" subscriptionItemNumber: ").append(toIndentedString(subscriptionItemNumber)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" processingType: ").append(toIndentedString(processingType)).append("\n");
sb.append(" productName: ").append(toIndentedString(productName)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" serviceStartDate: ").append(toIndentedString(serviceStartDate)).append("\n");
sb.append(" serviceEndDate: ").append(toIndentedString(serviceEndDate)).append("\n");
sb.append(" subscriptionId: ").append(toIndentedString(subscriptionId)).append("\n");
sb.append(" subscriptionNumber: ").append(toIndentedString(subscriptionNumber)).append("\n");
sb.append(" subscriptionName: ").append(toIndentedString(subscriptionName)).append("\n");
sb.append(" tax: ").append(toIndentedString(tax)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy