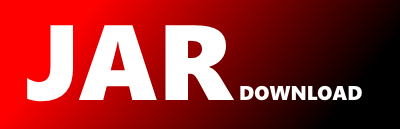
org.openapitools.client.model.LineItemCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.InvoiceItemListResponse;
import org.openapitools.client.model.Revenue;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Order line items
*/
@ApiModel(description = "Order line items")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class LineItemCreateRequest {
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unit_of_measure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public static final String SERIALIZED_NAME_UNIT_AMOUNT = "unit_amount";
@SerializedName(SERIALIZED_NAME_UNIT_AMOUNT)
private BigDecimal unitAmount;
public static final String SERIALIZED_NAME_TARGET_DATE = "target_date";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT = "deferred_revenue_account";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT)
private String deferredRevenueAccount;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_REVENUE = "revenue";
@SerializedName(SERIALIZED_NAME_REVENUE)
private Revenue revenue;
public static final String SERIALIZED_NAME_DISCOUNT_UNIT_AMOUNT = "discount_unit_amount";
@SerializedName(SERIALIZED_NAME_DISCOUNT_UNIT_AMOUNT)
private BigDecimal discountUnitAmount;
public static final String SERIALIZED_NAME_DISCOUNT_PERCENT = "discount_percent";
@SerializedName(SERIALIZED_NAME_DISCOUNT_PERCENT)
private BigDecimal discountPercent;
/**
* The category for the order line item, to indicate a product sale or return.
*/
@JsonAdapter(CategoryEnum.Adapter.class)
public enum CategoryEnum {
SALE("sale"),
RETURN("return"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
CategoryEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static CategoryEnum fromValue(String value) {
for (CategoryEnum b : CategoryEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final CategoryEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public CategoryEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return CategoryEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_CATEGORY = "category";
@SerializedName(SERIALIZED_NAME_CATEGORY)
private CategoryEnum category;
/**
* The state of an order line item. If you want to generate billing documents for order line items, you must set this field to `sent_to_billing`. For invoice preview, you do not need to set this field.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
PENDING("pending"),
BOOKED("booked"),
SENT_TO_BILLING("sent_to_billing"),
COMPLETE("complete"),
CANCELED("canceled"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
/**
* The type of the order line item.
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
PRODUCT("product"),
FEE("fee"),
SERVICES("services"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TypeEnum type;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_ITEM_NUMBER = "item_number";
@SerializedName(SERIALIZED_NAME_ITEM_NUMBER)
private String itemNumber;
public static final String SERIALIZED_NAME_LIST_UNIT_PRICE = "list_unit_price";
@SerializedName(SERIALIZED_NAME_LIST_UNIT_PRICE)
private BigDecimal listUnitPrice;
public static final String SERIALIZED_NAME_PRODUCT_CODE = "product_code";
@SerializedName(SERIALIZED_NAME_PRODUCT_CODE)
private String productCode;
public static final String SERIALIZED_NAME_PRICE_ID = "price_id";
@SerializedName(SERIALIZED_NAME_PRICE_ID)
private String priceId;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchase_order_number";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT = "recognized_revenue_account";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT)
private String recognizedRevenueAccount;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME = "revenue_recognition_rule_name";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME)
private String revenueRecognitionRuleName;
public static final String SERIALIZED_NAME_SOLD_TO_ID = "sold_to_id";
@SerializedName(SERIALIZED_NAME_SOLD_TO_ID)
private String soldToId;
public static final String SERIALIZED_NAME_TAX_CODE = "tax_code";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_INCLUSIVE = "tax_inclusive";
@SerializedName(SERIALIZED_NAME_TAX_INCLUSIVE)
private Boolean taxInclusive;
public static final String SERIALIZED_NAME_START_DATE = "start_date";
@SerializedName(SERIALIZED_NAME_START_DATE)
private LocalDate startDate;
public static final String SERIALIZED_NAME_END_DATE = "end_date";
@SerializedName(SERIALIZED_NAME_END_DATE)
private LocalDate endDate;
public static final String SERIALIZED_NAME_INVOICE_ITEMS = "invoice_items";
@SerializedName(SERIALIZED_NAME_INVOICE_ITEMS)
private InvoiceItemListResponse invoiceItems;
public static final String SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER = "related_subscription_number";
@SerializedName(SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER)
private String relatedSubscriptionNumber;
/**
* The billing rule for the Order Line Item.
*/
@JsonAdapter(BillingRuleEnum.Adapter.class)
public enum BillingRuleEnum {
WITHOUT_FULFILLMENT("trigger_without_fulfillment"),
ON_FULFILLMENT("trigger_on_fulfillment"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
BillingRuleEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static BillingRuleEnum fromValue(String value) {
for (BillingRuleEnum b : BillingRuleEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final BillingRuleEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public BillingRuleEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return BillingRuleEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_BILLING_RULE = "billing_rule";
@SerializedName(SERIALIZED_NAME_BILLING_RULE)
private BillingRuleEnum billingRule;
public LineItemCreateRequest() {
}
public LineItemCreateRequest(
InvoiceItemListResponse invoiceItems
) {
this();
this.invoiceItems = invoiceItems;
}
public LineItemCreateRequest unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* A configured unit of measure. This field is required for per-unit prices.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A configured unit of measure. This field is required for per-unit prices.")
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
public LineItemCreateRequest unitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* The unit amount of the price. Specify this field if you want to override the original price with a per-unit price.
* @return unitAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unit amount of the price. Specify this field if you want to override the original price with a per-unit price.")
public BigDecimal getUnitAmount() {
return unitAmount;
}
public void setUnitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
}
public LineItemCreateRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date for the order line item to be picked up by bill run for billing.
* @return targetDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The target date for the order line item to be picked up by bill run for billing.")
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public LineItemCreateRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public LineItemCreateRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public LineItemCreateRequest deferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return deferredRevenueAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getDeferredRevenueAccount() {
return deferredRevenueAccount;
}
public void setDeferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
}
public LineItemCreateRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public LineItemCreateRequest revenue(Revenue revenue) {
this.revenue = revenue;
return this;
}
/**
* Get revenue
* @return revenue
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Revenue getRevenue() {
return revenue;
}
public void setRevenue(Revenue revenue) {
this.revenue = revenue;
}
public LineItemCreateRequest discountUnitAmount(BigDecimal discountUnitAmount) {
this.discountUnitAmount = discountUnitAmount;
return this;
}
/**
* Discount amount. Specify this field if you offer an amount-based discount.
* @return discountUnitAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Discount amount. Specify this field if you offer an amount-based discount.")
public BigDecimal getDiscountUnitAmount() {
return discountUnitAmount;
}
public void setDiscountUnitAmount(BigDecimal discountUnitAmount) {
this.discountUnitAmount = discountUnitAmount;
}
public LineItemCreateRequest discountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
return this;
}
/**
* Discount percent. Specify this field if you offer a percentage-based discount.
* @return discountPercent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Discount percent. Specify this field if you offer a percentage-based discount.")
public BigDecimal getDiscountPercent() {
return discountPercent;
}
public void setDiscountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
}
public LineItemCreateRequest category(CategoryEnum category) {
this.category = category;
return this;
}
/**
* The category for the order line item, to indicate a product sale or return.
* @return category
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The category for the order line item, to indicate a product sale or return.")
public CategoryEnum getCategory() {
return category;
}
public void setCategory(CategoryEnum category) {
this.category = category;
}
public LineItemCreateRequest state(StateEnum state) {
this.state = state;
return this;
}
/**
* The state of an order line item. If you want to generate billing documents for order line items, you must set this field to `sent_to_billing`. For invoice preview, you do not need to set this field.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The state of an order line item. If you want to generate billing documents for order line items, you must set this field to `sent_to_billing`. For invoice preview, you do not need to set this field.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public LineItemCreateRequest type(TypeEnum type) {
this.type = type;
return this;
}
/**
* The type of the order line item.
* @return type
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The type of the order line item.")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public LineItemCreateRequest name(String name) {
this.name = name;
return this;
}
/**
* The name of the order line item.
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The name of the order line item.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public LineItemCreateRequest itemNumber(String itemNumber) {
this.itemNumber = itemNumber;
return this;
}
/**
* Human-readable identifier of the order item. It can be user-supplied.
* @return itemNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the order item. It can be user-supplied.")
public String getItemNumber() {
return itemNumber;
}
public void setItemNumber(String itemNumber) {
this.itemNumber = itemNumber;
}
public LineItemCreateRequest listUnitPrice(BigDecimal listUnitPrice) {
this.listUnitPrice = listUnitPrice;
return this;
}
/**
* The list price per unit for the order line item.
* @return listUnitPrice
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The list price per unit for the order line item.")
public BigDecimal getListUnitPrice() {
return listUnitPrice;
}
public void setListUnitPrice(BigDecimal listUnitPrice) {
this.listUnitPrice = listUnitPrice;
}
public LineItemCreateRequest productCode(String productCode) {
this.productCode = productCode;
return this;
}
/**
* The product code for the order line item.
* @return productCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The product code for the order line item.")
public String getProductCode() {
return productCode;
}
public void setProductCode(String productCode) {
this.productCode = productCode;
}
public LineItemCreateRequest priceId(String priceId) {
this.priceId = priceId;
return this;
}
/**
* Identifier of the price.
* @return priceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the price.")
public String getPriceId() {
return priceId;
}
public void setPriceId(String priceId) {
this.priceId = priceId;
}
public LineItemCreateRequest purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* Used by customers to specify the Purchase Order Number provided by the buyer.
* @return purchaseOrderNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Used by customers to specify the Purchase Order Number provided by the buyer.")
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public LineItemCreateRequest quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The quantity of units, such as the number of authors in a hosted wiki service.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The quantity of units, such as the number of authors in a hosted wiki service.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public LineItemCreateRequest recognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return recognizedRevenueAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getRecognizedRevenueAccount() {
return recognizedRevenueAccount;
}
public void setRecognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
}
public LineItemCreateRequest revenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
return this;
}
/**
* The revenue recognition rule for the order line item.
* @return revenueRecognitionRuleName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The revenue recognition rule for the order line item.")
public String getRevenueRecognitionRuleName() {
return revenueRecognitionRuleName;
}
public void setRevenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
}
public LineItemCreateRequest soldToId(String soldToId) {
this.soldToId = soldToId;
return this;
}
/**
* Customer address used for calculating tax.
* @return soldToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad0823f8040e52d0180433026b156fe", value = "Customer address used for calculating tax.")
public String getSoldToId() {
return soldToId;
}
public void setSoldToId(String soldToId) {
this.soldToId = soldToId;
}
public LineItemCreateRequest taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* A tax code identifier. If a `tax_code` of a price is not provided when you create or update a price, Zuora will treat the charged amount as non-taxable. If this code is provide, Zuora considers that this price is taxable and the charged amount will be handled accordingly.
* @return taxCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A tax code identifier. If a `tax_code` of a price is not provided when you create or update a price, Zuora will treat the charged amount as non-taxable. If this code is provide, Zuora considers that this price is taxable and the charged amount will be handled accordingly.")
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public LineItemCreateRequest taxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
return this;
}
/**
* If this field is set to `true`, it indicates that amounts are inclusive of tax.
* @return taxInclusive
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If this field is set to `true`, it indicates that amounts are inclusive of tax.")
public Boolean getTaxInclusive() {
return taxInclusive;
}
public void setTaxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
}
public LineItemCreateRequest startDate(LocalDate startDate) {
this.startDate = startDate;
return this;
}
/**
* The date a transaction starts. The default value of this field is the order date.
* @return startDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "The date a transaction starts. The default value of this field is the order date.")
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public LineItemCreateRequest endDate(LocalDate endDate) {
this.endDate = endDate;
return this;
}
/**
* The date a transaction is completed. The default value of this field is the transaction start date. Also, the value of this field should always equal or be later than the value of the `start_date` field.
* @return endDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "The date a transaction is completed. The default value of this field is the transaction start date. Also, the value of this field should always equal or be later than the value of the `start_date` field.")
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
/**
* List of invoice items. EXPANDABLE
* @return invoiceItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of invoice items. EXPANDABLE")
public InvoiceItemListResponse getInvoiceItems() {
return invoiceItems;
}
public LineItemCreateRequest relatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
return this;
}
/**
* Relates an order line item to a subscription when you create the order line item.
* @return relatedSubscriptionNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Relates an order line item to a subscription when you create the order line item.")
public String getRelatedSubscriptionNumber() {
return relatedSubscriptionNumber;
}
public void setRelatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
}
public LineItemCreateRequest billingRule(BillingRuleEnum billingRule) {
this.billingRule = billingRule;
return this;
}
/**
* The billing rule for the Order Line Item.
* @return billingRule
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing rule for the Order Line Item.")
public BillingRuleEnum getBillingRule() {
return billingRule;
}
public void setBillingRule(BillingRuleEnum billingRule) {
this.billingRule = billingRule;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LineItemCreateRequest lineItemCreateRequest = (LineItemCreateRequest) o;
return Objects.equals(this.unitOfMeasure, lineItemCreateRequest.unitOfMeasure) &&
Objects.equals(this.unitAmount, lineItemCreateRequest.unitAmount) &&
Objects.equals(this.targetDate, lineItemCreateRequest.targetDate) &&
Objects.equals(this.customFields, lineItemCreateRequest.customFields) &&
Objects.equals(this.deferredRevenueAccount, lineItemCreateRequest.deferredRevenueAccount) &&
Objects.equals(this.description, lineItemCreateRequest.description) &&
Objects.equals(this.revenue, lineItemCreateRequest.revenue) &&
Objects.equals(this.discountUnitAmount, lineItemCreateRequest.discountUnitAmount) &&
Objects.equals(this.discountPercent, lineItemCreateRequest.discountPercent) &&
Objects.equals(this.category, lineItemCreateRequest.category) &&
Objects.equals(this.state, lineItemCreateRequest.state) &&
Objects.equals(this.type, lineItemCreateRequest.type) &&
Objects.equals(this.name, lineItemCreateRequest.name) &&
Objects.equals(this.itemNumber, lineItemCreateRequest.itemNumber) &&
Objects.equals(this.listUnitPrice, lineItemCreateRequest.listUnitPrice) &&
Objects.equals(this.productCode, lineItemCreateRequest.productCode) &&
Objects.equals(this.priceId, lineItemCreateRequest.priceId) &&
Objects.equals(this.purchaseOrderNumber, lineItemCreateRequest.purchaseOrderNumber) &&
Objects.equals(this.quantity, lineItemCreateRequest.quantity) &&
Objects.equals(this.recognizedRevenueAccount, lineItemCreateRequest.recognizedRevenueAccount) &&
Objects.equals(this.revenueRecognitionRuleName, lineItemCreateRequest.revenueRecognitionRuleName) &&
Objects.equals(this.soldToId, lineItemCreateRequest.soldToId) &&
Objects.equals(this.taxCode, lineItemCreateRequest.taxCode) &&
Objects.equals(this.taxInclusive, lineItemCreateRequest.taxInclusive) &&
Objects.equals(this.startDate, lineItemCreateRequest.startDate) &&
Objects.equals(this.endDate, lineItemCreateRequest.endDate) &&
Objects.equals(this.invoiceItems, lineItemCreateRequest.invoiceItems) &&
Objects.equals(this.relatedSubscriptionNumber, lineItemCreateRequest.relatedSubscriptionNumber) &&
Objects.equals(this.billingRule, lineItemCreateRequest.billingRule);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(unitOfMeasure, unitAmount, targetDate, customFields, deferredRevenueAccount, description, revenue, discountUnitAmount, discountPercent, category, state, type, name, itemNumber, listUnitPrice, productCode, priceId, purchaseOrderNumber, quantity, recognizedRevenueAccount, revenueRecognitionRuleName, soldToId, taxCode, taxInclusive, startDate, endDate, invoiceItems, relatedSubscriptionNumber, billingRule);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LineItemCreateRequest {\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append(" unitAmount: ").append(toIndentedString(unitAmount)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" deferredRevenueAccount: ").append(toIndentedString(deferredRevenueAccount)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" revenue: ").append(toIndentedString(revenue)).append("\n");
sb.append(" discountUnitAmount: ").append(toIndentedString(discountUnitAmount)).append("\n");
sb.append(" discountPercent: ").append(toIndentedString(discountPercent)).append("\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" itemNumber: ").append(toIndentedString(itemNumber)).append("\n");
sb.append(" listUnitPrice: ").append(toIndentedString(listUnitPrice)).append("\n");
sb.append(" productCode: ").append(toIndentedString(productCode)).append("\n");
sb.append(" priceId: ").append(toIndentedString(priceId)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" recognizedRevenueAccount: ").append(toIndentedString(recognizedRevenueAccount)).append("\n");
sb.append(" revenueRecognitionRuleName: ").append(toIndentedString(revenueRecognitionRuleName)).append("\n");
sb.append(" soldToId: ").append(toIndentedString(soldToId)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxInclusive: ").append(toIndentedString(taxInclusive)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" invoiceItems: ").append(toIndentedString(invoiceItems)).append("\n");
sb.append(" relatedSubscriptionNumber: ").append(toIndentedString(relatedSubscriptionNumber)).append("\n");
sb.append(" billingRule: ").append(toIndentedString(billingRule)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy