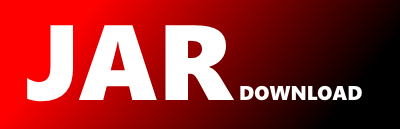
org.openapitools.client.model.LineItemPatchRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.OrderLineItemRevenue;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Update an order line item
*/
@ApiModel(description = "Update an order line item")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class LineItemPatchRequest {
public static final String SERIALIZED_NAME_REVENUE = "revenue";
@SerializedName(SERIALIZED_NAME_REVENUE)
private OrderLineItemRevenue revenue;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unit_of_measure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accounting_code";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNT = "adjustment_liability_account";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNT)
private String adjustmentLiabilityAccount;
public static final String SERIALIZED_NAME_UNIT_AMOUNT = "unit_amount";
@SerializedName(SERIALIZED_NAME_UNIT_AMOUNT)
private BigDecimal unitAmount;
public static final String SERIALIZED_NAME_TARGET_DATE = "target_date";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
/**
* The billing rule for the order line item.
*/
@JsonAdapter(BillingRuleEnum.Adapter.class)
public enum BillingRuleEnum {
WITHOUT_FULFILLMENT("trigger_without_fulfillment"),
ON_FULFILLMENT("trigger_on_fulfillment"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
BillingRuleEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static BillingRuleEnum fromValue(String value) {
for (BillingRuleEnum b : BillingRuleEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final BillingRuleEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public BillingRuleEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return BillingRuleEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_BILLING_RULE = "billing_rule";
@SerializedName(SERIALIZED_NAME_BILLING_RULE)
private BillingRuleEnum billingRule;
public static final String SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNT = "contract_asset_account";
@SerializedName(SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNT)
private String contractAssetAccount;
public static final String SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNT = "contract_liability_account";
@SerializedName(SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNT)
private String contractLiabilityAccount;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISCOUNT_UNIT_AMOUNT = "discount_unit_amount";
@SerializedName(SERIALIZED_NAME_DISCOUNT_UNIT_AMOUNT)
private BigDecimal discountUnitAmount;
public static final String SERIALIZED_NAME_DISCOUNT_PERCENT = "discount_percent";
@SerializedName(SERIALIZED_NAME_DISCOUNT_PERCENT)
private BigDecimal discountPercent;
/**
* Category of the order line item.
*/
@JsonAdapter(CategoryEnum.Adapter.class)
public enum CategoryEnum {
SALE("sale"),
RETURN("return"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
CategoryEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static CategoryEnum fromValue(String value) {
for (CategoryEnum b : CategoryEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final CategoryEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public CategoryEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return CategoryEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_CATEGORY = "category";
@SerializedName(SERIALIZED_NAME_CATEGORY)
private CategoryEnum category;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
/**
* The type of the order line item.
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
PRODUCT("product"),
FEE("fee"),
SERVICES("services"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TypeEnum type;
public static final String SERIALIZED_NAME_LIST_UNIT_PRICE = "list_unit_price";
@SerializedName(SERIALIZED_NAME_LIST_UNIT_PRICE)
private BigDecimal listUnitPrice;
public static final String SERIALIZED_NAME_PRODUCT_CODE = "product_code";
@SerializedName(SERIALIZED_NAME_PRODUCT_CODE)
private String productCode;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchase_order_number";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER = "related_subscription_number";
@SerializedName(SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER)
private String relatedSubscriptionNumber;
public static final String SERIALIZED_NAME_SOLD_TO_ID = "sold_to_id";
@SerializedName(SERIALIZED_NAME_SOLD_TO_ID)
private String soldToId;
public static final String SERIALIZED_NAME_TAX_CODE = "tax_code";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNT = "unbilled_receivables_account";
@SerializedName(SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNT)
private String unbilledReceivablesAccount;
/**
* The state of an order line item. If you want to generate billing documents for order line items, you must set this field to `sent_to_billing`. For invoice preview, you do not need to set this field.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
PENDING("pending"),
BOOKED("booked"),
SENT_TO_BILLING("sent_to_billing"),
COMPLETE("complete"),
CANCELED("canceled"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_ITEM_NUMBER = "item_number";
@SerializedName(SERIALIZED_NAME_ITEM_NUMBER)
private String itemNumber;
public static final String SERIALIZED_NAME_START_DATE = "start_date";
@SerializedName(SERIALIZED_NAME_START_DATE)
private LocalDate startDate;
public static final String SERIALIZED_NAME_END_DATE = "end_date";
@SerializedName(SERIALIZED_NAME_END_DATE)
private LocalDate endDate;
public LineItemPatchRequest() {
}
public LineItemPatchRequest revenue(OrderLineItemRevenue revenue) {
this.revenue = revenue;
return this;
}
/**
* Get revenue
* @return revenue
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public OrderLineItemRevenue getRevenue() {
return revenue;
}
public void setRevenue(OrderLineItemRevenue revenue) {
this.revenue = revenue;
}
public LineItemPatchRequest unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* A configured unit of measure.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A configured unit of measure.")
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
public LineItemPatchRequest accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return accountingCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public LineItemPatchRequest adjustmentLiabilityAccount(String adjustmentLiabilityAccount) {
this.adjustmentLiabilityAccount = adjustmentLiabilityAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return adjustmentLiabilityAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getAdjustmentLiabilityAccount() {
return adjustmentLiabilityAccount;
}
public void setAdjustmentLiabilityAccount(String adjustmentLiabilityAccount) {
this.adjustmentLiabilityAccount = adjustmentLiabilityAccount;
}
public LineItemPatchRequest unitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* The unit amount to be charged.
* @return unitAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unit amount to be charged.")
public BigDecimal getUnitAmount() {
return unitAmount;
}
public void setUnitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
}
public LineItemPatchRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* All order line items that were unbilled on or before this date are included in future bill runs.
* @return targetDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "All order line items that were unbilled on or before this date are included in future bill runs.")
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public LineItemPatchRequest billingRule(BillingRuleEnum billingRule) {
this.billingRule = billingRule;
return this;
}
/**
* The billing rule for the order line item.
* @return billingRule
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing rule for the order line item.")
public BillingRuleEnum getBillingRule() {
return billingRule;
}
public void setBillingRule(BillingRuleEnum billingRule) {
this.billingRule = billingRule;
}
public LineItemPatchRequest contractAssetAccount(String contractAssetAccount) {
this.contractAssetAccount = contractAssetAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return contractAssetAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getContractAssetAccount() {
return contractAssetAccount;
}
public void setContractAssetAccount(String contractAssetAccount) {
this.contractAssetAccount = contractAssetAccount;
}
public LineItemPatchRequest contractLiabilityAccount(String contractLiabilityAccount) {
this.contractLiabilityAccount = contractLiabilityAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return contractLiabilityAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getContractLiabilityAccount() {
return contractLiabilityAccount;
}
public void setContractLiabilityAccount(String contractLiabilityAccount) {
this.contractLiabilityAccount = contractLiabilityAccount;
}
public LineItemPatchRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public LineItemPatchRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public LineItemPatchRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public LineItemPatchRequest discountUnitAmount(BigDecimal discountUnitAmount) {
this.discountUnitAmount = discountUnitAmount;
return this;
}
/**
* Discount amount per unit.
* @return discountUnitAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Discount amount per unit.")
public BigDecimal getDiscountUnitAmount() {
return discountUnitAmount;
}
public void setDiscountUnitAmount(BigDecimal discountUnitAmount) {
this.discountUnitAmount = discountUnitAmount;
}
public LineItemPatchRequest discountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
return this;
}
/**
* Discount percent.
* @return discountPercent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Discount percent.")
public BigDecimal getDiscountPercent() {
return discountPercent;
}
public void setDiscountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
}
public LineItemPatchRequest category(CategoryEnum category) {
this.category = category;
return this;
}
/**
* Category of the order line item.
* @return category
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Category of the order line item.")
public CategoryEnum getCategory() {
return category;
}
public void setCategory(CategoryEnum category) {
this.category = category;
}
public LineItemPatchRequest name(String name) {
this.name = name;
return this;
}
/**
* The name of the order line item.
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the order line item.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public LineItemPatchRequest type(TypeEnum type) {
this.type = type;
return this;
}
/**
* The type of the order line item.
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The type of the order line item.")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public LineItemPatchRequest listUnitPrice(BigDecimal listUnitPrice) {
this.listUnitPrice = listUnitPrice;
return this;
}
/**
* The list price per unit for the order line item.
* @return listUnitPrice
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The list price per unit for the order line item.")
public BigDecimal getListUnitPrice() {
return listUnitPrice;
}
public void setListUnitPrice(BigDecimal listUnitPrice) {
this.listUnitPrice = listUnitPrice;
}
public LineItemPatchRequest productCode(String productCode) {
this.productCode = productCode;
return this;
}
/**
* The product code for the order line item.
* @return productCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The product code for the order line item.")
public String getProductCode() {
return productCode;
}
public void setProductCode(String productCode) {
this.productCode = productCode;
}
public LineItemPatchRequest purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* Used by customers to specify the Purchase Order Number provided by the buyer.
* @return purchaseOrderNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Used by customers to specify the Purchase Order Number provided by the buyer.")
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public LineItemPatchRequest quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The quantity of the product ordered.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The quantity of the product ordered.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public LineItemPatchRequest relatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
return this;
}
/**
* Use this field to relate an order line item to an subscription.
* @return relatedSubscriptionNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Use this field to relate an order line item to an subscription.")
public String getRelatedSubscriptionNumber() {
return relatedSubscriptionNumber;
}
public void setRelatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
}
public LineItemPatchRequest soldToId(String soldToId) {
this.soldToId = soldToId;
return this;
}
/**
* The unique identifier of a contact belonging to the billing account of the order line item. Use this field to assign and existing contact as the sold to contact of an order line item.
* @return soldToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad0823f8040e52d0180433026b156fe", value = "The unique identifier of a contact belonging to the billing account of the order line item. Use this field to assign and existing contact as the sold to contact of an order line item.")
public String getSoldToId() {
return soldToId;
}
public void setSoldToId(String soldToId) {
this.soldToId = soldToId;
}
public LineItemPatchRequest taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* The tax code for the order line item.
* @return taxCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The tax code for the order line item.")
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public LineItemPatchRequest unbilledReceivablesAccount(String unbilledReceivablesAccount) {
this.unbilledReceivablesAccount = unbilledReceivablesAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts..
* @return unbilledReceivablesAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts..")
public String getUnbilledReceivablesAccount() {
return unbilledReceivablesAccount;
}
public void setUnbilledReceivablesAccount(String unbilledReceivablesAccount) {
this.unbilledReceivablesAccount = unbilledReceivablesAccount;
}
public LineItemPatchRequest state(StateEnum state) {
this.state = state;
return this;
}
/**
* The state of an order line item. If you want to generate billing documents for order line items, you must set this field to `sent_to_billing`. For invoice preview, you do not need to set this field.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The state of an order line item. If you want to generate billing documents for order line items, you must set this field to `sent_to_billing`. For invoice preview, you do not need to set this field.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public LineItemPatchRequest itemNumber(String itemNumber) {
this.itemNumber = itemNumber;
return this;
}
/**
* Human-readable identifier of the order item. It can be user-supplied.
* @return itemNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the order item. It can be user-supplied.")
public String getItemNumber() {
return itemNumber;
}
public void setItemNumber(String itemNumber) {
this.itemNumber = itemNumber;
}
public LineItemPatchRequest startDate(LocalDate startDate) {
this.startDate = startDate;
return this;
}
/**
* The date a transaction starts. The default value of this field is the order date.
* @return startDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "The date a transaction starts. The default value of this field is the order date.")
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public LineItemPatchRequest endDate(LocalDate endDate) {
this.endDate = endDate;
return this;
}
/**
* The date the order line item transitions to complete.
* @return endDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "The date the order line item transitions to complete.")
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LineItemPatchRequest lineItemPatchRequest = (LineItemPatchRequest) o;
return Objects.equals(this.revenue, lineItemPatchRequest.revenue) &&
Objects.equals(this.unitOfMeasure, lineItemPatchRequest.unitOfMeasure) &&
Objects.equals(this.accountingCode, lineItemPatchRequest.accountingCode) &&
Objects.equals(this.adjustmentLiabilityAccount, lineItemPatchRequest.adjustmentLiabilityAccount) &&
Objects.equals(this.unitAmount, lineItemPatchRequest.unitAmount) &&
Objects.equals(this.targetDate, lineItemPatchRequest.targetDate) &&
Objects.equals(this.billingRule, lineItemPatchRequest.billingRule) &&
Objects.equals(this.contractAssetAccount, lineItemPatchRequest.contractAssetAccount) &&
Objects.equals(this.contractLiabilityAccount, lineItemPatchRequest.contractLiabilityAccount) &&
Objects.equals(this.customFields, lineItemPatchRequest.customFields) &&
Objects.equals(this.description, lineItemPatchRequest.description) &&
Objects.equals(this.discountUnitAmount, lineItemPatchRequest.discountUnitAmount) &&
Objects.equals(this.discountPercent, lineItemPatchRequest.discountPercent) &&
Objects.equals(this.category, lineItemPatchRequest.category) &&
Objects.equals(this.name, lineItemPatchRequest.name) &&
Objects.equals(this.type, lineItemPatchRequest.type) &&
Objects.equals(this.listUnitPrice, lineItemPatchRequest.listUnitPrice) &&
Objects.equals(this.productCode, lineItemPatchRequest.productCode) &&
Objects.equals(this.purchaseOrderNumber, lineItemPatchRequest.purchaseOrderNumber) &&
Objects.equals(this.quantity, lineItemPatchRequest.quantity) &&
Objects.equals(this.relatedSubscriptionNumber, lineItemPatchRequest.relatedSubscriptionNumber) &&
Objects.equals(this.soldToId, lineItemPatchRequest.soldToId) &&
Objects.equals(this.taxCode, lineItemPatchRequest.taxCode) &&
Objects.equals(this.unbilledReceivablesAccount, lineItemPatchRequest.unbilledReceivablesAccount) &&
Objects.equals(this.state, lineItemPatchRequest.state) &&
Objects.equals(this.itemNumber, lineItemPatchRequest.itemNumber) &&
Objects.equals(this.startDate, lineItemPatchRequest.startDate) &&
Objects.equals(this.endDate, lineItemPatchRequest.endDate);
}
@Override
public int hashCode() {
return Objects.hash(revenue, unitOfMeasure, accountingCode, adjustmentLiabilityAccount, unitAmount, targetDate, billingRule, contractAssetAccount, contractLiabilityAccount, customFields, description, discountUnitAmount, discountPercent, category, name, type, listUnitPrice, productCode, purchaseOrderNumber, quantity, relatedSubscriptionNumber, soldToId, taxCode, unbilledReceivablesAccount, state, itemNumber, startDate, endDate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LineItemPatchRequest {\n");
sb.append(" revenue: ").append(toIndentedString(revenue)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" adjustmentLiabilityAccount: ").append(toIndentedString(adjustmentLiabilityAccount)).append("\n");
sb.append(" unitAmount: ").append(toIndentedString(unitAmount)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" billingRule: ").append(toIndentedString(billingRule)).append("\n");
sb.append(" contractAssetAccount: ").append(toIndentedString(contractAssetAccount)).append("\n");
sb.append(" contractLiabilityAccount: ").append(toIndentedString(contractLiabilityAccount)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" discountUnitAmount: ").append(toIndentedString(discountUnitAmount)).append("\n");
sb.append(" discountPercent: ").append(toIndentedString(discountPercent)).append("\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" listUnitPrice: ").append(toIndentedString(listUnitPrice)).append("\n");
sb.append(" productCode: ").append(toIndentedString(productCode)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" relatedSubscriptionNumber: ").append(toIndentedString(relatedSubscriptionNumber)).append("\n");
sb.append(" soldToId: ").append(toIndentedString(soldToId)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" unbilledReceivablesAccount: ").append(toIndentedString(unbilledReceivablesAccount)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" itemNumber: ").append(toIndentedString(itemNumber)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy