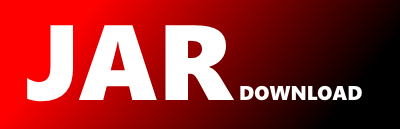
org.openapitools.client.model.OrderAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.openapitools.client.model.StartOnResponse;
import org.openapitools.client.model.SubscriptionAddPlanPatchResponse;
import org.openapitools.client.model.SubscriptionCancelPatchResponse;
import org.openapitools.client.model.SubscriptionPausePatchResponse;
import org.openapitools.client.model.SubscriptionPlanListResponse;
import org.openapitools.client.model.SubscriptionRemovePlanPatchResponse;
import org.openapitools.client.model.SubscriptionRenewPatchResponse;
import org.openapitools.client.model.SubscriptionReplacePlanPatchResponse;
import org.openapitools.client.model.SubscriptionResumePatchResponse;
import org.openapitools.client.model.SubscriptionTermPatchResponse;
import org.openapitools.client.model.SubscriptionUpdatePlanPatchResponse;
import org.openapitools.jackson.nullable.JsonNullable;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* OrderAction
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class OrderAction {
public static final String SERIALIZED_NAME_ACTION_ID = "action_id";
@SerializedName(SERIALIZED_NAME_ACTION_ID)
private String actionId;
/**
* The action associated with this metric.
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
CREATE_SUBSCRIPTION("create_subscription"),
TERMS("terms"),
ADD_SUBSCRIPTION_PLAN("add_subscription_plan"),
UPDATE_SUBSCRIPTION_PLAN("update_subscription_plan"),
REMOVE_SUBSCRIPTION_PLAN("remove_subscription_plan"),
RENEW("renew"),
CANCEL("cancel"),
OWNER_TRANSFER("owner_transfer"),
PAUSE("pause"),
RESUME("resume"),
REPLACE_SUBSCRIPTION_PLAN("replace_subscription_plan"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TypeEnum type;
public static final String SERIALIZED_NAME_SEQUENCE = "sequence";
@SerializedName(SERIALIZED_NAME_SEQUENCE)
private Integer sequence;
public static final String SERIALIZED_NAME_START_ON = "start_on";
@SerializedName(SERIALIZED_NAME_START_ON)
private StartOnResponse startOn;
public static final String SERIALIZED_NAME_SUBSCRIPTION_PLANS = "subscription_plans";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_PLANS)
private SubscriptionPlanListResponse subscriptionPlans;
public static final String SERIALIZED_NAME_ADD_SUBSCRIPTION_PLAN = "add_subscription_plan";
@SerializedName(SERIALIZED_NAME_ADD_SUBSCRIPTION_PLAN)
private SubscriptionAddPlanPatchResponse addSubscriptionPlan;
public static final String SERIALIZED_NAME_REMOVE_SUBSCRIPTION_PLAN = "remove_subscription_plan";
@SerializedName(SERIALIZED_NAME_REMOVE_SUBSCRIPTION_PLAN)
private SubscriptionRemovePlanPatchResponse removeSubscriptionPlan;
public static final String SERIALIZED_NAME_UPDATE_SUBSCRIPTION_PLAN = "update_subscription_plan";
@SerializedName(SERIALIZED_NAME_UPDATE_SUBSCRIPTION_PLAN)
private SubscriptionUpdatePlanPatchResponse updateSubscriptionPlan;
public static final String SERIALIZED_NAME_REPLACE_SUBSCRIPTION_PLAN = "replace_subscription_plan";
@SerializedName(SERIALIZED_NAME_REPLACE_SUBSCRIPTION_PLAN)
private SubscriptionReplacePlanPatchResponse replaceSubscriptionPlan;
public static final String SERIALIZED_NAME_RENEW = "renew";
@SerializedName(SERIALIZED_NAME_RENEW)
private SubscriptionRenewPatchResponse renew;
public static final String SERIALIZED_NAME_TERMS = "terms";
@SerializedName(SERIALIZED_NAME_TERMS)
private SubscriptionTermPatchResponse terms;
public static final String SERIALIZED_NAME_CANCEL = "cancel";
@SerializedName(SERIALIZED_NAME_CANCEL)
private SubscriptionCancelPatchResponse cancel;
public static final String SERIALIZED_NAME_PAUSE = "pause";
@SerializedName(SERIALIZED_NAME_PAUSE)
private SubscriptionPausePatchResponse pause;
public static final String SERIALIZED_NAME_RESUME = "resume";
@SerializedName(SERIALIZED_NAME_RESUME)
private SubscriptionResumePatchResponse resume;
public OrderAction() {
}
public OrderAction actionId(String actionId) {
this.actionId = actionId;
return this;
}
/**
* Identifier of the action.
* @return actionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the action.")
public String getActionId() {
return actionId;
}
public void setActionId(String actionId) {
this.actionId = actionId;
}
public OrderAction type(TypeEnum type) {
this.type = type;
return this;
}
/**
* The action associated with this metric.
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The action associated with this metric.")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public OrderAction sequence(Integer sequence) {
this.sequence = sequence;
return this;
}
/**
* The sequence number of the action.
* @return sequence
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The sequence number of the action.")
public Integer getSequence() {
return sequence;
}
public void setSequence(Integer sequence) {
this.sequence = sequence;
}
public OrderAction startOn(StartOnResponse startOn) {
this.startOn = startOn;
return this;
}
/**
* Get startOn
* @return startOn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public StartOnResponse getStartOn() {
return startOn;
}
public void setStartOn(StartOnResponse startOn) {
this.startOn = startOn;
}
public OrderAction subscriptionPlans(SubscriptionPlanListResponse subscriptionPlans) {
this.subscriptionPlans = subscriptionPlans;
return this;
}
/**
* Specify this field to add new plans to the new subscription.
* @return subscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field to add new plans to the new subscription.")
public SubscriptionPlanListResponse getSubscriptionPlans() {
return subscriptionPlans;
}
public void setSubscriptionPlans(SubscriptionPlanListResponse subscriptionPlans) {
this.subscriptionPlans = subscriptionPlans;
}
public OrderAction addSubscriptionPlan(SubscriptionAddPlanPatchResponse addSubscriptionPlan) {
this.addSubscriptionPlan = addSubscriptionPlan;
return this;
}
/**
* Specify this field to add subscription plans to existing subscriptions.
* @return addSubscriptionPlan
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field to add subscription plans to existing subscriptions.")
public SubscriptionAddPlanPatchResponse getAddSubscriptionPlan() {
return addSubscriptionPlan;
}
public void setAddSubscriptionPlan(SubscriptionAddPlanPatchResponse addSubscriptionPlan) {
this.addSubscriptionPlan = addSubscriptionPlan;
}
public OrderAction removeSubscriptionPlan(SubscriptionRemovePlanPatchResponse removeSubscriptionPlan) {
this.removeSubscriptionPlan = removeSubscriptionPlan;
return this;
}
/**
* Specify this field to remove subscription plans from existing subscriptions.
* @return removeSubscriptionPlan
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field to remove subscription plans from existing subscriptions.")
public SubscriptionRemovePlanPatchResponse getRemoveSubscriptionPlan() {
return removeSubscriptionPlan;
}
public void setRemoveSubscriptionPlan(SubscriptionRemovePlanPatchResponse removeSubscriptionPlan) {
this.removeSubscriptionPlan = removeSubscriptionPlan;
}
public OrderAction updateSubscriptionPlan(SubscriptionUpdatePlanPatchResponse updateSubscriptionPlan) {
this.updateSubscriptionPlan = updateSubscriptionPlan;
return this;
}
/**
* Specify this field to update subscription plans on the existing subscriptions.
* @return updateSubscriptionPlan
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field to update subscription plans on the existing subscriptions.")
public SubscriptionUpdatePlanPatchResponse getUpdateSubscriptionPlan() {
return updateSubscriptionPlan;
}
public void setUpdateSubscriptionPlan(SubscriptionUpdatePlanPatchResponse updateSubscriptionPlan) {
this.updateSubscriptionPlan = updateSubscriptionPlan;
}
public OrderAction replaceSubscriptionPlan(SubscriptionReplacePlanPatchResponse replaceSubscriptionPlan) {
this.replaceSubscriptionPlan = replaceSubscriptionPlan;
return this;
}
/**
* Specify this field to replace existing subscription plans with new plans.
* @return replaceSubscriptionPlan
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field to replace existing subscription plans with new plans.")
public SubscriptionReplacePlanPatchResponse getReplaceSubscriptionPlan() {
return replaceSubscriptionPlan;
}
public void setReplaceSubscriptionPlan(SubscriptionReplacePlanPatchResponse replaceSubscriptionPlan) {
this.replaceSubscriptionPlan = replaceSubscriptionPlan;
}
public OrderAction renew(SubscriptionRenewPatchResponse renew) {
this.renew = renew;
return this;
}
/**
* Specify this field to renew an existing subscription.
* @return renew
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field to renew an existing subscription.")
public SubscriptionRenewPatchResponse getRenew() {
return renew;
}
public void setRenew(SubscriptionRenewPatchResponse renew) {
this.renew = renew;
}
public OrderAction terms(SubscriptionTermPatchResponse terms) {
this.terms = terms;
return this;
}
/**
* Get terms
* @return terms
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SubscriptionTermPatchResponse getTerms() {
return terms;
}
public void setTerms(SubscriptionTermPatchResponse terms) {
this.terms = terms;
}
public OrderAction cancel(SubscriptionCancelPatchResponse cancel) {
this.cancel = cancel;
return this;
}
/**
* Specify this field to cancel an existing subscription.
* @return cancel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field to cancel an existing subscription.")
public SubscriptionCancelPatchResponse getCancel() {
return cancel;
}
public void setCancel(SubscriptionCancelPatchResponse cancel) {
this.cancel = cancel;
}
public OrderAction pause(SubscriptionPausePatchResponse pause) {
this.pause = pause;
return this;
}
/**
* Specify this field to pause an existing subscription.
* @return pause
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field to pause an existing subscription.")
public SubscriptionPausePatchResponse getPause() {
return pause;
}
public void setPause(SubscriptionPausePatchResponse pause) {
this.pause = pause;
}
public OrderAction resume(SubscriptionResumePatchResponse resume) {
this.resume = resume;
return this;
}
/**
* Specify this field to resume a paused subscription.
* @return resume
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field to resume a paused subscription.")
public SubscriptionResumePatchResponse getResume() {
return resume;
}
public void setResume(SubscriptionResumePatchResponse resume) {
this.resume = resume;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OrderAction orderAction = (OrderAction) o;
return Objects.equals(this.actionId, orderAction.actionId) &&
Objects.equals(this.type, orderAction.type) &&
Objects.equals(this.sequence, orderAction.sequence) &&
Objects.equals(this.startOn, orderAction.startOn) &&
Objects.equals(this.subscriptionPlans, orderAction.subscriptionPlans) &&
Objects.equals(this.addSubscriptionPlan, orderAction.addSubscriptionPlan) &&
Objects.equals(this.removeSubscriptionPlan, orderAction.removeSubscriptionPlan) &&
Objects.equals(this.updateSubscriptionPlan, orderAction.updateSubscriptionPlan) &&
Objects.equals(this.replaceSubscriptionPlan, orderAction.replaceSubscriptionPlan) &&
Objects.equals(this.renew, orderAction.renew) &&
Objects.equals(this.terms, orderAction.terms) &&
Objects.equals(this.cancel, orderAction.cancel) &&
Objects.equals(this.pause, orderAction.pause) &&
Objects.equals(this.resume, orderAction.resume);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(actionId, type, sequence, startOn, subscriptionPlans, addSubscriptionPlan, removeSubscriptionPlan, updateSubscriptionPlan, replaceSubscriptionPlan, renew, terms, cancel, pause, resume);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OrderAction {\n");
sb.append(" actionId: ").append(toIndentedString(actionId)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" sequence: ").append(toIndentedString(sequence)).append("\n");
sb.append(" startOn: ").append(toIndentedString(startOn)).append("\n");
sb.append(" subscriptionPlans: ").append(toIndentedString(subscriptionPlans)).append("\n");
sb.append(" addSubscriptionPlan: ").append(toIndentedString(addSubscriptionPlan)).append("\n");
sb.append(" removeSubscriptionPlan: ").append(toIndentedString(removeSubscriptionPlan)).append("\n");
sb.append(" updateSubscriptionPlan: ").append(toIndentedString(updateSubscriptionPlan)).append("\n");
sb.append(" replaceSubscriptionPlan: ").append(toIndentedString(replaceSubscriptionPlan)).append("\n");
sb.append(" renew: ").append(toIndentedString(renew)).append("\n");
sb.append(" terms: ").append(toIndentedString(terms)).append("\n");
sb.append(" cancel: ").append(toIndentedString(cancel)).append("\n");
sb.append(" pause: ").append(toIndentedString(pause)).append("\n");
sb.append(" resume: ").append(toIndentedString(resume)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy