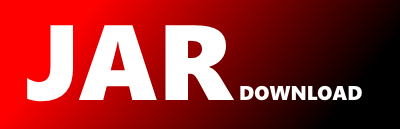
org.openapitools.client.model.OrderCancelResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.Account;
import org.openapitools.client.model.ArTransactionsOrders;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.LineItemListResponse;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.PostSubscriptionOrderResponse;
import org.openapitools.client.model.WriteOff;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* OrderCancelResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class OrderCancelResponse {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_ORDER_NUMBER = "order_number";
@SerializedName(SERIALIZED_NAME_ORDER_NUMBER)
private String orderNumber;
public static final String SERIALIZED_NAME_ORDER_DATE = "order_date";
@SerializedName(SERIALIZED_NAME_ORDER_DATE)
private LocalDate orderDate;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
/**
* Category of the order to indicate a product sale or return. Default value is `sale`.
*/
@JsonAdapter(CategoryEnum.Adapter.class)
public enum CategoryEnum {
SALE("sale"),
RETURN("return"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
CategoryEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static CategoryEnum fromValue(String value) {
for (CategoryEnum b : CategoryEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final CategoryEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public CategoryEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return CategoryEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_CATEGORY = "category";
@SerializedName(SERIALIZED_NAME_CATEGORY)
private CategoryEnum category;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private Account account;
public static final String SERIALIZED_NAME_LINE_ITEMS = "line_items";
@SerializedName(SERIALIZED_NAME_LINE_ITEMS)
private List lineItems = null;
public static final String SERIALIZED_NAME_SUBSCRIPTIONS = "subscriptions";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTIONS)
private List subscriptions = null;
/**
* The status of the order.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
PENDING("pending"),
COMPLETE("complete"),
DRAFT("draft"),
CANCELED("canceled"),
SCHEDULED("scheduled"),
EXECUTING("executing"),
FAILED("failed"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_SCHEDULED_DATE = "scheduled_date";
@SerializedName(SERIALIZED_NAME_SCHEDULED_DATE)
private LocalDate scheduledDate;
public static final String SERIALIZED_NAME_SCHEDULED_DATE_POLICY = "scheduled_date_policy";
@SerializedName(SERIALIZED_NAME_SCHEDULED_DATE_POLICY)
private String scheduledDatePolicy;
public static final String SERIALIZED_NAME_AR_TRANSACTIONS = "ar_transactions";
@SerializedName(SERIALIZED_NAME_AR_TRANSACTIONS)
private ArTransactionsOrders arTransactions;
public static final String SERIALIZED_NAME_WRITE_OFFS = "write_offs";
@SerializedName(SERIALIZED_NAME_WRITE_OFFS)
private List writeOffs = null;
public OrderCancelResponse() {
}
public OrderCancelResponse(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public OrderCancelResponse customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public OrderCancelResponse putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public OrderCancelResponse orderNumber(String orderNumber) {
this.orderNumber = orderNumber;
return this;
}
/**
* The order number of the new order. If not provided, system will auto-generate a number for this order. Note: Ensure that the order number does not contain a slash.
* @return orderNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The order number of the new order. If not provided, system will auto-generate a number for this order. Note: Ensure that the order number does not contain a slash.")
public String getOrderNumber() {
return orderNumber;
}
public void setOrderNumber(String orderNumber) {
this.orderNumber = orderNumber;
}
public OrderCancelResponse orderDate(LocalDate orderDate) {
this.orderDate = orderDate;
return this;
}
/**
* The date when the order is signed. All the order actions under this order will use this order date as the contract effective date if the contract effective date field is skipped or its value is left as null.
* @return orderDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "The date when the order is signed. All the order actions under this order will use this order date as the contract effective date if the contract effective date field is skipped or its value is left as null.")
public LocalDate getOrderDate() {
return orderDate;
}
public void setOrderDate(LocalDate orderDate) {
this.orderDate = orderDate;
}
public OrderCancelResponse description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "description of test account", value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public OrderCancelResponse category(CategoryEnum category) {
this.category = category;
return this;
}
/**
* Category of the order to indicate a product sale or return. Default value is `sale`.
* @return category
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Category of the order to indicate a product sale or return. Default value is `sale`.")
public CategoryEnum getCategory() {
return category;
}
public void setCategory(CategoryEnum category) {
this.category = category;
}
public OrderCancelResponse accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the account associated with this subscription.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account associated with this subscription.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public OrderCancelResponse account(Account account) {
this.account = account;
return this;
}
/**
* Information of the new account associated with the subscription.
* @return account
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Information of the new account associated with the subscription.")
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
public OrderCancelResponse lineItems(List lineItems) {
this.lineItems = lineItems;
return this;
}
public OrderCancelResponse addLineItemsItem(LineItemListResponse lineItemsItem) {
if (this.lineItems == null) {
this.lineItems = new ArrayList();
}
this.lineItems.add(lineItemsItem);
return this;
}
/**
* Order line items are non-subscription-based items created by an order, representing transactional charges such as one-time fees, physical goods, or professional service charges that are not sold as subscription services. By specifying this field, you can launch non-subscription and unified monetization business models in Zuora, in addition to subscription business models.
* @return lineItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Order line items are non-subscription-based items created by an order, representing transactional charges such as one-time fees, physical goods, or professional service charges that are not sold as subscription services. By specifying this field, you can launch non-subscription and unified monetization business models in Zuora, in addition to subscription business models.")
public List getLineItems() {
return lineItems;
}
public void setLineItems(List lineItems) {
this.lineItems = lineItems;
}
public OrderCancelResponse subscriptions(List subscriptions) {
this.subscriptions = subscriptions;
return this;
}
public OrderCancelResponse addSubscriptionsItem(PostSubscriptionOrderResponse subscriptionsItem) {
if (this.subscriptions == null) {
this.subscriptions = new ArrayList();
}
this.subscriptions.add(subscriptionsItem);
return this;
}
/**
* Each item includes specific fields based on the intended order action.
* @return subscriptions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Each item includes specific fields based on the intended order action.")
public List getSubscriptions() {
return subscriptions;
}
public void setSubscriptions(List subscriptions) {
this.subscriptions = subscriptions;
}
public OrderCancelResponse state(StateEnum state) {
this.state = state;
return this;
}
/**
* The status of the order.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The status of the order.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public OrderCancelResponse scheduledDate(LocalDate scheduledDate) {
this.scheduledDate = scheduledDate;
return this;
}
/**
* The date when the order is scheduled to be processed.
* @return scheduledDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date when the order is scheduled to be processed.")
public LocalDate getScheduledDate() {
return scheduledDate;
}
public void setScheduledDate(LocalDate scheduledDate) {
this.scheduledDate = scheduledDate;
}
public OrderCancelResponse scheduledDatePolicy(String scheduledDatePolicy) {
this.scheduledDatePolicy = scheduledDatePolicy;
return this;
}
/**
* Date policy of the scheduled order.
* @return scheduledDatePolicy
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Date policy of the scheduled order.")
public String getScheduledDatePolicy() {
return scheduledDatePolicy;
}
public void setScheduledDatePolicy(String scheduledDatePolicy) {
this.scheduledDatePolicy = scheduledDatePolicy;
}
public OrderCancelResponse arTransactions(ArTransactionsOrders arTransactions) {
this.arTransactions = arTransactions;
return this;
}
/**
* Get arTransactions
* @return arTransactions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ArTransactionsOrders getArTransactions() {
return arTransactions;
}
public void setArTransactions(ArTransactionsOrders arTransactions) {
this.arTransactions = arTransactions;
}
public OrderCancelResponse writeOffs(List writeOffs) {
this.writeOffs = writeOffs;
return this;
}
public OrderCancelResponse addWriteOffsItem(WriteOff writeOffsItem) {
if (this.writeOffs == null) {
this.writeOffs = new ArrayList();
}
this.writeOffs.add(writeOffsItem);
return this;
}
/**
* The billing documents that are written off.
* @return writeOffs
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing documents that are written off.")
public List getWriteOffs() {
return writeOffs;
}
public void setWriteOffs(List writeOffs) {
this.writeOffs = writeOffs;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OrderCancelResponse orderCancelResponse = (OrderCancelResponse) o;
return Objects.equals(this.id, orderCancelResponse.id) &&
Objects.equals(this.updatedById, orderCancelResponse.updatedById) &&
Objects.equals(this.updatedTime, orderCancelResponse.updatedTime) &&
Objects.equals(this.createdById, orderCancelResponse.createdById) &&
Objects.equals(this.createdTime, orderCancelResponse.createdTime) &&
Objects.equals(this.customFields, orderCancelResponse.customFields) &&
Objects.equals(this.customObjects, orderCancelResponse.customObjects) &&
Objects.equals(this.orderNumber, orderCancelResponse.orderNumber) &&
Objects.equals(this.orderDate, orderCancelResponse.orderDate) &&
Objects.equals(this.description, orderCancelResponse.description) &&
Objects.equals(this.category, orderCancelResponse.category) &&
Objects.equals(this.accountId, orderCancelResponse.accountId) &&
Objects.equals(this.account, orderCancelResponse.account) &&
Objects.equals(this.lineItems, orderCancelResponse.lineItems) &&
Objects.equals(this.subscriptions, orderCancelResponse.subscriptions) &&
Objects.equals(this.state, orderCancelResponse.state) &&
Objects.equals(this.scheduledDate, orderCancelResponse.scheduledDate) &&
Objects.equals(this.scheduledDatePolicy, orderCancelResponse.scheduledDatePolicy) &&
Objects.equals(this.arTransactions, orderCancelResponse.arTransactions) &&
Objects.equals(this.writeOffs, orderCancelResponse.writeOffs);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, orderNumber, orderDate, description, category, accountId, account, lineItems, subscriptions, state, scheduledDate, scheduledDatePolicy, arTransactions, writeOffs);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OrderCancelResponse {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" orderNumber: ").append(toIndentedString(orderNumber)).append("\n");
sb.append(" orderDate: ").append(toIndentedString(orderDate)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" lineItems: ").append(toIndentedString(lineItems)).append("\n");
sb.append(" subscriptions: ").append(toIndentedString(subscriptions)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" scheduledDate: ").append(toIndentedString(scheduledDate)).append("\n");
sb.append(" scheduledDatePolicy: ").append(toIndentedString(scheduledDatePolicy)).append("\n");
sb.append(" arTransactions: ").append(toIndentedString(arTransactions)).append("\n");
sb.append(" writeOffs: ").append(toIndentedString(writeOffs)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy