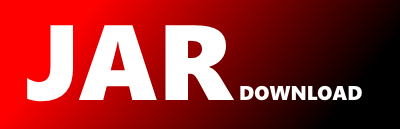
org.openapitools.client.model.OrderLineItemRevenue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.openapitools.jackson.nullable.JsonNullable;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Accounting configuration if you have Zuora Revenue enabled.
*/
@ApiModel(description = "Accounting configuration if you have Zuora Revenue enabled.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class OrderLineItemRevenue {
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "exclude_item_billing_from_revenue_accounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING = "exclude_item_booking_from_revenue_accounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBookingFromRevenueAccounting;
public static final String SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNT = "adjustment_revenue_account";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNT)
private String adjustmentRevenueAccount;
public static final String SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNT = "contract_recognized_revenue_account";
@SerializedName(SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNT)
private String contractRecognizedRevenueAccount;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT = "deferred_revenue_account";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT)
private String deferredRevenueAccount;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT = "recognized_revenue_account";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT)
private String recognizedRevenueAccount;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME = "revenue_recognition_rule_name";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME)
private String revenueRecognitionRuleName;
public OrderLineItemRevenue() {
}
public OrderLineItemRevenue excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* If set to `true`, any associated billing document items are excluded from the revenue accounting.
* @return excludeItemBillingFromRevenueAccounting
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If set to `true`, any associated billing document items are excluded from the revenue accounting.")
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public OrderLineItemRevenue excludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
return this;
}
/**
* If set to `true`, any associated subscription items are excluded from the revenue accounting.
* @return excludeItemBookingFromRevenueAccounting
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If set to `true`, any associated subscription items are excluded from the revenue accounting.")
public Boolean getExcludeItemBookingFromRevenueAccounting() {
return excludeItemBookingFromRevenueAccounting;
}
public void setExcludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
}
public OrderLineItemRevenue adjustmentRevenueAccount(String adjustmentRevenueAccount) {
this.adjustmentRevenueAccount = adjustmentRevenueAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return adjustmentRevenueAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getAdjustmentRevenueAccount() {
return adjustmentRevenueAccount;
}
public void setAdjustmentRevenueAccount(String adjustmentRevenueAccount) {
this.adjustmentRevenueAccount = adjustmentRevenueAccount;
}
public OrderLineItemRevenue contractRecognizedRevenueAccount(String contractRecognizedRevenueAccount) {
this.contractRecognizedRevenueAccount = contractRecognizedRevenueAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return contractRecognizedRevenueAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getContractRecognizedRevenueAccount() {
return contractRecognizedRevenueAccount;
}
public void setContractRecognizedRevenueAccount(String contractRecognizedRevenueAccount) {
this.contractRecognizedRevenueAccount = contractRecognizedRevenueAccount;
}
public OrderLineItemRevenue deferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return deferredRevenueAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getDeferredRevenueAccount() {
return deferredRevenueAccount;
}
public void setDeferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
}
public OrderLineItemRevenue recognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return recognizedRevenueAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getRecognizedRevenueAccount() {
return recognizedRevenueAccount;
}
public void setRecognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
}
public OrderLineItemRevenue revenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
return this;
}
/**
* The revenue recognition rule for the order line item.
* @return revenueRecognitionRuleName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The revenue recognition rule for the order line item.")
public String getRevenueRecognitionRuleName() {
return revenueRecognitionRuleName;
}
public void setRevenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OrderLineItemRevenue orderLineItemRevenue = (OrderLineItemRevenue) o;
return Objects.equals(this.excludeItemBillingFromRevenueAccounting, orderLineItemRevenue.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.excludeItemBookingFromRevenueAccounting, orderLineItemRevenue.excludeItemBookingFromRevenueAccounting) &&
Objects.equals(this.adjustmentRevenueAccount, orderLineItemRevenue.adjustmentRevenueAccount) &&
Objects.equals(this.contractRecognizedRevenueAccount, orderLineItemRevenue.contractRecognizedRevenueAccount) &&
Objects.equals(this.deferredRevenueAccount, orderLineItemRevenue.deferredRevenueAccount) &&
Objects.equals(this.recognizedRevenueAccount, orderLineItemRevenue.recognizedRevenueAccount) &&
Objects.equals(this.revenueRecognitionRuleName, orderLineItemRevenue.revenueRecognitionRuleName);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(excludeItemBillingFromRevenueAccounting, excludeItemBookingFromRevenueAccounting, adjustmentRevenueAccount, contractRecognizedRevenueAccount, deferredRevenueAccount, recognizedRevenueAccount, revenueRecognitionRuleName);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OrderLineItemRevenue {\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" excludeItemBookingFromRevenueAccounting: ").append(toIndentedString(excludeItemBookingFromRevenueAccounting)).append("\n");
sb.append(" adjustmentRevenueAccount: ").append(toIndentedString(adjustmentRevenueAccount)).append("\n");
sb.append(" contractRecognizedRevenueAccount: ").append(toIndentedString(contractRecognizedRevenueAccount)).append("\n");
sb.append(" deferredRevenueAccount: ").append(toIndentedString(deferredRevenueAccount)).append("\n");
sb.append(" recognizedRevenueAccount: ").append(toIndentedString(recognizedRevenueAccount)).append("\n");
sb.append(" revenueRecognitionRuleName: ").append(toIndentedString(revenueRecognitionRuleName)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy