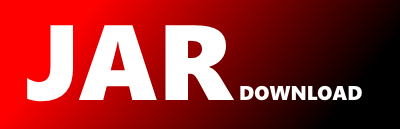
org.openapitools.client.model.OrderPreviewResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.openapitools.client.model.LineItemsPreviewResponse;
import org.openapitools.client.model.OrderSubscriptionPreviewResponse;
import org.openapitools.client.model.SubscriptionPreviewBillingDocumentsResponse;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* OrderPreviewResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class OrderPreviewResponse {
public static final String SERIALIZED_NAME_SUBSCRIPTIONS = "subscriptions";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTIONS)
private List subscriptions = null;
public static final String SERIALIZED_NAME_BILLING_DOCUMENTS = "billing_documents";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENTS)
private List billingDocuments = null;
public static final String SERIALIZED_NAME_LINE_ITEMS = "line_items";
@SerializedName(SERIALIZED_NAME_LINE_ITEMS)
private List lineItems = null;
public OrderPreviewResponse() {
}
public OrderPreviewResponse subscriptions(List subscriptions) {
this.subscriptions = subscriptions;
return this;
}
public OrderPreviewResponse addSubscriptionsItem(OrderSubscriptionPreviewResponse subscriptionsItem) {
if (this.subscriptions == null) {
this.subscriptions = new ArrayList();
}
this.subscriptions.add(subscriptionsItem);
return this;
}
/**
* Get subscriptions
* @return subscriptions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getSubscriptions() {
return subscriptions;
}
public void setSubscriptions(List subscriptions) {
this.subscriptions = subscriptions;
}
public OrderPreviewResponse billingDocuments(List billingDocuments) {
this.billingDocuments = billingDocuments;
return this;
}
public OrderPreviewResponse addBillingDocumentsItem(SubscriptionPreviewBillingDocumentsResponse billingDocumentsItem) {
if (this.billingDocuments == null) {
this.billingDocuments = new ArrayList();
}
this.billingDocuments.add(billingDocumentsItem);
return this;
}
/**
* Get billingDocuments
* @return billingDocuments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getBillingDocuments() {
return billingDocuments;
}
public void setBillingDocuments(List billingDocuments) {
this.billingDocuments = billingDocuments;
}
public OrderPreviewResponse lineItems(List lineItems) {
this.lineItems = lineItems;
return this;
}
public OrderPreviewResponse addLineItemsItem(LineItemsPreviewResponse lineItemsItem) {
if (this.lineItems == null) {
this.lineItems = new ArrayList();
}
this.lineItems.add(lineItemsItem);
return this;
}
/**
* The order line items on this order.
* @return lineItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The order line items on this order.")
public List getLineItems() {
return lineItems;
}
public void setLineItems(List lineItems) {
this.lineItems = lineItems;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OrderPreviewResponse orderPreviewResponse = (OrderPreviewResponse) o;
return Objects.equals(this.subscriptions, orderPreviewResponse.subscriptions) &&
Objects.equals(this.billingDocuments, orderPreviewResponse.billingDocuments) &&
Objects.equals(this.lineItems, orderPreviewResponse.lineItems);
}
@Override
public int hashCode() {
return Objects.hash(subscriptions, billingDocuments, lineItems);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OrderPreviewResponse {\n");
sb.append(" subscriptions: ").append(toIndentedString(subscriptions)).append("\n");
sb.append(" billingDocuments: ").append(toIndentedString(billingDocuments)).append("\n");
sb.append(" lineItems: ").append(toIndentedString(lineItems)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy