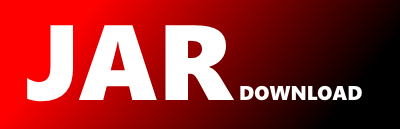
org.openapitools.client.model.OrdersProcessingOption Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Processing options for the invoice or payment.
*/
@ApiModel(description = "Processing options for the invoice or payment.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class OrdersProcessingOption {
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "document_date";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
public static final String SERIALIZED_NAME_TARGET_DATE = "target_date";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
/**
* Specifies whether to just create an invoice, or to create an invoice and collect payment.
*/
@JsonAdapter(CollectionMethodEnum.Adapter.class)
public enum CollectionMethodEnum {
COLLECT_PAYMENT("collect_payment"),
CREATE_INVOICE("create_invoice"),
PROCESS_REFUND("process_refund"),
RUN_BILLING("run_billing"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
CollectionMethodEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static CollectionMethodEnum fromValue(String value) {
for (CollectionMethodEnum b : CollectionMethodEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final CollectionMethodEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public CollectionMethodEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return CollectionMethodEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_COLLECTION_METHOD = "collection_method";
@SerializedName(SERIALIZED_NAME_COLLECTION_METHOD)
private CollectionMethodEnum collectionMethod;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "payment_method_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_ID = "payment_gateway_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_ID)
private String paymentGatewayId;
public static final String SERIALIZED_NAME_DRAFT_INVOICE = "draft_invoice";
@SerializedName(SERIALIZED_NAME_DRAFT_INVOICE)
private Boolean draftInvoice;
public static final String SERIALIZED_NAME_REFUND_REASON_CODE = "refund_reason_code";
@SerializedName(SERIALIZED_NAME_REFUND_REASON_CODE)
private String refundReasonCode;
public static final String SERIALIZED_NAME_APPLY_CREDIT = "apply_credit";
@SerializedName(SERIALIZED_NAME_APPLY_CREDIT)
private Boolean applyCredit;
public OrdersProcessingOption() {
}
public OrdersProcessingOption documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* The date printed on billing documents.
* @return documentDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date printed on billing documents.")
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public OrdersProcessingOption targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date for the order to be picked up by bill run for billing.
* @return targetDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The target date for the order to be picked up by bill run for billing.")
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public OrdersProcessingOption collectionMethod(CollectionMethodEnum collectionMethod) {
this.collectionMethod = collectionMethod;
return this;
}
/**
* Specifies whether to just create an invoice, or to create an invoice and collect payment.
* @return collectionMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies whether to just create an invoice, or to create an invoice and collect payment.")
public CollectionMethodEnum getCollectionMethod() {
return collectionMethod;
}
public void setCollectionMethod(CollectionMethodEnum collectionMethod) {
this.collectionMethod = collectionMethod;
}
public OrdersProcessingOption paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* Payment method Id used to pay billing documents.
* @return paymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Payment method Id used to pay billing documents.")
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public OrdersProcessingOption paymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
return this;
}
/**
* Identifier of the payment gateway Zuora will use to authorize the payments that are made with this payment method.
* @return paymentGatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad093d07ae636bb017ae97518762aa3", value = "Identifier of the payment gateway Zuora will use to authorize the payments that are made with this payment method.")
public String getPaymentGatewayId() {
return paymentGatewayId;
}
public void setPaymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
}
public OrdersProcessingOption draftInvoice(Boolean draftInvoice) {
this.draftInvoice = draftInvoice;
return this;
}
/**
* Get draftInvoice
* @return draftInvoice
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getDraftInvoice() {
return draftInvoice;
}
public void setDraftInvoice(Boolean draftInvoice) {
this.draftInvoice = draftInvoice;
}
public OrdersProcessingOption refundReasonCode(String refundReasonCode) {
this.refundReasonCode = refundReasonCode;
return this;
}
/**
* Get refundReasonCode
* @return refundReasonCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getRefundReasonCode() {
return refundReasonCode;
}
public void setRefundReasonCode(String refundReasonCode) {
this.refundReasonCode = refundReasonCode;
}
public OrdersProcessingOption applyCredit(Boolean applyCredit) {
this.applyCredit = applyCredit;
return this;
}
/**
* Whether to automatically apply credit memos or unapplied payments, or both to an invoice.
* @return applyCredit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether to automatically apply credit memos or unapplied payments, or both to an invoice.")
public Boolean getApplyCredit() {
return applyCredit;
}
public void setApplyCredit(Boolean applyCredit) {
this.applyCredit = applyCredit;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OrdersProcessingOption ordersProcessingOption = (OrdersProcessingOption) o;
return Objects.equals(this.documentDate, ordersProcessingOption.documentDate) &&
Objects.equals(this.targetDate, ordersProcessingOption.targetDate) &&
Objects.equals(this.collectionMethod, ordersProcessingOption.collectionMethod) &&
Objects.equals(this.paymentMethodId, ordersProcessingOption.paymentMethodId) &&
Objects.equals(this.paymentGatewayId, ordersProcessingOption.paymentGatewayId) &&
Objects.equals(this.draftInvoice, ordersProcessingOption.draftInvoice) &&
Objects.equals(this.refundReasonCode, ordersProcessingOption.refundReasonCode) &&
Objects.equals(this.applyCredit, ordersProcessingOption.applyCredit);
}
@Override
public int hashCode() {
return Objects.hash(documentDate, targetDate, collectionMethod, paymentMethodId, paymentGatewayId, draftInvoice, refundReasonCode, applyCredit);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OrdersProcessingOption {\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" collectionMethod: ").append(toIndentedString(collectionMethod)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" paymentGatewayId: ").append(toIndentedString(paymentGatewayId)).append("\n");
sb.append(" draftInvoice: ").append(toIndentedString(draftInvoice)).append("\n");
sb.append(" refundReasonCode: ").append(toIndentedString(refundReasonCode)).append("\n");
sb.append(" applyCredit: ").append(toIndentedString(applyCredit)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy