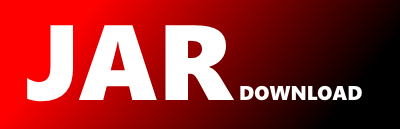
org.openapitools.client.model.PayInvoiceRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PayInvoiceRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PayInvoiceRequest {
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_AUTHORIZATION_ID = "authorization_id";
@SerializedName(SERIALIZED_NAME_AUTHORIZATION_ID)
private String authorizationId;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_BANK_ACCOUNT_ACCOUNT = "bank_account_account";
@SerializedName(SERIALIZED_NAME_BANK_ACCOUNT_ACCOUNT)
private String bankAccountAccount;
public static final String SERIALIZED_NAME_PAYMENT_DATE = "payment_date";
@SerializedName(SERIALIZED_NAME_PAYMENT_DATE)
private LocalDate paymentDate;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "payment_method_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gateway_id";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public static final String SERIALIZED_NAME_GATEWAY_ORDER_ID = "gateway_order_id";
@SerializedName(SERIALIZED_NAME_GATEWAY_ORDER_ID)
private String gatewayOrderId;
public static final String SERIALIZED_NAME_REFERENCE_ID = "reference_id";
@SerializedName(SERIALIZED_NAME_REFERENCE_ID)
private String referenceId;
public static final String SERIALIZED_NAME_GATEWAY_OPTIONS = "gateway_options";
@SerializedName(SERIALIZED_NAME_GATEWAY_OPTIONS)
private Map gatewayOptions = null;
public static final String SERIALIZED_NAME_STATEMENT_DESCRIPTOR = "statement_descriptor";
@SerializedName(SERIALIZED_NAME_STATEMENT_DESCRIPTOR)
private String statementDescriptor;
public static final String SERIALIZED_NAME_STATEMENT_DESCRIPTOR_PHONE = "statement_descriptor_phone";
@SerializedName(SERIALIZED_NAME_STATEMENT_DESCRIPTOR_PHONE)
private String statementDescriptorPhone;
public static final String SERIALIZED_NAME_EXTERNAL = "external";
@SerializedName(SERIALIZED_NAME_EXTERNAL)
private Boolean external;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public PayInvoiceRequest() {
}
public PayInvoiceRequest amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount of the payment.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of the payment.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public PayInvoiceRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the account that owns the invoice. Either `account_id` or `account_number` is required.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the invoice. Either `account_id` or `account_number` is required.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public PayInvoiceRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Human-readable identifier of the account that owns the invoice. Either `account_number` or `account_id` is required.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the account that owns the invoice. Either `account_number` or `account_id` is required.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public PayInvoiceRequest authorizationId(String authorizationId) {
this.authorizationId = authorizationId;
return this;
}
/**
* Identifier of the authorization transaction from the payment gateway.
* @return authorizationId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the authorization transaction from the payment gateway.")
public String getAuthorizationId() {
return authorizationId;
}
public void setAuthorizationId(String authorizationId) {
this.authorizationId = authorizationId;
}
public PayInvoiceRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public PayInvoiceRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* 3-letter ISO 4217 currency code.
* @return currency
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "3-letter ISO 4217 currency code.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PayInvoiceRequest bankAccountAccount(String bankAccountAccount) {
this.bankAccountAccount = bankAccountAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return bankAccountAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getBankAccountAccount() {
return bankAccountAccount;
}
public void setBankAccountAccount(String bankAccountAccount) {
this.bankAccountAccount = bankAccountAccount;
}
public PayInvoiceRequest paymentDate(LocalDate paymentDate) {
this.paymentDate = paymentDate;
return this;
}
/**
* The date and time when the payment takes effect.
* @return paymentDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the payment takes effect.")
public LocalDate getPaymentDate() {
return paymentDate;
}
public void setPaymentDate(LocalDate paymentDate) {
this.paymentDate = paymentDate;
}
public PayInvoiceRequest paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* Identifier of the payment method used to create this payment.
* @return paymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payment method used to create this payment.")
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public PayInvoiceRequest gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* Identifier of the payment gateway that Zuora will use to authorize this payment.
* @return gatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payment gateway that Zuora will use to authorize this payment.")
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
public PayInvoiceRequest gatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
return this;
}
/**
* A merchant-specified natural key value that can be passed to the payment gateway when a payment is created. If not specified, the payment number will be passed in instead.
* @return gatewayOrderId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A merchant-specified natural key value that can be passed to the payment gateway when a payment is created. If not specified, the payment number will be passed in instead.")
public String getGatewayOrderId() {
return gatewayOrderId;
}
public void setGatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
}
public PayInvoiceRequest referenceId(String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* A second transaction identifier returned by the payment gateway if there is an additional transaction for the refunds. You may use this field to reconcile payments between your payment gateway and Zuora Payments.
* @return referenceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A second transaction identifier returned by the payment gateway if there is an additional transaction for the refunds. You may use this field to reconcile payments between your payment gateway and Zuora Payments.")
public String getReferenceId() {
return referenceId;
}
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
public PayInvoiceRequest gatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
return this;
}
public PayInvoiceRequest putGatewayOptionsItem(String key, String gatewayOptionsItem) {
if (this.gatewayOptions == null) {
this.gatewayOptions = new HashMap();
}
this.gatewayOptions.put(key, gatewayOptionsItem);
return this;
}
/**
* Get gatewayOptions
* @return gatewayOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"key\":\"value\"}", value = "")
public Map getGatewayOptions() {
return gatewayOptions;
}
public void setGatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
}
public PayInvoiceRequest statementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
return this;
}
/**
* A payment gateway-specific field used by Orbital, Vantiv and Verifi.
* @return statementDescriptor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A payment gateway-specific field used by Orbital, Vantiv and Verifi.")
public String getStatementDescriptor() {
return statementDescriptor;
}
public void setStatementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
public PayInvoiceRequest statementDescriptorPhone(String statementDescriptorPhone) {
this.statementDescriptorPhone = statementDescriptorPhone;
return this;
}
/**
* A payment gateway-specific field used by Orbital, Vantiv and Verifi.
* @return statementDescriptorPhone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A payment gateway-specific field used by Orbital, Vantiv and Verifi.")
public String getStatementDescriptorPhone() {
return statementDescriptorPhone;
}
public void setStatementDescriptorPhone(String statementDescriptorPhone) {
this.statementDescriptorPhone = statementDescriptorPhone;
}
public PayInvoiceRequest external(Boolean external) {
this.external = external;
return this;
}
/**
* If true, indicates that this payment is not handled by Zuora.
* @return external
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, indicates that this payment is not handled by Zuora.")
public Boolean getExternal() {
return external;
}
public void setExternal(Boolean external) {
this.external = external;
}
public PayInvoiceRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public PayInvoiceRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PayInvoiceRequest payInvoiceRequest = (PayInvoiceRequest) o;
return Objects.equals(this.amount, payInvoiceRequest.amount) &&
Objects.equals(this.accountId, payInvoiceRequest.accountId) &&
Objects.equals(this.accountNumber, payInvoiceRequest.accountNumber) &&
Objects.equals(this.authorizationId, payInvoiceRequest.authorizationId) &&
Objects.equals(this.description, payInvoiceRequest.description) &&
Objects.equals(this.currency, payInvoiceRequest.currency) &&
Objects.equals(this.bankAccountAccount, payInvoiceRequest.bankAccountAccount) &&
Objects.equals(this.paymentDate, payInvoiceRequest.paymentDate) &&
Objects.equals(this.paymentMethodId, payInvoiceRequest.paymentMethodId) &&
Objects.equals(this.gatewayId, payInvoiceRequest.gatewayId) &&
Objects.equals(this.gatewayOrderId, payInvoiceRequest.gatewayOrderId) &&
Objects.equals(this.referenceId, payInvoiceRequest.referenceId) &&
Objects.equals(this.gatewayOptions, payInvoiceRequest.gatewayOptions) &&
Objects.equals(this.statementDescriptor, payInvoiceRequest.statementDescriptor) &&
Objects.equals(this.statementDescriptorPhone, payInvoiceRequest.statementDescriptorPhone) &&
Objects.equals(this.external, payInvoiceRequest.external) &&
Objects.equals(this.customFields, payInvoiceRequest.customFields);
}
@Override
public int hashCode() {
return Objects.hash(amount, accountId, accountNumber, authorizationId, description, currency, bankAccountAccount, paymentDate, paymentMethodId, gatewayId, gatewayOrderId, referenceId, gatewayOptions, statementDescriptor, statementDescriptorPhone, external, customFields);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PayInvoiceRequest {\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" authorizationId: ").append(toIndentedString(authorizationId)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" bankAccountAccount: ").append(toIndentedString(bankAccountAccount)).append("\n");
sb.append(" paymentDate: ").append(toIndentedString(paymentDate)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append(" gatewayOrderId: ").append(toIndentedString(gatewayOrderId)).append("\n");
sb.append(" referenceId: ").append(toIndentedString(referenceId)).append("\n");
sb.append(" gatewayOptions: ").append(toIndentedString(gatewayOptions)).append("\n");
sb.append(" statementDescriptor: ").append(toIndentedString(statementDescriptor)).append("\n");
sb.append(" statementDescriptorPhone: ").append(toIndentedString(statementDescriptorPhone)).append("\n");
sb.append(" external: ").append(toIndentedString(external)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy