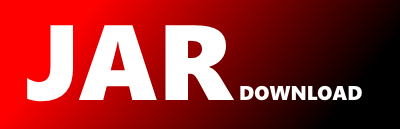
org.openapitools.client.model.Payment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.Account;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.GatewayStateTransitions;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.PaymentMethod;
import org.openapitools.client.model.PaymentScheduleItem;
import org.openapitools.client.model.PaymentStateTransitions;
import org.openapitools.client.model.PaymentsAppliedToResponse;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Payment
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Payment {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_REFERENCE_ID = "reference_id";
@SerializedName(SERIALIZED_NAME_REFERENCE_ID)
private String referenceId;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_AUTHORIZATION_ID = "authorization_id";
@SerializedName(SERIALIZED_NAME_AUTHORIZATION_ID)
private String authorizationId;
public static final String SERIALIZED_NAME_PAYMENT_DATE = "payment_date";
@SerializedName(SERIALIZED_NAME_PAYMENT_DATE)
private LocalDate paymentDate;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "payment_method_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gateway_id";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public static final String SERIALIZED_NAME_GATEWAY_ORDER_ID = "gateway_order_id";
@SerializedName(SERIALIZED_NAME_GATEWAY_ORDER_ID)
private String gatewayOrderId;
public static final String SERIALIZED_NAME_SECOND_REFERENCE_ID = "second_reference_id";
@SerializedName(SERIALIZED_NAME_SECOND_REFERENCE_ID)
private String secondReferenceId;
public static final String SERIALIZED_NAME_GATEWAY_OPTIONS = "gateway_options";
@SerializedName(SERIALIZED_NAME_GATEWAY_OPTIONS)
private Map gatewayOptions = null;
public static final String SERIALIZED_NAME_STATEMENT_DESCRIPTOR = "statement_descriptor";
@SerializedName(SERIALIZED_NAME_STATEMENT_DESCRIPTOR)
private String statementDescriptor;
public static final String SERIALIZED_NAME_STATEMENT_DESCRIPTOR_PHONE = "statement_descriptor_phone";
@SerializedName(SERIALIZED_NAME_STATEMENT_DESCRIPTOR_PHONE)
private String statementDescriptorPhone;
public static final String SERIALIZED_NAME_EXTERNAL = "external";
@SerializedName(SERIALIZED_NAME_EXTERNAL)
private Boolean external;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private Account account;
public static final String SERIALIZED_NAME_AMOUNT_APPLIED = "amount_applied";
@SerializedName(SERIALIZED_NAME_AMOUNT_APPLIED)
private BigDecimal amountApplied;
public static final String SERIALIZED_NAME_REMAINING_BALANCE = "remaining_balance";
@SerializedName(SERIALIZED_NAME_REMAINING_BALANCE)
private BigDecimal remainingBalance;
public static final String SERIALIZED_NAME_AMOUNT_REFUNDED = "amount_refunded";
@SerializedName(SERIALIZED_NAME_AMOUNT_REFUNDED)
private BigDecimal amountRefunded;
/**
* The state of the payment.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
DRAFT("draft"),
POSTED("posted"),
PROCESSING("processing"),
PROCESSED("processed"),
ERROR("error"),
CANCELED("canceled"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_PAYOUT_ID = "payout_id";
@SerializedName(SERIALIZED_NAME_PAYOUT_ID)
private String payoutId;
public static final String SERIALIZED_NAME_PAYMENT_NUMBER = "payment_number";
@SerializedName(SERIALIZED_NAME_PAYMENT_NUMBER)
private String paymentNumber;
public static final String SERIALIZED_NAME_GATEWAY_RESPONSE_CODE = "gateway_response_code";
@SerializedName(SERIALIZED_NAME_GATEWAY_RESPONSE_CODE)
private String gatewayResponseCode;
public static final String SERIALIZED_NAME_PAYMENT_METHOD = "payment_method";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD)
private PaymentMethod paymentMethod;
public static final String SERIALIZED_NAME_GATEWAY_RESPONSE = "gateway_response";
@SerializedName(SERIALIZED_NAME_GATEWAY_RESPONSE)
private String gatewayResponse;
public static final String SERIALIZED_NAME_GATEWAY_RECONCILIATION_STATUS = "gateway_reconciliation_status";
@SerializedName(SERIALIZED_NAME_GATEWAY_RECONCILIATION_STATUS)
private String gatewayReconciliationStatus;
public static final String SERIALIZED_NAME_GATEWAY_RECONCILIATION_REASON = "gateway_reconciliation_reason";
@SerializedName(SERIALIZED_NAME_GATEWAY_RECONCILIATION_REASON)
private String gatewayReconciliationReason;
/**
* The payment gateway state of the payment.
*/
@JsonAdapter(GatewayStateEnum.Adapter.class)
public enum GatewayStateEnum {
MARKED_FOR_SUBMISSION("marked_for_submission"),
SUBMITTED("submitted"),
SETTLED("settled"),
NOT_SUBMITTED("not_submitted"),
FAILED("failed"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
GatewayStateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static GatewayStateEnum fromValue(String value) {
for (GatewayStateEnum b : GatewayStateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final GatewayStateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public GatewayStateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return GatewayStateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_GATEWAY_STATE = "gateway_state";
@SerializedName(SERIALIZED_NAME_GATEWAY_STATE)
private GatewayStateEnum gatewayState;
public static final String SERIALIZED_NAME_APPLIED_TO = "applied_to";
@SerializedName(SERIALIZED_NAME_APPLIED_TO)
private List appliedTo = null;
public static final String SERIALIZED_NAME_PAYMENT_SCHEDULE_ITEMS = "payment_schedule_items";
@SerializedName(SERIALIZED_NAME_PAYMENT_SCHEDULE_ITEMS)
private List paymentScheduleItems = null;
public static final String SERIALIZED_NAME_STATE_TRANSITIONS = "state_transitions";
@SerializedName(SERIALIZED_NAME_STATE_TRANSITIONS)
private PaymentStateTransitions stateTransitions;
public static final String SERIALIZED_NAME_GATEWAY_STATE_TRANSITIONS = "gateway_state_transitions";
@SerializedName(SERIALIZED_NAME_GATEWAY_STATE_TRANSITIONS)
private GatewayStateTransitions gatewayStateTransitions;
public Payment() {
}
public Payment(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects,
StateEnum state,
GatewayStateEnum gatewayState,
List appliedTo
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
this.state = state;
this.gatewayState = gatewayState;
this.appliedTo = appliedTo;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public Payment customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public Payment putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public Payment description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Payment referenceId(String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* Transaction identifier returned by the payment gateway. You may use this field to reconcile payments between your payment gateway and Zuora Payments.
* @return referenceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Transaction identifier returned by the payment gateway. You may use this field to reconcile payments between your payment gateway and Zuora Payments.")
public String getReferenceId() {
return referenceId;
}
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
public Payment accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the customer account associated with this payment.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the customer account associated with this payment.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public Payment accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Human-readable identifier of the account associated with this payment. It can be user-supplied.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the account associated with this payment. It can be user-supplied.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public Payment amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The total amount of the payment.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount of the payment.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public Payment authorizationId(String authorizationId) {
this.authorizationId = authorizationId;
return this;
}
/**
* Identifier of the authorization transaction from the payment gateway.
* @return authorizationId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the authorization transaction from the payment gateway.")
public String getAuthorizationId() {
return authorizationId;
}
public void setAuthorizationId(String authorizationId) {
this.authorizationId = authorizationId;
}
public Payment paymentDate(LocalDate paymentDate) {
this.paymentDate = paymentDate;
return this;
}
/**
* The date and time when the payment takes effect.
* @return paymentDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the payment takes effect.")
public LocalDate getPaymentDate() {
return paymentDate;
}
public void setPaymentDate(LocalDate paymentDate) {
this.paymentDate = paymentDate;
}
public Payment paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* Identifier of the payment method used to create this payment.
* @return paymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payment method used to create this payment.")
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public Payment gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* Identifier of the payment gateway that Zuora will use to authorize this payment.
* @return gatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payment gateway that Zuora will use to authorize this payment.")
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
public Payment gatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
return this;
}
/**
* A merchant-specified natural key value that can be passed to the payment gateway when a payment is created. If not specified, the payment number will be passed in instead.
* @return gatewayOrderId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A merchant-specified natural key value that can be passed to the payment gateway when a payment is created. If not specified, the payment number will be passed in instead.")
public String getGatewayOrderId() {
return gatewayOrderId;
}
public void setGatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
}
public Payment secondReferenceId(String secondReferenceId) {
this.secondReferenceId = secondReferenceId;
return this;
}
/**
* A second transaction identifier returned by the payment gateway if there is an additional transaction for the refunds. You may use this field to reconcile payments between your payment gateway and Zuora Payments.
* @return secondReferenceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A second transaction identifier returned by the payment gateway if there is an additional transaction for the refunds. You may use this field to reconcile payments between your payment gateway and Zuora Payments.")
public String getSecondReferenceId() {
return secondReferenceId;
}
public void setSecondReferenceId(String secondReferenceId) {
this.secondReferenceId = secondReferenceId;
}
public Payment gatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
return this;
}
public Payment putGatewayOptionsItem(String key, String gatewayOptionsItem) {
if (this.gatewayOptions == null) {
this.gatewayOptions = new HashMap();
}
this.gatewayOptions.put(key, gatewayOptionsItem);
return this;
}
/**
* Get gatewayOptions
* @return gatewayOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"key\":\"value\"}", value = "")
public Map getGatewayOptions() {
return gatewayOptions;
}
public void setGatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
}
public Payment statementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
return this;
}
/**
* A payment gateway-specific field used by Orbital, Vantiv and Verifi.
* @return statementDescriptor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A payment gateway-specific field used by Orbital, Vantiv and Verifi.")
public String getStatementDescriptor() {
return statementDescriptor;
}
public void setStatementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
public Payment statementDescriptorPhone(String statementDescriptorPhone) {
this.statementDescriptorPhone = statementDescriptorPhone;
return this;
}
/**
* A payment gateway-specific field used by Orbital, Vantiv and Verifi.
* @return statementDescriptorPhone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A payment gateway-specific field used by Orbital, Vantiv and Verifi.")
public String getStatementDescriptorPhone() {
return statementDescriptorPhone;
}
public void setStatementDescriptorPhone(String statementDescriptorPhone) {
this.statementDescriptorPhone = statementDescriptorPhone;
}
public Payment external(Boolean external) {
this.external = external;
return this;
}
/**
* If true, indicates that this payment is not handled by Zuora.
* @return external
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, indicates that this payment is not handled by Zuora.")
public Boolean getExternal() {
return external;
}
public void setExternal(Boolean external) {
this.external = external;
}
public Payment currency(String currency) {
this.currency = currency;
return this;
}
/**
* 3-letter ISO 4217 currency code.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "3-letter ISO 4217 currency code.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public Payment account(Account account) {
this.account = account;
return this;
}
/**
* The customer account associated with this payment.
* @return account
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The customer account associated with this payment.")
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
public Payment amountApplied(BigDecimal amountApplied) {
this.amountApplied = amountApplied;
return this;
}
/**
* The total amount applied.
* @return amountApplied
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount applied.")
public BigDecimal getAmountApplied() {
return amountApplied;
}
public void setAmountApplied(BigDecimal amountApplied) {
this.amountApplied = amountApplied;
}
public Payment remainingBalance(BigDecimal remainingBalance) {
this.remainingBalance = remainingBalance;
return this;
}
/**
* The total remaining balance.
* @return remainingBalance
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total remaining balance.")
public BigDecimal getRemainingBalance() {
return remainingBalance;
}
public void setRemainingBalance(BigDecimal remainingBalance) {
this.remainingBalance = remainingBalance;
}
public Payment amountRefunded(BigDecimal amountRefunded) {
this.amountRefunded = amountRefunded;
return this;
}
/**
* The total amount refunded.
* @return amountRefunded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount refunded.")
public BigDecimal getAmountRefunded() {
return amountRefunded;
}
public void setAmountRefunded(BigDecimal amountRefunded) {
this.amountRefunded = amountRefunded;
}
/**
* The state of the payment.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The state of the payment.")
public StateEnum getState() {
return state;
}
public Payment payoutId(String payoutId) {
this.payoutId = payoutId;
return this;
}
/**
* Identifier of the payout associated with this payment.
* @return payoutId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payout associated with this payment.")
public String getPayoutId() {
return payoutId;
}
public void setPayoutId(String payoutId) {
this.payoutId = payoutId;
}
public Payment paymentNumber(String paymentNumber) {
this.paymentNumber = paymentNumber;
return this;
}
/**
* Human-readable identifier for this object; may be user-supplied.
* @return paymentNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier for this object; may be user-supplied.")
public String getPaymentNumber() {
return paymentNumber;
}
public void setPaymentNumber(String paymentNumber) {
this.paymentNumber = paymentNumber;
}
public Payment gatewayResponseCode(String gatewayResponseCode) {
this.gatewayResponseCode = gatewayResponseCode;
return this;
}
/**
* Code returned by the payment gateway for this payment.
* @return gatewayResponseCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Code returned by the payment gateway for this payment.")
public String getGatewayResponseCode() {
return gatewayResponseCode;
}
public void setGatewayResponseCode(String gatewayResponseCode) {
this.gatewayResponseCode = gatewayResponseCode;
}
public Payment paymentMethod(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* Get paymentMethod
* @return paymentMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PaymentMethod getPaymentMethod() {
return paymentMethod;
}
public void setPaymentMethod(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
public Payment gatewayResponse(String gatewayResponse) {
this.gatewayResponse = gatewayResponse;
return this;
}
/**
* Message returned by the payment gateway for this payment.
* @return gatewayResponse
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Message returned by the payment gateway for this payment.")
public String getGatewayResponse() {
return gatewayResponse;
}
public void setGatewayResponse(String gatewayResponse) {
this.gatewayResponse = gatewayResponse;
}
public Payment gatewayReconciliationStatus(String gatewayReconciliationStatus) {
this.gatewayReconciliationStatus = gatewayReconciliationStatus;
return this;
}
/**
* Gateway reconciliation state.
* @return gatewayReconciliationStatus
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Gateway reconciliation state.")
public String getGatewayReconciliationStatus() {
return gatewayReconciliationStatus;
}
public void setGatewayReconciliationStatus(String gatewayReconciliationStatus) {
this.gatewayReconciliationStatus = gatewayReconciliationStatus;
}
public Payment gatewayReconciliationReason(String gatewayReconciliationReason) {
this.gatewayReconciliationReason = gatewayReconciliationReason;
return this;
}
/**
* Gateway reconciliation reason.
* @return gatewayReconciliationReason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Gateway reconciliation reason.")
public String getGatewayReconciliationReason() {
return gatewayReconciliationReason;
}
public void setGatewayReconciliationReason(String gatewayReconciliationReason) {
this.gatewayReconciliationReason = gatewayReconciliationReason;
}
/**
* The payment gateway state of the payment.
* @return gatewayState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The payment gateway state of the payment.")
public GatewayStateEnum getGatewayState() {
return gatewayState;
}
/**
* Get appliedTo
* @return appliedTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getAppliedTo() {
return appliedTo;
}
public Payment paymentScheduleItems(List paymentScheduleItems) {
this.paymentScheduleItems = paymentScheduleItems;
return this;
}
public Payment addPaymentScheduleItemsItem(PaymentScheduleItem paymentScheduleItemsItem) {
if (this.paymentScheduleItems == null) {
this.paymentScheduleItems = new ArrayList();
}
this.paymentScheduleItems.add(paymentScheduleItemsItem);
return this;
}
/**
* Get paymentScheduleItems
* @return paymentScheduleItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getPaymentScheduleItems() {
return paymentScheduleItems;
}
public void setPaymentScheduleItems(List paymentScheduleItems) {
this.paymentScheduleItems = paymentScheduleItems;
}
public Payment stateTransitions(PaymentStateTransitions stateTransitions) {
this.stateTransitions = stateTransitions;
return this;
}
/**
* Get stateTransitions
* @return stateTransitions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PaymentStateTransitions getStateTransitions() {
return stateTransitions;
}
public void setStateTransitions(PaymentStateTransitions stateTransitions) {
this.stateTransitions = stateTransitions;
}
public Payment gatewayStateTransitions(GatewayStateTransitions gatewayStateTransitions) {
this.gatewayStateTransitions = gatewayStateTransitions;
return this;
}
/**
* Get gatewayStateTransitions
* @return gatewayStateTransitions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public GatewayStateTransitions getGatewayStateTransitions() {
return gatewayStateTransitions;
}
public void setGatewayStateTransitions(GatewayStateTransitions gatewayStateTransitions) {
this.gatewayStateTransitions = gatewayStateTransitions;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Payment payment = (Payment) o;
return Objects.equals(this.id, payment.id) &&
Objects.equals(this.updatedById, payment.updatedById) &&
Objects.equals(this.updatedTime, payment.updatedTime) &&
Objects.equals(this.createdById, payment.createdById) &&
Objects.equals(this.createdTime, payment.createdTime) &&
Objects.equals(this.customFields, payment.customFields) &&
Objects.equals(this.customObjects, payment.customObjects) &&
Objects.equals(this.description, payment.description) &&
Objects.equals(this.referenceId, payment.referenceId) &&
Objects.equals(this.accountId, payment.accountId) &&
Objects.equals(this.accountNumber, payment.accountNumber) &&
Objects.equals(this.amount, payment.amount) &&
Objects.equals(this.authorizationId, payment.authorizationId) &&
Objects.equals(this.paymentDate, payment.paymentDate) &&
Objects.equals(this.paymentMethodId, payment.paymentMethodId) &&
Objects.equals(this.gatewayId, payment.gatewayId) &&
Objects.equals(this.gatewayOrderId, payment.gatewayOrderId) &&
Objects.equals(this.secondReferenceId, payment.secondReferenceId) &&
Objects.equals(this.gatewayOptions, payment.gatewayOptions) &&
Objects.equals(this.statementDescriptor, payment.statementDescriptor) &&
Objects.equals(this.statementDescriptorPhone, payment.statementDescriptorPhone) &&
Objects.equals(this.external, payment.external) &&
Objects.equals(this.currency, payment.currency) &&
Objects.equals(this.account, payment.account) &&
Objects.equals(this.amountApplied, payment.amountApplied) &&
Objects.equals(this.remainingBalance, payment.remainingBalance) &&
Objects.equals(this.amountRefunded, payment.amountRefunded) &&
Objects.equals(this.state, payment.state) &&
Objects.equals(this.payoutId, payment.payoutId) &&
Objects.equals(this.paymentNumber, payment.paymentNumber) &&
Objects.equals(this.gatewayResponseCode, payment.gatewayResponseCode) &&
Objects.equals(this.paymentMethod, payment.paymentMethod) &&
Objects.equals(this.gatewayResponse, payment.gatewayResponse) &&
Objects.equals(this.gatewayReconciliationStatus, payment.gatewayReconciliationStatus) &&
Objects.equals(this.gatewayReconciliationReason, payment.gatewayReconciliationReason) &&
Objects.equals(this.gatewayState, payment.gatewayState) &&
Objects.equals(this.appliedTo, payment.appliedTo) &&
Objects.equals(this.paymentScheduleItems, payment.paymentScheduleItems) &&
Objects.equals(this.stateTransitions, payment.stateTransitions) &&
Objects.equals(this.gatewayStateTransitions, payment.gatewayStateTransitions);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, description, referenceId, accountId, accountNumber, amount, authorizationId, paymentDate, paymentMethodId, gatewayId, gatewayOrderId, secondReferenceId, gatewayOptions, statementDescriptor, statementDescriptorPhone, external, currency, account, amountApplied, remainingBalance, amountRefunded, state, payoutId, paymentNumber, gatewayResponseCode, paymentMethod, gatewayResponse, gatewayReconciliationStatus, gatewayReconciliationReason, gatewayState, appliedTo, paymentScheduleItems, stateTransitions, gatewayStateTransitions);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Payment {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" referenceId: ").append(toIndentedString(referenceId)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" authorizationId: ").append(toIndentedString(authorizationId)).append("\n");
sb.append(" paymentDate: ").append(toIndentedString(paymentDate)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append(" gatewayOrderId: ").append(toIndentedString(gatewayOrderId)).append("\n");
sb.append(" secondReferenceId: ").append(toIndentedString(secondReferenceId)).append("\n");
sb.append(" gatewayOptions: ").append(toIndentedString(gatewayOptions)).append("\n");
sb.append(" statementDescriptor: ").append(toIndentedString(statementDescriptor)).append("\n");
sb.append(" statementDescriptorPhone: ").append(toIndentedString(statementDescriptorPhone)).append("\n");
sb.append(" external: ").append(toIndentedString(external)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" amountApplied: ").append(toIndentedString(amountApplied)).append("\n");
sb.append(" remainingBalance: ").append(toIndentedString(remainingBalance)).append("\n");
sb.append(" amountRefunded: ").append(toIndentedString(amountRefunded)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" payoutId: ").append(toIndentedString(payoutId)).append("\n");
sb.append(" paymentNumber: ").append(toIndentedString(paymentNumber)).append("\n");
sb.append(" gatewayResponseCode: ").append(toIndentedString(gatewayResponseCode)).append("\n");
sb.append(" paymentMethod: ").append(toIndentedString(paymentMethod)).append("\n");
sb.append(" gatewayResponse: ").append(toIndentedString(gatewayResponse)).append("\n");
sb.append(" gatewayReconciliationStatus: ").append(toIndentedString(gatewayReconciliationStatus)).append("\n");
sb.append(" gatewayReconciliationReason: ").append(toIndentedString(gatewayReconciliationReason)).append("\n");
sb.append(" gatewayState: ").append(toIndentedString(gatewayState)).append("\n");
sb.append(" appliedTo: ").append(toIndentedString(appliedTo)).append("\n");
sb.append(" paymentScheduleItems: ").append(toIndentedString(paymentScheduleItems)).append("\n");
sb.append(" stateTransitions: ").append(toIndentedString(stateTransitions)).append("\n");
sb.append(" gatewayStateTransitions: ").append(toIndentedString(gatewayStateTransitions)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy