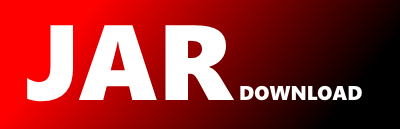
org.openapitools.client.model.PaymentMethod Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.Account;
import org.openapitools.client.model.AchDebit;
import org.openapitools.client.model.ApplePay;
import org.openapitools.client.model.AuBecsDebit;
import org.openapitools.client.model.AutogiroDebit;
import org.openapitools.client.model.BacsDebit;
import org.openapitools.client.model.BetalingsDebit;
import org.openapitools.client.model.BillingDetails;
import org.openapitools.client.model.CcRef;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.GetCardList;
import org.openapitools.client.model.GooglePay;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.NzBecsDebit;
import org.openapitools.client.model.PadDebit;
import org.openapitools.client.model.PaypalAdaptive;
import org.openapitools.client.model.PaypalExpress;
import org.openapitools.client.model.PaypalExpressNative;
import org.openapitools.client.model.SepaDebit;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Payment method information.
*/
@ApiModel(description = "Payment method information.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentMethod {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
/**
* The type of the payment method. An additional hash is included on the payment method with a name matching this value. It contains additional information specific to the payment method type.
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
PAYPAL_EXPRESS("paypal_express"),
PAYPAL_EXPRESS_NATIVE("paypal_express_native"),
PAYPAL_ADAPTIVE("paypal_adaptive"),
CARD("card"),
CC_REF("cc_ref"),
ACH_DEBIT("ach_debit"),
SEPA_DEBIT("sepa_debit"),
BETALINGS_DEBIT("betalings_debit"),
AUTOGIRO_DEBIT("autogiro_debit"),
BACS_DEBIT("bacs_debit"),
AU_BECS_DEBIT("au_becs_debit"),
NZ_BECS_DEBIT("nz_becs_debit"),
PAD_DEBIT("pad_debit"),
APPLE_PAY("apple_pay"),
WIRE_TRANSFER("wire_transfer"),
CHECK("check"),
CASH("cash"),
OTHER("other"),
PAYPAL("paypal"),
ADYEN_GOOGLE_PAY("adyen_google_pay"),
ADYEN_APPLE_PAY("adyen_apple_pay"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TypeEnum type;
public static final String SERIALIZED_NAME_CUSTOM_TYPE = "custom_type";
@SerializedName(SERIALIZED_NAME_CUSTOM_TYPE)
private String customType;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private Account account;
public static final String SERIALIZED_NAME_BILLING_DETAILS = "billing_details";
@SerializedName(SERIALIZED_NAME_BILLING_DETAILS)
private BillingDetails billingDetails;
public static final String SERIALIZED_NAME_MAXIMUM_PAYMENT_ATTEMPTS = "maximum_payment_attempts";
@SerializedName(SERIALIZED_NAME_MAXIMUM_PAYMENT_ATTEMPTS)
private BigDecimal maximumPaymentAttempts;
public static final String SERIALIZED_NAME_PAYMENT_RETRY_INTERVAL = "payment_retry_interval";
@SerializedName(SERIALIZED_NAME_PAYMENT_RETRY_INTERVAL)
private Integer paymentRetryInterval;
public static final String SERIALIZED_NAME_DEVICE_SESSION_ID = "device_session_id";
@SerializedName(SERIALIZED_NAME_DEVICE_SESSION_ID)
private String deviceSessionId;
public static final String SERIALIZED_NAME_IP_ADDRESS = "ip_address";
@SerializedName(SERIALIZED_NAME_IP_ADDRESS)
private String ipAddress;
public static final String SERIALIZED_NAME_BANK_IDENTIFICATION_NUMBER = "bank_identification_number";
@SerializedName(SERIALIZED_NAME_BANK_IDENTIFICATION_NUMBER)
private String bankIdentificationNumber;
public static final String SERIALIZED_NAME_CARD = "card";
@SerializedName(SERIALIZED_NAME_CARD)
private GetCardList card;
public static final String SERIALIZED_NAME_PAYPAL_EXPRESS_NATIVE = "paypal_express_native";
@SerializedName(SERIALIZED_NAME_PAYPAL_EXPRESS_NATIVE)
private PaypalExpressNative paypalExpressNative;
public static final String SERIALIZED_NAME_PAYPAL_EXPRESS = "paypal_express";
@SerializedName(SERIALIZED_NAME_PAYPAL_EXPRESS)
private PaypalExpress paypalExpress;
public static final String SERIALIZED_NAME_PAYPAL_ADAPTIVE = "paypal_adaptive";
@SerializedName(SERIALIZED_NAME_PAYPAL_ADAPTIVE)
private PaypalAdaptive paypalAdaptive;
public static final String SERIALIZED_NAME_SEPA_DEBIT = "sepa_debit";
@SerializedName(SERIALIZED_NAME_SEPA_DEBIT)
private SepaDebit sepaDebit;
public static final String SERIALIZED_NAME_CC_REF = "cc_ref";
@SerializedName(SERIALIZED_NAME_CC_REF)
private CcRef ccRef;
public static final String SERIALIZED_NAME_APPLE_PAY = "apple_pay";
@SerializedName(SERIALIZED_NAME_APPLE_PAY)
private ApplePay applePay;
public static final String SERIALIZED_NAME_GOOGLE_PAY = "google_pay";
@SerializedName(SERIALIZED_NAME_GOOGLE_PAY)
private GooglePay googlePay;
public static final String SERIALIZED_NAME_ACH_DEBIT = "ach_debit";
@SerializedName(SERIALIZED_NAME_ACH_DEBIT)
private AchDebit achDebit;
public static final String SERIALIZED_NAME_BETALINGS_DEBIT = "betalings_debit";
@SerializedName(SERIALIZED_NAME_BETALINGS_DEBIT)
private BetalingsDebit betalingsDebit;
public static final String SERIALIZED_NAME_AUTOGIRO_DEBIT = "autogiro_debit";
@SerializedName(SERIALIZED_NAME_AUTOGIRO_DEBIT)
private AutogiroDebit autogiroDebit;
public static final String SERIALIZED_NAME_BACS_DEBIT = "bacs_debit";
@SerializedName(SERIALIZED_NAME_BACS_DEBIT)
private BacsDebit bacsDebit;
public static final String SERIALIZED_NAME_AU_BECS_DEBIT = "au_becs_debit";
@SerializedName(SERIALIZED_NAME_AU_BECS_DEBIT)
private AuBecsDebit auBecsDebit;
public static final String SERIALIZED_NAME_NZ_BECS_DEBIT = "nz_becs_debit";
@SerializedName(SERIALIZED_NAME_NZ_BECS_DEBIT)
private NzBecsDebit nzBecsDebit;
public static final String SERIALIZED_NAME_PAD_DEBIT = "pad_debit";
@SerializedName(SERIALIZED_NAME_PAD_DEBIT)
private PadDebit padDebit;
/**
* The state of the payment method.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
ACTIVE("active"),
CLOSED("closed"),
SCRUBBED("scrubbed"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_AUTO_GENERATED = "auto_generated";
@SerializedName(SERIALIZED_NAME_AUTO_GENERATED)
private Boolean autoGenerated;
public static final String SERIALIZED_NAME_USE_DEFAULT_RETRY_RULE = "use_default_retry_rule";
@SerializedName(SERIALIZED_NAME_USE_DEFAULT_RETRY_RULE)
private Boolean useDefaultRetryRule;
public static final String SERIALIZED_NAME_EXISTING_MANDATE = "existing_mandate";
@SerializedName(SERIALIZED_NAME_EXISTING_MANDATE)
private Boolean existingMandate;
public static final String SERIALIZED_NAME_LAST_FAILED_SALE_TRANSACTION_TIME = "last_failed_sale_transaction_time";
@SerializedName(SERIALIZED_NAME_LAST_FAILED_SALE_TRANSACTION_TIME)
private OffsetDateTime lastFailedSaleTransactionTime;
public static final String SERIALIZED_NAME_LAST_TRANSACTION_TIME = "last_transaction_time";
@SerializedName(SERIALIZED_NAME_LAST_TRANSACTION_TIME)
private OffsetDateTime lastTransactionTime;
public static final String SERIALIZED_NAME_LAST_TRANSACTION_STATUS = "last_transaction_status";
@SerializedName(SERIALIZED_NAME_LAST_TRANSACTION_STATUS)
private String lastTransactionStatus;
public static final String SERIALIZED_NAME_NUMBER_OF_CONSECUTIVE_FAILURES = "number_of_consecutive_failures";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_CONSECUTIVE_FAILURES)
private Integer numberOfConsecutiveFailures;
public static final String SERIALIZED_NAME_TOTAL_NUMBER_OF_PROCESSED_PAYMENTS = "total_number_of_processed_payments";
@SerializedName(SERIALIZED_NAME_TOTAL_NUMBER_OF_PROCESSED_PAYMENTS)
private Integer totalNumberOfProcessedPayments;
public static final String SERIALIZED_NAME_TOTAL_NUMBER_OF_ERROR_PAYMENTS = "total_number_of_error_payments";
@SerializedName(SERIALIZED_NAME_TOTAL_NUMBER_OF_ERROR_PAYMENTS)
private Integer totalNumberOfErrorPayments;
public PaymentMethod() {
}
public PaymentMethod(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects,
Account account
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
this.account = account;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public PaymentMethod customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public PaymentMethod putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public PaymentMethod type(TypeEnum type) {
this.type = type;
return this;
}
/**
* The type of the payment method. An additional hash is included on the payment method with a name matching this value. It contains additional information specific to the payment method type.
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The type of the payment method. An additional hash is included on the payment method with a name matching this value. It contains additional information specific to the payment method type.")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public PaymentMethod customType(String customType) {
this.customType = customType;
return this;
}
/**
* The custom type of the payment method from Universal Payment Connector.
* @return customType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom type of the payment method from Universal Payment Connector.")
public String getCustomType() {
return customType;
}
public void setCustomType(String customType) {
this.customType = customType;
}
public PaymentMethod accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* A customer account identifier.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "A customer account identifier.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
* The customer account associated with this payment method.
* @return account
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The customer account associated with this payment method.")
public Account getAccount() {
return account;
}
public PaymentMethod billingDetails(BillingDetails billingDetails) {
this.billingDetails = billingDetails;
return this;
}
/**
* Get billingDetails
* @return billingDetails
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public BillingDetails getBillingDetails() {
return billingDetails;
}
public void setBillingDetails(BillingDetails billingDetails) {
this.billingDetails = billingDetails;
}
public PaymentMethod maximumPaymentAttempts(BigDecimal maximumPaymentAttempts) {
this.maximumPaymentAttempts = maximumPaymentAttempts;
return this;
}
/**
* Maximum number of consecutive failed retry payment attempts using this payment method before retries are stopped.
* @return maximumPaymentAttempts
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "6", value = "Maximum number of consecutive failed retry payment attempts using this payment method before retries are stopped.")
public BigDecimal getMaximumPaymentAttempts() {
return maximumPaymentAttempts;
}
public void setMaximumPaymentAttempts(BigDecimal maximumPaymentAttempts) {
this.maximumPaymentAttempts = maximumPaymentAttempts;
}
public PaymentMethod paymentRetryInterval(Integer paymentRetryInterval) {
this.paymentRetryInterval = paymentRetryInterval;
return this;
}
/**
* The retry interval in hours.
* @return paymentRetryInterval
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "3", value = "The retry interval in hours.")
public Integer getPaymentRetryInterval() {
return paymentRetryInterval;
}
public void setPaymentRetryInterval(Integer paymentRetryInterval) {
this.paymentRetryInterval = paymentRetryInterval;
}
public PaymentMethod deviceSessionId(String deviceSessionId) {
this.deviceSessionId = deviceSessionId;
return this;
}
/**
* Get deviceSessionId
* @return deviceSessionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getDeviceSessionId() {
return deviceSessionId;
}
public void setDeviceSessionId(String deviceSessionId) {
this.deviceSessionId = deviceSessionId;
}
public PaymentMethod ipAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* The IP address from which the Mandate was accepted by the customer.
* @return ipAddress
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "192.10.1.123", value = "The IP address from which the Mandate was accepted by the customer.")
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public PaymentMethod bankIdentificationNumber(String bankIdentificationNumber) {
this.bankIdentificationNumber = bankIdentificationNumber;
return this;
}
/**
* Get bankIdentificationNumber
* @return bankIdentificationNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getBankIdentificationNumber() {
return bankIdentificationNumber;
}
public void setBankIdentificationNumber(String bankIdentificationNumber) {
this.bankIdentificationNumber = bankIdentificationNumber;
}
public PaymentMethod card(GetCardList card) {
this.card = card;
return this;
}
/**
* Get card
* @return card
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public GetCardList getCard() {
return card;
}
public void setCard(GetCardList card) {
this.card = card;
}
public PaymentMethod paypalExpressNative(PaypalExpressNative paypalExpressNative) {
this.paypalExpressNative = paypalExpressNative;
return this;
}
/**
* Get paypalExpressNative
* @return paypalExpressNative
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PaypalExpressNative getPaypalExpressNative() {
return paypalExpressNative;
}
public void setPaypalExpressNative(PaypalExpressNative paypalExpressNative) {
this.paypalExpressNative = paypalExpressNative;
}
public PaymentMethod paypalExpress(PaypalExpress paypalExpress) {
this.paypalExpress = paypalExpress;
return this;
}
/**
* Get paypalExpress
* @return paypalExpress
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PaypalExpress getPaypalExpress() {
return paypalExpress;
}
public void setPaypalExpress(PaypalExpress paypalExpress) {
this.paypalExpress = paypalExpress;
}
public PaymentMethod paypalAdaptive(PaypalAdaptive paypalAdaptive) {
this.paypalAdaptive = paypalAdaptive;
return this;
}
/**
* Get paypalAdaptive
* @return paypalAdaptive
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PaypalAdaptive getPaypalAdaptive() {
return paypalAdaptive;
}
public void setPaypalAdaptive(PaypalAdaptive paypalAdaptive) {
this.paypalAdaptive = paypalAdaptive;
}
public PaymentMethod sepaDebit(SepaDebit sepaDebit) {
this.sepaDebit = sepaDebit;
return this;
}
/**
* Get sepaDebit
* @return sepaDebit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SepaDebit getSepaDebit() {
return sepaDebit;
}
public void setSepaDebit(SepaDebit sepaDebit) {
this.sepaDebit = sepaDebit;
}
public PaymentMethod ccRef(CcRef ccRef) {
this.ccRef = ccRef;
return this;
}
/**
* Get ccRef
* @return ccRef
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CcRef getCcRef() {
return ccRef;
}
public void setCcRef(CcRef ccRef) {
this.ccRef = ccRef;
}
public PaymentMethod applePay(ApplePay applePay) {
this.applePay = applePay;
return this;
}
/**
* Get applePay
* @return applePay
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ApplePay getApplePay() {
return applePay;
}
public void setApplePay(ApplePay applePay) {
this.applePay = applePay;
}
public PaymentMethod googlePay(GooglePay googlePay) {
this.googlePay = googlePay;
return this;
}
/**
* Get googlePay
* @return googlePay
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public GooglePay getGooglePay() {
return googlePay;
}
public void setGooglePay(GooglePay googlePay) {
this.googlePay = googlePay;
}
public PaymentMethod achDebit(AchDebit achDebit) {
this.achDebit = achDebit;
return this;
}
/**
* Get achDebit
* @return achDebit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public AchDebit getAchDebit() {
return achDebit;
}
public void setAchDebit(AchDebit achDebit) {
this.achDebit = achDebit;
}
public PaymentMethod betalingsDebit(BetalingsDebit betalingsDebit) {
this.betalingsDebit = betalingsDebit;
return this;
}
/**
* Get betalingsDebit
* @return betalingsDebit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public BetalingsDebit getBetalingsDebit() {
return betalingsDebit;
}
public void setBetalingsDebit(BetalingsDebit betalingsDebit) {
this.betalingsDebit = betalingsDebit;
}
public PaymentMethod autogiroDebit(AutogiroDebit autogiroDebit) {
this.autogiroDebit = autogiroDebit;
return this;
}
/**
* Get autogiroDebit
* @return autogiroDebit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public AutogiroDebit getAutogiroDebit() {
return autogiroDebit;
}
public void setAutogiroDebit(AutogiroDebit autogiroDebit) {
this.autogiroDebit = autogiroDebit;
}
public PaymentMethod bacsDebit(BacsDebit bacsDebit) {
this.bacsDebit = bacsDebit;
return this;
}
/**
* Get bacsDebit
* @return bacsDebit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public BacsDebit getBacsDebit() {
return bacsDebit;
}
public void setBacsDebit(BacsDebit bacsDebit) {
this.bacsDebit = bacsDebit;
}
public PaymentMethod auBecsDebit(AuBecsDebit auBecsDebit) {
this.auBecsDebit = auBecsDebit;
return this;
}
/**
* Get auBecsDebit
* @return auBecsDebit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public AuBecsDebit getAuBecsDebit() {
return auBecsDebit;
}
public void setAuBecsDebit(AuBecsDebit auBecsDebit) {
this.auBecsDebit = auBecsDebit;
}
public PaymentMethod nzBecsDebit(NzBecsDebit nzBecsDebit) {
this.nzBecsDebit = nzBecsDebit;
return this;
}
/**
* Get nzBecsDebit
* @return nzBecsDebit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public NzBecsDebit getNzBecsDebit() {
return nzBecsDebit;
}
public void setNzBecsDebit(NzBecsDebit nzBecsDebit) {
this.nzBecsDebit = nzBecsDebit;
}
public PaymentMethod padDebit(PadDebit padDebit) {
this.padDebit = padDebit;
return this;
}
/**
* Get padDebit
* @return padDebit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PadDebit getPadDebit() {
return padDebit;
}
public void setPadDebit(PadDebit padDebit) {
this.padDebit = padDebit;
}
public PaymentMethod state(StateEnum state) {
this.state = state;
return this;
}
/**
* The state of the payment method.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The state of the payment method.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public PaymentMethod autoGenerated(Boolean autoGenerated) {
this.autoGenerated = autoGenerated;
return this;
}
/**
* Get autoGenerated
* @return autoGenerated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getAutoGenerated() {
return autoGenerated;
}
public void setAutoGenerated(Boolean autoGenerated) {
this.autoGenerated = autoGenerated;
}
public PaymentMethod useDefaultRetryRule(Boolean useDefaultRetryRule) {
this.useDefaultRetryRule = useDefaultRetryRule;
return this;
}
/**
* Get useDefaultRetryRule
* @return useDefaultRetryRule
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getUseDefaultRetryRule() {
return useDefaultRetryRule;
}
public void setUseDefaultRetryRule(Boolean useDefaultRetryRule) {
this.useDefaultRetryRule = useDefaultRetryRule;
}
public PaymentMethod existingMandate(Boolean existingMandate) {
this.existingMandate = existingMandate;
return this;
}
/**
* Get existingMandate
* @return existingMandate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getExistingMandate() {
return existingMandate;
}
public void setExistingMandate(Boolean existingMandate) {
this.existingMandate = existingMandate;
}
public PaymentMethod lastFailedSaleTransactionTime(OffsetDateTime lastFailedSaleTransactionTime) {
this.lastFailedSaleTransactionTime = lastFailedSaleTransactionTime;
return this;
}
/**
* Get lastFailedSaleTransactionTime
* @return lastFailedSaleTransactionTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public OffsetDateTime getLastFailedSaleTransactionTime() {
return lastFailedSaleTransactionTime;
}
public void setLastFailedSaleTransactionTime(OffsetDateTime lastFailedSaleTransactionTime) {
this.lastFailedSaleTransactionTime = lastFailedSaleTransactionTime;
}
public PaymentMethod lastTransactionTime(OffsetDateTime lastTransactionTime) {
this.lastTransactionTime = lastTransactionTime;
return this;
}
/**
* Get lastTransactionTime
* @return lastTransactionTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public OffsetDateTime getLastTransactionTime() {
return lastTransactionTime;
}
public void setLastTransactionTime(OffsetDateTime lastTransactionTime) {
this.lastTransactionTime = lastTransactionTime;
}
public PaymentMethod lastTransactionStatus(String lastTransactionStatus) {
this.lastTransactionStatus = lastTransactionStatus;
return this;
}
/**
* Get lastTransactionStatus
* @return lastTransactionStatus
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getLastTransactionStatus() {
return lastTransactionStatus;
}
public void setLastTransactionStatus(String lastTransactionStatus) {
this.lastTransactionStatus = lastTransactionStatus;
}
public PaymentMethod numberOfConsecutiveFailures(Integer numberOfConsecutiveFailures) {
this.numberOfConsecutiveFailures = numberOfConsecutiveFailures;
return this;
}
/**
* Get numberOfConsecutiveFailures
* @return numberOfConsecutiveFailures
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getNumberOfConsecutiveFailures() {
return numberOfConsecutiveFailures;
}
public void setNumberOfConsecutiveFailures(Integer numberOfConsecutiveFailures) {
this.numberOfConsecutiveFailures = numberOfConsecutiveFailures;
}
public PaymentMethod totalNumberOfProcessedPayments(Integer totalNumberOfProcessedPayments) {
this.totalNumberOfProcessedPayments = totalNumberOfProcessedPayments;
return this;
}
/**
* Get totalNumberOfProcessedPayments
* @return totalNumberOfProcessedPayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getTotalNumberOfProcessedPayments() {
return totalNumberOfProcessedPayments;
}
public void setTotalNumberOfProcessedPayments(Integer totalNumberOfProcessedPayments) {
this.totalNumberOfProcessedPayments = totalNumberOfProcessedPayments;
}
public PaymentMethod totalNumberOfErrorPayments(Integer totalNumberOfErrorPayments) {
this.totalNumberOfErrorPayments = totalNumberOfErrorPayments;
return this;
}
/**
* Get totalNumberOfErrorPayments
* @return totalNumberOfErrorPayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getTotalNumberOfErrorPayments() {
return totalNumberOfErrorPayments;
}
public void setTotalNumberOfErrorPayments(Integer totalNumberOfErrorPayments) {
this.totalNumberOfErrorPayments = totalNumberOfErrorPayments;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentMethod paymentMethod = (PaymentMethod) o;
return Objects.equals(this.id, paymentMethod.id) &&
Objects.equals(this.updatedById, paymentMethod.updatedById) &&
Objects.equals(this.updatedTime, paymentMethod.updatedTime) &&
Objects.equals(this.createdById, paymentMethod.createdById) &&
Objects.equals(this.createdTime, paymentMethod.createdTime) &&
Objects.equals(this.customFields, paymentMethod.customFields) &&
Objects.equals(this.customObjects, paymentMethod.customObjects) &&
Objects.equals(this.type, paymentMethod.type) &&
Objects.equals(this.customType, paymentMethod.customType) &&
Objects.equals(this.accountId, paymentMethod.accountId) &&
Objects.equals(this.account, paymentMethod.account) &&
Objects.equals(this.billingDetails, paymentMethod.billingDetails) &&
Objects.equals(this.maximumPaymentAttempts, paymentMethod.maximumPaymentAttempts) &&
Objects.equals(this.paymentRetryInterval, paymentMethod.paymentRetryInterval) &&
Objects.equals(this.deviceSessionId, paymentMethod.deviceSessionId) &&
Objects.equals(this.ipAddress, paymentMethod.ipAddress) &&
Objects.equals(this.bankIdentificationNumber, paymentMethod.bankIdentificationNumber) &&
Objects.equals(this.card, paymentMethod.card) &&
Objects.equals(this.paypalExpressNative, paymentMethod.paypalExpressNative) &&
Objects.equals(this.paypalExpress, paymentMethod.paypalExpress) &&
Objects.equals(this.paypalAdaptive, paymentMethod.paypalAdaptive) &&
Objects.equals(this.sepaDebit, paymentMethod.sepaDebit) &&
Objects.equals(this.ccRef, paymentMethod.ccRef) &&
Objects.equals(this.applePay, paymentMethod.applePay) &&
Objects.equals(this.googlePay, paymentMethod.googlePay) &&
Objects.equals(this.achDebit, paymentMethod.achDebit) &&
Objects.equals(this.betalingsDebit, paymentMethod.betalingsDebit) &&
Objects.equals(this.autogiroDebit, paymentMethod.autogiroDebit) &&
Objects.equals(this.bacsDebit, paymentMethod.bacsDebit) &&
Objects.equals(this.auBecsDebit, paymentMethod.auBecsDebit) &&
Objects.equals(this.nzBecsDebit, paymentMethod.nzBecsDebit) &&
Objects.equals(this.padDebit, paymentMethod.padDebit) &&
Objects.equals(this.state, paymentMethod.state) &&
Objects.equals(this.autoGenerated, paymentMethod.autoGenerated) &&
Objects.equals(this.useDefaultRetryRule, paymentMethod.useDefaultRetryRule) &&
Objects.equals(this.existingMandate, paymentMethod.existingMandate) &&
Objects.equals(this.lastFailedSaleTransactionTime, paymentMethod.lastFailedSaleTransactionTime) &&
Objects.equals(this.lastTransactionTime, paymentMethod.lastTransactionTime) &&
Objects.equals(this.lastTransactionStatus, paymentMethod.lastTransactionStatus) &&
Objects.equals(this.numberOfConsecutiveFailures, paymentMethod.numberOfConsecutiveFailures) &&
Objects.equals(this.totalNumberOfProcessedPayments, paymentMethod.totalNumberOfProcessedPayments) &&
Objects.equals(this.totalNumberOfErrorPayments, paymentMethod.totalNumberOfErrorPayments);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, type, customType, accountId, account, billingDetails, maximumPaymentAttempts, paymentRetryInterval, deviceSessionId, ipAddress, bankIdentificationNumber, card, paypalExpressNative, paypalExpress, paypalAdaptive, sepaDebit, ccRef, applePay, googlePay, achDebit, betalingsDebit, autogiroDebit, bacsDebit, auBecsDebit, nzBecsDebit, padDebit, state, autoGenerated, useDefaultRetryRule, existingMandate, lastFailedSaleTransactionTime, lastTransactionTime, lastTransactionStatus, numberOfConsecutiveFailures, totalNumberOfProcessedPayments, totalNumberOfErrorPayments);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentMethod {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" customType: ").append(toIndentedString(customType)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" billingDetails: ").append(toIndentedString(billingDetails)).append("\n");
sb.append(" maximumPaymentAttempts: ").append(toIndentedString(maximumPaymentAttempts)).append("\n");
sb.append(" paymentRetryInterval: ").append(toIndentedString(paymentRetryInterval)).append("\n");
sb.append(" deviceSessionId: ").append(toIndentedString(deviceSessionId)).append("\n");
sb.append(" ipAddress: ").append(toIndentedString(ipAddress)).append("\n");
sb.append(" bankIdentificationNumber: ").append(toIndentedString(bankIdentificationNumber)).append("\n");
sb.append(" card: ").append(toIndentedString(card)).append("\n");
sb.append(" paypalExpressNative: ").append(toIndentedString(paypalExpressNative)).append("\n");
sb.append(" paypalExpress: ").append(toIndentedString(paypalExpress)).append("\n");
sb.append(" paypalAdaptive: ").append(toIndentedString(paypalAdaptive)).append("\n");
sb.append(" sepaDebit: ").append(toIndentedString(sepaDebit)).append("\n");
sb.append(" ccRef: ").append(toIndentedString(ccRef)).append("\n");
sb.append(" applePay: ").append(toIndentedString(applePay)).append("\n");
sb.append(" googlePay: ").append(toIndentedString(googlePay)).append("\n");
sb.append(" achDebit: ").append(toIndentedString(achDebit)).append("\n");
sb.append(" betalingsDebit: ").append(toIndentedString(betalingsDebit)).append("\n");
sb.append(" autogiroDebit: ").append(toIndentedString(autogiroDebit)).append("\n");
sb.append(" bacsDebit: ").append(toIndentedString(bacsDebit)).append("\n");
sb.append(" auBecsDebit: ").append(toIndentedString(auBecsDebit)).append("\n");
sb.append(" nzBecsDebit: ").append(toIndentedString(nzBecsDebit)).append("\n");
sb.append(" padDebit: ").append(toIndentedString(padDebit)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" autoGenerated: ").append(toIndentedString(autoGenerated)).append("\n");
sb.append(" useDefaultRetryRule: ").append(toIndentedString(useDefaultRetryRule)).append("\n");
sb.append(" existingMandate: ").append(toIndentedString(existingMandate)).append("\n");
sb.append(" lastFailedSaleTransactionTime: ").append(toIndentedString(lastFailedSaleTransactionTime)).append("\n");
sb.append(" lastTransactionTime: ").append(toIndentedString(lastTransactionTime)).append("\n");
sb.append(" lastTransactionStatus: ").append(toIndentedString(lastTransactionStatus)).append("\n");
sb.append(" numberOfConsecutiveFailures: ").append(toIndentedString(numberOfConsecutiveFailures)).append("\n");
sb.append(" totalNumberOfProcessedPayments: ").append(toIndentedString(totalNumberOfProcessedPayments)).append("\n");
sb.append(" totalNumberOfErrorPayments: ").append(toIndentedString(totalNumberOfErrorPayments)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy