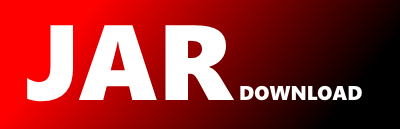
org.openapitools.client.model.PaymentMethodAuthorizationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PaymentMethodAuthorizationResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentMethodAuthorizationResponse {
public static final String SERIALIZED_NAME_AUTH_TRANSACTION_ID = "auth_transaction_id";
@SerializedName(SERIALIZED_NAME_AUTH_TRANSACTION_ID)
private String authTransactionId;
public static final String SERIALIZED_NAME_GATEWAY_ORDER_ID = "gateway_order_id";
@SerializedName(SERIALIZED_NAME_GATEWAY_ORDER_ID)
private String gatewayOrderId;
/**
* The status of the payment authorization.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
APPROVED("approved"),
QUEUED_FOR_SUBMISSION("queued_for_submission"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public PaymentMethodAuthorizationResponse() {
}
public PaymentMethodAuthorizationResponse authTransactionId(String authTransactionId) {
this.authTransactionId = authTransactionId;
return this;
}
/**
* Identifier of the authorization transaction from the payment gateway.
* @return authTransactionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the authorization transaction from the payment gateway.")
public String getAuthTransactionId() {
return authTransactionId;
}
public void setAuthTransactionId(String authTransactionId) {
this.authTransactionId = authTransactionId;
}
public PaymentMethodAuthorizationResponse gatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
return this;
}
/**
* A merchant-specified natural key value that can be passed to the electronic payment gateway when a payment is created. If not specified, the payment number will be passed in instead. Gateways check duplicates on the gateway order ID to ensure that the merchant do not accidentally enter the same transaction twice. This ID can also be used to do reconciliation and tie the payment to a natural key in external systems. The source of this ID varies by merchant. Some merchants use their shopping cart order IDs, and others use something different. Merchants use this ID to track transactions in their eCommerce systems. When you create a payment for capturing the authorized funds, it is highly recommended to pass in the `gateway_order_id` that you used when authorizing the funds by using the Create authorization operation, together with the `authorization_id` field.
* @return gatewayOrderId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A merchant-specified natural key value that can be passed to the electronic payment gateway when a payment is created. If not specified, the payment number will be passed in instead. Gateways check duplicates on the gateway order ID to ensure that the merchant do not accidentally enter the same transaction twice. This ID can also be used to do reconciliation and tie the payment to a natural key in external systems. The source of this ID varies by merchant. Some merchants use their shopping cart order IDs, and others use something different. Merchants use this ID to track transactions in their eCommerce systems. When you create a payment for capturing the authorized funds, it is highly recommended to pass in the `gateway_order_id` that you used when authorizing the funds by using the Create authorization operation, together with the `authorization_id` field.")
public String getGatewayOrderId() {
return gatewayOrderId;
}
public void setGatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
}
public PaymentMethodAuthorizationResponse state(StateEnum state) {
this.state = state;
return this;
}
/**
* The status of the payment authorization.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The status of the payment authorization.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentMethodAuthorizationResponse paymentMethodAuthorizationResponse = (PaymentMethodAuthorizationResponse) o;
return Objects.equals(this.authTransactionId, paymentMethodAuthorizationResponse.authTransactionId) &&
Objects.equals(this.gatewayOrderId, paymentMethodAuthorizationResponse.gatewayOrderId) &&
Objects.equals(this.state, paymentMethodAuthorizationResponse.state);
}
@Override
public int hashCode() {
return Objects.hash(authTransactionId, gatewayOrderId, state);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentMethodAuthorizationResponse {\n");
sb.append(" authTransactionId: ").append(toIndentedString(authTransactionId)).append("\n");
sb.append(" gatewayOrderId: ").append(toIndentedString(gatewayOrderId)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy