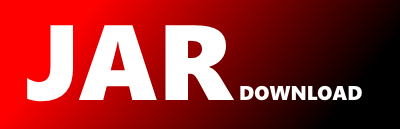
org.openapitools.client.model.PaymentMethodPatchRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.AchDebitUpdate;
import org.openapitools.client.model.BillingDetails;
import org.openapitools.client.model.CardUpdate;
import org.openapitools.client.model.CcRefMandate;
import org.openapitools.client.model.CcRefUpdate;
import org.openapitools.jackson.nullable.JsonNullable;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PaymentMethodPatchRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentMethodPatchRequest {
public static final String SERIALIZED_NAME_BILLING_DETAILS = "billing_details";
@SerializedName(SERIALIZED_NAME_BILLING_DETAILS)
private BillingDetails billingDetails;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CARD = "card";
@SerializedName(SERIALIZED_NAME_CARD)
private CardUpdate card;
public static final String SERIALIZED_NAME_CC_REF = "cc_ref";
@SerializedName(SERIALIZED_NAME_CC_REF)
private CcRefUpdate ccRef;
public static final String SERIALIZED_NAME_MANDATE = "mandate";
@SerializedName(SERIALIZED_NAME_MANDATE)
private CcRefMandate mandate;
public static final String SERIALIZED_NAME_ACH_DEBIT = "ach_debit";
@SerializedName(SERIALIZED_NAME_ACH_DEBIT)
private AchDebitUpdate achDebit;
public static final String SERIALIZED_NAME_MAXIMUM_PAYMENT_ATTEMPTS = "maximum_payment_attempts";
@SerializedName(SERIALIZED_NAME_MAXIMUM_PAYMENT_ATTEMPTS)
private BigDecimal maximumPaymentAttempts;
public static final String SERIALIZED_NAME_PAYMENT_RETRY_INTERVAL = "payment_retry_interval";
@SerializedName(SERIALIZED_NAME_PAYMENT_RETRY_INTERVAL)
private Integer paymentRetryInterval;
public static final String SERIALIZED_NAME_DEVICE_SESSION_ID = "device_session_id";
@SerializedName(SERIALIZED_NAME_DEVICE_SESSION_ID)
private String deviceSessionId;
public static final String SERIALIZED_NAME_GATEWAY_OPTIONS = "gateway_options";
@SerializedName(SERIALIZED_NAME_GATEWAY_OPTIONS)
private Map gatewayOptions = null;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gateway_id";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public PaymentMethodPatchRequest() {
}
public PaymentMethodPatchRequest billingDetails(BillingDetails billingDetails) {
this.billingDetails = billingDetails;
return this;
}
/**
* Get billingDetails
* @return billingDetails
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public BillingDetails getBillingDetails() {
return billingDetails;
}
public void setBillingDetails(BillingDetails billingDetails) {
this.billingDetails = billingDetails;
}
public PaymentMethodPatchRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* A customer account identifier.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "A customer account identifier.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public PaymentMethodPatchRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* A human-readable customer account identifier. It can be user-supplied.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "A-100001", value = "A human-readable customer account identifier. It can be user-supplied.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public PaymentMethodPatchRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public PaymentMethodPatchRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public PaymentMethodPatchRequest card(CardUpdate card) {
this.card = card;
return this;
}
/**
* Credit card information. When providing a card number, you must meet the requirements for PCI compliance. We strongly recommend using Zuora.js instead of interacting with this API directly.
* @return card
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Credit card information. When providing a card number, you must meet the requirements for PCI compliance. We strongly recommend using Zuora.js instead of interacting with this API directly.")
public CardUpdate getCard() {
return card;
}
public void setCard(CardUpdate card) {
this.card = card;
}
public PaymentMethodPatchRequest ccRef(CcRefUpdate ccRef) {
this.ccRef = ccRef;
return this;
}
/**
* Credit Card Reference Transaction. See Supported payment methods for payment gateways that support this type of payment method.
* @return ccRef
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Credit Card Reference Transaction. See Supported payment methods for payment gateways that support this type of payment method.")
public CcRefUpdate getCcRef() {
return ccRef;
}
public void setCcRef(CcRefUpdate ccRef) {
this.ccRef = ccRef;
}
public PaymentMethodPatchRequest mandate(CcRefMandate mandate) {
this.mandate = mandate;
return this;
}
/**
* Get mandate
* @return mandate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CcRefMandate getMandate() {
return mandate;
}
public void setMandate(CcRefMandate mandate) {
this.mandate = mandate;
}
public PaymentMethodPatchRequest achDebit(AchDebitUpdate achDebit) {
this.achDebit = achDebit;
return this;
}
/**
* If this is an ach_debit payment method, this hash contains details about the ACH debit bank account.
* @return achDebit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If this is an ach_debit payment method, this hash contains details about the ACH debit bank account.")
public AchDebitUpdate getAchDebit() {
return achDebit;
}
public void setAchDebit(AchDebitUpdate achDebit) {
this.achDebit = achDebit;
}
public PaymentMethodPatchRequest maximumPaymentAttempts(BigDecimal maximumPaymentAttempts) {
this.maximumPaymentAttempts = maximumPaymentAttempts;
return this;
}
/**
* Maximum number of consecutive failed retry payment attempts using this payment method before retries are stopped.
* @return maximumPaymentAttempts
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "6", value = "Maximum number of consecutive failed retry payment attempts using this payment method before retries are stopped.")
public BigDecimal getMaximumPaymentAttempts() {
return maximumPaymentAttempts;
}
public void setMaximumPaymentAttempts(BigDecimal maximumPaymentAttempts) {
this.maximumPaymentAttempts = maximumPaymentAttempts;
}
public PaymentMethodPatchRequest paymentRetryInterval(Integer paymentRetryInterval) {
this.paymentRetryInterval = paymentRetryInterval;
return this;
}
/**
* The retry interval in hours.
* @return paymentRetryInterval
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "3", value = "The retry interval in hours.")
public Integer getPaymentRetryInterval() {
return paymentRetryInterval;
}
public void setPaymentRetryInterval(Integer paymentRetryInterval) {
this.paymentRetryInterval = paymentRetryInterval;
}
public PaymentMethodPatchRequest deviceSessionId(String deviceSessionId) {
this.deviceSessionId = deviceSessionId;
return this;
}
/**
* Identifier of the device browser session.
* @return deviceSessionId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2cadffe03", value = "Identifier of the device browser session.")
public String getDeviceSessionId() {
return deviceSessionId;
}
public void setDeviceSessionId(String deviceSessionId) {
this.deviceSessionId = deviceSessionId;
}
public PaymentMethodPatchRequest gatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
return this;
}
public PaymentMethodPatchRequest putGatewayOptionsItem(String key, String gatewayOptionsItem) {
if (this.gatewayOptions == null) {
this.gatewayOptions = new HashMap();
}
this.gatewayOptions.put(key, gatewayOptionsItem);
return this;
}
/**
* Get gatewayOptions
* @return gatewayOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"key\":\"value\"}", value = "")
public Map getGatewayOptions() {
return gatewayOptions;
}
public void setGatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
}
public PaymentMethodPatchRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* 3-letter ISO 4217 currency code.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "3-letter ISO 4217 currency code.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PaymentMethodPatchRequest gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* Identifier of the payment gateway Zuora will use to authorize the payments that are made with this payment method.
* @return gatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payment gateway Zuora will use to authorize the payments that are made with this payment method.")
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentMethodPatchRequest paymentMethodPatchRequest = (PaymentMethodPatchRequest) o;
return Objects.equals(this.billingDetails, paymentMethodPatchRequest.billingDetails) &&
Objects.equals(this.accountId, paymentMethodPatchRequest.accountId) &&
Objects.equals(this.accountNumber, paymentMethodPatchRequest.accountNumber) &&
Objects.equals(this.customFields, paymentMethodPatchRequest.customFields) &&
Objects.equals(this.card, paymentMethodPatchRequest.card) &&
Objects.equals(this.ccRef, paymentMethodPatchRequest.ccRef) &&
Objects.equals(this.mandate, paymentMethodPatchRequest.mandate) &&
Objects.equals(this.achDebit, paymentMethodPatchRequest.achDebit) &&
Objects.equals(this.maximumPaymentAttempts, paymentMethodPatchRequest.maximumPaymentAttempts) &&
Objects.equals(this.paymentRetryInterval, paymentMethodPatchRequest.paymentRetryInterval) &&
Objects.equals(this.deviceSessionId, paymentMethodPatchRequest.deviceSessionId) &&
Objects.equals(this.gatewayOptions, paymentMethodPatchRequest.gatewayOptions) &&
Objects.equals(this.currency, paymentMethodPatchRequest.currency) &&
Objects.equals(this.gatewayId, paymentMethodPatchRequest.gatewayId);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(billingDetails, accountId, accountNumber, customFields, card, ccRef, mandate, achDebit, maximumPaymentAttempts, paymentRetryInterval, deviceSessionId, gatewayOptions, currency, gatewayId);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentMethodPatchRequest {\n");
sb.append(" billingDetails: ").append(toIndentedString(billingDetails)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" card: ").append(toIndentedString(card)).append("\n");
sb.append(" ccRef: ").append(toIndentedString(ccRef)).append("\n");
sb.append(" mandate: ").append(toIndentedString(mandate)).append("\n");
sb.append(" achDebit: ").append(toIndentedString(achDebit)).append("\n");
sb.append(" maximumPaymentAttempts: ").append(toIndentedString(maximumPaymentAttempts)).append("\n");
sb.append(" paymentRetryInterval: ").append(toIndentedString(paymentRetryInterval)).append("\n");
sb.append(" deviceSessionId: ").append(toIndentedString(deviceSessionId)).append("\n");
sb.append(" gatewayOptions: ").append(toIndentedString(gatewayOptions)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy