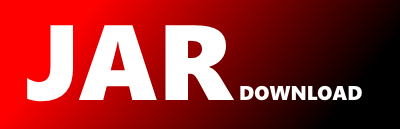
org.openapitools.client.model.PaymentRun Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.PaymentRunSummary;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PaymentRun
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentRun {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_APPLY_CREDIT_MEMOS = "apply_credit_memos";
@SerializedName(SERIALIZED_NAME_APPLY_CREDIT_MEMOS)
private Boolean applyCreditMemos;
public static final String SERIALIZED_NAME_APPLY_UNAPPLIED_PAYMENTS = "apply_unapplied_payments";
@SerializedName(SERIALIZED_NAME_APPLY_UNAPPLIED_PAYMENTS)
private Boolean applyUnappliedPayments;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_CONSOLIDATE_PAYMENT = "consolidate_payment";
@SerializedName(SERIALIZED_NAME_CONSOLIDATE_PAYMENT)
private Boolean consolidatePayment;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "bill_cycle_day";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Integer billCycleDay;
public static final String SERIALIZED_NAME_BILL_RUN_ID = "bill_run_id";
@SerializedName(SERIALIZED_NAME_BILL_RUN_ID)
private String billRunId;
public static final String SERIALIZED_NAME_COLLECT_PAYMENT = "collect_payment";
@SerializedName(SERIALIZED_NAME_COLLECT_PAYMENT)
private Boolean collectPayment;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_STATE_TRANSITIONS = "state_transitions";
@SerializedName(SERIALIZED_NAME_STATE_TRANSITIONS)
private Object stateTransitions;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_ID = "payment_gateway_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_ID)
private String paymentGatewayId;
public static final String SERIALIZED_NAME_PAYMENT_COLLECTION_DATE = "payment_collection_date";
@SerializedName(SERIALIZED_NAME_PAYMENT_COLLECTION_DATE)
private OffsetDateTime paymentCollectionDate;
public static final String SERIALIZED_NAME_PAYMENT_RUN_NUMBER = "payment_run_number";
@SerializedName(SERIALIZED_NAME_PAYMENT_RUN_NUMBER)
private String paymentRunNumber;
public static final String SERIALIZED_NAME_PAYMENT_RUN_DATE = "payment_run_date";
@SerializedName(SERIALIZED_NAME_PAYMENT_RUN_DATE)
private OffsetDateTime paymentRunDate;
public static final String SERIALIZED_NAME_TARGET_DATE = "target_date";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
/**
* Status of the payment run.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
PROCESSING("processing"),
PENDING("pending"),
COMPLETED("completed"),
CANCELED("canceled"),
FAILED("failed"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_SUMMARY = "summary";
@SerializedName(SERIALIZED_NAME_SUMMARY)
private PaymentRunSummary summary;
public PaymentRun() {
}
public PaymentRun(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects,
OffsetDateTime paymentCollectionDate,
PaymentRunSummary summary
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
this.paymentCollectionDate = paymentCollectionDate;
this.summary = summary;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public PaymentRun customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public PaymentRun putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public PaymentRun applyCreditMemos(Boolean applyCreditMemos) {
this.applyCreditMemos = applyCreditMemos;
return this;
}
/**
* If true, any posted credit memos are applied first.
* @return applyCreditMemos
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, any posted credit memos are applied first.")
public Boolean getApplyCreditMemos() {
return applyCreditMemos;
}
public void setApplyCreditMemos(Boolean applyCreditMemos) {
this.applyCreditMemos = applyCreditMemos;
}
public PaymentRun applyUnappliedPayments(Boolean applyUnappliedPayments) {
this.applyUnappliedPayments = applyUnappliedPayments;
return this;
}
/**
* If true, any unapplied payments are applied first.
* @return applyUnappliedPayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, any unapplied payments are applied first.")
public Boolean getApplyUnappliedPayments() {
return applyUnappliedPayments;
}
public void setApplyUnappliedPayments(Boolean applyUnappliedPayments) {
this.applyUnappliedPayments = applyUnappliedPayments;
}
public PaymentRun batch(String batch) {
this.batch = batch;
return this;
}
/**
* Identifier of the customer account batch to be included in this payment run.
* @return batch
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the customer account batch to be included in this payment run.")
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public PaymentRun consolidatePayment(Boolean consolidatePayment) {
this.consolidatePayment = consolidatePayment;
return this;
}
/**
* If true, a single payment will be collected for all receivables due on an account.
* @return consolidatePayment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, a single payment will be collected for all receivables due on an account.")
public Boolean getConsolidatePayment() {
return consolidatePayment;
}
public void setConsolidatePayment(Boolean consolidatePayment) {
this.consolidatePayment = consolidatePayment;
}
public PaymentRun billCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* The day of the month to bill multiple customer accounts.
* minimum: 0
* maximum: 31
* @return billCycleDay
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The day of the month to bill multiple customer accounts.")
public Integer getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
}
public PaymentRun billRunId(String billRunId) {
this.billRunId = billRunId;
return this;
}
/**
* The unique identifier of a bill run.
* @return billRunId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unique identifier of a bill run.")
public String getBillRunId() {
return billRunId;
}
public void setBillRunId(String billRunId) {
this.billRunId = billRunId;
}
public PaymentRun collectPayment(Boolean collectPayment) {
this.collectPayment = collectPayment;
return this;
}
/**
* Indicates whether to process electronic payments during the execution of payment runs.
* @return collectPayment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to process electronic payments during the execution of payment runs.")
public Boolean getCollectPayment() {
return collectPayment;
}
public void setCollectPayment(Boolean collectPayment) {
this.collectPayment = collectPayment;
}
public PaymentRun currency(String currency) {
this.currency = currency;
return this;
}
/**
* Three-letter ISO currency code.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USD", value = "Three-letter ISO currency code.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PaymentRun stateTransitions(Object stateTransitions) {
this.stateTransitions = stateTransitions;
return this;
}
/**
* The date and time when the payment run executed, in the `yyyy-mm-dd hh:mm:ss` format.
* @return stateTransitions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the payment run executed, in the `yyyy-mm-dd hh:mm:ss` format.")
public Object getStateTransitions() {
return stateTransitions;
}
public void setStateTransitions(Object stateTransitions) {
this.stateTransitions = stateTransitions;
}
public PaymentRun paymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
return this;
}
/**
* Unique identifier for the payment gateway.
* @return paymentGatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the payment gateway.")
public String getPaymentGatewayId() {
return paymentGatewayId;
}
public void setPaymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
}
/**
* Get paymentCollectionDate
* @return paymentCollectionDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public OffsetDateTime getPaymentCollectionDate() {
return paymentCollectionDate;
}
public PaymentRun paymentRunNumber(String paymentRunNumber) {
this.paymentRunNumber = paymentRunNumber;
return this;
}
/**
* Human-readable identifier for this object.
* @return paymentRunNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier for this object.")
public String getPaymentRunNumber() {
return paymentRunNumber;
}
public void setPaymentRunNumber(String paymentRunNumber) {
this.paymentRunNumber = paymentRunNumber;
}
public PaymentRun paymentRunDate(OffsetDateTime paymentRunDate) {
this.paymentRunDate = paymentRunDate;
return this;
}
/**
* The date and time when the scheduled payment run is to be executed, in `yyyy-mm-dd hh:mm:ss` format.
* @return paymentRunDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the scheduled payment run is to be executed, in `yyyy-mm-dd hh:mm:ss` format.")
public OffsetDateTime getPaymentRunDate() {
return paymentRunDate;
}
public void setPaymentRunDate(OffsetDateTime paymentRunDate) {
this.paymentRunDate = paymentRunDate;
}
public PaymentRun targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date used to determine which receivables to be paid in the payment run. The payments are collected for all receivables with the due date no later than the target date.
* @return targetDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The target date used to determine which receivables to be paid in the payment run. The payments are collected for all receivables with the due date no later than the target date.")
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public PaymentRun state(StateEnum state) {
this.state = state;
return this;
}
/**
* Status of the payment run.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Status of the payment run.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
/**
* Summary of the payment run.
* @return summary
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Summary of the payment run.")
public PaymentRunSummary getSummary() {
return summary;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentRun paymentRun = (PaymentRun) o;
return Objects.equals(this.id, paymentRun.id) &&
Objects.equals(this.updatedById, paymentRun.updatedById) &&
Objects.equals(this.updatedTime, paymentRun.updatedTime) &&
Objects.equals(this.createdById, paymentRun.createdById) &&
Objects.equals(this.createdTime, paymentRun.createdTime) &&
Objects.equals(this.customFields, paymentRun.customFields) &&
Objects.equals(this.customObjects, paymentRun.customObjects) &&
Objects.equals(this.applyCreditMemos, paymentRun.applyCreditMemos) &&
Objects.equals(this.applyUnappliedPayments, paymentRun.applyUnappliedPayments) &&
Objects.equals(this.batch, paymentRun.batch) &&
Objects.equals(this.consolidatePayment, paymentRun.consolidatePayment) &&
Objects.equals(this.billCycleDay, paymentRun.billCycleDay) &&
Objects.equals(this.billRunId, paymentRun.billRunId) &&
Objects.equals(this.collectPayment, paymentRun.collectPayment) &&
Objects.equals(this.currency, paymentRun.currency) &&
Objects.equals(this.stateTransitions, paymentRun.stateTransitions) &&
Objects.equals(this.paymentGatewayId, paymentRun.paymentGatewayId) &&
Objects.equals(this.paymentCollectionDate, paymentRun.paymentCollectionDate) &&
Objects.equals(this.paymentRunNumber, paymentRun.paymentRunNumber) &&
Objects.equals(this.paymentRunDate, paymentRun.paymentRunDate) &&
Objects.equals(this.targetDate, paymentRun.targetDate) &&
Objects.equals(this.state, paymentRun.state) &&
Objects.equals(this.summary, paymentRun.summary);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, applyCreditMemos, applyUnappliedPayments, batch, consolidatePayment, billCycleDay, billRunId, collectPayment, currency, stateTransitions, paymentGatewayId, paymentCollectionDate, paymentRunNumber, paymentRunDate, targetDate, state, summary);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentRun {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" applyCreditMemos: ").append(toIndentedString(applyCreditMemos)).append("\n");
sb.append(" applyUnappliedPayments: ").append(toIndentedString(applyUnappliedPayments)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" consolidatePayment: ").append(toIndentedString(consolidatePayment)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billRunId: ").append(toIndentedString(billRunId)).append("\n");
sb.append(" collectPayment: ").append(toIndentedString(collectPayment)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" stateTransitions: ").append(toIndentedString(stateTransitions)).append("\n");
sb.append(" paymentGatewayId: ").append(toIndentedString(paymentGatewayId)).append("\n");
sb.append(" paymentCollectionDate: ").append(toIndentedString(paymentCollectionDate)).append("\n");
sb.append(" paymentRunNumber: ").append(toIndentedString(paymentRunNumber)).append("\n");
sb.append(" paymentRunDate: ").append(toIndentedString(paymentRunDate)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" summary: ").append(toIndentedString(summary)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy