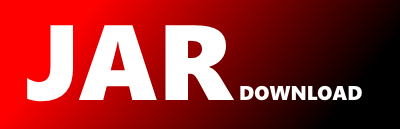
org.openapitools.client.model.PaymentRunPatchRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PaymentRunPatchRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentRunPatchRequest {
public static final String SERIALIZED_NAME_APPLY_CREDIT_MEMOS = "apply_credit_memos";
@SerializedName(SERIALIZED_NAME_APPLY_CREDIT_MEMOS)
private Boolean applyCreditMemos;
public static final String SERIALIZED_NAME_APPLY_UNAPPLIED_PAYMENTS = "apply_unapplied_payments";
@SerializedName(SERIALIZED_NAME_APPLY_UNAPPLIED_PAYMENTS)
private Boolean applyUnappliedPayments;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "bill_cycle_day";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private String billCycleDay;
public static final String SERIALIZED_NAME_BILL_RUN_ID = "bill_run_id";
@SerializedName(SERIALIZED_NAME_BILL_RUN_ID)
private String billRunId;
public static final String SERIALIZED_NAME_COLLECT_PAYMENT = "collect_payment";
@SerializedName(SERIALIZED_NAME_COLLECT_PAYMENT)
private Boolean collectPayment;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_CONSOLIDATED_PAYMENT = "consolidated_payment";
@SerializedName(SERIALIZED_NAME_CONSOLIDATED_PAYMENT)
private Boolean consolidatedPayment;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gateway_id";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public static final String SERIALIZED_NAME_PAYMENT_RUN_DATE = "payment_run_date";
@SerializedName(SERIALIZED_NAME_PAYMENT_RUN_DATE)
private OffsetDateTime paymentRunDate;
public static final String SERIALIZED_NAME_TARGET_DATE = "target_date";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public PaymentRunPatchRequest() {
}
public PaymentRunPatchRequest applyCreditMemos(Boolean applyCreditMemos) {
this.applyCreditMemos = applyCreditMemos;
return this;
}
/**
* If true, any posted credit memos are applied first.
* @return applyCreditMemos
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, any posted credit memos are applied first.")
public Boolean getApplyCreditMemos() {
return applyCreditMemos;
}
public void setApplyCreditMemos(Boolean applyCreditMemos) {
this.applyCreditMemos = applyCreditMemos;
}
public PaymentRunPatchRequest applyUnappliedPayments(Boolean applyUnappliedPayments) {
this.applyUnappliedPayments = applyUnappliedPayments;
return this;
}
/**
* If true, any unapplied payments are applied first.
* @return applyUnappliedPayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, any unapplied payments are applied first.")
public Boolean getApplyUnappliedPayments() {
return applyUnappliedPayments;
}
public void setApplyUnappliedPayments(Boolean applyUnappliedPayments) {
this.applyUnappliedPayments = applyUnappliedPayments;
}
public PaymentRunPatchRequest batch(String batch) {
this.batch = batch;
return this;
}
/**
* Identifier of the customer account batch to be included in this payment run.
* @return batch
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the customer account batch to be included in this payment run.")
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public PaymentRunPatchRequest billCycleDay(String billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* The day of the month to bill multiple customer accounts.
* @return billCycleDay
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The day of the month to bill multiple customer accounts.")
public String getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(String billCycleDay) {
this.billCycleDay = billCycleDay;
}
public PaymentRunPatchRequest billRunId(String billRunId) {
this.billRunId = billRunId;
return this;
}
/**
* The unique identifier of a bill run.
* @return billRunId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unique identifier of a bill run.")
public String getBillRunId() {
return billRunId;
}
public void setBillRunId(String billRunId) {
this.billRunId = billRunId;
}
public PaymentRunPatchRequest collectPayment(Boolean collectPayment) {
this.collectPayment = collectPayment;
return this;
}
/**
* Indicates whether to process electronic payments during the execution of payment runs. If the Payment user permission \"Process Electronic Payment\" is disabled, this field will be ignored.
* @return collectPayment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to process electronic payments during the execution of payment runs. If the Payment user permission \"Process Electronic Payment\" is disabled, this field will be ignored.")
public Boolean getCollectPayment() {
return collectPayment;
}
public void setCollectPayment(Boolean collectPayment) {
this.collectPayment = collectPayment;
}
public PaymentRunPatchRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* Three-letter ISO currency code.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USD", value = "Three-letter ISO currency code.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PaymentRunPatchRequest consolidatedPayment(Boolean consolidatedPayment) {
this.consolidatedPayment = consolidatedPayment;
return this;
}
/**
* If true, a single payment will be collected for all receivables due on an account.
* @return consolidatedPayment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, a single payment will be collected for all receivables due on an account.")
public Boolean getConsolidatedPayment() {
return consolidatedPayment;
}
public void setConsolidatedPayment(Boolean consolidatedPayment) {
this.consolidatedPayment = consolidatedPayment;
}
public PaymentRunPatchRequest gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* Unique identifier for the payment gateway.
* @return gatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the payment gateway.")
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
public PaymentRunPatchRequest paymentRunDate(OffsetDateTime paymentRunDate) {
this.paymentRunDate = paymentRunDate;
return this;
}
/**
* The date and time when the scheduled payment run is to be executed, in `yyyy-mm-dd hh:mm:ss` format. The backend will ignore minutes and seconds in the field value. For example, if you specify `2017-03-01 11:30:37` for this value, this payment run will be run at 2017-03-01 11:00:00. <br /> You must specify either the `payment_run_date` field or the `target_date` field in the request body. If you specify the `payment_run_date` field, the scheduced payment run is to be executed on the specified payment run date. If you specify the `target_date` field, the payment run is executed immediately after it is created.
* @return paymentRunDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the scheduled payment run is to be executed, in `yyyy-mm-dd hh:mm:ss` format. The backend will ignore minutes and seconds in the field value. For example, if you specify `2017-03-01 11:30:37` for this value, this payment run will be run at 2017-03-01 11:00:00.
You must specify either the `payment_run_date` field or the `target_date` field in the request body. If you specify the `payment_run_date` field, the scheduced payment run is to be executed on the specified payment run date. If you specify the `target_date` field, the payment run is executed immediately after it is created.")
public OffsetDateTime getPaymentRunDate() {
return paymentRunDate;
}
public void setPaymentRunDate(OffsetDateTime paymentRunDate) {
this.paymentRunDate = paymentRunDate;
}
public PaymentRunPatchRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date used to determine which receivables to be paid in the payment run. The payments are collected for all receivables with the due date no later than the target date.
* @return targetDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The target date used to determine which receivables to be paid in the payment run. The payments are collected for all receivables with the due date no later than the target date.")
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentRunPatchRequest paymentRunPatchRequest = (PaymentRunPatchRequest) o;
return Objects.equals(this.applyCreditMemos, paymentRunPatchRequest.applyCreditMemos) &&
Objects.equals(this.applyUnappliedPayments, paymentRunPatchRequest.applyUnappliedPayments) &&
Objects.equals(this.batch, paymentRunPatchRequest.batch) &&
Objects.equals(this.billCycleDay, paymentRunPatchRequest.billCycleDay) &&
Objects.equals(this.billRunId, paymentRunPatchRequest.billRunId) &&
Objects.equals(this.collectPayment, paymentRunPatchRequest.collectPayment) &&
Objects.equals(this.currency, paymentRunPatchRequest.currency) &&
Objects.equals(this.consolidatedPayment, paymentRunPatchRequest.consolidatedPayment) &&
Objects.equals(this.gatewayId, paymentRunPatchRequest.gatewayId) &&
Objects.equals(this.paymentRunDate, paymentRunPatchRequest.paymentRunDate) &&
Objects.equals(this.targetDate, paymentRunPatchRequest.targetDate);
}
@Override
public int hashCode() {
return Objects.hash(applyCreditMemos, applyUnappliedPayments, batch, billCycleDay, billRunId, collectPayment, currency, consolidatedPayment, gatewayId, paymentRunDate, targetDate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentRunPatchRequest {\n");
sb.append(" applyCreditMemos: ").append(toIndentedString(applyCreditMemos)).append("\n");
sb.append(" applyUnappliedPayments: ").append(toIndentedString(applyUnappliedPayments)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billRunId: ").append(toIndentedString(billRunId)).append("\n");
sb.append(" collectPayment: ").append(toIndentedString(collectPayment)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" consolidatedPayment: ").append(toIndentedString(consolidatedPayment)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append(" paymentRunDate: ").append(toIndentedString(paymentRunDate)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy