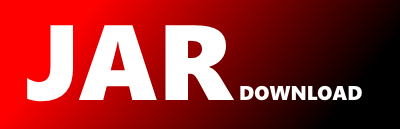
org.openapitools.client.model.PaymentRunSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PaymentRunSummary
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentRunSummary {
public static final String SERIALIZED_NAME_NUMBER_OF_ERRORS = "number_of_errors";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_ERRORS)
private Integer numberOfErrors;
public static final String SERIALIZED_NAME_NUMBER_OF_INVOICES = "number_of_invoices";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_INVOICES)
private Integer numberOfInvoices;
public static final String SERIALIZED_NAME_NUMBER_OF_PAYMENTS = "number_of_payments";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PAYMENTS)
private Integer numberOfPayments;
public static final String SERIALIZED_NAME_NUMBER_OF_CREDIT_MEMOS = "number_of_credit_memos";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_CREDIT_MEMOS)
private Integer numberOfCreditMemos;
public static final String SERIALIZED_NAME_NUMBER_OF_DEBIT_MEMOS = "number_of_debit_memos";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_DEBIT_MEMOS)
private Integer numberOfDebitMemos;
public static final String SERIALIZED_NAME_NUMBER_OF_UNPROCESSED_DEBIT_MEMOS = "number_of_unprocessed_debit_memos";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_UNPROCESSED_DEBIT_MEMOS)
private Integer numberOfUnprocessedDebitMemos;
public static final String SERIALIZED_NAME_NUMBER_OF_UNAPPLIED_PAYMENTS = "number_of_unapplied_payments";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_UNAPPLIED_PAYMENTS)
private Integer numberOfUnappliedPayments;
public static final String SERIALIZED_NAME_NUMBER_OF_UNPROCESSED_RECEIVABLES = "number_of_unprocessed_receivables";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_UNPROCESSED_RECEIVABLES)
private Integer numberOfUnprocessedReceivables;
public static final String SERIALIZED_NAME_ERRORS_TOTAL = "errors_total";
@SerializedName(SERIALIZED_NAME_ERRORS_TOTAL)
private Integer errorsTotal;
public static final String SERIALIZED_NAME_INVOICES_TOTAL = "invoices_total";
@SerializedName(SERIALIZED_NAME_INVOICES_TOTAL)
private Integer invoicesTotal;
public static final String SERIALIZED_NAME_PAYMENTS_TOTAL = "payments_total";
@SerializedName(SERIALIZED_NAME_PAYMENTS_TOTAL)
private Integer paymentsTotal;
public static final String SERIALIZED_NAME_UNPROCESSED_RECEIVABLES_TOTAL = "unprocessed_receivables_total";
@SerializedName(SERIALIZED_NAME_UNPROCESSED_RECEIVABLES_TOTAL)
private Integer unprocessedReceivablesTotal;
public PaymentRunSummary() {
}
public PaymentRunSummary numberOfErrors(Integer numberOfErrors) {
this.numberOfErrors = numberOfErrors;
return this;
}
/**
* The number of payments not processed.
* @return numberOfErrors
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of payments not processed.")
public Integer getNumberOfErrors() {
return numberOfErrors;
}
public void setNumberOfErrors(Integer numberOfErrors) {
this.numberOfErrors = numberOfErrors;
}
public PaymentRunSummary numberOfInvoices(Integer numberOfInvoices) {
this.numberOfInvoices = numberOfInvoices;
return this;
}
/**
* The number of invoices processed.
* @return numberOfInvoices
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of invoices processed.")
public Integer getNumberOfInvoices() {
return numberOfInvoices;
}
public void setNumberOfInvoices(Integer numberOfInvoices) {
this.numberOfInvoices = numberOfInvoices;
}
public PaymentRunSummary numberOfPayments(Integer numberOfPayments) {
this.numberOfPayments = numberOfPayments;
return this;
}
/**
* The number of payments processed.
* @return numberOfPayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of payments processed.")
public Integer getNumberOfPayments() {
return numberOfPayments;
}
public void setNumberOfPayments(Integer numberOfPayments) {
this.numberOfPayments = numberOfPayments;
}
public PaymentRunSummary numberOfCreditMemos(Integer numberOfCreditMemos) {
this.numberOfCreditMemos = numberOfCreditMemos;
return this;
}
/**
* The number of credit memos processed.
* @return numberOfCreditMemos
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of credit memos processed.")
public Integer getNumberOfCreditMemos() {
return numberOfCreditMemos;
}
public void setNumberOfCreditMemos(Integer numberOfCreditMemos) {
this.numberOfCreditMemos = numberOfCreditMemos;
}
public PaymentRunSummary numberOfDebitMemos(Integer numberOfDebitMemos) {
this.numberOfDebitMemos = numberOfDebitMemos;
return this;
}
/**
* The number of debit memos processed.
* @return numberOfDebitMemos
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of debit memos processed.")
public Integer getNumberOfDebitMemos() {
return numberOfDebitMemos;
}
public void setNumberOfDebitMemos(Integer numberOfDebitMemos) {
this.numberOfDebitMemos = numberOfDebitMemos;
}
public PaymentRunSummary numberOfUnprocessedDebitMemos(Integer numberOfUnprocessedDebitMemos) {
this.numberOfUnprocessedDebitMemos = numberOfUnprocessedDebitMemos;
return this;
}
/**
* The number of unprocessed debit memos.
* @return numberOfUnprocessedDebitMemos
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of unprocessed debit memos.")
public Integer getNumberOfUnprocessedDebitMemos() {
return numberOfUnprocessedDebitMemos;
}
public void setNumberOfUnprocessedDebitMemos(Integer numberOfUnprocessedDebitMemos) {
this.numberOfUnprocessedDebitMemos = numberOfUnprocessedDebitMemos;
}
public PaymentRunSummary numberOfUnappliedPayments(Integer numberOfUnappliedPayments) {
this.numberOfUnappliedPayments = numberOfUnappliedPayments;
return this;
}
/**
* The number of unapplied payments.
* @return numberOfUnappliedPayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of unapplied payments.")
public Integer getNumberOfUnappliedPayments() {
return numberOfUnappliedPayments;
}
public void setNumberOfUnappliedPayments(Integer numberOfUnappliedPayments) {
this.numberOfUnappliedPayments = numberOfUnappliedPayments;
}
public PaymentRunSummary numberOfUnprocessedReceivables(Integer numberOfUnprocessedReceivables) {
this.numberOfUnprocessedReceivables = numberOfUnprocessedReceivables;
return this;
}
/**
* The number of receivables with positive remamining balances.
* @return numberOfUnprocessedReceivables
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of receivables with positive remamining balances.")
public Integer getNumberOfUnprocessedReceivables() {
return numberOfUnprocessedReceivables;
}
public void setNumberOfUnprocessedReceivables(Integer numberOfUnprocessedReceivables) {
this.numberOfUnprocessedReceivables = numberOfUnprocessedReceivables;
}
public PaymentRunSummary errorsTotal(Integer errorsTotal) {
this.errorsTotal = errorsTotal;
return this;
}
/**
* The total number of all receivables with payments not processed.
* @return errorsTotal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total number of all receivables with payments not processed.")
public Integer getErrorsTotal() {
return errorsTotal;
}
public void setErrorsTotal(Integer errorsTotal) {
this.errorsTotal = errorsTotal;
}
public PaymentRunSummary invoicesTotal(Integer invoicesTotal) {
this.invoicesTotal = invoicesTotal;
return this;
}
/**
* The total number of all invoices.
* @return invoicesTotal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total number of all invoices.")
public Integer getInvoicesTotal() {
return invoicesTotal;
}
public void setInvoicesTotal(Integer invoicesTotal) {
this.invoicesTotal = invoicesTotal;
}
public PaymentRunSummary paymentsTotal(Integer paymentsTotal) {
this.paymentsTotal = paymentsTotal;
return this;
}
/**
* The total number of all processed and unapplied payments.
* @return paymentsTotal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total number of all processed and unapplied payments.")
public Integer getPaymentsTotal() {
return paymentsTotal;
}
public void setPaymentsTotal(Integer paymentsTotal) {
this.paymentsTotal = paymentsTotal;
}
public PaymentRunSummary unprocessedReceivablesTotal(Integer unprocessedReceivablesTotal) {
this.unprocessedReceivablesTotal = unprocessedReceivablesTotal;
return this;
}
/**
* The total number of positive remaining balances of all receivables.
* @return unprocessedReceivablesTotal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total number of positive remaining balances of all receivables.")
public Integer getUnprocessedReceivablesTotal() {
return unprocessedReceivablesTotal;
}
public void setUnprocessedReceivablesTotal(Integer unprocessedReceivablesTotal) {
this.unprocessedReceivablesTotal = unprocessedReceivablesTotal;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentRunSummary paymentRunSummary = (PaymentRunSummary) o;
return Objects.equals(this.numberOfErrors, paymentRunSummary.numberOfErrors) &&
Objects.equals(this.numberOfInvoices, paymentRunSummary.numberOfInvoices) &&
Objects.equals(this.numberOfPayments, paymentRunSummary.numberOfPayments) &&
Objects.equals(this.numberOfCreditMemos, paymentRunSummary.numberOfCreditMemos) &&
Objects.equals(this.numberOfDebitMemos, paymentRunSummary.numberOfDebitMemos) &&
Objects.equals(this.numberOfUnprocessedDebitMemos, paymentRunSummary.numberOfUnprocessedDebitMemos) &&
Objects.equals(this.numberOfUnappliedPayments, paymentRunSummary.numberOfUnappliedPayments) &&
Objects.equals(this.numberOfUnprocessedReceivables, paymentRunSummary.numberOfUnprocessedReceivables) &&
Objects.equals(this.errorsTotal, paymentRunSummary.errorsTotal) &&
Objects.equals(this.invoicesTotal, paymentRunSummary.invoicesTotal) &&
Objects.equals(this.paymentsTotal, paymentRunSummary.paymentsTotal) &&
Objects.equals(this.unprocessedReceivablesTotal, paymentRunSummary.unprocessedReceivablesTotal);
}
@Override
public int hashCode() {
return Objects.hash(numberOfErrors, numberOfInvoices, numberOfPayments, numberOfCreditMemos, numberOfDebitMemos, numberOfUnprocessedDebitMemos, numberOfUnappliedPayments, numberOfUnprocessedReceivables, errorsTotal, invoicesTotal, paymentsTotal, unprocessedReceivablesTotal);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentRunSummary {\n");
sb.append(" numberOfErrors: ").append(toIndentedString(numberOfErrors)).append("\n");
sb.append(" numberOfInvoices: ").append(toIndentedString(numberOfInvoices)).append("\n");
sb.append(" numberOfPayments: ").append(toIndentedString(numberOfPayments)).append("\n");
sb.append(" numberOfCreditMemos: ").append(toIndentedString(numberOfCreditMemos)).append("\n");
sb.append(" numberOfDebitMemos: ").append(toIndentedString(numberOfDebitMemos)).append("\n");
sb.append(" numberOfUnprocessedDebitMemos: ").append(toIndentedString(numberOfUnprocessedDebitMemos)).append("\n");
sb.append(" numberOfUnappliedPayments: ").append(toIndentedString(numberOfUnappliedPayments)).append("\n");
sb.append(" numberOfUnprocessedReceivables: ").append(toIndentedString(numberOfUnprocessedReceivables)).append("\n");
sb.append(" errorsTotal: ").append(toIndentedString(errorsTotal)).append("\n");
sb.append(" invoicesTotal: ").append(toIndentedString(invoicesTotal)).append("\n");
sb.append(" paymentsTotal: ").append(toIndentedString(paymentsTotal)).append("\n");
sb.append(" unprocessedReceivablesTotal: ").append(toIndentedString(unprocessedReceivablesTotal)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy