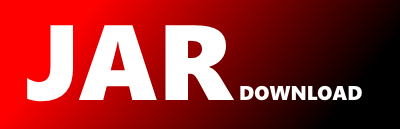
org.openapitools.client.model.PaymentScheduleCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.PaymentScheduleBillingDocumentRequest;
import org.openapitools.client.model.PaymentScheduleItemRequest;
import org.openapitools.client.model.PaymentSchedulePaymentOptionRequest;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PaymentScheduleCreateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentScheduleCreateRequest {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT = "billing_document";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT)
private PaymentScheduleBillingDocumentRequest billingDocument;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_ITEMS = "items";
@SerializedName(SERIALIZED_NAME_ITEMS)
private List items = null;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_ID = "payment_gateway_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_ID)
private String paymentGatewayId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER = "payment_gateway_number";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER)
private String paymentGatewayNumber;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_NUMBER = "payment_method_number";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_NUMBER)
private String paymentMethodNumber;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "payment_method_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PAYMENT_SCHEDULE_NUMBER = "payment_schedule_number";
@SerializedName(SERIALIZED_NAME_PAYMENT_SCHEDULE_NUMBER)
private String paymentScheduleNumber;
public static final String SERIALIZED_NAME_PAYMENT_OPTIONS = "payment_options";
@SerializedName(SERIALIZED_NAME_PAYMENT_OPTIONS)
private List paymentOptions = null;
public static final String SERIALIZED_NAME_STANDALONE = "standalone";
@SerializedName(SERIALIZED_NAME_STANDALONE)
private Boolean standalone;
public static final String SERIALIZED_NAME_START_DATE = "start_date";
@SerializedName(SERIALIZED_NAME_START_DATE)
private LocalDate startDate;
public static final String SERIALIZED_NAME_PREPAYMENT = "prepayment";
@SerializedName(SERIALIZED_NAME_PREPAYMENT)
private Boolean prepayment;
/**
* Unit in which term duration is defined. One of week or month.
*/
@JsonAdapter(PeriodEnum.Adapter.class)
public enum PeriodEnum {
WEEK("week"),
MONTH("month"),
BIWEEKLY("biweekly"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
PeriodEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PeriodEnum fromValue(String value) {
for (PeriodEnum b : PeriodEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PeriodEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PeriodEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PeriodEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PERIOD = "period";
@SerializedName(SERIALIZED_NAME_PERIOD)
private PeriodEnum period;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_TOTAL_AMOUNT = "total_amount";
@SerializedName(SERIALIZED_NAME_TOTAL_AMOUNT)
private BigDecimal totalAmount;
public static final String SERIALIZED_NAME_NUMBER_OF_PAYMENTS = "number_of_payments";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PAYMENTS)
private Integer numberOfPayments;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public PaymentScheduleCreateRequest() {
}
public PaymentScheduleCreateRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Unique identifier of the customer account the payment schedule belongs to.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the customer account the payment schedule belongs to.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public PaymentScheduleCreateRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Account number of the customer account the payment schedule belongs to.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Account number of the customer account the payment schedule belongs to.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public PaymentScheduleCreateRequest billingDocument(PaymentScheduleBillingDocumentRequest billingDocument) {
this.billingDocument = billingDocument;
return this;
}
/**
* The billing document with which the payment schedule is associated. Note: This field is optional. If you have the Standalone Payment feature enabled, you can leave this field blank and set standalone to true to create standalone payments. You can also choose to create unapplied payments by leaving this object blank and setting standalone to false. If Standalone Payment is not enabled, leaving this object unspecified will create unapplied payments.
* @return billingDocument
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing document with which the payment schedule is associated. Note: This field is optional. If you have the Standalone Payment feature enabled, you can leave this field blank and set standalone to true to create standalone payments. You can also choose to create unapplied payments by leaving this object blank and setting standalone to false. If Standalone Payment is not enabled, leaving this object unspecified will create unapplied payments.")
public PaymentScheduleBillingDocumentRequest getBillingDocument() {
return billingDocument;
}
public void setBillingDocument(PaymentScheduleBillingDocumentRequest billingDocument) {
this.billingDocument = billingDocument;
}
public PaymentScheduleCreateRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* Currency of the payment schedule. Note: This field is optional. The default value is the account's default currency. This field will be ignored when items is specified.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USD", value = "Currency of the payment schedule. Note: This field is optional. The default value is the account's default currency. This field will be ignored when items is specified.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PaymentScheduleCreateRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "description of test account", value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public PaymentScheduleCreateRequest items(List items) {
this.items = items;
return this;
}
public PaymentScheduleCreateRequest addItemsItem(PaymentScheduleItemRequest itemsItem) {
if (this.items == null) {
this.items = new ArrayList();
}
this.items.add(itemsItem);
return this;
}
/**
* Container for payment schedule items.
* @return items
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Container for payment schedule items.")
public List getItems() {
return items;
}
public void setItems(List items) {
this.items = items;
}
public PaymentScheduleCreateRequest paymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
return this;
}
/**
* ID of the payment gateway used to collect payments. Note: This field is optional. The default value is the account's default payment gateway ID. If no payment gateway ID is found on the customer account level, the default value will be the tenant's default payment gateway ID. This field will be ignored when items is specified.
* @return paymentGatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad093f27d6eee80017d6effd7a66759", value = "ID of the payment gateway used to collect payments. Note: This field is optional. The default value is the account's default payment gateway ID. If no payment gateway ID is found on the customer account level, the default value will be the tenant's default payment gateway ID. This field will be ignored when items is specified.")
public String getPaymentGatewayId() {
return paymentGatewayId;
}
public void setPaymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
}
public PaymentScheduleCreateRequest paymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
return this;
}
/**
* The payment gateway number of the payment gateway used to collect scheduled payments.
* @return paymentGatewayNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The payment gateway number of the payment gateway used to collect scheduled payments.")
public String getPaymentGatewayNumber() {
return paymentGatewayNumber;
}
public void setPaymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
}
public PaymentScheduleCreateRequest paymentMethodNumber(String paymentMethodNumber) {
this.paymentMethodNumber = paymentMethodNumber;
return this;
}
/**
* The payment method number of the payment method to be used to collect payments.
* @return paymentMethodNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The payment method number of the payment method to be used to collect payments.")
public String getPaymentMethodNumber() {
return paymentMethodNumber;
}
public void setPaymentMethodNumber(String paymentMethodNumber) {
this.paymentMethodNumber = paymentMethodNumber;
}
public PaymentScheduleCreateRequest paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* ID of the payment method. Note: This field is optional. The default value is the account's default payment method ID. This field will be ignored when items is specified.
* @return paymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8a95b1946b6aeac8718c32aab8c395f", value = "ID of the payment method. Note: This field is optional. The default value is the account's default payment method ID. This field will be ignored when items is specified.")
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public PaymentScheduleCreateRequest paymentScheduleNumber(String paymentScheduleNumber) {
this.paymentScheduleNumber = paymentScheduleNumber;
return this;
}
/**
* Number of the payment schedule.
* @return paymentScheduleNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Number of the payment schedule.")
public String getPaymentScheduleNumber() {
return paymentScheduleNumber;
}
public void setPaymentScheduleNumber(String paymentScheduleNumber) {
this.paymentScheduleNumber = paymentScheduleNumber;
}
public PaymentScheduleCreateRequest paymentOptions(List paymentOptions) {
this.paymentOptions = paymentOptions;
return this;
}
public PaymentScheduleCreateRequest addPaymentOptionsItem(PaymentSchedulePaymentOptionRequest paymentOptionsItem) {
if (this.paymentOptions == null) {
this.paymentOptions = new ArrayList();
}
this.paymentOptions.add(paymentOptionsItem);
return this;
}
/**
* Container for the payment options, which describe the transactional level rules for processing payments. Currently, only the gateway_options type is supported. Payment schedule payment_options take precedence over payment schedule item payment_options.
* @return paymentOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Container for the payment options, which describe the transactional level rules for processing payments. Currently, only the gateway_options type is supported. Payment schedule payment_options take precedence over payment schedule item payment_options.")
public List getPaymentOptions() {
return paymentOptions;
}
public void setPaymentOptions(List paymentOptions) {
this.paymentOptions = paymentOptions;
}
public PaymentScheduleCreateRequest standalone(Boolean standalone) {
this.standalone = standalone;
return this;
}
/**
* Indicates whether the payments created by the payment schedule are standalone payments or not. When setting to `true`, standalone payments will be created. When setting to `false`, you can either specify a billing document, or not specifying any billing documents. In the latter case, unapplied payments will be created. If set to `null`, standalone payments will be created. **Note**: This parameter is only available if standalone payments are enabled in your tenant. The default value is `true` if standalone payments are enabled in your tenant.
* @return standalone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether the payments created by the payment schedule are standalone payments or not. When setting to `true`, standalone payments will be created. When setting to `false`, you can either specify a billing document, or not specifying any billing documents. In the latter case, unapplied payments will be created. If set to `null`, standalone payments will be created. **Note**: This parameter is only available if standalone payments are enabled in your tenant. The default value is `true` if standalone payments are enabled in your tenant.")
public Boolean getStandalone() {
return standalone;
}
public void setStandalone(Boolean standalone) {
this.standalone = standalone;
}
public PaymentScheduleCreateRequest startDate(LocalDate startDate) {
this.startDate = startDate;
return this;
}
/**
* The date of the first scheduled payment collection. Note: This parameter is required when `items` is not specified. This parameter will be ignored when `items` is specified.
* @return startDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The date of the first scheduled payment collection. Note: This parameter is required when `items` is not specified. This parameter will be ignored when `items` is specified.")
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public PaymentScheduleCreateRequest prepayment(Boolean prepayment) {
this.prepayment = prepayment;
return this;
}
/**
* Indicates whether the payments created by the payment schedule will be used as reserved payments. This field will only be available if the prepaid cash drawdown permission is enabled. See <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_for_usage_or_prepaid_products/Advanced_Consumption_Billing/Prepaid_with_Drawdown\" target=\"_blank\">Prepaid Cash with Drawdown for more information.</a>
* @return prepayment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether the payments created by the payment schedule will be used as reserved payments. This field will only be available if the prepaid cash drawdown permission is enabled. See Prepaid Cash with Drawdown for more information.")
public Boolean getPrepayment() {
return prepayment;
}
public void setPrepayment(Boolean prepayment) {
this.prepayment = prepayment;
}
public PaymentScheduleCreateRequest period(PeriodEnum period) {
this.period = period;
return this;
}
/**
* Unit in which term duration is defined. One of week or month.
* @return period
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unit in which term duration is defined. One of week or month.")
public PeriodEnum getPeriod() {
return period;
}
public void setPeriod(PeriodEnum period) {
this.period = period;
}
public PaymentScheduleCreateRequest amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount of each payment schedule item in the payment schedule.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of each payment schedule item in the payment schedule.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public PaymentScheduleCreateRequest totalAmount(BigDecimal totalAmount) {
this.totalAmount = totalAmount;
return this;
}
/**
* The total amount to be collected by the payment schedule.
* @return totalAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount to be collected by the payment schedule.")
public BigDecimal getTotalAmount() {
return totalAmount;
}
public void setTotalAmount(BigDecimal totalAmount) {
this.totalAmount = totalAmount;
}
public PaymentScheduleCreateRequest numberOfPayments(Integer numberOfPayments) {
this.numberOfPayments = numberOfPayments;
return this;
}
/**
* The number of payment schedule items to be created for this payment schedule.
* @return numberOfPayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of payment schedule items to be created for this payment schedule.")
public Integer getNumberOfPayments() {
return numberOfPayments;
}
public void setNumberOfPayments(Integer numberOfPayments) {
this.numberOfPayments = numberOfPayments;
}
public PaymentScheduleCreateRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public PaymentScheduleCreateRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentScheduleCreateRequest paymentScheduleCreateRequest = (PaymentScheduleCreateRequest) o;
return Objects.equals(this.accountId, paymentScheduleCreateRequest.accountId) &&
Objects.equals(this.accountNumber, paymentScheduleCreateRequest.accountNumber) &&
Objects.equals(this.billingDocument, paymentScheduleCreateRequest.billingDocument) &&
Objects.equals(this.currency, paymentScheduleCreateRequest.currency) &&
Objects.equals(this.description, paymentScheduleCreateRequest.description) &&
Objects.equals(this.items, paymentScheduleCreateRequest.items) &&
Objects.equals(this.paymentGatewayId, paymentScheduleCreateRequest.paymentGatewayId) &&
Objects.equals(this.paymentGatewayNumber, paymentScheduleCreateRequest.paymentGatewayNumber) &&
Objects.equals(this.paymentMethodNumber, paymentScheduleCreateRequest.paymentMethodNumber) &&
Objects.equals(this.paymentMethodId, paymentScheduleCreateRequest.paymentMethodId) &&
Objects.equals(this.paymentScheduleNumber, paymentScheduleCreateRequest.paymentScheduleNumber) &&
Objects.equals(this.paymentOptions, paymentScheduleCreateRequest.paymentOptions) &&
Objects.equals(this.standalone, paymentScheduleCreateRequest.standalone) &&
Objects.equals(this.startDate, paymentScheduleCreateRequest.startDate) &&
Objects.equals(this.prepayment, paymentScheduleCreateRequest.prepayment) &&
Objects.equals(this.period, paymentScheduleCreateRequest.period) &&
Objects.equals(this.amount, paymentScheduleCreateRequest.amount) &&
Objects.equals(this.totalAmount, paymentScheduleCreateRequest.totalAmount) &&
Objects.equals(this.numberOfPayments, paymentScheduleCreateRequest.numberOfPayments) &&
Objects.equals(this.customFields, paymentScheduleCreateRequest.customFields);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(accountId, accountNumber, billingDocument, currency, description, items, paymentGatewayId, paymentGatewayNumber, paymentMethodNumber, paymentMethodId, paymentScheduleNumber, paymentOptions, standalone, startDate, prepayment, period, amount, totalAmount, numberOfPayments, customFields);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentScheduleCreateRequest {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" billingDocument: ").append(toIndentedString(billingDocument)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" items: ").append(toIndentedString(items)).append("\n");
sb.append(" paymentGatewayId: ").append(toIndentedString(paymentGatewayId)).append("\n");
sb.append(" paymentGatewayNumber: ").append(toIndentedString(paymentGatewayNumber)).append("\n");
sb.append(" paymentMethodNumber: ").append(toIndentedString(paymentMethodNumber)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" paymentScheduleNumber: ").append(toIndentedString(paymentScheduleNumber)).append("\n");
sb.append(" paymentOptions: ").append(toIndentedString(paymentOptions)).append("\n");
sb.append(" standalone: ").append(toIndentedString(standalone)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" prepayment: ").append(toIndentedString(prepayment)).append("\n");
sb.append(" period: ").append(toIndentedString(period)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" totalAmount: ").append(toIndentedString(totalAmount)).append("\n");
sb.append(" numberOfPayments: ").append(toIndentedString(numberOfPayments)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy