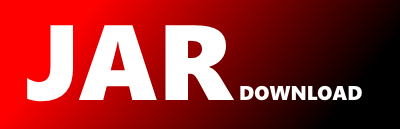
org.openapitools.client.model.PaymentScheduleItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.Payment;
import org.openapitools.client.model.PaymentSchedule;
import org.openapitools.client.model.PaymentScheduleBillingDocumentResponse;
import org.openapitools.client.model.PaymentScheduleItemPayments;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PaymentScheduleItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentScheduleItem {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT = "billing_document";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT)
private PaymentScheduleBillingDocumentResponse billingDocument;
public static final String SERIALIZED_NAME_CANCELLATION_REASON = "cancellation_reason";
@SerializedName(SERIALIZED_NAME_CANCELLATION_REASON)
private String cancellationReason;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_PAYMENT_SCHEDULE_ITEM_NUMBER = "payment_schedule_item_number";
@SerializedName(SERIALIZED_NAME_PAYMENT_SCHEDULE_ITEM_NUMBER)
private String paymentScheduleItemNumber;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_ID = "payment_gateway_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_ID)
private String paymentGatewayId;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "payment_method_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PAYMENT_OPTION_ID = "payment_option_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_OPTION_ID)
private String paymentOptionId;
public static final String SERIALIZED_NAME_PAYMENT_SCHEDULE_ID = "payment_schedule_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_SCHEDULE_ID)
private String paymentScheduleId;
public static final String SERIALIZED_NAME_PAYMENT_SCHEDULE_NUMBER = "payment_schedule_number";
@SerializedName(SERIALIZED_NAME_PAYMENT_SCHEDULE_NUMBER)
private String paymentScheduleNumber;
public static final String SERIALIZED_NAME_PAYMENTS = "payments";
@SerializedName(SERIALIZED_NAME_PAYMENTS)
private List payments = null;
public static final String SERIALIZED_NAME_RUN_HOUR = "run_hour";
@SerializedName(SERIALIZED_NAME_RUN_HOUR)
private Integer runHour;
public static final String SERIALIZED_NAME_SCHEDULED_DATE = "scheduled_date";
@SerializedName(SERIALIZED_NAME_SCHEDULED_DATE)
private LocalDate scheduledDate;
public static final String SERIALIZED_NAME_STANDALONE = "standalone";
@SerializedName(SERIALIZED_NAME_STANDALONE)
private Boolean standalone;
/**
* The status of the payment schedule item. active: there are unprocessed payment schedule items. canceled: the payment schedule has been canceled. complete: the payment schedule is complete and all items have been processed.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
PENDING("pending"),
CANCELED("canceled"),
COMPLETE("complete"),
ERROR("error"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_ERROR_MESSAGE = "error_message";
@SerializedName(SERIALIZED_NAME_ERROR_MESSAGE)
private String errorMessage;
public static final String SERIALIZED_NAME_PAYMENT = "payment";
@SerializedName(SERIALIZED_NAME_PAYMENT)
private Payment payment;
public static final String SERIALIZED_NAME_PAYMENT_SCHEDULE = "payment_schedule";
@SerializedName(SERIALIZED_NAME_PAYMENT_SCHEDULE)
private PaymentSchedule paymentSchedule;
public PaymentScheduleItem() {
}
public PaymentScheduleItem(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects,
Payment payment,
PaymentSchedule paymentSchedule
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
this.payment = payment;
this.paymentSchedule = paymentSchedule;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public PaymentScheduleItem customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public PaymentScheduleItem putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public PaymentScheduleItem accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Unique identifier of the customer account the payment schedule belongs to.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad093f27d6eee80017d6effd7a66759", value = "Unique identifier of the customer account the payment schedule belongs to.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public PaymentScheduleItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount to be collected by this payment schedule item.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount to be collected by this payment schedule item.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public PaymentScheduleItem balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* The remaining balance of payment schedule item.
* @return balance
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The remaining balance of payment schedule item.")
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public PaymentScheduleItem billingDocument(PaymentScheduleBillingDocumentResponse billingDocument) {
this.billingDocument = billingDocument;
return this;
}
/**
* The billing document with which the payment schedule is associated. If you have the Standalone Payment feature enabled, you can leave this field blank and set standalone to true to create standalone payments. You can also choose to create unapplied payments by leaving this object blank and setting standalone to false. If Standalone Payment is not enabled, leaving this object unspecified will create unapplied payments.
* @return billingDocument
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing document with which the payment schedule is associated. If you have the Standalone Payment feature enabled, you can leave this field blank and set standalone to true to create standalone payments. You can also choose to create unapplied payments by leaving this object blank and setting standalone to false. If Standalone Payment is not enabled, leaving this object unspecified will create unapplied payments.")
public PaymentScheduleBillingDocumentResponse getBillingDocument() {
return billingDocument;
}
public void setBillingDocument(PaymentScheduleBillingDocumentResponse billingDocument) {
this.billingDocument = billingDocument;
}
public PaymentScheduleItem cancellationReason(String cancellationReason) {
this.cancellationReason = cancellationReason;
return this;
}
/**
* The reason for the cancellation of payment schedule item.
* @return cancellationReason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The reason for the cancellation of payment schedule item.")
public String getCancellationReason() {
return cancellationReason;
}
public void setCancellationReason(String cancellationReason) {
this.cancellationReason = cancellationReason;
}
public PaymentScheduleItem currency(String currency) {
this.currency = currency;
return this;
}
/**
* Currency of the payment schedule. The default value is the account's default currency. This field will be ignored when items is specified.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USD", value = "Currency of the payment schedule. The default value is the account's default currency. This field will be ignored when items is specified.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PaymentScheduleItem description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "description of payment schedule item", value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public PaymentScheduleItem paymentScheduleItemNumber(String paymentScheduleItemNumber) {
this.paymentScheduleItemNumber = paymentScheduleItemNumber;
return this;
}
/**
* Number of the payment schedule item.
* @return paymentScheduleItemNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Number of the payment schedule item.")
public String getPaymentScheduleItemNumber() {
return paymentScheduleItemNumber;
}
public void setPaymentScheduleItemNumber(String paymentScheduleItemNumber) {
this.paymentScheduleItemNumber = paymentScheduleItemNumber;
}
public PaymentScheduleItem paymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
return this;
}
/**
* ID of the payment gateway used to collect payments. The default value is the account's default payment gateway ID. If no payment gateway ID is found on the customer account level, the default value will be the tenant's default payment gateway ID. This field will be ignored when `items` is specified.
* @return paymentGatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad093f27d6eee80017d6effd7a66759", value = "ID of the payment gateway used to collect payments. The default value is the account's default payment gateway ID. If no payment gateway ID is found on the customer account level, the default value will be the tenant's default payment gateway ID. This field will be ignored when `items` is specified.")
public String getPaymentGatewayId() {
return paymentGatewayId;
}
public void setPaymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
}
public PaymentScheduleItem paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* ID of the payment method. The default value is the account's default payment method ID. This field will be ignored when `items` is specified.
* @return paymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8a95b1946b6aeac8718c32aab8c395f", value = "ID of the payment method. The default value is the account's default payment method ID. This field will be ignored when `items` is specified.")
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public PaymentScheduleItem paymentOptionId(String paymentOptionId) {
this.paymentOptionId = paymentOptionId;
return this;
}
/**
* ID of the payment option.
* @return paymentOptionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "ID of the payment option.")
public String getPaymentOptionId() {
return paymentOptionId;
}
public void setPaymentOptionId(String paymentOptionId) {
this.paymentOptionId = paymentOptionId;
}
public PaymentScheduleItem paymentScheduleId(String paymentScheduleId) {
this.paymentScheduleId = paymentScheduleId;
return this;
}
/**
* ID of the payment schedule.
* @return paymentScheduleId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "ID of the payment schedule.")
public String getPaymentScheduleId() {
return paymentScheduleId;
}
public void setPaymentScheduleId(String paymentScheduleId) {
this.paymentScheduleId = paymentScheduleId;
}
public PaymentScheduleItem paymentScheduleNumber(String paymentScheduleNumber) {
this.paymentScheduleNumber = paymentScheduleNumber;
return this;
}
/**
* Number of the payment schedule.
* @return paymentScheduleNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Number of the payment schedule.")
public String getPaymentScheduleNumber() {
return paymentScheduleNumber;
}
public void setPaymentScheduleNumber(String paymentScheduleNumber) {
this.paymentScheduleNumber = paymentScheduleNumber;
}
public PaymentScheduleItem payments(List payments) {
this.payments = payments;
return this;
}
public PaymentScheduleItem addPaymentsItem(PaymentScheduleItemPayments paymentsItem) {
if (this.payments == null) {
this.payments = new ArrayList();
}
this.payments.add(paymentsItem);
return this;
}
/**
* Get payments
* @return payments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getPayments() {
return payments;
}
public void setPayments(List payments) {
this.payments = payments;
}
public PaymentScheduleItem runHour(Integer runHour) {
this.runHour = runHour;
return this;
}
/**
* At which hour in the day in the tenant's timezone this payment will be collected. Available values:[0,1,2,~,22,23]. If the time difference between your tenant’s timezone and the timezone where Zuora servers are located is not in full hours, for example, 2.5 hours, the payment schedule items will be triggered half an hour later than your scheduled time. The default value is 0. If the payment run_hour and scheduled_date are backdated, the system will collect the payment when the next run_hour occurs.
* minimum: 0
* maximum: 23
* @return runHour
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "At which hour in the day in the tenant's timezone this payment will be collected. Available values:[0,1,2,~,22,23]. If the time difference between your tenant’s timezone and the timezone where Zuora servers are located is not in full hours, for example, 2.5 hours, the payment schedule items will be triggered half an hour later than your scheduled time. The default value is 0. If the payment run_hour and scheduled_date are backdated, the system will collect the payment when the next run_hour occurs.")
public Integer getRunHour() {
return runHour;
}
public void setRunHour(Integer runHour) {
this.runHour = runHour;
}
public PaymentScheduleItem scheduledDate(LocalDate scheduledDate) {
this.scheduledDate = scheduledDate;
return this;
}
/**
* The scheduled date of collection.
* @return scheduledDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "The scheduled date of collection.")
public LocalDate getScheduledDate() {
return scheduledDate;
}
public void setScheduledDate(LocalDate scheduledDate) {
this.scheduledDate = scheduledDate;
}
public PaymentScheduleItem standalone(Boolean standalone) {
this.standalone = standalone;
return this;
}
/**
* Indicates whether the payments created by the payment schedule are standalone payments or not. When setting to true, standalone payments will be created. When setting to false, you can either specify a billing document, or not specifying any billing documents. In the later case, unapplied payments will be created. If set to null, standalone payments will be created. Note: This parameter is only available if standalone payments are enabled in your tenant. Do not include this parameter if standalone payment have not been enabled in your tenant. If standalone payments are enabled, the default value is true.
* @return standalone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether the payments created by the payment schedule are standalone payments or not. When setting to true, standalone payments will be created. When setting to false, you can either specify a billing document, or not specifying any billing documents. In the later case, unapplied payments will be created. If set to null, standalone payments will be created. Note: This parameter is only available if standalone payments are enabled in your tenant. Do not include this parameter if standalone payment have not been enabled in your tenant. If standalone payments are enabled, the default value is true.")
public Boolean getStandalone() {
return standalone;
}
public void setStandalone(Boolean standalone) {
this.standalone = standalone;
}
public PaymentScheduleItem state(StateEnum state) {
this.state = state;
return this;
}
/**
* The status of the payment schedule item. active: there are unprocessed payment schedule items. canceled: the payment schedule has been canceled. complete: the payment schedule is complete and all items have been processed.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The status of the payment schedule item. active: there are unprocessed payment schedule items. canceled: the payment schedule has been canceled. complete: the payment schedule is complete and all items have been processed.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public PaymentScheduleItem errorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
/**
* An error message indicating why payment collection failed for this payment schedule item.
* @return errorMessage
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An error message indicating why payment collection failed for this payment schedule item.")
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
/**
* List of customer payments.
* @return payment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of customer payments.")
public Payment getPayment() {
return payment;
}
/**
* Payment schedule record.
* @return paymentSchedule
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Payment schedule record.")
public PaymentSchedule getPaymentSchedule() {
return paymentSchedule;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentScheduleItem paymentScheduleItem = (PaymentScheduleItem) o;
return Objects.equals(this.id, paymentScheduleItem.id) &&
Objects.equals(this.updatedById, paymentScheduleItem.updatedById) &&
Objects.equals(this.updatedTime, paymentScheduleItem.updatedTime) &&
Objects.equals(this.createdById, paymentScheduleItem.createdById) &&
Objects.equals(this.createdTime, paymentScheduleItem.createdTime) &&
Objects.equals(this.customFields, paymentScheduleItem.customFields) &&
Objects.equals(this.customObjects, paymentScheduleItem.customObjects) &&
Objects.equals(this.accountId, paymentScheduleItem.accountId) &&
Objects.equals(this.amount, paymentScheduleItem.amount) &&
Objects.equals(this.balance, paymentScheduleItem.balance) &&
Objects.equals(this.billingDocument, paymentScheduleItem.billingDocument) &&
Objects.equals(this.cancellationReason, paymentScheduleItem.cancellationReason) &&
Objects.equals(this.currency, paymentScheduleItem.currency) &&
Objects.equals(this.description, paymentScheduleItem.description) &&
Objects.equals(this.paymentScheduleItemNumber, paymentScheduleItem.paymentScheduleItemNumber) &&
Objects.equals(this.paymentGatewayId, paymentScheduleItem.paymentGatewayId) &&
Objects.equals(this.paymentMethodId, paymentScheduleItem.paymentMethodId) &&
Objects.equals(this.paymentOptionId, paymentScheduleItem.paymentOptionId) &&
Objects.equals(this.paymentScheduleId, paymentScheduleItem.paymentScheduleId) &&
Objects.equals(this.paymentScheduleNumber, paymentScheduleItem.paymentScheduleNumber) &&
Objects.equals(this.payments, paymentScheduleItem.payments) &&
Objects.equals(this.runHour, paymentScheduleItem.runHour) &&
Objects.equals(this.scheduledDate, paymentScheduleItem.scheduledDate) &&
Objects.equals(this.standalone, paymentScheduleItem.standalone) &&
Objects.equals(this.state, paymentScheduleItem.state) &&
Objects.equals(this.errorMessage, paymentScheduleItem.errorMessage) &&
Objects.equals(this.payment, paymentScheduleItem.payment) &&
Objects.equals(this.paymentSchedule, paymentScheduleItem.paymentSchedule);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, accountId, amount, balance, billingDocument, cancellationReason, currency, description, paymentScheduleItemNumber, paymentGatewayId, paymentMethodId, paymentOptionId, paymentScheduleId, paymentScheduleNumber, payments, runHour, scheduledDate, standalone, state, errorMessage, payment, paymentSchedule);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentScheduleItem {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" billingDocument: ").append(toIndentedString(billingDocument)).append("\n");
sb.append(" cancellationReason: ").append(toIndentedString(cancellationReason)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" paymentScheduleItemNumber: ").append(toIndentedString(paymentScheduleItemNumber)).append("\n");
sb.append(" paymentGatewayId: ").append(toIndentedString(paymentGatewayId)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" paymentOptionId: ").append(toIndentedString(paymentOptionId)).append("\n");
sb.append(" paymentScheduleId: ").append(toIndentedString(paymentScheduleId)).append("\n");
sb.append(" paymentScheduleNumber: ").append(toIndentedString(paymentScheduleNumber)).append("\n");
sb.append(" payments: ").append(toIndentedString(payments)).append("\n");
sb.append(" runHour: ").append(toIndentedString(runHour)).append("\n");
sb.append(" scheduledDate: ").append(toIndentedString(scheduledDate)).append("\n");
sb.append(" standalone: ").append(toIndentedString(standalone)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" errorMessage: ").append(toIndentedString(errorMessage)).append("\n");
sb.append(" payment: ").append(toIndentedString(payment)).append("\n");
sb.append(" paymentSchedule: ").append(toIndentedString(paymentSchedule)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy