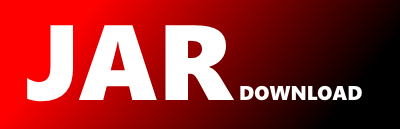
org.openapitools.client.model.PaymentScheduleItemRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PaymentScheduleItemRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentScheduleItemRequest {
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gateway_id";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "payment_method_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_RUN_HOUR = "run_hour";
@SerializedName(SERIALIZED_NAME_RUN_HOUR)
private Integer runHour;
public static final String SERIALIZED_NAME_SCHEDULED_DATE = "scheduled_date";
@SerializedName(SERIALIZED_NAME_SCHEDULED_DATE)
private LocalDate scheduledDate;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public PaymentScheduleItemRequest() {
}
public PaymentScheduleItemRequest amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount to be collected by this payment schedule item.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount to be collected by this payment schedule item.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public PaymentScheduleItemRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* Three-letter ISO currency code. Once the currency is set for an account, it cannot be updated.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USD", value = "Three-letter ISO currency code. Once the currency is set for an account, it cannot be updated.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PaymentScheduleItemRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "description of test account", value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public PaymentScheduleItemRequest gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* Get gatewayId
* @return gatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad093f27d6eee80017d6effd7a66759", value = "")
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
public PaymentScheduleItemRequest paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* Identifier of the payment method on the customer account.
* @return paymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8a95b1946b6aeac8718c32aab8c395f", value = "Identifier of the payment method on the customer account.")
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public PaymentScheduleItemRequest runHour(Integer runHour) {
this.runHour = runHour;
return this;
}
/**
* At which hour in the day in the tenant's timezone this payment will be collected. Available values:[0,1,2,~,22,23]. If the time difference between your tenant’s timezone and the timezone where Zuora servers are located is not in full hours, for example, 2.5 hours, the payment schedule items will be triggered half an hour later than your scheduled time. The default value is `0`. If the payment `run_hour` and `scheduled_date` are backdated, the system will collect the payment when the next `run_hour` occurs.
* minimum: 0
* maximum: 23
* @return runHour
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "At which hour in the day in the tenant's timezone this payment will be collected. Available values:[0,1,2,~,22,23]. If the time difference between your tenant’s timezone and the timezone where Zuora servers are located is not in full hours, for example, 2.5 hours, the payment schedule items will be triggered half an hour later than your scheduled time. The default value is `0`. If the payment `run_hour` and `scheduled_date` are backdated, the system will collect the payment when the next `run_hour` occurs.")
public Integer getRunHour() {
return runHour;
}
public void setRunHour(Integer runHour) {
this.runHour = runHour;
}
public PaymentScheduleItemRequest scheduledDate(LocalDate scheduledDate) {
this.scheduledDate = scheduledDate;
return this;
}
/**
* The scheduled date of the payment collection.
* @return scheduledDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "The scheduled date of the payment collection.")
public LocalDate getScheduledDate() {
return scheduledDate;
}
public void setScheduledDate(LocalDate scheduledDate) {
this.scheduledDate = scheduledDate;
}
public PaymentScheduleItemRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public PaymentScheduleItemRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentScheduleItemRequest paymentScheduleItemRequest = (PaymentScheduleItemRequest) o;
return Objects.equals(this.amount, paymentScheduleItemRequest.amount) &&
Objects.equals(this.currency, paymentScheduleItemRequest.currency) &&
Objects.equals(this.description, paymentScheduleItemRequest.description) &&
Objects.equals(this.gatewayId, paymentScheduleItemRequest.gatewayId) &&
Objects.equals(this.paymentMethodId, paymentScheduleItemRequest.paymentMethodId) &&
Objects.equals(this.runHour, paymentScheduleItemRequest.runHour) &&
Objects.equals(this.scheduledDate, paymentScheduleItemRequest.scheduledDate) &&
Objects.equals(this.customFields, paymentScheduleItemRequest.customFields);
}
@Override
public int hashCode() {
return Objects.hash(amount, currency, description, gatewayId, paymentMethodId, runHour, scheduledDate, customFields);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentScheduleItemRequest {\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" runHour: ").append(toIndentedString(runHour)).append("\n");
sb.append(" scheduledDate: ").append(toIndentedString(scheduledDate)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy