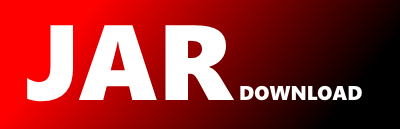
org.openapitools.client.model.PaymentSchedulePatchRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.PaymentSchedulePaymentOptionRequest;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PaymentSchedulePatchRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PaymentSchedulePatchRequest {
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_NUMBER_OF_PAYMENTS = "number_of_payments";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PAYMENTS)
private Integer numberOfPayments;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_ID = "payment_gateway_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_ID)
private String paymentGatewayId;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "payment_method_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_NUMBER = "payment_method_number";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_NUMBER)
private String paymentMethodNumber;
public static final String SERIALIZED_NAME_RUN_HOUR = "run_hour";
@SerializedName(SERIALIZED_NAME_RUN_HOUR)
private Integer runHour;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_PAYMENT_OPTIONS = "payment_options";
@SerializedName(SERIALIZED_NAME_PAYMENT_OPTIONS)
private List paymentOptions = null;
public static final String SERIALIZED_NAME_START_DATE = "start_date";
@SerializedName(SERIALIZED_NAME_START_DATE)
private LocalDate startDate;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
/**
* Unit in which term duration is defined. One of week or month.
*/
@JsonAdapter(PeriodEnum.Adapter.class)
public enum PeriodEnum {
WEEK("week"),
MONTH("month"),
BIWEEKLY("biweekly"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
PeriodEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PeriodEnum fromValue(String value) {
for (PeriodEnum b : PeriodEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PeriodEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PeriodEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PeriodEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PERIOD = "period";
@SerializedName(SERIALIZED_NAME_PERIOD)
private PeriodEnum period;
public PaymentSchedulePatchRequest() {
}
public PaymentSchedulePatchRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* Currency of the payment schedule. Note: This field is optional. The default value is the account's default currency. This field will be ignored when items is specified.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USD", value = "Currency of the payment schedule. Note: This field is optional. The default value is the account's default currency. This field will be ignored when items is specified.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PaymentSchedulePatchRequest numberOfPayments(Integer numberOfPayments) {
this.numberOfPayments = numberOfPayments;
return this;
}
/**
* The number of payment schedule items to be created for this payment schedule.
* @return numberOfPayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of payment schedule items to be created for this payment schedule.")
public Integer getNumberOfPayments() {
return numberOfPayments;
}
public void setNumberOfPayments(Integer numberOfPayments) {
this.numberOfPayments = numberOfPayments;
}
public PaymentSchedulePatchRequest paymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
return this;
}
/**
* ID of the payment gateway used to collect payments. Note: This field is optional. The default value is the account's default payment gateway ID. If no payment gateway ID is found on the customer account level, the default value will be the tenant's default payment gateway ID. This field will be ignored when items is specified.
* @return paymentGatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8ad093f27d6eee80017d6effd7a66759", value = "ID of the payment gateway used to collect payments. Note: This field is optional. The default value is the account's default payment gateway ID. If no payment gateway ID is found on the customer account level, the default value will be the tenant's default payment gateway ID. This field will be ignored when items is specified.")
public String getPaymentGatewayId() {
return paymentGatewayId;
}
public void setPaymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
}
public PaymentSchedulePatchRequest paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* ID of the payment method. Note: This field is optional. The default value is the account's default payment method ID. This field will be ignored when items is specified.
* @return paymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "8a95b1946b6aeac8718c32aab8c395f", value = "ID of the payment method. Note: This field is optional. The default value is the account's default payment method ID. This field will be ignored when items is specified.")
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public PaymentSchedulePatchRequest paymentMethodNumber(String paymentMethodNumber) {
this.paymentMethodNumber = paymentMethodNumber;
return this;
}
/**
* The payment method number of the payment method to be used to collect payments.
* @return paymentMethodNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The payment method number of the payment method to be used to collect payments.")
public String getPaymentMethodNumber() {
return paymentMethodNumber;
}
public void setPaymentMethodNumber(String paymentMethodNumber) {
this.paymentMethodNumber = paymentMethodNumber;
}
public PaymentSchedulePatchRequest runHour(Integer runHour) {
this.runHour = runHour;
return this;
}
/**
* At which hour in the day in the tenant's timezone this payment will be collected. Available values:[0,1,2,~,22,23]. If the time difference between your tenant’s timezone and the timezone where Zuora servers are located is not in full hours, for example, 2.5 hours, the payment schedule items will be triggered half an hour later than your scheduled time. The default value is `0`. If the payment `run_hour` and `scheduled_date` are backdated, the system will collect the payment when the next `run_hour` occurs.
* minimum: 0
* maximum: 23
* @return runHour
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "At which hour in the day in the tenant's timezone this payment will be collected. Available values:[0,1,2,~,22,23]. If the time difference between your tenant’s timezone and the timezone where Zuora servers are located is not in full hours, for example, 2.5 hours, the payment schedule items will be triggered half an hour later than your scheduled time. The default value is `0`. If the payment `run_hour` and `scheduled_date` are backdated, the system will collect the payment when the next `run_hour` occurs.")
public Integer getRunHour() {
return runHour;
}
public void setRunHour(Integer runHour) {
this.runHour = runHour;
}
public PaymentSchedulePatchRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public PaymentSchedulePatchRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public PaymentSchedulePatchRequest paymentOptions(List paymentOptions) {
this.paymentOptions = paymentOptions;
return this;
}
public PaymentSchedulePatchRequest addPaymentOptionsItem(PaymentSchedulePaymentOptionRequest paymentOptionsItem) {
if (this.paymentOptions == null) {
this.paymentOptions = new ArrayList();
}
this.paymentOptions.add(paymentOptionsItem);
return this;
}
/**
* Container for the payment options, which describe the transactional level rules for processing payments. Currently, only the gateway_options type is supported. Payment schedule payment_options take precedence over payment schedule item payment_options.
* @return paymentOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Container for the payment options, which describe the transactional level rules for processing payments. Currently, only the gateway_options type is supported. Payment schedule payment_options take precedence over payment schedule item payment_options.")
public List getPaymentOptions() {
return paymentOptions;
}
public void setPaymentOptions(List paymentOptions) {
this.paymentOptions = paymentOptions;
}
public PaymentSchedulePatchRequest startDate(LocalDate startDate) {
this.startDate = startDate;
return this;
}
/**
* The date of the first scheduled payment collection. Note: This parameter is required when `items` is not specified. This parameter will be ignored when `items` is specified.
* @return startDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The date of the first scheduled payment collection. Note: This parameter is required when `items` is not specified. This parameter will be ignored when `items` is specified.")
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public PaymentSchedulePatchRequest amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount of each payment schedule item in the payment schedule.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of each payment schedule item in the payment schedule.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public PaymentSchedulePatchRequest period(PeriodEnum period) {
this.period = period;
return this;
}
/**
* Unit in which term duration is defined. One of week or month.
* @return period
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unit in which term duration is defined. One of week or month.")
public PeriodEnum getPeriod() {
return period;
}
public void setPeriod(PeriodEnum period) {
this.period = period;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentSchedulePatchRequest paymentSchedulePatchRequest = (PaymentSchedulePatchRequest) o;
return Objects.equals(this.currency, paymentSchedulePatchRequest.currency) &&
Objects.equals(this.numberOfPayments, paymentSchedulePatchRequest.numberOfPayments) &&
Objects.equals(this.paymentGatewayId, paymentSchedulePatchRequest.paymentGatewayId) &&
Objects.equals(this.paymentMethodId, paymentSchedulePatchRequest.paymentMethodId) &&
Objects.equals(this.paymentMethodNumber, paymentSchedulePatchRequest.paymentMethodNumber) &&
Objects.equals(this.runHour, paymentSchedulePatchRequest.runHour) &&
Objects.equals(this.customFields, paymentSchedulePatchRequest.customFields) &&
Objects.equals(this.paymentOptions, paymentSchedulePatchRequest.paymentOptions) &&
Objects.equals(this.startDate, paymentSchedulePatchRequest.startDate) &&
Objects.equals(this.amount, paymentSchedulePatchRequest.amount) &&
Objects.equals(this.period, paymentSchedulePatchRequest.period);
}
@Override
public int hashCode() {
return Objects.hash(currency, numberOfPayments, paymentGatewayId, paymentMethodId, paymentMethodNumber, runHour, customFields, paymentOptions, startDate, amount, period);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentSchedulePatchRequest {\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" numberOfPayments: ").append(toIndentedString(numberOfPayments)).append("\n");
sb.append(" paymentGatewayId: ").append(toIndentedString(paymentGatewayId)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" paymentMethodNumber: ").append(toIndentedString(paymentMethodNumber)).append("\n");
sb.append(" runHour: ").append(toIndentedString(runHour)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" paymentOptions: ").append(toIndentedString(paymentOptions)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" period: ").append(toIndentedString(period)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy