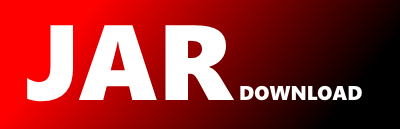
org.openapitools.client.model.PlanCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* PlanCreateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class PlanCreateRequest {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_START_DATE = "start_date";
@SerializedName(SERIALIZED_NAME_START_DATE)
private LocalDate startDate;
public static final String SERIALIZED_NAME_END_DATE = "end_date";
@SerializedName(SERIALIZED_NAME_END_DATE)
private LocalDate endDate;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_PLAN_NUMBER = "plan_number";
@SerializedName(SERIALIZED_NAME_PLAN_NUMBER)
private String planNumber;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_ACTIVE_CURRENCIES = "active_currencies";
@SerializedName(SERIALIZED_NAME_ACTIVE_CURRENCIES)
private List activeCurrencies = null;
public static final String SERIALIZED_NAME_PRODUCT_ID = "product_id";
@SerializedName(SERIALIZED_NAME_PRODUCT_ID)
private String productId;
public PlanCreateRequest() {
}
public PlanCreateRequest(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public PlanCreateRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public PlanCreateRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public PlanCreateRequest startDate(LocalDate startDate) {
this.startDate = startDate;
return this;
}
/**
* The date from which the plan can be used for new purchases.
* @return startDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date from which the plan can be used for new purchases.")
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public PlanCreateRequest endDate(LocalDate endDate) {
this.endDate = endDate;
return this;
}
/**
* The date on which the plan can no longer be used for new purchases.
* @return endDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date on which the plan can no longer be used for new purchases.")
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
public PlanCreateRequest name(String name) {
this.name = name;
return this;
}
/**
* The name of the plan.
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The name of the plan.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public PlanCreateRequest planNumber(String planNumber) {
this.planNumber = planNumber;
return this;
}
/**
* Human-readable identifier of the plan. It can be user-supplied.
* @return planNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the plan. It can be user-supplied.")
public String getPlanNumber() {
return planNumber;
}
public void setPlanNumber(String planNumber) {
this.planNumber = planNumber;
}
public PlanCreateRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public PlanCreateRequest activeCurrencies(List activeCurrencies) {
this.activeCurrencies = activeCurrencies;
return this;
}
public PlanCreateRequest addActiveCurrenciesItem(String activeCurrenciesItem) {
if (this.activeCurrencies == null) {
this.activeCurrencies = new ArrayList();
}
this.activeCurrencies.add(activeCurrenciesItem);
return this;
}
/**
* A list of 3-letter ISO-standard currency codes representing active currencies for the plan.
* @return activeCurrencies
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of 3-letter ISO-standard currency codes representing active currencies for the plan.")
public List getActiveCurrencies() {
return activeCurrencies;
}
public void setActiveCurrencies(List activeCurrencies) {
this.activeCurrencies = activeCurrencies;
}
public PlanCreateRequest productId(String productId) {
this.productId = productId;
return this;
}
/**
* Identifier of the product associated with this plan.
* @return productId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Identifier of the product associated with this plan.")
public String getProductId() {
return productId;
}
public void setProductId(String productId) {
this.productId = productId;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PlanCreateRequest planCreateRequest = (PlanCreateRequest) o;
return Objects.equals(this.id, planCreateRequest.id) &&
Objects.equals(this.updatedById, planCreateRequest.updatedById) &&
Objects.equals(this.updatedTime, planCreateRequest.updatedTime) &&
Objects.equals(this.createdById, planCreateRequest.createdById) &&
Objects.equals(this.createdTime, planCreateRequest.createdTime) &&
Objects.equals(this.customFields, planCreateRequest.customFields) &&
Objects.equals(this.customObjects, planCreateRequest.customObjects) &&
Objects.equals(this.startDate, planCreateRequest.startDate) &&
Objects.equals(this.endDate, planCreateRequest.endDate) &&
Objects.equals(this.name, planCreateRequest.name) &&
Objects.equals(this.planNumber, planCreateRequest.planNumber) &&
Objects.equals(this.description, planCreateRequest.description) &&
Objects.equals(this.activeCurrencies, planCreateRequest.activeCurrencies) &&
Objects.equals(this.productId, planCreateRequest.productId);
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, startDate, endDate, name, planNumber, description, activeCurrencies, productId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PlanCreateRequest {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" planNumber: ").append(toIndentedString(planNumber)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" activeCurrencies: ").append(toIndentedString(activeCurrencies)).append("\n");
sb.append(" productId: ").append(toIndentedString(productId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy