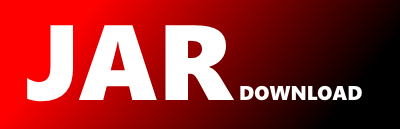
org.openapitools.client.model.Price Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.Drawdown;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.Overage;
import org.openapitools.client.model.Prepayment;
import org.openapitools.client.model.Recurring;
import org.openapitools.client.model.Revenue;
import org.openapitools.client.model.Tier;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Price information.
*/
@ApiModel(description = "Price information.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Price {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "recognized_revenue_accounting_code";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String recognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE = "deferred_revenue_accounting_code";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE)
private String deferredRevenueAccountingCode;
public static final String SERIALIZED_NAME_RECURRING = "recurring";
@SerializedName(SERIALIZED_NAME_RECURRING)
private Recurring recurring;
/**
* Specifies when to start billing your customer.
*/
@JsonAdapter(StartEventEnum.Adapter.class)
public enum StartEventEnum {
CONTRACT_EFFECTIVE("contract_effective"),
SERVICE_ACTIVATION("service_activation"),
CUSTOMER_ACCEPTANCE("customer_acceptance"),
SPECIFIC_DATE("specific_date"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StartEventEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StartEventEnum fromValue(String value) {
for (StartEventEnum b : StartEventEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StartEventEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StartEventEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StartEventEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_START_EVENT = "start_event";
@SerializedName(SERIALIZED_NAME_START_EVENT)
private StartEventEnum startEvent;
/**
* Specifies the mode for tiered prices.
*/
@JsonAdapter(TiersModeEnum.Adapter.class)
public enum TiersModeEnum {
GRADUATED("graduated"),
VOLUME("volume"),
HIGH_WATERMARK_VOLUME("high_watermark_volume"),
HIGH_WATERMARK_GRADUATED("high_watermark_graduated"),
GRADUATED_WITH_OVERAGE("graduated_with_overage"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TiersModeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TiersModeEnum fromValue(String value) {
for (TiersModeEnum b : TiersModeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TiersModeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TiersModeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TiersModeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TIERS_MODE = "tiers_mode";
@SerializedName(SERIALIZED_NAME_TIERS_MODE)
private TiersModeEnum tiersMode;
/**
* Gets or Sets applyDiscountTo
*/
@JsonAdapter(ApplyDiscountToEnum.Adapter.class)
public enum ApplyDiscountToEnum {
ONE_TIME("one_time"),
RECURRING("recurring"),
USAGE("usage"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ApplyDiscountToEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ApplyDiscountToEnum fromValue(String value) {
for (ApplyDiscountToEnum b : ApplyDiscountToEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ApplyDiscountToEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ApplyDiscountToEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ApplyDiscountToEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_APPLY_DISCOUNT_TO = "apply_discount_to";
@SerializedName(SERIALIZED_NAME_APPLY_DISCOUNT_TO)
private List applyDiscountTo = null;
public static final String SERIALIZED_NAME_TIERS = "tiers";
@SerializedName(SERIALIZED_NAME_TIERS)
private List tiers = null;
public static final String SERIALIZED_NAME_TAX_CODE = "tax_code";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_INCLUSIVE = "tax_inclusive";
@SerializedName(SERIALIZED_NAME_TAX_INCLUSIVE)
private Boolean taxInclusive;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unit_of_measure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_MIN_QUANTITY = "min_quantity";
@SerializedName(SERIALIZED_NAME_MIN_QUANTITY)
private BigDecimal minQuantity;
public static final String SERIALIZED_NAME_MAX_QUANTITY = "max_quantity";
@SerializedName(SERIALIZED_NAME_MAX_QUANTITY)
private BigDecimal maxQuantity;
/**
* Specifies at what level a discount should be applied: account, subscription, or plan.
*/
@JsonAdapter(DiscountLevelEnum.Adapter.class)
public enum DiscountLevelEnum {
ACCOUNT("account"),
SUBSCRIPTION("subscription"),
PLAN("plan"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
DiscountLevelEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DiscountLevelEnum fromValue(String value) {
for (DiscountLevelEnum b : DiscountLevelEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DiscountLevelEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DiscountLevelEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DiscountLevelEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DISCOUNT_LEVEL = "discount_level";
@SerializedName(SERIALIZED_NAME_DISCOUNT_LEVEL)
private DiscountLevelEnum discountLevel;
/**
* Determines when to recognize the revenue for this charge. You can choose to recognize upon invoicing or daily over time.
*/
@JsonAdapter(RevenueRecognitionRuleEnum.Adapter.class)
public enum RevenueRecognitionRuleEnum {
ON_INVOICE("on_invoice"),
DAILY_OVER_TIME("daily_over_time"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
RevenueRecognitionRuleEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static RevenueRecognitionRuleEnum fromValue(String value) {
for (RevenueRecognitionRuleEnum b : RevenueRecognitionRuleEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final RevenueRecognitionRuleEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public RevenueRecognitionRuleEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return RevenueRecognitionRuleEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE = "revenue_recognition_rule";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE)
private RevenueRecognitionRuleEnum revenueRecognitionRule;
public static final String SERIALIZED_NAME_STACKED_DISCOUNT = "stacked_discount";
@SerializedName(SERIALIZED_NAME_STACKED_DISCOUNT)
private Boolean stackedDiscount;
public static final String SERIALIZED_NAME_AMOUNTS = "amounts";
@SerializedName(SERIALIZED_NAME_AMOUNTS)
private Map amounts = null;
public static final String SERIALIZED_NAME_UNIT_AMOUNTS = "unit_amounts";
@SerializedName(SERIALIZED_NAME_UNIT_AMOUNTS)
private Map unitAmounts = null;
public static final String SERIALIZED_NAME_DISCOUNT_AMOUNTS = "discount_amounts";
@SerializedName(SERIALIZED_NAME_DISCOUNT_AMOUNTS)
private Map discountAmounts = null;
public static final String SERIALIZED_NAME_DISCOUNT_PERCENT = "discount_percent";
@SerializedName(SERIALIZED_NAME_DISCOUNT_PERCENT)
private BigDecimal discountPercent;
/**
* Specifies the base interval of a price. If not provided, this field defaults to `billing_period`.
*/
@JsonAdapter(PriceBaseIntervalEnum.Adapter.class)
public enum PriceBaseIntervalEnum {
MONTH("month"),
BILLING_PERIOD("billing_period"),
WEEK("week"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
PriceBaseIntervalEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PriceBaseIntervalEnum fromValue(String value) {
for (PriceBaseIntervalEnum b : PriceBaseIntervalEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PriceBaseIntervalEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PriceBaseIntervalEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PriceBaseIntervalEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PRICE_BASE_INTERVAL = "price_base_interval";
@SerializedName(SERIALIZED_NAME_PRICE_BASE_INTERVAL)
private PriceBaseIntervalEnum priceBaseInterval;
public static final String SERIALIZED_NAME_OVERAGE = "overage";
@SerializedName(SERIALIZED_NAME_OVERAGE)
private Overage overage;
public static final String SERIALIZED_NAME_REVENUE = "revenue";
@SerializedName(SERIALIZED_NAME_REVENUE)
private Revenue revenue;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accounting_code";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_PREPAYMENT = "prepayment";
@SerializedName(SERIALIZED_NAME_PREPAYMENT)
private Prepayment prepayment;
public static final String SERIALIZED_NAME_DRAWDOWN = "drawdown";
@SerializedName(SERIALIZED_NAME_DRAWDOWN)
private Drawdown drawdown;
public static final String SERIALIZED_NAME_TAXABLE = "taxable";
@SerializedName(SERIALIZED_NAME_TAXABLE)
private Boolean taxable;
public static final String SERIALIZED_NAME_PRICE_CHANGE_PERCENTAGE = "price_change_percentage";
@SerializedName(SERIALIZED_NAME_PRICE_CHANGE_PERCENTAGE)
private BigDecimal priceChangePercentage;
/**
* Applies an automatic price change when a termed subscription is renewed.
*/
@JsonAdapter(PriceChangeOptionEnum.Adapter.class)
public enum PriceChangeOptionEnum {
LATEST_CATALOG_PRICING("latest_catalog_pricing"),
PERCENTAGE("percentage"),
NONE("none"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
PriceChangeOptionEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PriceChangeOptionEnum fromValue(String value) {
for (PriceChangeOptionEnum b : PriceChangeOptionEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PriceChangeOptionEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PriceChangeOptionEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PriceChangeOptionEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PRICE_CHANGE_OPTION = "price_change_option";
@SerializedName(SERIALIZED_NAME_PRICE_CHANGE_OPTION)
private PriceChangeOptionEnum priceChangeOption;
public static final String SERIALIZED_NAME_PRICE_INCREASE_OPTION = "price_increase_option";
@SerializedName(SERIALIZED_NAME_PRICE_INCREASE_OPTION)
private Boolean priceIncreaseOption;
public static final String SERIALIZED_NAME_PLAN_ID = "plan_id";
@SerializedName(SERIALIZED_NAME_PLAN_ID)
private String planId;
public static final String SERIALIZED_NAME_PLAN_NUMBER = "plan_number";
@SerializedName(SERIALIZED_NAME_PLAN_NUMBER)
private String planNumber;
public static final String SERIALIZED_NAME_CUSTOM_FIELD_PER_UNIT_RATE = "custom_field_per_unit_rate";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELD_PER_UNIT_RATE)
private String customFieldPerUnitRate;
public static final String SERIALIZED_NAME_CUSTOM_FIELD_TOTAL_AMOUNT = "custom_field_total_amount";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELD_TOTAL_AMOUNT)
private String customFieldTotalAmount;
public static final String SERIALIZED_NAME_ACTIVE = "active";
@SerializedName(SERIALIZED_NAME_ACTIVE)
private Boolean active;
public static final String SERIALIZED_NAME_CHARGE_TYPE = "charge_type";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE)
private String chargeType;
public static final String SERIALIZED_NAME_CHARGE_MODEL = "charge_model";
@SerializedName(SERIALIZED_NAME_CHARGE_MODEL)
private String chargeModel;
public Price() {
}
public Price(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public Price customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public Price putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public Price name(String name) {
this.name = name;
return this;
}
/**
* The name of the price.
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the price.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Price description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Price recognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return recognizedRevenueAccountingCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getRecognizedRevenueAccountingCode() {
return recognizedRevenueAccountingCode;
}
public void setRecognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
}
public Price deferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
return this;
}
/**
* An active accounting code in your Zuora chart of accounts.
* @return deferredRevenueAccountingCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code in your Zuora chart of accounts.")
public String getDeferredRevenueAccountingCode() {
return deferredRevenueAccountingCode;
}
public void setDeferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
}
public Price recurring(Recurring recurring) {
this.recurring = recurring;
return this;
}
/**
* Get recurring
* @return recurring
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Recurring getRecurring() {
return recurring;
}
public void setRecurring(Recurring recurring) {
this.recurring = recurring;
}
public Price startEvent(StartEventEnum startEvent) {
this.startEvent = startEvent;
return this;
}
/**
* Specifies when to start billing your customer.
* @return startEvent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies when to start billing your customer.")
public StartEventEnum getStartEvent() {
return startEvent;
}
public void setStartEvent(StartEventEnum startEvent) {
this.startEvent = startEvent;
}
public Price tiersMode(TiersModeEnum tiersMode) {
this.tiersMode = tiersMode;
return this;
}
/**
* Specifies the mode for tiered prices.
* @return tiersMode
* @deprecated
**/
@Deprecated
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the mode for tiered prices.")
public TiersModeEnum getTiersMode() {
return tiersMode;
}
public void setTiersMode(TiersModeEnum tiersMode) {
this.tiersMode = tiersMode;
}
public Price applyDiscountTo(List applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
return this;
}
public Price addApplyDiscountToItem(ApplyDiscountToEnum applyDiscountToItem) {
if (this.applyDiscountTo == null) {
this.applyDiscountTo = new ArrayList();
}
this.applyDiscountTo.add(applyDiscountToItem);
return this;
}
/**
* Any combination of one_time, recurring and plan.
* @return applyDiscountTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Any combination of one_time, recurring and plan.")
public List getApplyDiscountTo() {
return applyDiscountTo;
}
public void setApplyDiscountTo(List applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
}
public Price tiers(List tiers) {
this.tiers = tiers;
return this;
}
public Price addTiersItem(Tier tiersItem) {
if (this.tiers == null) {
this.tiers = new ArrayList();
}
this.tiers.add(tiersItem);
return this;
}
/**
* Price information for different tiers. When creating or updating tiered prices, you must specify this field and the `tiers_mode` field.
* @return tiers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Price information for different tiers. When creating or updating tiered prices, you must specify this field and the `tiers_mode` field.")
public List getTiers() {
return tiers;
}
public void setTiers(List tiers) {
this.tiers = tiers;
}
public Price taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* A tax code identifier. If a `tax_code` of a price is not provided when you create or update a price, Zuora will treat the charged amount as non-taxable. If this code is provide, Zuora considers that this price is taxable and the charged amount will be handled accordingly.
* @return taxCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A tax code identifier. If a `tax_code` of a price is not provided when you create or update a price, Zuora will treat the charged amount as non-taxable. If this code is provide, Zuora considers that this price is taxable and the charged amount will be handled accordingly.")
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public Price taxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
return this;
}
/**
* If this field is set to `true`, it indicates that amounts are inclusive of tax.
* @return taxInclusive
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If this field is set to `true`, it indicates that amounts are inclusive of tax.")
public Boolean getTaxInclusive() {
return taxInclusive;
}
public void setTaxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
}
public Price unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* A configured unit of measure. This field is required for per-unit prices.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A configured unit of measure. This field is required for per-unit prices.")
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
public Price quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* Quantity of the product to which your customers subscribe.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Quantity of the product to which your customers subscribe.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public Price minQuantity(BigDecimal minQuantity) {
this.minQuantity = minQuantity;
return this;
}
/**
* The minimum quantity for a price. Specify this field and the `max_quantity` field to create a range of quantities allowed in a price.
* @return minQuantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The minimum quantity for a price. Specify this field and the `max_quantity` field to create a range of quantities allowed in a price.")
public BigDecimal getMinQuantity() {
return minQuantity;
}
public void setMinQuantity(BigDecimal minQuantity) {
this.minQuantity = minQuantity;
}
public Price maxQuantity(BigDecimal maxQuantity) {
this.maxQuantity = maxQuantity;
return this;
}
/**
* The maximum quantity for a price. Specify this field and the `min_quantity` field to create a range of quantities allowed in a price.
* @return maxQuantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The maximum quantity for a price. Specify this field and the `min_quantity` field to create a range of quantities allowed in a price.")
public BigDecimal getMaxQuantity() {
return maxQuantity;
}
public void setMaxQuantity(BigDecimal maxQuantity) {
this.maxQuantity = maxQuantity;
}
public Price discountLevel(DiscountLevelEnum discountLevel) {
this.discountLevel = discountLevel;
return this;
}
/**
* Specifies at what level a discount should be applied: account, subscription, or plan.
* @return discountLevel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies at what level a discount should be applied: account, subscription, or plan.")
public DiscountLevelEnum getDiscountLevel() {
return discountLevel;
}
public void setDiscountLevel(DiscountLevelEnum discountLevel) {
this.discountLevel = discountLevel;
}
public Price revenueRecognitionRule(RevenueRecognitionRuleEnum revenueRecognitionRule) {
this.revenueRecognitionRule = revenueRecognitionRule;
return this;
}
/**
* Determines when to recognize the revenue for this charge. You can choose to recognize upon invoicing or daily over time.
* @return revenueRecognitionRule
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Determines when to recognize the revenue for this charge. You can choose to recognize upon invoicing or daily over time.")
public RevenueRecognitionRuleEnum getRevenueRecognitionRule() {
return revenueRecognitionRule;
}
public void setRevenueRecognitionRule(RevenueRecognitionRuleEnum revenueRecognitionRule) {
this.revenueRecognitionRule = revenueRecognitionRule;
}
public Price stackedDiscount(Boolean stackedDiscount) {
this.stackedDiscount = stackedDiscount;
return this;
}
/**
* This field is only applicable for the Percentage Discount price. This field indicates whether the discount is to be calculated as stacked discount. Possible values are as follows: <ul> <li>`true`: This is a stacked discount, which should be calculated by stacking with other discounts.</li> <li> `false`: This is not a stacked discount, which should be calculated in sequence with other discounts.</li></ul> For more information, see <a href='https://knowledgecenter.zuora.com/Zuora_Billing/Products/Product_Catalog/B_Charge_Models/B_Discount_Charge_Models' target='_blank'>Stacked discounts</a>
* @return stackedDiscount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "This field is only applicable for the Percentage Discount price. This field indicates whether the discount is to be calculated as stacked discount. Possible values are as follows: - `true`: This is a stacked discount, which should be calculated by stacking with other discounts.
- `false`: This is not a stacked discount, which should be calculated in sequence with other discounts.
For more information, see Stacked discounts")
public Boolean getStackedDiscount() {
return stackedDiscount;
}
public void setStackedDiscount(Boolean stackedDiscount) {
this.stackedDiscount = stackedDiscount;
}
public Price amounts(Map amounts) {
this.amounts = amounts;
return this;
}
public Price putAmountsItem(String key, BigDecimal amountsItem) {
if (this.amounts == null) {
this.amounts = new HashMap();
}
this.amounts.put(key, amountsItem);
return this;
}
/**
* Get amounts
* @return amounts
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"USD\":10,\"GBP\":15}", value = "")
public Map getAmounts() {
return amounts;
}
public void setAmounts(Map amounts) {
this.amounts = amounts;
}
public Price unitAmounts(Map unitAmounts) {
this.unitAmounts = unitAmounts;
return this;
}
public Price putUnitAmountsItem(String key, BigDecimal unitAmountsItem) {
if (this.unitAmounts == null) {
this.unitAmounts = new HashMap();
}
this.unitAmounts.put(key, unitAmountsItem);
return this;
}
/**
* Get unitAmounts
* @return unitAmounts
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"USD\":10,\"GBP\":15}", value = "")
public Map getUnitAmounts() {
return unitAmounts;
}
public void setUnitAmounts(Map unitAmounts) {
this.unitAmounts = unitAmounts;
}
public Price discountAmounts(Map discountAmounts) {
this.discountAmounts = discountAmounts;
return this;
}
public Price putDiscountAmountsItem(String key, BigDecimal discountAmountsItem) {
if (this.discountAmounts == null) {
this.discountAmounts = new HashMap();
}
this.discountAmounts.put(key, discountAmountsItem);
return this;
}
/**
* Get discountAmounts
* @return discountAmounts
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"USD\":10,\"GBP\":15}", value = "")
public Map getDiscountAmounts() {
return discountAmounts;
}
public void setDiscountAmounts(Map discountAmounts) {
this.discountAmounts = discountAmounts;
}
public Price discountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
return this;
}
/**
* Discount percent. Specify this field if you offer a percentage-based discount.
* @return discountPercent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Discount percent. Specify this field if you offer a percentage-based discount.")
public BigDecimal getDiscountPercent() {
return discountPercent;
}
public void setDiscountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
}
public Price priceBaseInterval(PriceBaseIntervalEnum priceBaseInterval) {
this.priceBaseInterval = priceBaseInterval;
return this;
}
/**
* Specifies the base interval of a price. If not provided, this field defaults to `billing_period`.
* @return priceBaseInterval
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the base interval of a price. If not provided, this field defaults to `billing_period`.")
public PriceBaseIntervalEnum getPriceBaseInterval() {
return priceBaseInterval;
}
public void setPriceBaseInterval(PriceBaseIntervalEnum priceBaseInterval) {
this.priceBaseInterval = priceBaseInterval;
}
public Price overage(Overage overage) {
this.overage = overage;
return this;
}
/**
* Get overage
* @return overage
* @deprecated
**/
@Deprecated
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Overage getOverage() {
return overage;
}
public void setOverage(Overage overage) {
this.overage = overage;
}
public Price revenue(Revenue revenue) {
this.revenue = revenue;
return this;
}
/**
* Get revenue
* @return revenue
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Revenue getRevenue() {
return revenue;
}
public void setRevenue(Revenue revenue) {
this.revenue = revenue;
}
public Price accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* An active accounting code defined in **Finance Settings > Configure Accounting Codes** in your Zuora tenant.
* @return accountingCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active accounting code defined in **Finance Settings > Configure Accounting Codes** in your Zuora tenant.")
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public Price prepayment(Prepayment prepayment) {
this.prepayment = prepayment;
return this;
}
/**
* Get prepayment
* @return prepayment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Prepayment getPrepayment() {
return prepayment;
}
public void setPrepayment(Prepayment prepayment) {
this.prepayment = prepayment;
}
public Price drawdown(Drawdown drawdown) {
this.drawdown = drawdown;
return this;
}
/**
* Get drawdown
* @return drawdown
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Drawdown getDrawdown() {
return drawdown;
}
public void setDrawdown(Drawdown drawdown) {
this.drawdown = drawdown;
}
public Price taxable(Boolean taxable) {
this.taxable = taxable;
return this;
}
/**
* Get taxable
* @return taxable
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getTaxable() {
return taxable;
}
public void setTaxable(Boolean taxable) {
this.taxable = taxable;
}
public Price priceChangePercentage(BigDecimal priceChangePercentage) {
this.priceChangePercentage = priceChangePercentage;
return this;
}
/**
* The percentage to increase or decrease the price of a termed subscription's renewal.
* @return priceChangePercentage
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The percentage to increase or decrease the price of a termed subscription's renewal.")
public BigDecimal getPriceChangePercentage() {
return priceChangePercentage;
}
public void setPriceChangePercentage(BigDecimal priceChangePercentage) {
this.priceChangePercentage = priceChangePercentage;
}
public Price priceChangeOption(PriceChangeOptionEnum priceChangeOption) {
this.priceChangeOption = priceChangeOption;
return this;
}
/**
* Applies an automatic price change when a termed subscription is renewed.
* @return priceChangeOption
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Applies an automatic price change when a termed subscription is renewed.")
public PriceChangeOptionEnum getPriceChangeOption() {
return priceChangeOption;
}
public void setPriceChangeOption(PriceChangeOptionEnum priceChangeOption) {
this.priceChangeOption = priceChangeOption;
}
public Price priceIncreaseOption(Boolean priceIncreaseOption) {
this.priceIncreaseOption = priceIncreaseOption;
return this;
}
/**
* Indicates whether to apply an automatic price change when a termed subscription is renewed.
* @return priceIncreaseOption
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to apply an automatic price change when a termed subscription is renewed.")
public Boolean getPriceIncreaseOption() {
return priceIncreaseOption;
}
public void setPriceIncreaseOption(Boolean priceIncreaseOption) {
this.priceIncreaseOption = priceIncreaseOption;
}
public Price planId(String planId) {
this.planId = planId;
return this;
}
/**
* Specify the ID of a plan to which this price is associated.
* @return planId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify the ID of a plan to which this price is associated.")
public String getPlanId() {
return planId;
}
public void setPlanId(String planId) {
this.planId = planId;
}
public Price planNumber(String planNumber) {
this.planNumber = planNumber;
return this;
}
/**
* Specify the number of a plan to which this price is associated. This field is required if plan_id is not supplied
* @return planNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify the number of a plan to which this price is associated. This field is required if plan_id is not supplied")
public String getPlanNumber() {
return planNumber;
}
public void setPlanNumber(String planNumber) {
this.planNumber = planNumber;
}
public Price customFieldPerUnitRate(String customFieldPerUnitRate) {
this.customFieldPerUnitRate = customFieldPerUnitRate;
return this;
}
/**
* Name of the custom field that will be used to set a per unit rate under the `Pre-Rated Per Unit` charge model
* @return customFieldPerUnitRate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the custom field that will be used to set a per unit rate under the `Pre-Rated Per Unit` charge model ")
public String getCustomFieldPerUnitRate() {
return customFieldPerUnitRate;
}
public void setCustomFieldPerUnitRate(String customFieldPerUnitRate) {
this.customFieldPerUnitRate = customFieldPerUnitRate;
}
public Price customFieldTotalAmount(String customFieldTotalAmount) {
this.customFieldTotalAmount = customFieldTotalAmount;
return this;
}
/**
* Name of the custom field that will be used to set a total amount under the `Pre-Rated` charge model
* @return customFieldTotalAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the custom field that will be used to set a total amount under the `Pre-Rated` charge model ")
public String getCustomFieldTotalAmount() {
return customFieldTotalAmount;
}
public void setCustomFieldTotalAmount(String customFieldTotalAmount) {
this.customFieldTotalAmount = customFieldTotalAmount;
}
public Price active(Boolean active) {
this.active = active;
return this;
}
/**
* Whether the price can be used for new purchases.
* @return active
* @deprecated
**/
@Deprecated
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether the price can be used for new purchases.")
public Boolean getActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public Price chargeType(String chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* The type of charge. Can be `one_time`,`recurring`, or `usage`.
* @return chargeType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The type of charge. Can be `one_time`,`recurring`, or `usage`.")
public String getChargeType() {
return chargeType;
}
public void setChargeType(String chargeType) {
this.chargeType = chargeType;
}
public Price chargeModel(String chargeModel) {
this.chargeModel = chargeModel;
return this;
}
/**
* The charge model of the price, which determines how users are charged. Common charge models include flat fee, per-unit, volume, and tiered prices.
* @return chargeModel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The charge model of the price, which determines how users are charged. Common charge models include flat fee, per-unit, volume, and tiered prices.")
public String getChargeModel() {
return chargeModel;
}
public void setChargeModel(String chargeModel) {
this.chargeModel = chargeModel;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Price price = (Price) o;
return Objects.equals(this.id, price.id) &&
Objects.equals(this.updatedById, price.updatedById) &&
Objects.equals(this.updatedTime, price.updatedTime) &&
Objects.equals(this.createdById, price.createdById) &&
Objects.equals(this.createdTime, price.createdTime) &&
Objects.equals(this.customFields, price.customFields) &&
Objects.equals(this.customObjects, price.customObjects) &&
Objects.equals(this.name, price.name) &&
Objects.equals(this.description, price.description) &&
Objects.equals(this.recognizedRevenueAccountingCode, price.recognizedRevenueAccountingCode) &&
Objects.equals(this.deferredRevenueAccountingCode, price.deferredRevenueAccountingCode) &&
Objects.equals(this.recurring, price.recurring) &&
Objects.equals(this.startEvent, price.startEvent) &&
Objects.equals(this.tiersMode, price.tiersMode) &&
Objects.equals(this.applyDiscountTo, price.applyDiscountTo) &&
Objects.equals(this.tiers, price.tiers) &&
Objects.equals(this.taxCode, price.taxCode) &&
Objects.equals(this.taxInclusive, price.taxInclusive) &&
Objects.equals(this.unitOfMeasure, price.unitOfMeasure) &&
Objects.equals(this.quantity, price.quantity) &&
Objects.equals(this.minQuantity, price.minQuantity) &&
Objects.equals(this.maxQuantity, price.maxQuantity) &&
Objects.equals(this.discountLevel, price.discountLevel) &&
Objects.equals(this.revenueRecognitionRule, price.revenueRecognitionRule) &&
Objects.equals(this.stackedDiscount, price.stackedDiscount) &&
Objects.equals(this.amounts, price.amounts) &&
Objects.equals(this.unitAmounts, price.unitAmounts) &&
Objects.equals(this.discountAmounts, price.discountAmounts) &&
Objects.equals(this.discountPercent, price.discountPercent) &&
Objects.equals(this.priceBaseInterval, price.priceBaseInterval) &&
Objects.equals(this.overage, price.overage) &&
Objects.equals(this.revenue, price.revenue) &&
Objects.equals(this.accountingCode, price.accountingCode) &&
Objects.equals(this.prepayment, price.prepayment) &&
Objects.equals(this.drawdown, price.drawdown) &&
Objects.equals(this.taxable, price.taxable) &&
Objects.equals(this.priceChangePercentage, price.priceChangePercentage) &&
Objects.equals(this.priceChangeOption, price.priceChangeOption) &&
Objects.equals(this.priceIncreaseOption, price.priceIncreaseOption) &&
Objects.equals(this.planId, price.planId) &&
Objects.equals(this.planNumber, price.planNumber) &&
Objects.equals(this.customFieldPerUnitRate, price.customFieldPerUnitRate) &&
Objects.equals(this.customFieldTotalAmount, price.customFieldTotalAmount) &&
Objects.equals(this.active, price.active) &&
Objects.equals(this.chargeType, price.chargeType) &&
Objects.equals(this.chargeModel, price.chargeModel);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, name, description, recognizedRevenueAccountingCode, deferredRevenueAccountingCode, recurring, startEvent, tiersMode, applyDiscountTo, tiers, taxCode, taxInclusive, unitOfMeasure, quantity, minQuantity, maxQuantity, discountLevel, revenueRecognitionRule, stackedDiscount, amounts, unitAmounts, discountAmounts, discountPercent, priceBaseInterval, overage, revenue, accountingCode, prepayment, drawdown, taxable, priceChangePercentage, priceChangeOption, priceIncreaseOption, planId, planNumber, customFieldPerUnitRate, customFieldTotalAmount, active, chargeType, chargeModel);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Price {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" recognizedRevenueAccountingCode: ").append(toIndentedString(recognizedRevenueAccountingCode)).append("\n");
sb.append(" deferredRevenueAccountingCode: ").append(toIndentedString(deferredRevenueAccountingCode)).append("\n");
sb.append(" recurring: ").append(toIndentedString(recurring)).append("\n");
sb.append(" startEvent: ").append(toIndentedString(startEvent)).append("\n");
sb.append(" tiersMode: ").append(toIndentedString(tiersMode)).append("\n");
sb.append(" applyDiscountTo: ").append(toIndentedString(applyDiscountTo)).append("\n");
sb.append(" tiers: ").append(toIndentedString(tiers)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxInclusive: ").append(toIndentedString(taxInclusive)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" minQuantity: ").append(toIndentedString(minQuantity)).append("\n");
sb.append(" maxQuantity: ").append(toIndentedString(maxQuantity)).append("\n");
sb.append(" discountLevel: ").append(toIndentedString(discountLevel)).append("\n");
sb.append(" revenueRecognitionRule: ").append(toIndentedString(revenueRecognitionRule)).append("\n");
sb.append(" stackedDiscount: ").append(toIndentedString(stackedDiscount)).append("\n");
sb.append(" amounts: ").append(toIndentedString(amounts)).append("\n");
sb.append(" unitAmounts: ").append(toIndentedString(unitAmounts)).append("\n");
sb.append(" discountAmounts: ").append(toIndentedString(discountAmounts)).append("\n");
sb.append(" discountPercent: ").append(toIndentedString(discountPercent)).append("\n");
sb.append(" priceBaseInterval: ").append(toIndentedString(priceBaseInterval)).append("\n");
sb.append(" overage: ").append(toIndentedString(overage)).append("\n");
sb.append(" revenue: ").append(toIndentedString(revenue)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" prepayment: ").append(toIndentedString(prepayment)).append("\n");
sb.append(" drawdown: ").append(toIndentedString(drawdown)).append("\n");
sb.append(" taxable: ").append(toIndentedString(taxable)).append("\n");
sb.append(" priceChangePercentage: ").append(toIndentedString(priceChangePercentage)).append("\n");
sb.append(" priceChangeOption: ").append(toIndentedString(priceChangeOption)).append("\n");
sb.append(" priceIncreaseOption: ").append(toIndentedString(priceIncreaseOption)).append("\n");
sb.append(" planId: ").append(toIndentedString(planId)).append("\n");
sb.append(" planNumber: ").append(toIndentedString(planNumber)).append("\n");
sb.append(" customFieldPerUnitRate: ").append(toIndentedString(customFieldPerUnitRate)).append("\n");
sb.append(" customFieldTotalAmount: ").append(toIndentedString(customFieldTotalAmount)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" chargeModel: ").append(toIndentedString(chargeModel)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy