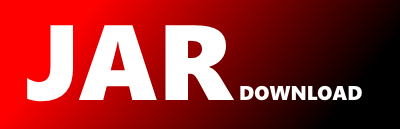
org.openapitools.client.model.QueryRun Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import org.openapitools.client.model.QueryRunFile;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Query run information.
*/
@ApiModel(description = "Query run information.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class QueryRun {
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_SQL = "sql";
@SerializedName(SERIALIZED_NAME_SQL)
private String sql;
public static final String SERIALIZED_NAME_REMAINING_ATTEMPTS = "remaining_attempts";
@SerializedName(SERIALIZED_NAME_REMAINING_ATTEMPTS)
private BigDecimal remainingAttempts;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_FILE = "file";
@SerializedName(SERIALIZED_NAME_FILE)
private QueryRunFile _file;
public static final String SERIALIZED_NAME_NUMBER_OF_ROWS = "number_of_rows";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_ROWS)
private BigDecimal numberOfRows;
public static final String SERIALIZED_NAME_PROCESSING_DURATION = "processing_duration";
@SerializedName(SERIALIZED_NAME_PROCESSING_DURATION)
private BigDecimal processingDuration;
/**
* The query's execution state, which will be completed for successful runs.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
PENDING("pending"),
ACCEPTED("accepted"),
IN_PROGRESS("in_progress"),
COMPLETE("complete"),
FAILED("failed"),
CANCELED("canceled"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_COLUMN_SEPARATOR = "column_separator";
@SerializedName(SERIALIZED_NAME_COLUMN_SEPARATOR)
private String columnSeparator;
public QueryRun() {
}
public QueryRun(
String createdById,
String id,
OffsetDateTime updatedTime
) {
this();
this.createdById = createdById;
this.id = id;
this.updatedTime = updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object.
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object.")
public String getCreatedById() {
return createdById;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
public QueryRun sql(String sql) {
this.sql = sql;
return this;
}
/**
* The SQL statement of the query.
* @return sql
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The SQL statement of the query.")
public String getSql() {
return sql;
}
public void setSql(String sql) {
this.sql = sql;
}
public QueryRun remainingAttempts(BigDecimal remainingAttempts) {
this.remainingAttempts = remainingAttempts;
return this;
}
/**
* The remaining number of times Zuora will attempt the query before terminating the query and setting the state to `failed`.
* @return remainingAttempts
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The remaining number of times Zuora will attempt the query before terminating the query and setting the state to `failed`.")
public BigDecimal getRemainingAttempts() {
return remainingAttempts;
}
public void setRemainingAttempts(BigDecimal remainingAttempts) {
this.remainingAttempts = remainingAttempts;
}
/**
* The date and time when the object was last updated in ISO-8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO-8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
public QueryRun _file(QueryRunFile _file) {
this._file = _file;
return this;
}
/**
* Get _file
* @return _file
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public QueryRunFile getFile() {
return _file;
}
public void setFile(QueryRunFile _file) {
this._file = _file;
}
public QueryRun numberOfRows(BigDecimal numberOfRows) {
this.numberOfRows = numberOfRows;
return this;
}
/**
* The number of rows in the output file.
* @return numberOfRows
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of rows in the output file.")
public BigDecimal getNumberOfRows() {
return numberOfRows;
}
public void setNumberOfRows(BigDecimal numberOfRows) {
this.numberOfRows = numberOfRows;
}
public QueryRun processingDuration(BigDecimal processingDuration) {
this.processingDuration = processingDuration;
return this;
}
/**
* The time taken to process the query in milliseconds.
* @return processingDuration
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The time taken to process the query in milliseconds.")
public BigDecimal getProcessingDuration() {
return processingDuration;
}
public void setProcessingDuration(BigDecimal processingDuration) {
this.processingDuration = processingDuration;
}
public QueryRun state(StateEnum state) {
this.state = state;
return this;
}
/**
* The query's execution state, which will be completed for successful runs.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The query's execution state, which will be completed for successful runs.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public QueryRun columnSeparator(String columnSeparator) {
this.columnSeparator = columnSeparator;
return this;
}
/**
* The character used as delimiter to separate values in the output file.
* @return columnSeparator
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The character used as delimiter to separate values in the output file.")
public String getColumnSeparator() {
return columnSeparator;
}
public void setColumnSeparator(String columnSeparator) {
this.columnSeparator = columnSeparator;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
QueryRun queryRun = (QueryRun) o;
return Objects.equals(this.createdById, queryRun.createdById) &&
Objects.equals(this.id, queryRun.id) &&
Objects.equals(this.sql, queryRun.sql) &&
Objects.equals(this.remainingAttempts, queryRun.remainingAttempts) &&
Objects.equals(this.updatedTime, queryRun.updatedTime) &&
Objects.equals(this._file, queryRun._file) &&
Objects.equals(this.numberOfRows, queryRun.numberOfRows) &&
Objects.equals(this.processingDuration, queryRun.processingDuration) &&
Objects.equals(this.state, queryRun.state) &&
Objects.equals(this.columnSeparator, queryRun.columnSeparator);
}
@Override
public int hashCode() {
return Objects.hash(createdById, id, sql, remainingAttempts, updatedTime, _file, numberOfRows, processingDuration, state, columnSeparator);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class QueryRun {\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" sql: ").append(toIndentedString(sql)).append("\n");
sb.append(" remainingAttempts: ").append(toIndentedString(remainingAttempts)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" _file: ").append(toIndentedString(_file)).append("\n");
sb.append(" numberOfRows: ").append(toIndentedString(numberOfRows)).append("\n");
sb.append(" processingDuration: ").append(toIndentedString(processingDuration)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" columnSeparator: ").append(toIndentedString(columnSeparator)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy