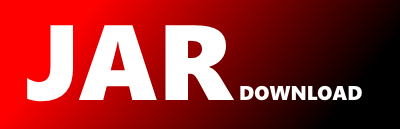
org.openapitools.client.model.Refund Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.Account;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.PaymentMethod;
import org.openapitools.client.model.RefundCreditMemoRequest;
import org.openapitools.client.model.RefundStateTransitions;
import org.openapitools.client.model.RefundsAppliedToResponse;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Refund
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Refund {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_GATEWAY_OPTIONS = "gateway_options";
@SerializedName(SERIALIZED_NAME_GATEWAY_OPTIONS)
private Map gatewayOptions = null;
public static final String SERIALIZED_NAME_REFUND_DATE = "refund_date";
@SerializedName(SERIALIZED_NAME_REFUND_DATE)
private LocalDate refundDate;
/**
* Gets or Sets refundMethodType
*/
@JsonAdapter(RefundMethodTypeEnum.Adapter.class)
public enum RefundMethodTypeEnum {
CASH("cash"),
CHECK("check"),
WIRE_TRANSFER("wire_transfer"),
PAY_PAL("pay_pal"),
CREDIT_CARD("credit_card"),
CC_REF("cc_ref"),
ACH_DEBIT("ach_debit"),
DEBIT_CARD("debit_card"),
OTHER("other"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
RefundMethodTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static RefundMethodTypeEnum fromValue(String value) {
for (RefundMethodTypeEnum b : RefundMethodTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final RefundMethodTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public RefundMethodTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return RefundMethodTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_REFUND_METHOD_TYPE = "refund_method_type";
@SerializedName(SERIALIZED_NAME_REFUND_METHOD_TYPE)
private RefundMethodTypeEnum refundMethodType;
public static final String SERIALIZED_NAME_PAYMENT_ID = "payment_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_ID)
private String paymentId;
public static final String SERIALIZED_NAME_REASON_CODE = "reason_code";
@SerializedName(SERIALIZED_NAME_REASON_CODE)
private String reasonCode;
public static final String SERIALIZED_NAME_STATEMENT_DESCRIPTOR = "statement_descriptor";
@SerializedName(SERIALIZED_NAME_STATEMENT_DESCRIPTOR)
private String statementDescriptor;
public static final String SERIALIZED_NAME_STATEMENT_DESCRIPTOR_PHONE = "statement_descriptor_phone";
@SerializedName(SERIALIZED_NAME_STATEMENT_DESCRIPTOR_PHONE)
private String statementDescriptorPhone;
public static final String SERIALIZED_NAME_EXTERNAL = "external";
@SerializedName(SERIALIZED_NAME_EXTERNAL)
private Boolean external;
public static final String SERIALIZED_NAME_REFERENCE_ID = "reference_id";
@SerializedName(SERIALIZED_NAME_REFERENCE_ID)
private String referenceId;
public static final String SERIALIZED_NAME_SECOND_REFERENCE_ID = "second_reference_id";
@SerializedName(SERIALIZED_NAME_SECOND_REFERENCE_ID)
private String secondReferenceId;
public static final String SERIALIZED_NAME_BANK_ACCOUNT_ACCOUNT = "bank_account_account";
@SerializedName(SERIALIZED_NAME_BANK_ACCOUNT_ACCOUNT)
private String bankAccountAccount;
public static final String SERIALIZED_NAME_ON_ACCOUNT_ACCOUNT = "on_account_account";
@SerializedName(SERIALIZED_NAME_ON_ACCOUNT_ACCOUNT)
private String onAccountAccount;
public static final String SERIALIZED_NAME_UNAPPLIED_PAYMENT_ACCOUNT = "unapplied_payment_account";
@SerializedName(SERIALIZED_NAME_UNAPPLIED_PAYMENT_ACCOUNT)
private String unappliedPaymentAccount;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "payment_method_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_CREDIT_MEMO = "credit_memo";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO)
private RefundCreditMemoRequest creditMemo;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private Account account;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gateway_id";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public static final String SERIALIZED_NAME_COMMENT = "comment";
@SerializedName(SERIALIZED_NAME_COMMENT)
private String comment;
public static final String SERIALIZED_NAME_GATEWAY_RESPONSE = "gateway_response";
@SerializedName(SERIALIZED_NAME_GATEWAY_RESPONSE)
private String gatewayResponse;
public static final String SERIALIZED_NAME_GATEWAY_RESPONSE_CODE = "gateway_response_code";
@SerializedName(SERIALIZED_NAME_GATEWAY_RESPONSE_CODE)
private String gatewayResponseCode;
/**
* The payment gateway state of the refund.
*/
@JsonAdapter(GatewayStateEnum.Adapter.class)
public enum GatewayStateEnum {
MARKED_FOR_SUBMISSION("marked_for_submission"),
SUBMITTED("submitted"),
SETTLED("settled"),
NOT_SUBMITTED("not_submitted"),
FAILED("failed"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
GatewayStateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static GatewayStateEnum fromValue(String value) {
for (GatewayStateEnum b : GatewayStateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final GatewayStateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public GatewayStateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return GatewayStateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_GATEWAY_STATE = "gateway_state";
@SerializedName(SERIALIZED_NAME_GATEWAY_STATE)
private GatewayStateEnum gatewayState;
public static final String SERIALIZED_NAME_PAYMENT_METHOD = "payment_method";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD)
private PaymentMethod paymentMethod;
public static final String SERIALIZED_NAME_REFUND_NUMBER = "refund_number";
@SerializedName(SERIALIZED_NAME_REFUND_NUMBER)
private String refundNumber;
public static final String SERIALIZED_NAME_STATE_TRANSITIONS = "state_transitions";
@SerializedName(SERIALIZED_NAME_STATE_TRANSITIONS)
private RefundStateTransitions stateTransitions;
/**
* The state of the refund.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
DRAFT("draft"),
POSTED("posted"),
PROCESSING("processing"),
PROCESSED("processed"),
ERROR("error"),
CANCELED("canceled"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_GATEWAY_RECONCILIATION_REASON = "gateway_reconciliation_reason";
@SerializedName(SERIALIZED_NAME_GATEWAY_RECONCILIATION_REASON)
private String gatewayReconciliationReason;
public static final String SERIALIZED_NAME_GATEWAY_RECONCILIATION_STATUS = "gateway_reconciliation_status";
@SerializedName(SERIALIZED_NAME_GATEWAY_RECONCILIATION_STATUS)
private String gatewayReconciliationStatus;
public static final String SERIALIZED_NAME_PAYOUT_ID = "payout_id";
@SerializedName(SERIALIZED_NAME_PAYOUT_ID)
private String payoutId;
public static final String SERIALIZED_NAME_APPLIED_TO = "applied_to";
@SerializedName(SERIALIZED_NAME_APPLIED_TO)
private List appliedTo = null;
public Refund() {
}
public Refund(
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects,
String paymentId,
String paymentMethodId,
String gatewayResponse,
String gatewayResponseCode,
GatewayStateEnum gatewayState,
StateEnum state,
String gatewayReconciliationReason,
String gatewayReconciliationStatus,
String payoutId,
List appliedTo
) {
this();
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
this.paymentId = paymentId;
this.paymentMethodId = paymentMethodId;
this.gatewayResponse = gatewayResponse;
this.gatewayResponseCode = gatewayResponseCode;
this.gatewayState = gatewayState;
this.state = state;
this.gatewayReconciliationReason = gatewayReconciliationReason;
this.gatewayReconciliationStatus = gatewayReconciliationStatus;
this.payoutId = payoutId;
this.appliedTo = appliedTo;
}
public Refund id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public Refund customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public Refund putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public Refund amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* Refund amount.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Refund amount.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public Refund description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Refund gatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
return this;
}
public Refund putGatewayOptionsItem(String key, String gatewayOptionsItem) {
if (this.gatewayOptions == null) {
this.gatewayOptions = new HashMap();
}
this.gatewayOptions.put(key, gatewayOptionsItem);
return this;
}
/**
* Get gatewayOptions
* @return gatewayOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"key\":\"value\"}", value = "")
public Map getGatewayOptions() {
return gatewayOptions;
}
public void setGatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
}
public Refund refundDate(LocalDate refundDate) {
this.refundDate = refundDate;
return this;
}
/**
* The date when the refund takes effect.
* @return refundDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date when the refund takes effect.")
public LocalDate getRefundDate() {
return refundDate;
}
public void setRefundDate(LocalDate refundDate) {
this.refundDate = refundDate;
}
public Refund refundMethodType(RefundMethodTypeEnum refundMethodType) {
this.refundMethodType = refundMethodType;
return this;
}
/**
* Get refundMethodType
* @return refundMethodType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public RefundMethodTypeEnum getRefundMethodType() {
return refundMethodType;
}
public void setRefundMethodType(RefundMethodTypeEnum refundMethodType) {
this.refundMethodType = refundMethodType;
}
/**
* Identifier for the payment, either `payment_number` or `payment_id.
* @return paymentId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier for the payment, either `payment_number` or `payment_id.")
public String getPaymentId() {
return paymentId;
}
public Refund reasonCode(String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* User-provided reason for the refund.
* @return reasonCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "User-provided reason for the refund.")
public String getReasonCode() {
return reasonCode;
}
public void setReasonCode(String reasonCode) {
this.reasonCode = reasonCode;
}
public Refund statementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
return this;
}
/**
* A payment gateway-specific field used by Orbital, Vantiv and Verifi.
* @return statementDescriptor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A payment gateway-specific field used by Orbital, Vantiv and Verifi.")
public String getStatementDescriptor() {
return statementDescriptor;
}
public void setStatementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
public Refund statementDescriptorPhone(String statementDescriptorPhone) {
this.statementDescriptorPhone = statementDescriptorPhone;
return this;
}
/**
* A payment gateway-specific field used by Orbital, Vantiv and Verifi.
* @return statementDescriptorPhone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A payment gateway-specific field used by Orbital, Vantiv and Verifi.")
public String getStatementDescriptorPhone() {
return statementDescriptorPhone;
}
public void setStatementDescriptorPhone(String statementDescriptorPhone) {
this.statementDescriptorPhone = statementDescriptorPhone;
}
public Refund external(Boolean external) {
this.external = external;
return this;
}
/**
* If true, indicates that this refund is not handled by Zuora.
* @return external
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, indicates that this refund is not handled by Zuora.")
public Boolean getExternal() {
return external;
}
public void setExternal(Boolean external) {
this.external = external;
}
public Refund referenceId(String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* Transaction identifier returned by the payment gateway. You may use this field to reconcile refunds between your payment gateway and Zuora Payments.
* @return referenceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Transaction identifier returned by the payment gateway. You may use this field to reconcile refunds between your payment gateway and Zuora Payments.")
public String getReferenceId() {
return referenceId;
}
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
public Refund secondReferenceId(String secondReferenceId) {
this.secondReferenceId = secondReferenceId;
return this;
}
/**
* A second transaction identifier returned by the payment gateway if there is an additional transaction for the refunds. You may use this field to reconcile payments between your payment gateway and Zuora Payments.
* @return secondReferenceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A second transaction identifier returned by the payment gateway if there is an additional transaction for the refunds. You may use this field to reconcile payments between your payment gateway and Zuora Payments.")
public String getSecondReferenceId() {
return secondReferenceId;
}
public void setSecondReferenceId(String secondReferenceId) {
this.secondReferenceId = secondReferenceId;
}
public Refund bankAccountAccount(String bankAccountAccount) {
this.bankAccountAccount = bankAccountAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return bankAccountAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getBankAccountAccount() {
return bankAccountAccount;
}
public void setBankAccountAccount(String bankAccountAccount) {
this.bankAccountAccount = bankAccountAccount;
}
public Refund onAccountAccount(String onAccountAccount) {
this.onAccountAccount = onAccountAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return onAccountAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getOnAccountAccount() {
return onAccountAccount;
}
public void setOnAccountAccount(String onAccountAccount) {
this.onAccountAccount = onAccountAccount;
}
public Refund unappliedPaymentAccount(String unappliedPaymentAccount) {
this.unappliedPaymentAccount = unappliedPaymentAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return unappliedPaymentAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getUnappliedPaymentAccount() {
return unappliedPaymentAccount;
}
public void setUnappliedPaymentAccount(String unappliedPaymentAccount) {
this.unappliedPaymentAccount = unappliedPaymentAccount;
}
/**
* Identifier of the payment method used to create this refund.
* @return paymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payment method used to create this refund.")
public String getPaymentMethodId() {
return paymentMethodId;
}
public Refund creditMemo(RefundCreditMemoRequest creditMemo) {
this.creditMemo = creditMemo;
return this;
}
/**
* The related credit memo.
* @return creditMemo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The related credit memo.")
public RefundCreditMemoRequest getCreditMemo() {
return creditMemo;
}
public void setCreditMemo(RefundCreditMemoRequest creditMemo) {
this.creditMemo = creditMemo;
}
public Refund accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the customer this refund is for, if one exists.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the customer this refund is for, if one exists.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public Refund account(Account account) {
this.account = account;
return this;
}
/**
* The account that owns the refund
* @return account
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The account that owns the refund")
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
public Refund gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* Identifier of the payment gateway that Zuora will use to authorize the payments that are made with this payment method. If you do not set this field, Zuora will use one of the following payment gateways instead: The default payment gateway of the customer account that owns the payment method, if the payment method is associated with a customer account or the default payment gateway of your Zuora tenant.
* @return gatewayId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payment gateway that Zuora will use to authorize the payments that are made with this payment method. If you do not set this field, Zuora will use one of the following payment gateways instead: The default payment gateway of the customer account that owns the payment method, if the payment method is associated with a customer account or the default payment gateway of your Zuora tenant.")
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
public Refund comment(String comment) {
this.comment = comment;
return this;
}
/**
* Comments about the refund.
* @return comment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Comments about the refund.")
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
/**
* Message returned by the payment gateway for this refund.
* @return gatewayResponse
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Message returned by the payment gateway for this refund.")
public String getGatewayResponse() {
return gatewayResponse;
}
/**
* Code returned by the payment gateway for this refund.
* @return gatewayResponseCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Code returned by the payment gateway for this refund.")
public String getGatewayResponseCode() {
return gatewayResponseCode;
}
/**
* The payment gateway state of the refund.
* @return gatewayState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The payment gateway state of the refund.")
public GatewayStateEnum getGatewayState() {
return gatewayState;
}
public Refund paymentMethod(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* Get paymentMethod
* @return paymentMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PaymentMethod getPaymentMethod() {
return paymentMethod;
}
public void setPaymentMethod(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
public Refund refundNumber(String refundNumber) {
this.refundNumber = refundNumber;
return this;
}
/**
* Human-readable identifier for this object; may be user-supplied.
* @return refundNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier for this object; may be user-supplied.")
public String getRefundNumber() {
return refundNumber;
}
public void setRefundNumber(String refundNumber) {
this.refundNumber = refundNumber;
}
public Refund stateTransitions(RefundStateTransitions stateTransitions) {
this.stateTransitions = stateTransitions;
return this;
}
/**
* Get stateTransitions
* @return stateTransitions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public RefundStateTransitions getStateTransitions() {
return stateTransitions;
}
public void setStateTransitions(RefundStateTransitions stateTransitions) {
this.stateTransitions = stateTransitions;
}
/**
* The state of the refund.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The state of the refund.")
public StateEnum getState() {
return state;
}
/**
* Gateway reconciliation reason.
* @return gatewayReconciliationReason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Gateway reconciliation reason.")
public String getGatewayReconciliationReason() {
return gatewayReconciliationReason;
}
/**
* Gateway reconciliation state.
* @return gatewayReconciliationStatus
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Gateway reconciliation state.")
public String getGatewayReconciliationStatus() {
return gatewayReconciliationStatus;
}
/**
* Identifier of the payout from the payment gateway.
* @return payoutId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payout from the payment gateway.")
public String getPayoutId() {
return payoutId;
}
/**
* Get appliedTo
* @return appliedTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getAppliedTo() {
return appliedTo;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Refund refund = (Refund) o;
return Objects.equals(this.id, refund.id) &&
Objects.equals(this.updatedById, refund.updatedById) &&
Objects.equals(this.updatedTime, refund.updatedTime) &&
Objects.equals(this.createdById, refund.createdById) &&
Objects.equals(this.createdTime, refund.createdTime) &&
Objects.equals(this.customFields, refund.customFields) &&
Objects.equals(this.customObjects, refund.customObjects) &&
Objects.equals(this.amount, refund.amount) &&
Objects.equals(this.description, refund.description) &&
Objects.equals(this.gatewayOptions, refund.gatewayOptions) &&
Objects.equals(this.refundDate, refund.refundDate) &&
Objects.equals(this.refundMethodType, refund.refundMethodType) &&
Objects.equals(this.paymentId, refund.paymentId) &&
Objects.equals(this.reasonCode, refund.reasonCode) &&
Objects.equals(this.statementDescriptor, refund.statementDescriptor) &&
Objects.equals(this.statementDescriptorPhone, refund.statementDescriptorPhone) &&
Objects.equals(this.external, refund.external) &&
Objects.equals(this.referenceId, refund.referenceId) &&
Objects.equals(this.secondReferenceId, refund.secondReferenceId) &&
Objects.equals(this.bankAccountAccount, refund.bankAccountAccount) &&
Objects.equals(this.onAccountAccount, refund.onAccountAccount) &&
Objects.equals(this.unappliedPaymentAccount, refund.unappliedPaymentAccount) &&
Objects.equals(this.paymentMethodId, refund.paymentMethodId) &&
Objects.equals(this.creditMemo, refund.creditMemo) &&
Objects.equals(this.accountId, refund.accountId) &&
Objects.equals(this.account, refund.account) &&
Objects.equals(this.gatewayId, refund.gatewayId) &&
Objects.equals(this.comment, refund.comment) &&
Objects.equals(this.gatewayResponse, refund.gatewayResponse) &&
Objects.equals(this.gatewayResponseCode, refund.gatewayResponseCode) &&
Objects.equals(this.gatewayState, refund.gatewayState) &&
Objects.equals(this.paymentMethod, refund.paymentMethod) &&
Objects.equals(this.refundNumber, refund.refundNumber) &&
Objects.equals(this.stateTransitions, refund.stateTransitions) &&
Objects.equals(this.state, refund.state) &&
Objects.equals(this.gatewayReconciliationReason, refund.gatewayReconciliationReason) &&
Objects.equals(this.gatewayReconciliationStatus, refund.gatewayReconciliationStatus) &&
Objects.equals(this.payoutId, refund.payoutId) &&
Objects.equals(this.appliedTo, refund.appliedTo);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, amount, description, gatewayOptions, refundDate, refundMethodType, paymentId, reasonCode, statementDescriptor, statementDescriptorPhone, external, referenceId, secondReferenceId, bankAccountAccount, onAccountAccount, unappliedPaymentAccount, paymentMethodId, creditMemo, accountId, account, gatewayId, comment, gatewayResponse, gatewayResponseCode, gatewayState, paymentMethod, refundNumber, stateTransitions, state, gatewayReconciliationReason, gatewayReconciliationStatus, payoutId, appliedTo);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Refund {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" gatewayOptions: ").append(toIndentedString(gatewayOptions)).append("\n");
sb.append(" refundDate: ").append(toIndentedString(refundDate)).append("\n");
sb.append(" refundMethodType: ").append(toIndentedString(refundMethodType)).append("\n");
sb.append(" paymentId: ").append(toIndentedString(paymentId)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" statementDescriptor: ").append(toIndentedString(statementDescriptor)).append("\n");
sb.append(" statementDescriptorPhone: ").append(toIndentedString(statementDescriptorPhone)).append("\n");
sb.append(" external: ").append(toIndentedString(external)).append("\n");
sb.append(" referenceId: ").append(toIndentedString(referenceId)).append("\n");
sb.append(" secondReferenceId: ").append(toIndentedString(secondReferenceId)).append("\n");
sb.append(" bankAccountAccount: ").append(toIndentedString(bankAccountAccount)).append("\n");
sb.append(" onAccountAccount: ").append(toIndentedString(onAccountAccount)).append("\n");
sb.append(" unappliedPaymentAccount: ").append(toIndentedString(unappliedPaymentAccount)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" creditMemo: ").append(toIndentedString(creditMemo)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append(" gatewayResponse: ").append(toIndentedString(gatewayResponse)).append("\n");
sb.append(" gatewayResponseCode: ").append(toIndentedString(gatewayResponseCode)).append("\n");
sb.append(" gatewayState: ").append(toIndentedString(gatewayState)).append("\n");
sb.append(" paymentMethod: ").append(toIndentedString(paymentMethod)).append("\n");
sb.append(" refundNumber: ").append(toIndentedString(refundNumber)).append("\n");
sb.append(" stateTransitions: ").append(toIndentedString(stateTransitions)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" gatewayReconciliationReason: ").append(toIndentedString(gatewayReconciliationReason)).append("\n");
sb.append(" gatewayReconciliationStatus: ").append(toIndentedString(gatewayReconciliationStatus)).append("\n");
sb.append(" payoutId: ").append(toIndentedString(payoutId)).append("\n");
sb.append(" appliedTo: ").append(toIndentedString(appliedTo)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy