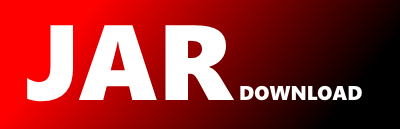
org.openapitools.client.model.RefundCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.BillingDocumentPaymentApplicationRequest;
import org.openapitools.client.model.RefundCreditMemoRequest;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* RefundCreateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class RefundCreateRequest {
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_GATEWAY_OPTIONS = "gateway_options";
@SerializedName(SERIALIZED_NAME_GATEWAY_OPTIONS)
private Map gatewayOptions = null;
public static final String SERIALIZED_NAME_REFUND_DATE = "refund_date";
@SerializedName(SERIALIZED_NAME_REFUND_DATE)
private LocalDate refundDate;
/**
* Gets or Sets refundMethodType
*/
@JsonAdapter(RefundMethodTypeEnum.Adapter.class)
public enum RefundMethodTypeEnum {
CASH("cash"),
CHECK("check"),
WIRE_TRANSFER("wire_transfer"),
PAY_PAL("pay_pal"),
CREDIT_CARD("credit_card"),
CC_REF("cc_ref"),
ACH_DEBIT("ach_debit"),
DEBIT_CARD("debit_card"),
OTHER("other"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
RefundMethodTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static RefundMethodTypeEnum fromValue(String value) {
for (RefundMethodTypeEnum b : RefundMethodTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final RefundMethodTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public RefundMethodTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return RefundMethodTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_REFUND_METHOD_TYPE = "refund_method_type";
@SerializedName(SERIALIZED_NAME_REFUND_METHOD_TYPE)
private RefundMethodTypeEnum refundMethodType;
public static final String SERIALIZED_NAME_PAYMENT_ID = "payment_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_ID)
private String paymentId;
public static final String SERIALIZED_NAME_REASON_CODE = "reason_code";
@SerializedName(SERIALIZED_NAME_REASON_CODE)
private String reasonCode;
public static final String SERIALIZED_NAME_STATEMENT_DESCRIPTOR = "statement_descriptor";
@SerializedName(SERIALIZED_NAME_STATEMENT_DESCRIPTOR)
private String statementDescriptor;
public static final String SERIALIZED_NAME_STATEMENT_DESCRIPTOR_PHONE = "statement_descriptor_phone";
@SerializedName(SERIALIZED_NAME_STATEMENT_DESCRIPTOR_PHONE)
private String statementDescriptorPhone;
public static final String SERIALIZED_NAME_EXTERNAL = "external";
@SerializedName(SERIALIZED_NAME_EXTERNAL)
private Boolean external;
public static final String SERIALIZED_NAME_REFERENCE_ID = "reference_id";
@SerializedName(SERIALIZED_NAME_REFERENCE_ID)
private String referenceId;
public static final String SERIALIZED_NAME_SECOND_REFERENCE_ID = "second_reference_id";
@SerializedName(SERIALIZED_NAME_SECOND_REFERENCE_ID)
private String secondReferenceId;
public static final String SERIALIZED_NAME_BANK_ACCOUNT_ACCOUNT = "bank_account_account";
@SerializedName(SERIALIZED_NAME_BANK_ACCOUNT_ACCOUNT)
private String bankAccountAccount;
public static final String SERIALIZED_NAME_ON_ACCOUNT_ACCOUNT = "on_account_account";
@SerializedName(SERIALIZED_NAME_ON_ACCOUNT_ACCOUNT)
private String onAccountAccount;
public static final String SERIALIZED_NAME_UNAPPLIED_PAYMENT_ACCOUNT = "unapplied_payment_account";
@SerializedName(SERIALIZED_NAME_UNAPPLIED_PAYMENT_ACCOUNT)
private String unappliedPaymentAccount;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "payment_method_id";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_CREDIT_MEMO = "credit_memo";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO)
private RefundCreditMemoRequest creditMemo;
public static final String SERIALIZED_NAME_BILLING_DOCUMENTS = "billing_documents";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENTS)
private List billingDocuments = null;
public RefundCreateRequest() {
}
public RefundCreateRequest(
String paymentId,
String paymentMethodId
) {
this();
this.paymentId = paymentId;
this.paymentMethodId = paymentMethodId;
}
public RefundCreateRequest amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* Refund amount.
* @return amount
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Refund amount.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public RefundCreateRequest description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public RefundCreateRequest gatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
return this;
}
public RefundCreateRequest putGatewayOptionsItem(String key, String gatewayOptionsItem) {
if (this.gatewayOptions == null) {
this.gatewayOptions = new HashMap();
}
this.gatewayOptions.put(key, gatewayOptionsItem);
return this;
}
/**
* Get gatewayOptions
* @return gatewayOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"key\":\"value\"}", value = "")
public Map getGatewayOptions() {
return gatewayOptions;
}
public void setGatewayOptions(Map gatewayOptions) {
this.gatewayOptions = gatewayOptions;
}
public RefundCreateRequest refundDate(LocalDate refundDate) {
this.refundDate = refundDate;
return this;
}
/**
* The date when the refund takes effect.
* @return refundDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date when the refund takes effect.")
public LocalDate getRefundDate() {
return refundDate;
}
public void setRefundDate(LocalDate refundDate) {
this.refundDate = refundDate;
}
public RefundCreateRequest refundMethodType(RefundMethodTypeEnum refundMethodType) {
this.refundMethodType = refundMethodType;
return this;
}
/**
* Get refundMethodType
* @return refundMethodType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public RefundMethodTypeEnum getRefundMethodType() {
return refundMethodType;
}
public void setRefundMethodType(RefundMethodTypeEnum refundMethodType) {
this.refundMethodType = refundMethodType;
}
/**
* Identifier for the payment, either `payment_number` or `payment_id.
* @return paymentId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier for the payment, either `payment_number` or `payment_id.")
public String getPaymentId() {
return paymentId;
}
public RefundCreateRequest reasonCode(String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* User-provided reason for the refund.
* @return reasonCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "User-provided reason for the refund.")
public String getReasonCode() {
return reasonCode;
}
public void setReasonCode(String reasonCode) {
this.reasonCode = reasonCode;
}
public RefundCreateRequest statementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
return this;
}
/**
* A payment gateway-specific field used by Orbital, Vantiv and Verifi.
* @return statementDescriptor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A payment gateway-specific field used by Orbital, Vantiv and Verifi.")
public String getStatementDescriptor() {
return statementDescriptor;
}
public void setStatementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
public RefundCreateRequest statementDescriptorPhone(String statementDescriptorPhone) {
this.statementDescriptorPhone = statementDescriptorPhone;
return this;
}
/**
* A payment gateway-specific field used by Orbital, Vantiv and Verifi.
* @return statementDescriptorPhone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A payment gateway-specific field used by Orbital, Vantiv and Verifi.")
public String getStatementDescriptorPhone() {
return statementDescriptorPhone;
}
public void setStatementDescriptorPhone(String statementDescriptorPhone) {
this.statementDescriptorPhone = statementDescriptorPhone;
}
public RefundCreateRequest external(Boolean external) {
this.external = external;
return this;
}
/**
* If true, indicates that this refund is not handled by Zuora.
* @return external
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, indicates that this refund is not handled by Zuora.")
public Boolean getExternal() {
return external;
}
public void setExternal(Boolean external) {
this.external = external;
}
public RefundCreateRequest referenceId(String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* Transaction identifier returned by the payment gateway. You may use this field to reconcile refunds between your payment gateway and Zuora Payments.
* @return referenceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Transaction identifier returned by the payment gateway. You may use this field to reconcile refunds between your payment gateway and Zuora Payments.")
public String getReferenceId() {
return referenceId;
}
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
public RefundCreateRequest secondReferenceId(String secondReferenceId) {
this.secondReferenceId = secondReferenceId;
return this;
}
/**
* A second transaction identifier returned by the payment gateway if there is an additional transaction for the refunds. You may use this field to reconcile payments between your payment gateway and Zuora Payments.
* @return secondReferenceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A second transaction identifier returned by the payment gateway if there is an additional transaction for the refunds. You may use this field to reconcile payments between your payment gateway and Zuora Payments.")
public String getSecondReferenceId() {
return secondReferenceId;
}
public void setSecondReferenceId(String secondReferenceId) {
this.secondReferenceId = secondReferenceId;
}
public RefundCreateRequest bankAccountAccount(String bankAccountAccount) {
this.bankAccountAccount = bankAccountAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return bankAccountAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getBankAccountAccount() {
return bankAccountAccount;
}
public void setBankAccountAccount(String bankAccountAccount) {
this.bankAccountAccount = bankAccountAccount;
}
public RefundCreateRequest onAccountAccount(String onAccountAccount) {
this.onAccountAccount = onAccountAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return onAccountAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getOnAccountAccount() {
return onAccountAccount;
}
public void setOnAccountAccount(String onAccountAccount) {
this.onAccountAccount = onAccountAccount;
}
public RefundCreateRequest unappliedPaymentAccount(String unappliedPaymentAccount) {
this.unappliedPaymentAccount = unappliedPaymentAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return unappliedPaymentAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getUnappliedPaymentAccount() {
return unappliedPaymentAccount;
}
public void setUnappliedPaymentAccount(String unappliedPaymentAccount) {
this.unappliedPaymentAccount = unappliedPaymentAccount;
}
public RefundCreateRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public RefundCreateRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* Identifier of the payment method used to create this refund.
* @return paymentMethodId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the payment method used to create this refund.")
public String getPaymentMethodId() {
return paymentMethodId;
}
public RefundCreateRequest creditMemo(RefundCreditMemoRequest creditMemo) {
this.creditMemo = creditMemo;
return this;
}
/**
* The related credit memo.
* @return creditMemo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The related credit memo.")
public RefundCreditMemoRequest getCreditMemo() {
return creditMemo;
}
public void setCreditMemo(RefundCreditMemoRequest creditMemo) {
this.creditMemo = creditMemo;
}
public RefundCreateRequest billingDocuments(List billingDocuments) {
this.billingDocuments = billingDocuments;
return this;
}
public RefundCreateRequest addBillingDocumentsItem(BillingDocumentPaymentApplicationRequest billingDocumentsItem) {
if (this.billingDocuments == null) {
this.billingDocuments = new ArrayList();
}
this.billingDocuments.add(billingDocumentsItem);
return this;
}
/**
* Indicates to which billing documents (invoices or debit memos) is the refund applied.
* @return billingDocuments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates to which billing documents (invoices or debit memos) is the refund applied.")
public List getBillingDocuments() {
return billingDocuments;
}
public void setBillingDocuments(List billingDocuments) {
this.billingDocuments = billingDocuments;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RefundCreateRequest refundCreateRequest = (RefundCreateRequest) o;
return Objects.equals(this.amount, refundCreateRequest.amount) &&
Objects.equals(this.description, refundCreateRequest.description) &&
Objects.equals(this.gatewayOptions, refundCreateRequest.gatewayOptions) &&
Objects.equals(this.refundDate, refundCreateRequest.refundDate) &&
Objects.equals(this.refundMethodType, refundCreateRequest.refundMethodType) &&
Objects.equals(this.paymentId, refundCreateRequest.paymentId) &&
Objects.equals(this.reasonCode, refundCreateRequest.reasonCode) &&
Objects.equals(this.statementDescriptor, refundCreateRequest.statementDescriptor) &&
Objects.equals(this.statementDescriptorPhone, refundCreateRequest.statementDescriptorPhone) &&
Objects.equals(this.external, refundCreateRequest.external) &&
Objects.equals(this.referenceId, refundCreateRequest.referenceId) &&
Objects.equals(this.secondReferenceId, refundCreateRequest.secondReferenceId) &&
Objects.equals(this.bankAccountAccount, refundCreateRequest.bankAccountAccount) &&
Objects.equals(this.onAccountAccount, refundCreateRequest.onAccountAccount) &&
Objects.equals(this.unappliedPaymentAccount, refundCreateRequest.unappliedPaymentAccount) &&
Objects.equals(this.customFields, refundCreateRequest.customFields) &&
Objects.equals(this.paymentMethodId, refundCreateRequest.paymentMethodId) &&
Objects.equals(this.creditMemo, refundCreateRequest.creditMemo) &&
Objects.equals(this.billingDocuments, refundCreateRequest.billingDocuments);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(amount, description, gatewayOptions, refundDate, refundMethodType, paymentId, reasonCode, statementDescriptor, statementDescriptorPhone, external, referenceId, secondReferenceId, bankAccountAccount, onAccountAccount, unappliedPaymentAccount, customFields, paymentMethodId, creditMemo, billingDocuments);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RefundCreateRequest {\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" gatewayOptions: ").append(toIndentedString(gatewayOptions)).append("\n");
sb.append(" refundDate: ").append(toIndentedString(refundDate)).append("\n");
sb.append(" refundMethodType: ").append(toIndentedString(refundMethodType)).append("\n");
sb.append(" paymentId: ").append(toIndentedString(paymentId)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" statementDescriptor: ").append(toIndentedString(statementDescriptor)).append("\n");
sb.append(" statementDescriptorPhone: ").append(toIndentedString(statementDescriptorPhone)).append("\n");
sb.append(" external: ").append(toIndentedString(external)).append("\n");
sb.append(" referenceId: ").append(toIndentedString(referenceId)).append("\n");
sb.append(" secondReferenceId: ").append(toIndentedString(secondReferenceId)).append("\n");
sb.append(" bankAccountAccount: ").append(toIndentedString(bankAccountAccount)).append("\n");
sb.append(" onAccountAccount: ").append(toIndentedString(onAccountAccount)).append("\n");
sb.append(" unappliedPaymentAccount: ").append(toIndentedString(unappliedPaymentAccount)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" creditMemo: ").append(toIndentedString(creditMemo)).append("\n");
sb.append(" billingDocuments: ").append(toIndentedString(billingDocuments)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy