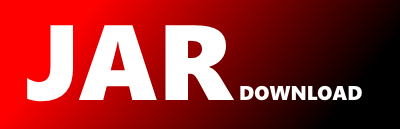
org.openapitools.client.model.RunWorkflowRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* RunWorkflowRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class RunWorkflowRequest {
public static final String SERIALIZED_NAME_INPUT_PARAMETERS = "input_parameters";
@SerializedName(SERIALIZED_NAME_INPUT_PARAMETERS)
private Map inputParameters = null;
public RunWorkflowRequest() {
}
public RunWorkflowRequest inputParameters(Map inputParameters) {
this.inputParameters = inputParameters;
return this;
}
public RunWorkflowRequest putInputParametersItem(String key, Object inputParametersItem) {
if (this.inputParameters == null) {
this.inputParameters = new HashMap();
}
this.inputParameters.put(key, inputParametersItem);
return this;
}
/**
* Include parameters that you want to pass to the workflow. For the parameters to be recognized and picked up by tasks in the workflow, you need to define the parameters first.
* @return inputParameters
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Include parameters that you want to pass to the workflow. For the parameters to be recognized and picked up by tasks in the workflow, you need to define the parameters first.")
public Map getInputParameters() {
return inputParameters;
}
public void setInputParameters(Map inputParameters) {
this.inputParameters = inputParameters;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RunWorkflowRequest runWorkflowRequest = (RunWorkflowRequest) o;
return Objects.equals(this.inputParameters, runWorkflowRequest.inputParameters);
}
@Override
public int hashCode() {
return Objects.hash(inputParameters);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RunWorkflowRequest {\n");
sb.append(" inputParameters: ").append(toIndentedString(inputParameters)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy