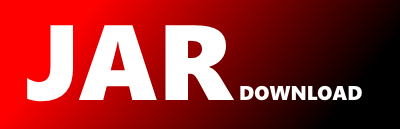
org.openapitools.client.model.StartOnResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Indicates on which date the subscription becomes effective.
*/
@ApiModel(description = "Indicates on which date the subscription becomes effective.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class StartOnResponse {
public static final String SERIALIZED_NAME_CONTRACT_EFFECTIVE = "contract_effective";
@SerializedName(SERIALIZED_NAME_CONTRACT_EFFECTIVE)
private LocalDate contractEffective;
public static final String SERIALIZED_NAME_SERVICE_ACTIVATION = "service_activation";
@SerializedName(SERIALIZED_NAME_SERVICE_ACTIVATION)
private LocalDate serviceActivation;
public static final String SERIALIZED_NAME_CUSTOMER_ACCEPTANCE = "customer_acceptance";
@SerializedName(SERIALIZED_NAME_CUSTOMER_ACCEPTANCE)
private LocalDate customerAcceptance;
public StartOnResponse() {
}
public StartOnResponse contractEffective(LocalDate contractEffective) {
this.contractEffective = contractEffective;
return this;
}
/**
* Effective contract date for this subscription, in the `yyyy-mm-dd` format.
* @return contractEffective
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Thu Jun 01 00:00:00 GMT 2023", value = "Effective contract date for this subscription, in the `yyyy-mm-dd` format.")
public LocalDate getContractEffective() {
return contractEffective;
}
public void setContractEffective(LocalDate contractEffective) {
this.contractEffective = contractEffective;
}
public StartOnResponse serviceActivation(LocalDate serviceActivation) {
this.serviceActivation = serviceActivation;
return this;
}
/**
* The date on which the services or products within a subscription have been activated and access has been provided to the customer, as the `yyyy-mm-dd` format. <ul> <li> If [Zuora is configured to require service activation](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Service_Activation_of_Orders.3F) and the `service_activation` field is not set for a `subscription_plans` order action or the \"Create a subscription\" operation, a `pending` order and/or a `pending_activation` subscription are created.</li> <li> If [Zuora is configured to require service activation](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Service_Activation_of_Orders.3F) and the `service_activation` field is not set for any of the following order actions or the \"Update a subscription\" operation, a `pending` order is created. The subscription status is not impacted. **Note**: This feature is in Limited Availability. If you want to have access to the feature, submit a request at [Zuora Global Support](https://support.zuora.com/). <ul> <li>`add_subscription_plans`</li> <li>`update_subscription_plans`</li> <li>`remove_subscription_plans`</li> <li>`renew`</li> <li>`terms`</li> </ul> </li> </ul>
* @return serviceActivation
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Thu Jun 01 00:00:00 GMT 2023", value = "The date on which the services or products within a subscription have been activated and access has been provided to the customer, as the `yyyy-mm-dd` format. - If [Zuora is configured to require service activation](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Service_Activation_of_Orders.3F) and the `service_activation` field is not set for a `subscription_plans` order action or the \"Create a subscription\" operation, a `pending` order and/or a `pending_activation` subscription are created.
- If [Zuora is configured to require service activation](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Service_Activation_of_Orders.3F) and the `service_activation` field is not set for any of the following order actions or the \"Update a subscription\" operation, a `pending` order is created. The subscription status is not impacted. **Note**: This feature is in Limited Availability. If you want to have access to the feature, submit a request at [Zuora Global Support](https://support.zuora.com/).
- `add_subscription_plans`
- `update_subscription_plans`
- `remove_subscription_plans`
- `renew`
- `terms`
")
public LocalDate getServiceActivation() {
return serviceActivation;
}
public void setServiceActivation(LocalDate serviceActivation) {
this.serviceActivation = serviceActivation;
}
public StartOnResponse customerAcceptance(LocalDate customerAcceptance) {
this.customerAcceptance = customerAcceptance;
return this;
}
/**
* The date on which the services or products within a subscription have been accepted by the customer, in the `yyyy-mm-dd` format. <ul> <li> If [Zuora is configured to require customer acceptance](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Customer_Acceptance_of_Orders.3F) and the `customer_acceptance` field is not set for a `subscription_plans` order action or the \"Create a subscription\" operation, a `pending` order and/or a `pending_acceptance` subscription are created. At the same time, if the service activation date field is also required and not set, a `pending` order and/or a `pending_activation` subscription are created instead.</li> <li> If [Zuora is configured to require customer acceptance](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Customer_Acceptance_of_Orders.3F) and the `customer_acceptance` field is not set for any of the following order actions or the \"Update a subscription\" operation, a `pending` order is created. The subscription status is not impacted. **Note**: This feature is in Limited Availability. If you want to have access to the feature, submit a request at [Zuora Global Support](https://support.zuora.com/). <ul> <li>`add_subscription_plans`</li> <li>`update_subscription_plans`</li> <li>`remove_subscription_plans`</li> <li>`renew`</li> <li>`terms`</li> </ul></li> </ul>
* @return customerAcceptance
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Thu Jun 01 00:00:00 GMT 2023", value = "The date on which the services or products within a subscription have been accepted by the customer, in the `yyyy-mm-dd` format. - If [Zuora is configured to require customer acceptance](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Customer_Acceptance_of_Orders.3F) and the `customer_acceptance` field is not set for a `subscription_plans` order action or the \"Create a subscription\" operation, a `pending` order and/or a `pending_acceptance` subscription are created. At the same time, if the service activation date field is also required and not set, a `pending` order and/or a `pending_activation` subscription are created instead.
- If [Zuora is configured to require customer acceptance](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Customer_Acceptance_of_Orders.3F) and the `customer_acceptance` field is not set for any of the following order actions or the \"Update a subscription\" operation, a `pending` order is created. The subscription status is not impacted. **Note**: This feature is in Limited Availability. If you want to have access to the feature, submit a request at [Zuora Global Support](https://support.zuora.com/).
- `add_subscription_plans`
- `update_subscription_plans`
- `remove_subscription_plans`
- `renew`
- `terms`
")
public LocalDate getCustomerAcceptance() {
return customerAcceptance;
}
public void setCustomerAcceptance(LocalDate customerAcceptance) {
this.customerAcceptance = customerAcceptance;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
StartOnResponse startOnResponse = (StartOnResponse) o;
return Objects.equals(this.contractEffective, startOnResponse.contractEffective) &&
Objects.equals(this.serviceActivation, startOnResponse.serviceActivation) &&
Objects.equals(this.customerAcceptance, startOnResponse.customerAcceptance);
}
@Override
public int hashCode() {
return Objects.hash(contractEffective, serviceActivation, customerAcceptance);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class StartOnResponse {\n");
sb.append(" contractEffective: ").append(toIndentedString(contractEffective)).append("\n");
sb.append(" serviceActivation: ").append(toIndentedString(serviceActivation)).append("\n");
sb.append(" customerAcceptance: ").append(toIndentedString(customerAcceptance)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy