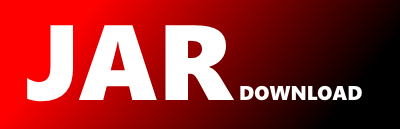
org.openapitools.client.model.Subscription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.Account;
import org.openapitools.client.model.Contact;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.FlexibleBillingDocumentSettings;
import org.openapitools.client.model.InvoiceItemListResponse;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.PrepaidBalance;
import org.openapitools.client.model.PrepaidBalances;
import org.openapitools.client.model.SubscriptionPlanListResponse;
import org.openapitools.client.model.Term;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Subscription information.
*/
@ApiModel(description = "Subscription information.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Subscription {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBER = "subscription_number";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBER)
private String subscriptionNumber;
/**
* Status of the subscription.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
DRAFT("draft"),
PENDING_ACTIVATION("pending_activation"),
PENDING_ACCEPTANCE("pending_acceptance"),
ACTIVE("active"),
EXPIRED("expired"),
CANCELED("canceled"),
PAUSED("paused"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
public static final String SERIALIZED_NAME_VERSION = "version";
@SerializedName(SERIALIZED_NAME_VERSION)
private Integer version;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private Account account;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID = "invoice_owner_account_id";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID)
private String invoiceOwnerAccountId;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT = "invoice_owner_account";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT)
private Account invoiceOwnerAccount;
public static final String SERIALIZED_NAME_AUTO_RENEW = "auto_renew";
@SerializedName(SERIALIZED_NAME_AUTO_RENEW)
private Boolean autoRenew;
public static final String SERIALIZED_NAME_LATEST_VERSION = "latest_version";
@SerializedName(SERIALIZED_NAME_LATEST_VERSION)
private Boolean latestVersion;
public static final String SERIALIZED_NAME_INITIAL_TERM = "initial_term";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM)
private Term initialTerm;
public static final String SERIALIZED_NAME_CURRENT_TERM = "current_term";
@SerializedName(SERIALIZED_NAME_CURRENT_TERM)
private Term currentTerm;
public static final String SERIALIZED_NAME_RENEWAL_TERM = "renewal_term";
@SerializedName(SERIALIZED_NAME_RENEWAL_TERM)
private Term renewalTerm;
public static final String SERIALIZED_NAME_START_DATE = "start_date";
@SerializedName(SERIALIZED_NAME_START_DATE)
private LocalDate startDate;
public static final String SERIALIZED_NAME_END_DATE = "end_date";
@SerializedName(SERIALIZED_NAME_END_DATE)
private LocalDate endDate;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_CONTRACT_EFFECTIVE = "contract_effective";
@SerializedName(SERIALIZED_NAME_CONTRACT_EFFECTIVE)
private LocalDate contractEffective;
public static final String SERIALIZED_NAME_SERVICE_ACTIVATION = "service_activation";
@SerializedName(SERIALIZED_NAME_SERVICE_ACTIVATION)
private LocalDate serviceActivation;
public static final String SERIALIZED_NAME_CUSTOMER_ACCEPTANCE = "customer_acceptance";
@SerializedName(SERIALIZED_NAME_CUSTOMER_ACCEPTANCE)
private LocalDate customerAcceptance;
public static final String SERIALIZED_NAME_INVOICE_SEPARATELY = "invoice_separately";
@SerializedName(SERIALIZED_NAME_INVOICE_SEPARATELY)
private Boolean invoiceSeparately;
public static final String SERIALIZED_NAME_ORDER_NUMBER = "order_number";
@SerializedName(SERIALIZED_NAME_ORDER_NUMBER)
private String orderNumber;
public static final String SERIALIZED_NAME_SUBSCRIPTION_PLANS = "subscription_plans";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_PLANS)
private SubscriptionPlanListResponse subscriptionPlans;
public static final String SERIALIZED_NAME_INVOICE_ITEMS = "invoice_items";
@SerializedName(SERIALIZED_NAME_INVOICE_ITEMS)
private InvoiceItemListResponse invoiceItems;
public static final String SERIALIZED_NAME_PREPAID_BALANCE = "prepaid_balance";
@SerializedName(SERIALIZED_NAME_PREPAID_BALANCE)
private List prepaidBalance = null;
public static final String SERIALIZED_NAME_PREPAID_BALANCES = "prepaid_balances";
@SerializedName(SERIALIZED_NAME_PREPAID_BALANCES)
private List prepaidBalances = null;
public static final String SERIALIZED_NAME_CONTRACTED_MRR = "contracted_mrr";
@SerializedName(SERIALIZED_NAME_CONTRACTED_MRR)
private String contractedMrr;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_CANCEL_REASON = "cancel_reason";
@SerializedName(SERIALIZED_NAME_CANCEL_REASON)
private String cancelReason;
public static final String SERIALIZED_NAME_LAST_BOOKING_DATE = "last_booking_date";
@SerializedName(SERIALIZED_NAME_LAST_BOOKING_DATE)
private LocalDate lastBookingDate;
public static final String SERIALIZED_NAME_BILL_TO_ID = "bill_to_id";
@SerializedName(SERIALIZED_NAME_BILL_TO_ID)
@JsonAdapter(NullableFieldAdapter.class)
private String billToId;
public static final String SERIALIZED_NAME_PAYMENT_TERMS = "payment_terms";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERMS)
@JsonAdapter(NullableFieldAdapter.class)
private String paymentTerms;
public static final String SERIALIZED_NAME_BILL_TO = "bill_to";
@SerializedName(SERIALIZED_NAME_BILL_TO)
private Contact billTo;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS = "billing_document_settings";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS)
private FlexibleBillingDocumentSettings billingDocumentSettings;
public static final String SERIALIZED_NAME_SOLD_TO_ID = "sold_to_id";
@SerializedName(SERIALIZED_NAME_SOLD_TO_ID)
@JsonAdapter(NullableFieldAdapter.class)
private String soldToId;
public static final String SERIALIZED_NAME_SOLD_TO = "sold_to";
@SerializedName(SERIALIZED_NAME_SOLD_TO)
private Contact soldTo;
public Subscription() {
}
public Subscription(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects,
SubscriptionPlanListResponse subscriptionPlans,
InvoiceItemListResponse invoiceItems,
List prepaidBalance,
List prepaidBalances,
Contact billTo,
Contact soldTo
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
this.subscriptionPlans = subscriptionPlans;
this.invoiceItems = invoiceItems;
this.prepaidBalance = prepaidBalance;
this.prepaidBalances = prepaidBalances;
this.billTo = billTo;
this.soldTo = soldTo;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public Subscription customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public Subscription putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public Subscription subscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
return this;
}
/**
* Human-readable identifier of the subscription. It can be user-supplied.
* @return subscriptionNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the subscription. It can be user-supplied.")
public String getSubscriptionNumber() {
return subscriptionNumber;
}
public void setSubscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
}
public Subscription state(StateEnum state) {
this.state = state;
return this;
}
/**
* Status of the subscription.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Status of the subscription.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public Subscription version(Integer version) {
this.version = version;
return this;
}
/**
* The version of the subscription. This version can be used in the `filter[]` query parameter to filter subscriptions.
* @return version
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The version of the subscription. This version can be used in the `filter[]` query parameter to filter subscriptions.")
public Integer getVersion() {
return version;
}
public void setVersion(Integer version) {
this.version = version;
}
public Subscription accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the account associated with this subscription.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account associated with this subscription.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public Subscription account(Account account) {
this.account = account;
return this;
}
/**
* Information of the new account associated with the subscription.
* @return account
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Information of the new account associated with the subscription.")
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
public Subscription invoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
return this;
}
/**
* Identifier of the account that owns the invoice associated with this subscription.
* @return invoiceOwnerAccountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the invoice associated with this subscription.")
public String getInvoiceOwnerAccountId() {
return invoiceOwnerAccountId;
}
public void setInvoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
}
public Subscription invoiceOwnerAccount(Account invoiceOwnerAccount) {
this.invoiceOwnerAccount = invoiceOwnerAccount;
return this;
}
/**
* Identifier of the account that owns the subscription.
* @return invoiceOwnerAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the subscription.")
public Account getInvoiceOwnerAccount() {
return invoiceOwnerAccount;
}
public void setInvoiceOwnerAccount(Account invoiceOwnerAccount) {
this.invoiceOwnerAccount = invoiceOwnerAccount;
}
public Subscription autoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
return this;
}
/**
* If this field is set to `true`, the subscription automatically renews at the end of the current term.
* @return autoRenew
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If this field is set to `true`, the subscription automatically renews at the end of the current term.")
public Boolean getAutoRenew() {
return autoRenew;
}
public void setAutoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
}
public Subscription latestVersion(Boolean latestVersion) {
this.latestVersion = latestVersion;
return this;
}
/**
* If true, this is the latest version of the subscription
* @return latestVersion
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, this is the latest version of the subscription")
public Boolean getLatestVersion() {
return latestVersion;
}
public void setLatestVersion(Boolean latestVersion) {
this.latestVersion = latestVersion;
}
public Subscription initialTerm(Term initialTerm) {
this.initialTerm = initialTerm;
return this;
}
/**
* Initial term information for the subscription.
* @return initialTerm
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Initial term information for the subscription.")
public Term getInitialTerm() {
return initialTerm;
}
public void setInitialTerm(Term initialTerm) {
this.initialTerm = initialTerm;
}
public Subscription currentTerm(Term currentTerm) {
this.currentTerm = currentTerm;
return this;
}
/**
* Current term information for the subscription
* @return currentTerm
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Current term information for the subscription")
public Term getCurrentTerm() {
return currentTerm;
}
public void setCurrentTerm(Term currentTerm) {
this.currentTerm = currentTerm;
}
public Subscription renewalTerm(Term renewalTerm) {
this.renewalTerm = renewalTerm;
return this;
}
/**
* Renewal term information for the subscription.
* @return renewalTerm
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Renewal term information for the subscription.")
public Term getRenewalTerm() {
return renewalTerm;
}
public void setRenewalTerm(Term renewalTerm) {
this.renewalTerm = renewalTerm;
}
public Subscription startDate(LocalDate startDate) {
this.startDate = startDate;
return this;
}
/**
* Date when the subscription starts.
* @return startDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Wed Jan 01 00:00:00 GMT 2020", value = "Date when the subscription starts.")
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public Subscription endDate(LocalDate endDate) {
this.endDate = endDate;
return this;
}
/**
* Date when the subscription ends.
* @return endDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "Date when the subscription ends.")
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
public Subscription description(String description) {
this.description = description;
return this;
}
/**
* Description of the subscription. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Description of the subscription. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Subscription contractEffective(LocalDate contractEffective) {
this.contractEffective = contractEffective;
return this;
}
/**
* Date when the subscriber contract is effective.
* @return contractEffective
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "Date when the subscriber contract is effective.")
public LocalDate getContractEffective() {
return contractEffective;
}
public void setContractEffective(LocalDate contractEffective) {
this.contractEffective = contractEffective;
}
public Subscription serviceActivation(LocalDate serviceActivation) {
this.serviceActivation = serviceActivation;
return this;
}
/**
* Date when the subscribed-to service is activated.
* @return serviceActivation
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "Date when the subscribed-to service is activated.")
public LocalDate getServiceActivation() {
return serviceActivation;
}
public void setServiceActivation(LocalDate serviceActivation) {
this.serviceActivation = serviceActivation;
}
public Subscription customerAcceptance(LocalDate customerAcceptance) {
this.customerAcceptance = customerAcceptance;
return this;
}
/**
* Date when all the services or products in the subscription are accepted by the subscriber.
* @return customerAcceptance
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "Date when all the services or products in the subscription are accepted by the subscriber.")
public LocalDate getCustomerAcceptance() {
return customerAcceptance;
}
public void setCustomerAcceptance(LocalDate customerAcceptance) {
this.customerAcceptance = customerAcceptance;
}
public Subscription invoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
return this;
}
/**
* If true, the subscription is billed separately from other subscriptions. If false, the subscription is included with other subscriptions in the account invoice. The default is false.
* @return invoiceSeparately
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, the subscription is billed separately from other subscriptions. If false, the subscription is included with other subscriptions in the account invoice. The default is false.")
public Boolean getInvoiceSeparately() {
return invoiceSeparately;
}
public void setInvoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
}
public Subscription orderNumber(String orderNumber) {
this.orderNumber = orderNumber;
return this;
}
/**
* The order number of the order created by Zuora.
* @return orderNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The order number of the order created by Zuora.")
public String getOrderNumber() {
return orderNumber;
}
public void setOrderNumber(String orderNumber) {
this.orderNumber = orderNumber;
}
/**
* List of subscription plans.
* @return subscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of subscription plans.")
public SubscriptionPlanListResponse getSubscriptionPlans() {
return subscriptionPlans;
}
/**
* List of invoice items.
* @return invoiceItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of invoice items.")
public InvoiceItemListResponse getInvoiceItems() {
return invoiceItems;
}
/**
* Total prepaid units available during a subscription. It is an aggregate of all funds under a subscription. **Deprecated, please use `prepaid_balances` instead.**
* @return prepaidBalance
* @deprecated
**/
@Deprecated
@javax.annotation.Nullable
@ApiModelProperty(value = "Total prepaid units available during a subscription. It is an aggregate of all funds under a subscription. **Deprecated, please use `prepaid_balances` instead.**")
public List getPrepaidBalance() {
return prepaidBalance;
}
/**
* Total prepaid units available during a subscription. It is an aggregate of all funds under a subscription.
* @return prepaidBalances
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Total prepaid units available during a subscription. It is an aggregate of all funds under a subscription.")
public List getPrepaidBalances() {
return prepaidBalances;
}
public Subscription contractedMrr(String contractedMrr) {
this.contractedMrr = contractedMrr;
return this;
}
/**
* Monthly recurring revenue of the subscription.
* @return contractedMrr
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Monthly recurring revenue of the subscription.")
public String getContractedMrr() {
return contractedMrr;
}
public void setContractedMrr(String contractedMrr) {
this.contractedMrr = contractedMrr;
}
public Subscription currency(String currency) {
this.currency = currency;
return this;
}
/**
* 3-letter ISO 4217 currency code. This field is available only if you have the [Multiple Currencies](https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Flexible_Billing/Multiple_Currencies) feature enabled.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "3-letter ISO 4217 currency code. This field is available only if you have the [Multiple Currencies](https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Flexible_Billing/Multiple_Currencies) feature enabled.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public Subscription cancelReason(String cancelReason) {
this.cancelReason = cancelReason;
return this;
}
/**
* The reason for cancelling the subscription.
* @return cancelReason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The reason for cancelling the subscription.")
public String getCancelReason() {
return cancelReason;
}
public void setCancelReason(String cancelReason) {
this.cancelReason = cancelReason;
}
public Subscription lastBookingDate(LocalDate lastBookingDate) {
this.lastBookingDate = lastBookingDate;
return this;
}
/**
* The last booking date of the subscription object. You can override the date value when creating a subscription through the \"Subscribe and Amend\" API. The default value `today` is set per the user's timezone. The value of this field is as follows: <ul> <li>For a new subscription created by the [Subscribe and Amend](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Orders_Harmonization/Orders_Migration_Guidance#Subscribe_and_Amend_APIs_to_Migrate) APIs, this field has the value of the subscription creation date. </li> <li>For a subscription changed by an amendment, this field has the value of the amendment booking date.</li> <li>For a subscription created or changed by an order, this field has the value of the order date.</li> </ul>
* @return lastBookingDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sun Jan 01 00:00:00 GMT 2023", value = "The last booking date of the subscription object. You can override the date value when creating a subscription through the \"Subscribe and Amend\" API. The default value `today` is set per the user's timezone. The value of this field is as follows: - For a new subscription created by the [Subscribe and Amend](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Orders_Harmonization/Orders_Migration_Guidance#Subscribe_and_Amend_APIs_to_Migrate) APIs, this field has the value of the subscription creation date.
- For a subscription changed by an amendment, this field has the value of the amendment booking date.
- For a subscription created or changed by an order, this field has the value of the order date.
")
public LocalDate getLastBookingDate() {
return lastBookingDate;
}
public void setLastBookingDate(LocalDate lastBookingDate) {
this.lastBookingDate = lastBookingDate;
}
public Subscription billToId(String billToId) {
this.billToId = billToId;
return this;
}
/**
* ID of the bill-to contact.
* @return billToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "ID of the bill-to contact.")
public String getBillToId() {
return billToId;
}
public void setBillToId(String billToId) {
this.billToId = billToId;
}
public Subscription paymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
return this;
}
/**
* The name of payment term associated with the invoice.
* @return paymentTerms
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of payment term associated with the invoice.")
public String getPaymentTerms() {
return paymentTerms;
}
public void setPaymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
}
/**
* The billing address for the customer.
* @return billTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing address for the customer.")
public Contact getBillTo() {
return billTo;
}
public Subscription billingDocumentSettings(FlexibleBillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
return this;
}
/**
* The billing document settings for the customer.
* @return billingDocumentSettings
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing document settings for the customer.")
public FlexibleBillingDocumentSettings getBillingDocumentSettings() {
return billingDocumentSettings;
}
public void setBillingDocumentSettings(FlexibleBillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
}
public Subscription soldToId(String soldToId) {
this.soldToId = soldToId;
return this;
}
/**
* ID of the sold-to contact.
* @return soldToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "ID of the sold-to contact.")
public String getSoldToId() {
return soldToId;
}
public void setSoldToId(String soldToId) {
this.soldToId = soldToId;
}
/**
* The selling address for the customer.
* @return soldTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The selling address for the customer.")
public Contact getSoldTo() {
return soldTo;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Subscription subscription = (Subscription) o;
return Objects.equals(this.id, subscription.id) &&
Objects.equals(this.updatedById, subscription.updatedById) &&
Objects.equals(this.updatedTime, subscription.updatedTime) &&
Objects.equals(this.createdById, subscription.createdById) &&
Objects.equals(this.createdTime, subscription.createdTime) &&
Objects.equals(this.customFields, subscription.customFields) &&
Objects.equals(this.customObjects, subscription.customObjects) &&
Objects.equals(this.subscriptionNumber, subscription.subscriptionNumber) &&
Objects.equals(this.state, subscription.state) &&
Objects.equals(this.version, subscription.version) &&
Objects.equals(this.accountId, subscription.accountId) &&
Objects.equals(this.account, subscription.account) &&
Objects.equals(this.invoiceOwnerAccountId, subscription.invoiceOwnerAccountId) &&
Objects.equals(this.invoiceOwnerAccount, subscription.invoiceOwnerAccount) &&
Objects.equals(this.autoRenew, subscription.autoRenew) &&
Objects.equals(this.latestVersion, subscription.latestVersion) &&
Objects.equals(this.initialTerm, subscription.initialTerm) &&
Objects.equals(this.currentTerm, subscription.currentTerm) &&
Objects.equals(this.renewalTerm, subscription.renewalTerm) &&
Objects.equals(this.startDate, subscription.startDate) &&
Objects.equals(this.endDate, subscription.endDate) &&
Objects.equals(this.description, subscription.description) &&
Objects.equals(this.contractEffective, subscription.contractEffective) &&
Objects.equals(this.serviceActivation, subscription.serviceActivation) &&
Objects.equals(this.customerAcceptance, subscription.customerAcceptance) &&
Objects.equals(this.invoiceSeparately, subscription.invoiceSeparately) &&
Objects.equals(this.orderNumber, subscription.orderNumber) &&
Objects.equals(this.subscriptionPlans, subscription.subscriptionPlans) &&
Objects.equals(this.invoiceItems, subscription.invoiceItems) &&
Objects.equals(this.prepaidBalance, subscription.prepaidBalance) &&
Objects.equals(this.prepaidBalances, subscription.prepaidBalances) &&
Objects.equals(this.contractedMrr, subscription.contractedMrr) &&
Objects.equals(this.currency, subscription.currency) &&
Objects.equals(this.cancelReason, subscription.cancelReason) &&
Objects.equals(this.lastBookingDate, subscription.lastBookingDate) &&
Objects.equals(this.billToId, subscription.billToId) &&
Objects.equals(this.paymentTerms, subscription.paymentTerms) &&
Objects.equals(this.billTo, subscription.billTo) &&
Objects.equals(this.billingDocumentSettings, subscription.billingDocumentSettings) &&
Objects.equals(this.soldToId, subscription.soldToId) &&
Objects.equals(this.soldTo, subscription.soldTo);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, subscriptionNumber, state, version, accountId, account, invoiceOwnerAccountId, invoiceOwnerAccount, autoRenew, latestVersion, initialTerm, currentTerm, renewalTerm, startDate, endDate, description, contractEffective, serviceActivation, customerAcceptance, invoiceSeparately, orderNumber, subscriptionPlans, invoiceItems, prepaidBalance, prepaidBalances, contractedMrr, currency, cancelReason, lastBookingDate, billToId, paymentTerms, billTo, billingDocumentSettings, soldToId, soldTo);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Subscription {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" subscriptionNumber: ").append(toIndentedString(subscriptionNumber)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" invoiceOwnerAccountId: ").append(toIndentedString(invoiceOwnerAccountId)).append("\n");
sb.append(" invoiceOwnerAccount: ").append(toIndentedString(invoiceOwnerAccount)).append("\n");
sb.append(" autoRenew: ").append(toIndentedString(autoRenew)).append("\n");
sb.append(" latestVersion: ").append(toIndentedString(latestVersion)).append("\n");
sb.append(" initialTerm: ").append(toIndentedString(initialTerm)).append("\n");
sb.append(" currentTerm: ").append(toIndentedString(currentTerm)).append("\n");
sb.append(" renewalTerm: ").append(toIndentedString(renewalTerm)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" contractEffective: ").append(toIndentedString(contractEffective)).append("\n");
sb.append(" serviceActivation: ").append(toIndentedString(serviceActivation)).append("\n");
sb.append(" customerAcceptance: ").append(toIndentedString(customerAcceptance)).append("\n");
sb.append(" invoiceSeparately: ").append(toIndentedString(invoiceSeparately)).append("\n");
sb.append(" orderNumber: ").append(toIndentedString(orderNumber)).append("\n");
sb.append(" subscriptionPlans: ").append(toIndentedString(subscriptionPlans)).append("\n");
sb.append(" invoiceItems: ").append(toIndentedString(invoiceItems)).append("\n");
sb.append(" prepaidBalance: ").append(toIndentedString(prepaidBalance)).append("\n");
sb.append(" prepaidBalances: ").append(toIndentedString(prepaidBalances)).append("\n");
sb.append(" contractedMrr: ").append(toIndentedString(contractedMrr)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" cancelReason: ").append(toIndentedString(cancelReason)).append("\n");
sb.append(" lastBookingDate: ").append(toIndentedString(lastBookingDate)).append("\n");
sb.append(" billToId: ").append(toIndentedString(billToId)).append("\n");
sb.append(" paymentTerms: ").append(toIndentedString(paymentTerms)).append("\n");
sb.append(" billTo: ").append(toIndentedString(billTo)).append("\n");
sb.append(" billingDocumentSettings: ").append(toIndentedString(billingDocumentSettings)).append("\n");
sb.append(" soldToId: ").append(toIndentedString(soldToId)).append("\n");
sb.append(" soldTo: ").append(toIndentedString(soldTo)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy