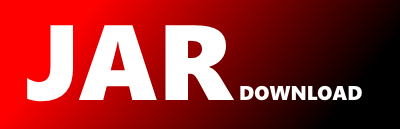
org.openapitools.client.model.SubscriptionItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ItemTier;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.openapitools.client.model.Overage;
import org.openapitools.client.model.Price;
import org.openapitools.client.model.Recurring;
import org.openapitools.client.model.SubscriptionPlan;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* Subscription item information.
*/
@ApiModel(description = "Subscription item information.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SubscriptionItem {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_NUMBER = "subscription_item_number";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_NUMBER)
private String subscriptionItemNumber;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_PRODUCT_ID = "product_id";
@SerializedName(SERIALIZED_NAME_PRODUCT_ID)
private String productId;
public static final String SERIALIZED_NAME_CHARGED_THROUGH_DATE = "charged_through_date";
@SerializedName(SERIALIZED_NAME_CHARGED_THROUGH_DATE)
private LocalDate chargedThroughDate;
public static final String SERIALIZED_NAME_RECURRING = "recurring";
@SerializedName(SERIALIZED_NAME_RECURRING)
private Recurring recurring;
public static final String SERIALIZED_NAME_ACTIVE = "active";
@SerializedName(SERIALIZED_NAME_ACTIVE)
private Boolean active;
/**
* Based on the current date to populate a state field with the enumeration: [inactive, active, expired] where inactive would represent charge segments with a start_date in the future and expired charge segments with an end_date in the past.
*/
@JsonAdapter(StateEnum.Adapter.class)
public enum StateEnum {
INACTIVE("inactive"),
ACTIVE("active"),
EXPIRED("expired"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StateEnum fromValue(String value) {
for (StateEnum b : StateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StateEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private StateEnum state;
/**
* Specifies when to start billing your customer.
*/
@JsonAdapter(StartEventEnum.Adapter.class)
public enum StartEventEnum {
CONTRACT_EFFECTIVE("contract_effective"),
SERVICE_ACTIVATION("service_activation"),
CUSTOMER_ACCEPTANCE("customer_acceptance"),
SPECIFIC_DATE("specific_date"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StartEventEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StartEventEnum fromValue(String value) {
for (StartEventEnum b : StartEventEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StartEventEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StartEventEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StartEventEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_START_EVENT = "start_event";
@SerializedName(SERIALIZED_NAME_START_EVENT)
private StartEventEnum startEvent;
/**
* Specifies the kind of tiering.
*/
@JsonAdapter(TiersModeEnum.Adapter.class)
public enum TiersModeEnum {
GRADUATED("graduated"),
VOLUME("volume"),
HIGH_WATERMARK_VOLUME("high_watermark_volume"),
HIGH_WATERMARK_GRADUATED("high_watermark_graduated"),
GRADUATED_WITH_OVERAGE("graduated_with_overage"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TiersModeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TiersModeEnum fromValue(String value) {
for (TiersModeEnum b : TiersModeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TiersModeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TiersModeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TiersModeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TIERS_MODE = "tiers_mode";
@SerializedName(SERIALIZED_NAME_TIERS_MODE)
private TiersModeEnum tiersMode;
public static final String SERIALIZED_NAME_TIERS = "tiers";
@SerializedName(SERIALIZED_NAME_TIERS)
private List tiers = null;
public static final String SERIALIZED_NAME_TAX_CODE = "tax_code";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_INCLUSIVE = "tax_inclusive";
@SerializedName(SERIALIZED_NAME_TAX_INCLUSIVE)
private Boolean taxInclusive;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unit_of_measure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_UNIT_AMOUNT = "unit_amount";
@SerializedName(SERIALIZED_NAME_UNIT_AMOUNT)
private BigDecimal unitAmount;
public static final String SERIALIZED_NAME_DISCOUNT_AMOUNT = "discount_amount";
@SerializedName(SERIALIZED_NAME_DISCOUNT_AMOUNT)
private BigDecimal discountAmount;
public static final String SERIALIZED_NAME_DISCOUNT_PERCENT = "discount_percent";
@SerializedName(SERIALIZED_NAME_DISCOUNT_PERCENT)
private BigDecimal discountPercent;
/**
* Gets or Sets applyDiscountTo
*/
@JsonAdapter(ApplyDiscountToEnum.Adapter.class)
public enum ApplyDiscountToEnum {
ONE_TIME("one_time"),
RECURRING("recurring"),
USAGE("usage"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ApplyDiscountToEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ApplyDiscountToEnum fromValue(String value) {
for (ApplyDiscountToEnum b : ApplyDiscountToEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ApplyDiscountToEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ApplyDiscountToEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ApplyDiscountToEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_APPLY_DISCOUNT_TO = "apply_discount_to";
@SerializedName(SERIALIZED_NAME_APPLY_DISCOUNT_TO)
private List applyDiscountTo = null;
/**
* Specifies at what level a discount should be applied: one of account, subscription or plan.
*/
@JsonAdapter(DiscountLevelEnum.Adapter.class)
public enum DiscountLevelEnum {
ACCOUNT("account"),
SUBSCRIPTION("subscription"),
PLAN("plan"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
DiscountLevelEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DiscountLevelEnum fromValue(String value) {
for (DiscountLevelEnum b : DiscountLevelEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DiscountLevelEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DiscountLevelEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DiscountLevelEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DISCOUNT_LEVEL = "discount_level";
@SerializedName(SERIALIZED_NAME_DISCOUNT_LEVEL)
private DiscountLevelEnum discountLevel;
/**
* Specifies the base interval of the price the subscriber is subscribed to. If not provided, this field defaults to `billing_period`.
*/
@JsonAdapter(PriceBaseIntervalEnum.Adapter.class)
public enum PriceBaseIntervalEnum {
MONTH("month"),
BILLING_PERIOD("billing_period"),
WEEK("week"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
PriceBaseIntervalEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PriceBaseIntervalEnum fromValue(String value) {
for (PriceBaseIntervalEnum b : PriceBaseIntervalEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PriceBaseIntervalEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PriceBaseIntervalEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PriceBaseIntervalEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PRICE_BASE_INTERVAL = "price_base_interval";
@SerializedName(SERIALIZED_NAME_PRICE_BASE_INTERVAL)
private PriceBaseIntervalEnum priceBaseInterval;
public static final String SERIALIZED_NAME_OVERAGE = "overage";
@SerializedName(SERIALIZED_NAME_OVERAGE)
private Overage overage;
public static final String SERIALIZED_NAME_CHARGE_MODEL = "charge_model";
@SerializedName(SERIALIZED_NAME_CHARGE_MODEL)
private String chargeModel;
public static final String SERIALIZED_NAME_CHARGE_TYPE = "charge_type";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE)
private String chargeType;
public static final String SERIALIZED_NAME_PRICE_ID = "price_id";
@SerializedName(SERIALIZED_NAME_PRICE_ID)
private String priceId;
public static final String SERIALIZED_NAME_PRICE = "price";
@SerializedName(SERIALIZED_NAME_PRICE)
private Price price;
public static final String SERIALIZED_NAME_SUBSCRIPTION_PLAN_ID = "subscription_plan_id";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_PLAN_ID)
private String subscriptionPlanId;
public static final String SERIALIZED_NAME_SUBSCRIPTION_PLAN = "subscription_plan";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_PLAN)
private SubscriptionPlan subscriptionPlan;
public static final String SERIALIZED_NAME_START_DATE = "start_date";
@SerializedName(SERIALIZED_NAME_START_DATE)
private LocalDate startDate;
public static final String SERIALIZED_NAME_END_DATE = "end_date";
@SerializedName(SERIALIZED_NAME_END_DATE)
private LocalDate endDate;
public static final String SERIALIZED_NAME_PROCESSED_THROUGH_DATE = "processed_through_date";
@SerializedName(SERIALIZED_NAME_PROCESSED_THROUGH_DATE)
private LocalDate processedThroughDate;
public SubscriptionItem() {
}
public SubscriptionItem(
String id,
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects,
LocalDate startDate,
LocalDate endDate,
LocalDate processedThroughDate
) {
this();
this.id = id;
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
this.startDate = startDate;
this.endDate = endDate;
this.processedThroughDate = processedThroughDate;
}
/**
* Unique identifier for the object.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the object.")
public String getId() {
return id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public SubscriptionItem customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public SubscriptionItem putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public SubscriptionItem subscriptionItemNumber(String subscriptionItemNumber) {
this.subscriptionItemNumber = subscriptionItemNumber;
return this;
}
/**
* Human-readable identifier of the subscription item. It can be user-supplied.
* @return subscriptionItemNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the subscription item. It can be user-supplied.")
public String getSubscriptionItemNumber() {
return subscriptionItemNumber;
}
public void setSubscriptionItemNumber(String subscriptionItemNumber) {
this.subscriptionItemNumber = subscriptionItemNumber;
}
public SubscriptionItem name(String name) {
this.name = name;
return this;
}
/**
* The name of the subscription item.
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the subscription item.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public SubscriptionItem description(String description) {
this.description = description;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An arbitrary string attached to the object. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public SubscriptionItem productId(String productId) {
this.productId = productId;
return this;
}
/**
* Identifier of the product with which this subscription is associated.
* @return productId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the product with which this subscription is associated.")
public String getProductId() {
return productId;
}
public void setProductId(String productId) {
this.productId = productId;
}
public SubscriptionItem chargedThroughDate(LocalDate chargedThroughDate) {
this.chargedThroughDate = chargedThroughDate;
return this;
}
/**
* The date through which a customer has been billed for the subscription item.
* @return chargedThroughDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date through which a customer has been billed for the subscription item.")
public LocalDate getChargedThroughDate() {
return chargedThroughDate;
}
public void setChargedThroughDate(LocalDate chargedThroughDate) {
this.chargedThroughDate = chargedThroughDate;
}
public SubscriptionItem recurring(Recurring recurring) {
this.recurring = recurring;
return this;
}
/**
* Get recurring
* @return recurring
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Recurring getRecurring() {
return recurring;
}
public void setRecurring(Recurring recurring) {
this.recurring = recurring;
}
public SubscriptionItem active(Boolean active) {
this.active = active;
return this;
}
/**
* Indicates whether the price is active and can be used for new purchases.
* @return active
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether the price is active and can be used for new purchases.")
public Boolean getActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public SubscriptionItem state(StateEnum state) {
this.state = state;
return this;
}
/**
* Based on the current date to populate a state field with the enumeration: [inactive, active, expired] where inactive would represent charge segments with a start_date in the future and expired charge segments with an end_date in the past.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Based on the current date to populate a state field with the enumeration: [inactive, active, expired] where inactive would represent charge segments with a start_date in the future and expired charge segments with an end_date in the past.")
public StateEnum getState() {
return state;
}
public void setState(StateEnum state) {
this.state = state;
}
public SubscriptionItem startEvent(StartEventEnum startEvent) {
this.startEvent = startEvent;
return this;
}
/**
* Specifies when to start billing your customer.
* @return startEvent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies when to start billing your customer.")
public StartEventEnum getStartEvent() {
return startEvent;
}
public void setStartEvent(StartEventEnum startEvent) {
this.startEvent = startEvent;
}
public SubscriptionItem tiersMode(TiersModeEnum tiersMode) {
this.tiersMode = tiersMode;
return this;
}
/**
* Specifies the kind of tiering.
* @return tiersMode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the kind of tiering.")
public TiersModeEnum getTiersMode() {
return tiersMode;
}
public void setTiersMode(TiersModeEnum tiersMode) {
this.tiersMode = tiersMode;
}
public SubscriptionItem tiers(List tiers) {
this.tiers = tiers;
return this;
}
public SubscriptionItem addTiersItem(ItemTier tiersItem) {
if (this.tiers == null) {
this.tiers = new ArrayList();
}
this.tiers.add(tiersItem);
return this;
}
/**
* Get tiers
* @return tiers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getTiers() {
return tiers;
}
public void setTiers(List tiers) {
this.tiers = tiers;
}
public SubscriptionItem taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* A tax code identifier. If the `tax_code` of a price is not provided when you create or override a price, Zuora will treat the charged amount as non-taxable. If this code is provide, Zuora considers that this price is taxable and the charged amount will be handled accordingly.
* @return taxCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A tax code identifier. If the `tax_code` of a price is not provided when you create or override a price, Zuora will treat the charged amount as non-taxable. If this code is provide, Zuora considers that this price is taxable and the charged amount will be handled accordingly.")
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public SubscriptionItem taxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
return this;
}
/**
* If this field is set to `true`, it indicates that amounts are inclusive of tax.
* @return taxInclusive
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If this field is set to `true`, it indicates that amounts are inclusive of tax.")
public Boolean getTaxInclusive() {
return taxInclusive;
}
public void setTaxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
}
public SubscriptionItem unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* A configured unit of measure. This field is required for per-unit prices.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A configured unit of measure. This field is required for per-unit prices.")
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
public SubscriptionItem quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* Quantity of the product to which your customers subscribe.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Quantity of the product to which your customers subscribe.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public SubscriptionItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount of the price. Specify this field if you want to override the original price with a flat-fee price
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of the price. Specify this field if you want to override the original price with a flat-fee price")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public SubscriptionItem unitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* The unit amount of the price. Specify this field if you want to override the original price with a per-unit price.
* @return unitAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unit amount of the price. Specify this field if you want to override the original price with a per-unit price.")
public BigDecimal getUnitAmount() {
return unitAmount;
}
public void setUnitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
}
public SubscriptionItem discountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
return this;
}
/**
* Discount amount. Specify this field if you offer an amount-based discount.
* @return discountAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Discount amount. Specify this field if you offer an amount-based discount.")
public BigDecimal getDiscountAmount() {
return discountAmount;
}
public void setDiscountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
}
public SubscriptionItem discountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
return this;
}
/**
* Discount percent. Specify this field if you offer a percentage-based discount.
* @return discountPercent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Discount percent. Specify this field if you offer a percentage-based discount.")
public BigDecimal getDiscountPercent() {
return discountPercent;
}
public void setDiscountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
}
public SubscriptionItem applyDiscountTo(List applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
return this;
}
public SubscriptionItem addApplyDiscountToItem(ApplyDiscountToEnum applyDiscountToItem) {
if (this.applyDiscountTo == null) {
this.applyDiscountTo = new ArrayList();
}
this.applyDiscountTo.add(applyDiscountToItem);
return this;
}
/**
* Any combination of one_time, recurring and plan.
* @return applyDiscountTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Any combination of one_time, recurring and plan.")
public List getApplyDiscountTo() {
return applyDiscountTo;
}
public void setApplyDiscountTo(List applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
}
public SubscriptionItem discountLevel(DiscountLevelEnum discountLevel) {
this.discountLevel = discountLevel;
return this;
}
/**
* Specifies at what level a discount should be applied: one of account, subscription or plan.
* @return discountLevel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies at what level a discount should be applied: one of account, subscription or plan.")
public DiscountLevelEnum getDiscountLevel() {
return discountLevel;
}
public void setDiscountLevel(DiscountLevelEnum discountLevel) {
this.discountLevel = discountLevel;
}
public SubscriptionItem priceBaseInterval(PriceBaseIntervalEnum priceBaseInterval) {
this.priceBaseInterval = priceBaseInterval;
return this;
}
/**
* Specifies the base interval of the price the subscriber is subscribed to. If not provided, this field defaults to `billing_period`.
* @return priceBaseInterval
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the base interval of the price the subscriber is subscribed to. If not provided, this field defaults to `billing_period`.")
public PriceBaseIntervalEnum getPriceBaseInterval() {
return priceBaseInterval;
}
public void setPriceBaseInterval(PriceBaseIntervalEnum priceBaseInterval) {
this.priceBaseInterval = priceBaseInterval;
}
public SubscriptionItem overage(Overage overage) {
this.overage = overage;
return this;
}
/**
* Get overage
* @return overage
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Overage getOverage() {
return overage;
}
public void setOverage(Overage overage) {
this.overage = overage;
}
public SubscriptionItem chargeModel(String chargeModel) {
this.chargeModel = chargeModel;
return this;
}
/**
* Charge model of the price. See [Charge models](https://knowledgecenter.zuora.com/Billing/Subscriptions/Product_Catalog/B_Charge_Models) for more information.
* @return chargeModel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Charge model of the price. See [Charge models](https://knowledgecenter.zuora.com/Billing/Subscriptions/Product_Catalog/B_Charge_Models) for more information.")
public String getChargeModel() {
return chargeModel;
}
public void setChargeModel(String chargeModel) {
this.chargeModel = chargeModel;
}
public SubscriptionItem chargeType(String chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Type of the charge. It can be one of the following types: one-time, recurring, or usage.
* @return chargeType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Type of the charge. It can be one of the following types: one-time, recurring, or usage.")
public String getChargeType() {
return chargeType;
}
public void setChargeType(String chargeType) {
this.chargeType = chargeType;
}
public SubscriptionItem priceId(String priceId) {
this.priceId = priceId;
return this;
}
/**
* Identifier of the price.
* @return priceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the price.")
public String getPriceId() {
return priceId;
}
public void setPriceId(String priceId) {
this.priceId = priceId;
}
public SubscriptionItem price(Price price) {
this.price = price;
return this;
}
/**
* Get price
* @return price
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Price getPrice() {
return price;
}
public void setPrice(Price price) {
this.price = price;
}
public SubscriptionItem subscriptionPlanId(String subscriptionPlanId) {
this.subscriptionPlanId = subscriptionPlanId;
return this;
}
/**
* Identifier of the subscription plan this subscription item belongs to.
* @return subscriptionPlanId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the subscription plan this subscription item belongs to.")
public String getSubscriptionPlanId() {
return subscriptionPlanId;
}
public void setSubscriptionPlanId(String subscriptionPlanId) {
this.subscriptionPlanId = subscriptionPlanId;
}
public SubscriptionItem subscriptionPlan(SubscriptionPlan subscriptionPlan) {
this.subscriptionPlan = subscriptionPlan;
return this;
}
/**
* Get subscriptionPlan
* @return subscriptionPlan
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SubscriptionPlan getSubscriptionPlan() {
return subscriptionPlan;
}
public void setSubscriptionPlan(SubscriptionPlan subscriptionPlan) {
this.subscriptionPlan = subscriptionPlan;
}
/**
* The date when the subscription item starts.
* @return startDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date when the subscription item starts.")
public LocalDate getStartDate() {
return startDate;
}
/**
* The date when the subscription item ends.
* @return endDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date when the subscription item ends.")
public LocalDate getEndDate() {
return endDate;
}
/**
* The date until when charges are processed. When billing takes place in arrears, such as usage-based prices, this field value is the the same as the `ChargedThroughDate` value. This date is the earliest date when a charge can be amended.
* @return processedThroughDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date until when charges are processed. When billing takes place in arrears, such as usage-based prices, this field value is the the same as the `ChargedThroughDate` value. This date is the earliest date when a charge can be amended.")
public LocalDate getProcessedThroughDate() {
return processedThroughDate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubscriptionItem subscriptionItem = (SubscriptionItem) o;
return Objects.equals(this.id, subscriptionItem.id) &&
Objects.equals(this.updatedById, subscriptionItem.updatedById) &&
Objects.equals(this.updatedTime, subscriptionItem.updatedTime) &&
Objects.equals(this.createdById, subscriptionItem.createdById) &&
Objects.equals(this.createdTime, subscriptionItem.createdTime) &&
Objects.equals(this.customFields, subscriptionItem.customFields) &&
Objects.equals(this.customObjects, subscriptionItem.customObjects) &&
Objects.equals(this.subscriptionItemNumber, subscriptionItem.subscriptionItemNumber) &&
Objects.equals(this.name, subscriptionItem.name) &&
Objects.equals(this.description, subscriptionItem.description) &&
Objects.equals(this.productId, subscriptionItem.productId) &&
Objects.equals(this.chargedThroughDate, subscriptionItem.chargedThroughDate) &&
Objects.equals(this.recurring, subscriptionItem.recurring) &&
Objects.equals(this.active, subscriptionItem.active) &&
Objects.equals(this.state, subscriptionItem.state) &&
Objects.equals(this.startEvent, subscriptionItem.startEvent) &&
Objects.equals(this.tiersMode, subscriptionItem.tiersMode) &&
Objects.equals(this.tiers, subscriptionItem.tiers) &&
Objects.equals(this.taxCode, subscriptionItem.taxCode) &&
Objects.equals(this.taxInclusive, subscriptionItem.taxInclusive) &&
Objects.equals(this.unitOfMeasure, subscriptionItem.unitOfMeasure) &&
Objects.equals(this.quantity, subscriptionItem.quantity) &&
Objects.equals(this.amount, subscriptionItem.amount) &&
Objects.equals(this.unitAmount, subscriptionItem.unitAmount) &&
Objects.equals(this.discountAmount, subscriptionItem.discountAmount) &&
Objects.equals(this.discountPercent, subscriptionItem.discountPercent) &&
Objects.equals(this.applyDiscountTo, subscriptionItem.applyDiscountTo) &&
Objects.equals(this.discountLevel, subscriptionItem.discountLevel) &&
Objects.equals(this.priceBaseInterval, subscriptionItem.priceBaseInterval) &&
Objects.equals(this.overage, subscriptionItem.overage) &&
Objects.equals(this.chargeModel, subscriptionItem.chargeModel) &&
Objects.equals(this.chargeType, subscriptionItem.chargeType) &&
Objects.equals(this.priceId, subscriptionItem.priceId) &&
Objects.equals(this.price, subscriptionItem.price) &&
Objects.equals(this.subscriptionPlanId, subscriptionItem.subscriptionPlanId) &&
Objects.equals(this.subscriptionPlan, subscriptionItem.subscriptionPlan) &&
Objects.equals(this.startDate, subscriptionItem.startDate) &&
Objects.equals(this.endDate, subscriptionItem.endDate) &&
Objects.equals(this.processedThroughDate, subscriptionItem.processedThroughDate);
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, subscriptionItemNumber, name, description, productId, chargedThroughDate, recurring, active, state, startEvent, tiersMode, tiers, taxCode, taxInclusive, unitOfMeasure, quantity, amount, unitAmount, discountAmount, discountPercent, applyDiscountTo, discountLevel, priceBaseInterval, overage, chargeModel, chargeType, priceId, price, subscriptionPlanId, subscriptionPlan, startDate, endDate, processedThroughDate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionItem {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" subscriptionItemNumber: ").append(toIndentedString(subscriptionItemNumber)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" productId: ").append(toIndentedString(productId)).append("\n");
sb.append(" chargedThroughDate: ").append(toIndentedString(chargedThroughDate)).append("\n");
sb.append(" recurring: ").append(toIndentedString(recurring)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" startEvent: ").append(toIndentedString(startEvent)).append("\n");
sb.append(" tiersMode: ").append(toIndentedString(tiersMode)).append("\n");
sb.append(" tiers: ").append(toIndentedString(tiers)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxInclusive: ").append(toIndentedString(taxInclusive)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" unitAmount: ").append(toIndentedString(unitAmount)).append("\n");
sb.append(" discountAmount: ").append(toIndentedString(discountAmount)).append("\n");
sb.append(" discountPercent: ").append(toIndentedString(discountPercent)).append("\n");
sb.append(" applyDiscountTo: ").append(toIndentedString(applyDiscountTo)).append("\n");
sb.append(" discountLevel: ").append(toIndentedString(discountLevel)).append("\n");
sb.append(" priceBaseInterval: ").append(toIndentedString(priceBaseInterval)).append("\n");
sb.append(" overage: ").append(toIndentedString(overage)).append("\n");
sb.append(" chargeModel: ").append(toIndentedString(chargeModel)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" priceId: ").append(toIndentedString(priceId)).append("\n");
sb.append(" price: ").append(toIndentedString(price)).append("\n");
sb.append(" subscriptionPlanId: ").append(toIndentedString(subscriptionPlanId)).append("\n");
sb.append(" subscriptionPlan: ").append(toIndentedString(subscriptionPlan)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" processedThroughDate: ").append(toIndentedString(processedThroughDate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy