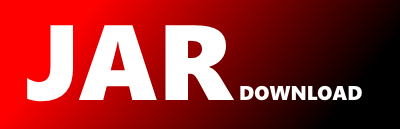
org.openapitools.client.model.SubscriptionItemCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.ItemTier;
import org.openapitools.client.model.Overage;
import org.openapitools.client.model.Recurring;
import org.openapitools.client.model.SubscriptionItemDrawdownField;
import org.openapitools.client.model.SubscriptionItemPrepaymentField;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* SubscriptionItemCreateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SubscriptionItemCreateRequest {
public static final String SERIALIZED_NAME_PRICE_ID = "price_id";
@SerializedName(SERIALIZED_NAME_PRICE_ID)
private String priceId;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_NUMBER = "subscription_item_number";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_NUMBER)
private String subscriptionItemNumber;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_RECURRING = "recurring";
@SerializedName(SERIALIZED_NAME_RECURRING)
private Recurring recurring;
/**
* Specifies when to start billing your customer.
*/
@JsonAdapter(StartEventEnum.Adapter.class)
public enum StartEventEnum {
CONTRACT_EFFECTIVE("contract_effective"),
SERVICE_ACTIVATION("service_activation"),
CUSTOMER_ACCEPTANCE("customer_acceptance"),
SPECIFIC_DATE("specific_date"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StartEventEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StartEventEnum fromValue(String value) {
for (StartEventEnum b : StartEventEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StartEventEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StartEventEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StartEventEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_START_EVENT = "start_event";
@SerializedName(SERIALIZED_NAME_START_EVENT)
private StartEventEnum startEvent;
public static final String SERIALIZED_NAME_START_DATE = "start_date";
@SerializedName(SERIALIZED_NAME_START_DATE)
private LocalDate startDate;
public static final String SERIALIZED_NAME_END_DATE = "end_date";
@SerializedName(SERIALIZED_NAME_END_DATE)
private LocalDate endDate;
/**
* Specifies the mode of tiering.
*/
@JsonAdapter(TiersModeEnum.Adapter.class)
public enum TiersModeEnum {
GRADUATED("graduated"),
VOLUME("volume"),
HIGH_WATERMARK_VOLUME("high_watermark_volume"),
HIGH_WATERMARK_GRADUATED("high_watermark_graduated"),
GRADUATED_WITH_OVERAGE("graduated_with_overage"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TiersModeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TiersModeEnum fromValue(String value) {
for (TiersModeEnum b : TiersModeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TiersModeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TiersModeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TiersModeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TIERS_MODE = "tiers_mode";
@SerializedName(SERIALIZED_NAME_TIERS_MODE)
private TiersModeEnum tiersMode;
public static final String SERIALIZED_NAME_TIERS = "tiers";
@SerializedName(SERIALIZED_NAME_TIERS)
private List tiers = null;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_UNIT_AMOUNT = "unit_amount";
@SerializedName(SERIALIZED_NAME_UNIT_AMOUNT)
private BigDecimal unitAmount;
public static final String SERIALIZED_NAME_DISCOUNT_AMOUNT = "discount_amount";
@SerializedName(SERIALIZED_NAME_DISCOUNT_AMOUNT)
private BigDecimal discountAmount;
public static final String SERIALIZED_NAME_DISCOUNT_PERCENT = "discount_percent";
@SerializedName(SERIALIZED_NAME_DISCOUNT_PERCENT)
private BigDecimal discountPercent;
/**
* Specifies the base interval of the price the subscriber is subscribed to. If not provided, this field defaults to `billing_period`.
*/
@JsonAdapter(PriceBaseIntervalEnum.Adapter.class)
public enum PriceBaseIntervalEnum {
MONTH("month"),
BILLING_PERIOD("billing_period"),
WEEK("week"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
PriceBaseIntervalEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PriceBaseIntervalEnum fromValue(String value) {
for (PriceBaseIntervalEnum b : PriceBaseIntervalEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PriceBaseIntervalEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PriceBaseIntervalEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PriceBaseIntervalEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PRICE_BASE_INTERVAL = "price_base_interval";
@SerializedName(SERIALIZED_NAME_PRICE_BASE_INTERVAL)
private PriceBaseIntervalEnum priceBaseInterval;
public static final String SERIALIZED_NAME_OVERAGE = "overage";
@SerializedName(SERIALIZED_NAME_OVERAGE)
private Overage overage;
public static final String SERIALIZED_NAME_UNIQUE_TOKEN = "unique_token";
@SerializedName(SERIALIZED_NAME_UNIQUE_TOKEN)
private String uniqueToken;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
/**
* Gets or Sets applyDiscountTo
*/
@JsonAdapter(ApplyDiscountToEnum.Adapter.class)
public enum ApplyDiscountToEnum {
ONE_TIME("one_time"),
RECURRING("recurring"),
USAGE("usage"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ApplyDiscountToEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ApplyDiscountToEnum fromValue(String value) {
for (ApplyDiscountToEnum b : ApplyDiscountToEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ApplyDiscountToEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ApplyDiscountToEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ApplyDiscountToEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_APPLY_DISCOUNT_TO = "apply_discount_to";
@SerializedName(SERIALIZED_NAME_APPLY_DISCOUNT_TO)
private List applyDiscountTo = null;
/**
* Specifies at what level a discount should be applied: one of account, subscription or plan.
*/
@JsonAdapter(DiscountLevelEnum.Adapter.class)
public enum DiscountLevelEnum {
ACCOUNT("account"),
SUBSCRIPTION("subscription"),
PLAN("plan"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
DiscountLevelEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DiscountLevelEnum fromValue(String value) {
for (DiscountLevelEnum b : DiscountLevelEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DiscountLevelEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DiscountLevelEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DiscountLevelEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DISCOUNT_LEVEL = "discount_level";
@SerializedName(SERIALIZED_NAME_DISCOUNT_LEVEL)
private DiscountLevelEnum discountLevel;
public static final String SERIALIZED_NAME_CUSTOM_FIELD_PER_UNIT_RATE = "custom_field_per_unit_rate";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELD_PER_UNIT_RATE)
private String customFieldPerUnitRate;
public static final String SERIALIZED_NAME_CUSTOM_FIELD_TOTAL_AMOUNT = "custom_field_total_amount";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELD_TOTAL_AMOUNT)
private String customFieldTotalAmount;
public static final String SERIALIZED_NAME_PRICE_CHANGE_PERCENTAGE = "price_change_percentage";
@SerializedName(SERIALIZED_NAME_PRICE_CHANGE_PERCENTAGE)
private BigDecimal priceChangePercentage;
/**
* Applies an automatic price change when a termed subscription is renewed.
*/
@JsonAdapter(PriceChangeOptionEnum.Adapter.class)
public enum PriceChangeOptionEnum {
LATEST_CATALOG_PRICING("latest_catalog_pricing"),
PERCENTAGE("percentage"),
NONE("none"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
PriceChangeOptionEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PriceChangeOptionEnum fromValue(String value) {
for (PriceChangeOptionEnum b : PriceChangeOptionEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PriceChangeOptionEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PriceChangeOptionEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PriceChangeOptionEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PRICE_CHANGE_OPTION = "price_change_option";
@SerializedName(SERIALIZED_NAME_PRICE_CHANGE_OPTION)
private PriceChangeOptionEnum priceChangeOption;
public static final String SERIALIZED_NAME_PREPAYMENT = "prepayment";
@SerializedName(SERIALIZED_NAME_PREPAYMENT)
private SubscriptionItemPrepaymentField prepayment;
public static final String SERIALIZED_NAME_DRAWDOWN = "drawdown";
@SerializedName(SERIALIZED_NAME_DRAWDOWN)
private SubscriptionItemDrawdownField drawdown;
public SubscriptionItemCreateRequest() {
}
public SubscriptionItemCreateRequest priceId(String priceId) {
this.priceId = priceId;
return this;
}
/**
* Identifier of the price.
* @return priceId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Identifier of the price.")
public String getPriceId() {
return priceId;
}
public void setPriceId(String priceId) {
this.priceId = priceId;
}
public SubscriptionItemCreateRequest subscriptionItemNumber(String subscriptionItemNumber) {
this.subscriptionItemNumber = subscriptionItemNumber;
return this;
}
/**
* Human-readable identifier of the subscription item. It can be user-supplied.
* @return subscriptionItemNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the subscription item. It can be user-supplied.")
public String getSubscriptionItemNumber() {
return subscriptionItemNumber;
}
public void setSubscriptionItemNumber(String subscriptionItemNumber) {
this.subscriptionItemNumber = subscriptionItemNumber;
}
public SubscriptionItemCreateRequest description(String description) {
this.description = description;
return this;
}
/**
* Description of the price. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Description of the price. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public SubscriptionItemCreateRequest recurring(Recurring recurring) {
this.recurring = recurring;
return this;
}
/**
* Get recurring
* @return recurring
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Recurring getRecurring() {
return recurring;
}
public void setRecurring(Recurring recurring) {
this.recurring = recurring;
}
public SubscriptionItemCreateRequest startEvent(StartEventEnum startEvent) {
this.startEvent = startEvent;
return this;
}
/**
* Specifies when to start billing your customer.
* @return startEvent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies when to start billing your customer.")
public StartEventEnum getStartEvent() {
return startEvent;
}
public void setStartEvent(StartEventEnum startEvent) {
this.startEvent = startEvent;
}
public SubscriptionItemCreateRequest startDate(LocalDate startDate) {
this.startDate = startDate;
return this;
}
/**
* The date when the subscription item starts
* @return startDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date when the subscription item starts")
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public SubscriptionItemCreateRequest endDate(LocalDate endDate) {
this.endDate = endDate;
return this;
}
/**
* The date when the subscription item ends or ended.
* @return endDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date when the subscription item ends or ended.")
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
public SubscriptionItemCreateRequest tiersMode(TiersModeEnum tiersMode) {
this.tiersMode = tiersMode;
return this;
}
/**
* Specifies the mode of tiering.
* @return tiersMode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the mode of tiering.")
public TiersModeEnum getTiersMode() {
return tiersMode;
}
public void setTiersMode(TiersModeEnum tiersMode) {
this.tiersMode = tiersMode;
}
public SubscriptionItemCreateRequest tiers(List tiers) {
this.tiers = tiers;
return this;
}
public SubscriptionItemCreateRequest addTiersItem(ItemTier tiersItem) {
if (this.tiers == null) {
this.tiers = new ArrayList();
}
this.tiers.add(tiersItem);
return this;
}
/**
* Information of all tiers if the price is a tiered price.
* @return tiers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Information of all tiers if the price is a tiered price.")
public List getTiers() {
return tiers;
}
public void setTiers(List tiers) {
this.tiers = tiers;
}
public SubscriptionItemCreateRequest quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* Quantity of the product to which your customers subscribe.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Quantity of the product to which your customers subscribe.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public SubscriptionItemCreateRequest amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount of the price. Specify this field if you want to override the original price with a flat-fee price
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of the price. Specify this field if you want to override the original price with a flat-fee price")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public SubscriptionItemCreateRequest unitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* The unit amount of the price. Specify this field if you want to override the original price with a per-unit price.
* @return unitAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unit amount of the price. Specify this field if you want to override the original price with a per-unit price.")
public BigDecimal getUnitAmount() {
return unitAmount;
}
public void setUnitAmount(BigDecimal unitAmount) {
this.unitAmount = unitAmount;
}
public SubscriptionItemCreateRequest discountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
return this;
}
/**
* Discount amount. Specify this field if you offer an amount-based discount.
* @return discountAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Discount amount. Specify this field if you offer an amount-based discount.")
public BigDecimal getDiscountAmount() {
return discountAmount;
}
public void setDiscountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
}
public SubscriptionItemCreateRequest discountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
return this;
}
/**
* Discount percent. Specify this field if you offer a percentage-based discount.
* @return discountPercent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Discount percent. Specify this field if you offer a percentage-based discount.")
public BigDecimal getDiscountPercent() {
return discountPercent;
}
public void setDiscountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
}
public SubscriptionItemCreateRequest priceBaseInterval(PriceBaseIntervalEnum priceBaseInterval) {
this.priceBaseInterval = priceBaseInterval;
return this;
}
/**
* Specifies the base interval of the price the subscriber is subscribed to. If not provided, this field defaults to `billing_period`.
* @return priceBaseInterval
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the base interval of the price the subscriber is subscribed to. If not provided, this field defaults to `billing_period`.")
public PriceBaseIntervalEnum getPriceBaseInterval() {
return priceBaseInterval;
}
public void setPriceBaseInterval(PriceBaseIntervalEnum priceBaseInterval) {
this.priceBaseInterval = priceBaseInterval;
}
public SubscriptionItemCreateRequest overage(Overage overage) {
this.overage = overage;
return this;
}
/**
* Get overage
* @return overage
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Overage getOverage() {
return overage;
}
public void setOverage(Overage overage) {
this.overage = overage;
}
public SubscriptionItemCreateRequest uniqueToken(String uniqueToken) {
this.uniqueToken = uniqueToken;
return this;
}
/**
* Unique identifier for the price. This identifier enables you to refer to the price before the price has an internal identifier in Zuora.
* @return uniqueToken
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the price. This identifier enables you to refer to the price before the price has an internal identifier in Zuora.")
public String getUniqueToken() {
return uniqueToken;
}
public void setUniqueToken(String uniqueToken) {
this.uniqueToken = uniqueToken;
}
public SubscriptionItemCreateRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public SubscriptionItemCreateRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public SubscriptionItemCreateRequest applyDiscountTo(List applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
return this;
}
public SubscriptionItemCreateRequest addApplyDiscountToItem(ApplyDiscountToEnum applyDiscountToItem) {
if (this.applyDiscountTo == null) {
this.applyDiscountTo = new ArrayList();
}
this.applyDiscountTo.add(applyDiscountToItem);
return this;
}
/**
* Any combination of one-time, recurring, and usage.
* @return applyDiscountTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Any combination of one-time, recurring, and usage.")
public List getApplyDiscountTo() {
return applyDiscountTo;
}
public void setApplyDiscountTo(List applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
}
public SubscriptionItemCreateRequest discountLevel(DiscountLevelEnum discountLevel) {
this.discountLevel = discountLevel;
return this;
}
/**
* Specifies at what level a discount should be applied: one of account, subscription or plan.
* @return discountLevel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies at what level a discount should be applied: one of account, subscription or plan.")
public DiscountLevelEnum getDiscountLevel() {
return discountLevel;
}
public void setDiscountLevel(DiscountLevelEnum discountLevel) {
this.discountLevel = discountLevel;
}
public SubscriptionItemCreateRequest customFieldPerUnitRate(String customFieldPerUnitRate) {
this.customFieldPerUnitRate = customFieldPerUnitRate;
return this;
}
/**
* Name of the custom field that will be used to set a per unit rate under the `Pre-Rated Per Unit` charge model
* @return customFieldPerUnitRate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the custom field that will be used to set a per unit rate under the `Pre-Rated Per Unit` charge model ")
public String getCustomFieldPerUnitRate() {
return customFieldPerUnitRate;
}
public void setCustomFieldPerUnitRate(String customFieldPerUnitRate) {
this.customFieldPerUnitRate = customFieldPerUnitRate;
}
public SubscriptionItemCreateRequest customFieldTotalAmount(String customFieldTotalAmount) {
this.customFieldTotalAmount = customFieldTotalAmount;
return this;
}
/**
* Name of the custom field that will be used to set a total amount under the `Pre-Rated` charge model
* @return customFieldTotalAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the custom field that will be used to set a total amount under the `Pre-Rated` charge model ")
public String getCustomFieldTotalAmount() {
return customFieldTotalAmount;
}
public void setCustomFieldTotalAmount(String customFieldTotalAmount) {
this.customFieldTotalAmount = customFieldTotalAmount;
}
public SubscriptionItemCreateRequest priceChangePercentage(BigDecimal priceChangePercentage) {
this.priceChangePercentage = priceChangePercentage;
return this;
}
/**
* The percentage to increase or decrease the price of a termed subscription's renewal.
* @return priceChangePercentage
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The percentage to increase or decrease the price of a termed subscription's renewal.")
public BigDecimal getPriceChangePercentage() {
return priceChangePercentage;
}
public void setPriceChangePercentage(BigDecimal priceChangePercentage) {
this.priceChangePercentage = priceChangePercentage;
}
public SubscriptionItemCreateRequest priceChangeOption(PriceChangeOptionEnum priceChangeOption) {
this.priceChangeOption = priceChangeOption;
return this;
}
/**
* Applies an automatic price change when a termed subscription is renewed.
* @return priceChangeOption
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Applies an automatic price change when a termed subscription is renewed.")
public PriceChangeOptionEnum getPriceChangeOption() {
return priceChangeOption;
}
public void setPriceChangeOption(PriceChangeOptionEnum priceChangeOption) {
this.priceChangeOption = priceChangeOption;
}
public SubscriptionItemCreateRequest prepayment(SubscriptionItemPrepaymentField prepayment) {
this.prepayment = prepayment;
return this;
}
/**
* Get prepayment
* @return prepayment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SubscriptionItemPrepaymentField getPrepayment() {
return prepayment;
}
public void setPrepayment(SubscriptionItemPrepaymentField prepayment) {
this.prepayment = prepayment;
}
public SubscriptionItemCreateRequest drawdown(SubscriptionItemDrawdownField drawdown) {
this.drawdown = drawdown;
return this;
}
/**
* Get drawdown
* @return drawdown
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SubscriptionItemDrawdownField getDrawdown() {
return drawdown;
}
public void setDrawdown(SubscriptionItemDrawdownField drawdown) {
this.drawdown = drawdown;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubscriptionItemCreateRequest subscriptionItemCreateRequest = (SubscriptionItemCreateRequest) o;
return Objects.equals(this.priceId, subscriptionItemCreateRequest.priceId) &&
Objects.equals(this.subscriptionItemNumber, subscriptionItemCreateRequest.subscriptionItemNumber) &&
Objects.equals(this.description, subscriptionItemCreateRequest.description) &&
Objects.equals(this.recurring, subscriptionItemCreateRequest.recurring) &&
Objects.equals(this.startEvent, subscriptionItemCreateRequest.startEvent) &&
Objects.equals(this.startDate, subscriptionItemCreateRequest.startDate) &&
Objects.equals(this.endDate, subscriptionItemCreateRequest.endDate) &&
Objects.equals(this.tiersMode, subscriptionItemCreateRequest.tiersMode) &&
Objects.equals(this.tiers, subscriptionItemCreateRequest.tiers) &&
Objects.equals(this.quantity, subscriptionItemCreateRequest.quantity) &&
Objects.equals(this.amount, subscriptionItemCreateRequest.amount) &&
Objects.equals(this.unitAmount, subscriptionItemCreateRequest.unitAmount) &&
Objects.equals(this.discountAmount, subscriptionItemCreateRequest.discountAmount) &&
Objects.equals(this.discountPercent, subscriptionItemCreateRequest.discountPercent) &&
Objects.equals(this.priceBaseInterval, subscriptionItemCreateRequest.priceBaseInterval) &&
Objects.equals(this.overage, subscriptionItemCreateRequest.overage) &&
Objects.equals(this.uniqueToken, subscriptionItemCreateRequest.uniqueToken) &&
Objects.equals(this.customFields, subscriptionItemCreateRequest.customFields) &&
Objects.equals(this.applyDiscountTo, subscriptionItemCreateRequest.applyDiscountTo) &&
Objects.equals(this.discountLevel, subscriptionItemCreateRequest.discountLevel) &&
Objects.equals(this.customFieldPerUnitRate, subscriptionItemCreateRequest.customFieldPerUnitRate) &&
Objects.equals(this.customFieldTotalAmount, subscriptionItemCreateRequest.customFieldTotalAmount) &&
Objects.equals(this.priceChangePercentage, subscriptionItemCreateRequest.priceChangePercentage) &&
Objects.equals(this.priceChangeOption, subscriptionItemCreateRequest.priceChangeOption) &&
Objects.equals(this.prepayment, subscriptionItemCreateRequest.prepayment) &&
Objects.equals(this.drawdown, subscriptionItemCreateRequest.drawdown);
}
@Override
public int hashCode() {
return Objects.hash(priceId, subscriptionItemNumber, description, recurring, startEvent, startDate, endDate, tiersMode, tiers, quantity, amount, unitAmount, discountAmount, discountPercent, priceBaseInterval, overage, uniqueToken, customFields, applyDiscountTo, discountLevel, customFieldPerUnitRate, customFieldTotalAmount, priceChangePercentage, priceChangeOption, prepayment, drawdown);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionItemCreateRequest {\n");
sb.append(" priceId: ").append(toIndentedString(priceId)).append("\n");
sb.append(" subscriptionItemNumber: ").append(toIndentedString(subscriptionItemNumber)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" recurring: ").append(toIndentedString(recurring)).append("\n");
sb.append(" startEvent: ").append(toIndentedString(startEvent)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" tiersMode: ").append(toIndentedString(tiersMode)).append("\n");
sb.append(" tiers: ").append(toIndentedString(tiers)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" unitAmount: ").append(toIndentedString(unitAmount)).append("\n");
sb.append(" discountAmount: ").append(toIndentedString(discountAmount)).append("\n");
sb.append(" discountPercent: ").append(toIndentedString(discountPercent)).append("\n");
sb.append(" priceBaseInterval: ").append(toIndentedString(priceBaseInterval)).append("\n");
sb.append(" overage: ").append(toIndentedString(overage)).append("\n");
sb.append(" uniqueToken: ").append(toIndentedString(uniqueToken)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" applyDiscountTo: ").append(toIndentedString(applyDiscountTo)).append("\n");
sb.append(" discountLevel: ").append(toIndentedString(discountLevel)).append("\n");
sb.append(" customFieldPerUnitRate: ").append(toIndentedString(customFieldPerUnitRate)).append("\n");
sb.append(" customFieldTotalAmount: ").append(toIndentedString(customFieldTotalAmount)).append("\n");
sb.append(" priceChangePercentage: ").append(toIndentedString(priceChangePercentage)).append("\n");
sb.append(" priceChangeOption: ").append(toIndentedString(priceChangeOption)).append("\n");
sb.append(" prepayment: ").append(toIndentedString(prepayment)).append("\n");
sb.append(" drawdown: ").append(toIndentedString(drawdown)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy